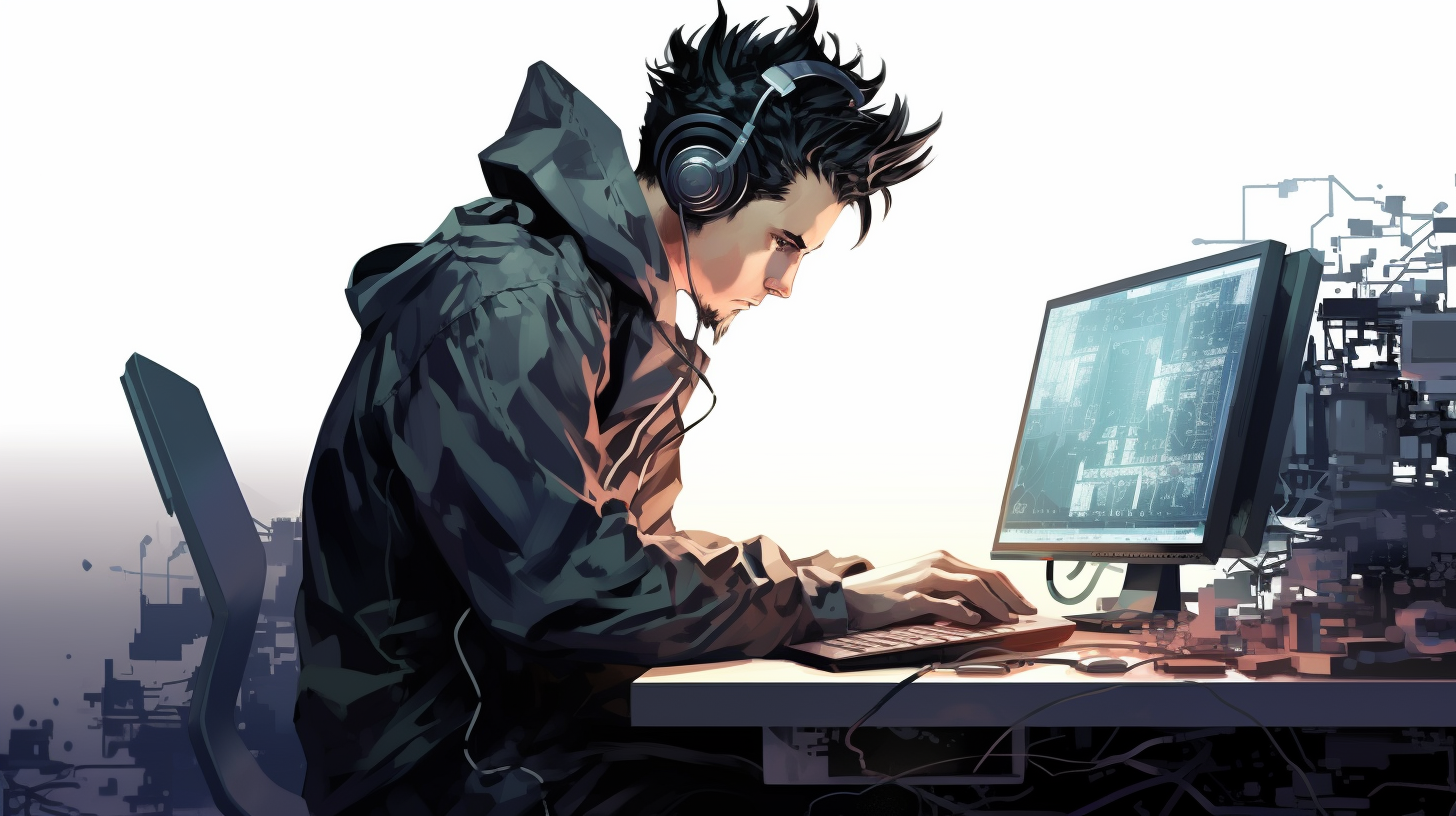
Streamlining Work with Bash Aliases
Bash aliases are a powerful feature that allow users to create shortcuts for longer commands or sequences of commands, enhancing productivity and streamlining workflows. By defining an alias, you can replace cumbersome commands with a simpler keyword that can be executed in the terminal.
At its core, an alias in Bash is a way to tell the shell, “Whenever I type this short word, execute this longer command instead.” This not only saves time but also reduces the risk of typos in frequently used commands.
To define a simple alias, you can use the following syntax:
alias shortname='long command'
For example, if you frequently use the command ls -l --color=auto
to list files in a human-readable format, you could create an alias named ll
:
alias ll='ls -l --color=auto'
After defining this alias, simply typing ll
will execute the longer command, producing a neatly formatted output.
Aliases are defined in the current session and will disappear once the terminal is closed. To make them persistent across sessions, you typically add them to your ~/.bashrc
or ~/.bash_profile
file. For example, you could append the previous alias to your .bashrc
like this:
echo "alias ll='ls -l --color=auto'" >> ~/.bashrc
After saving changes to your configuration file, you can apply them immediately by sourcing the file:
source ~/.bashrc
With aliases, you can also create shortcuts for more complex commands that include multiple options or even chains of commands connected by pipes. For instance, if you often check system uptime followed by a memory usage report, you could create an alias for that as well:
alias sysinfo='uptime; free -h'
Executing sysinfo
would yield both the system uptime and the memory usage in a single command call.
Bash aliases serve as a fundamental tool for enhancing command line efficiency. By reducing the amount of typing required for frequent tasks, they allow users to focus more on their work and less on the mechanics of command entry.
Creating Custom Aliases
Creating custom aliases in Bash is not only about convenience; it’s also about tailoring your command line interface to your specific workflow. By strategically crafting aliases, you can significantly reduce the amount of repetitive typing and streamline your interactions with the shell.
The process of creating an alias is simpler, but the real power lies in how you can customize them to fit your needs. Let’s explore some practical examples of how to create aliases that can enhance your efficiency.
Suppose you frequently use the command to change directories to a specific project folder. Instead of typing the full path every time, you can create an alias. For example, if your project is located at /home/user/projects/my_project, you could set up an alias like this:
alias proj='cd /home/user/projects/my_project'
Now, each time you want to navigate to your project directory, you simply type proj
and save several keystrokes.
Aliases can also be used to encapsulate more complex commands with multiple parameters. Imagine you often find yourself searching for a specific string within a file using grep
. Instead of repeatedly typing the full command, you can create a more user-friendly alias. For instance:
alias search='grep -r --color=auto'
With this alias, you can easily search for a term by typing search "your_term" /path/to/directory
, and it will apply the -r
option recursively while highlighting matches.
Another useful application of aliases is to simplify commands that require sudo privileges. For example, you might want to quickly update your package manager. Instead of typing out sudo apt update && sudo apt upgrade
every time, you can create a single alias:
alias update='sudo apt update && sudo apt upgrade'
Now, running update
will handle both tasks in one go, saving time and effort.
Furthermore, aliases can be grouped together to manage sets of related commands. For instance, if you have a set of commands for system maintenance, you could create a group of aliases like this:
alias clean='sudo apt autoremove && sudo apt clean' alias check='df -h && free -h'
With these aliases, you could quickly clean up your system and check disk and memory usage with simple commands: clean
and check
.
Custom aliases empower you to mold your command line experience. With a little creativity and foresight, you can reduce complexity in your daily tasks, making your workflow not only faster but also more enjoyable. Take the time to ponder about the commands you often use and consider how aliases can simplify your command line interactions.
Managing and Organizing Aliases
Once you’ve begun creating custom aliases, the next step is to manage and organize them effectively. As you accumulate more aliases, it’s vital to keep them organized to prevent clutter and improve efficiency. This organization can enhance your workflow by enabling you to quickly find and modify aliases as needed.
One of the best practices for managing your aliases is to categorize them based on their functionalities. For instance, you might have a section for system-related commands, another for development tasks, and yet another for personal shortcuts. This can be achieved by grouping related aliases together within your configuration files. For example:
# System Maintenance Aliases alias clean='sudo apt autoremove && sudo apt clean' alias update='sudo apt update && sudo apt upgrade' # Development Aliases alias serve='python -m http.server' alias npmstart='npm start' # Navigation Aliases alias proj='cd /home/user/projects/my_project' alias docs='cd /home/user/Documents'
By organizing your aliases in this way, you not only make it easier to locate them later, but you also provide a clear structure that can be readily understood by anyone else who may need to use or modify your shell configuration.
Another effective management strategy involves documenting your aliases. Adding comments next to each alias in your configuration file can clarify their purpose and usage. This documentation method can be invaluable if you return to your configuration after an extended period or if someone else needs to interpret your shell setup. A well-documented alias might look like this:
# Quick way to clean up unused packages alias clean='sudo apt autoremove && sudo apt clean' # Removes unnecessary packages
Additionally, using functions instead of aliases for more complex commands can also help in managing your command line environment. Functions allow for more flexibility and can even take parameters, making them more powerful than traditional aliases. For example, you could define a function to search for files by name:
search_file() { find . -name "*$1*" }
With this function, you can now search for files by simply typing search_file filename
, where filename
is the term you want to look for.
Moreover, consider using a separate file just for your aliases, such as ~/.bash_aliases
. This approach can keep your .bashrc
file clean and focused on environment settings. To include your aliases in your .bashrc
, simply add the following line:
if [ -f ~/.bash_aliases ]; then . ~/.bash_aliases fi
This way, any time you want to add or modify an alias, you can do it in ~/.bash_aliases
, which keeps your primary configuration file tidy.
Maintaining a well-organized collection of aliases not only enhances your productivity but also provides a clearer understanding of your command line environment. As you refine your aliases over time, take care to regularly review and update them, ensuring that they remain relevant to your current workflow. By doing so, you’ll optimize your command line experience and maintain a sense of control over your digital workspace.
Advanced Techniques for Bash Aliases
When it comes to Bash aliases, the possibilities extend far beyond mere shortcuts. Advanced techniques allow you to leverage the full power of the shell, transforming simple command substitutions into sophisticated tools that enhance interactivity and efficiency. These techniques can elevate your Bash experience by introducing dynamic functionality that adapts to your needs.
One such technique is using command substitution within aliases. This allows you to execute commands within an alias and use their output directly. For example, if you frequently need to check the current directory and list its contents, you could create an alias that combines these actions:
alias lsc='ls "$(pwd)"'
Now, when you type lsc, it will display the files in your current directory, dynamically resolving the path each time. This approach can be particularly beneficial for keeping your command streamlined while ensuring you always see the relevant files.
Another advanced technique is to utilize functions instead of aliases for more complex operations. Functions allow you to create more sophisticated scripts that can accept parameters, making them far more flexible. For instance, if you often want to copy files with a confirmation prompt, you could define the following function:
copy_with_prompt() { cp -i "$1" "$2" }
Now you can use this function by typing copy_with_prompt source_file destination_file
, and it will ask for confirmation before overwriting any existing files. This level of interactivity cannot be achieved with a simple alias.
Furthermore, you can create conditional aliases that execute different commands based on the context. For example, if you want to check whether you’re on a Linux or macOS system, you can define an alias that behaves differently depending on the operating system:
alias check_system='[[ $(uname) == "Darwin" ]] && echo "macOS" || echo "Linux"
This alias leverages the uname
command to determine the OS and provides feedback accordingly, allowing for context-sensitive behavior in your workflow.
Moreover, you can implement date and time formatting within your aliases. Suppose you regularly need to create backups with timestamps. You can define an alias that incorporates the current date and time:
alias backup='cp -r /path/to/source /path/to/destination/backup_$(date +%Y%m%d_%H%M%S)'
This command not only copies files but also appends a timestamp to the backup folder name, helping you keep track of multiple backup instances simply.
Lastly, using global aliases can help you maintain consistency across different terminal sessions. For instance, if you want your ls command to always use specific options, you can define a global alias:
alias -g G='| grep'
With this global alias, you can now use G after any command, such as ls G “search_term”, making it a powerful tool for rapid filtering of command outputs.
These advanced techniques demonstrate the flexibility of Bash aliases and functions. By integrating command substitution, functions, conditionals, date formatting, and global aliases into your workflow, you can create a command line environment that is not only efficient but also tailored to your specific needs. The more you explore these options, the more control you will have over your interaction with the shell, ultimately enhancing your productivity and command line mastery.