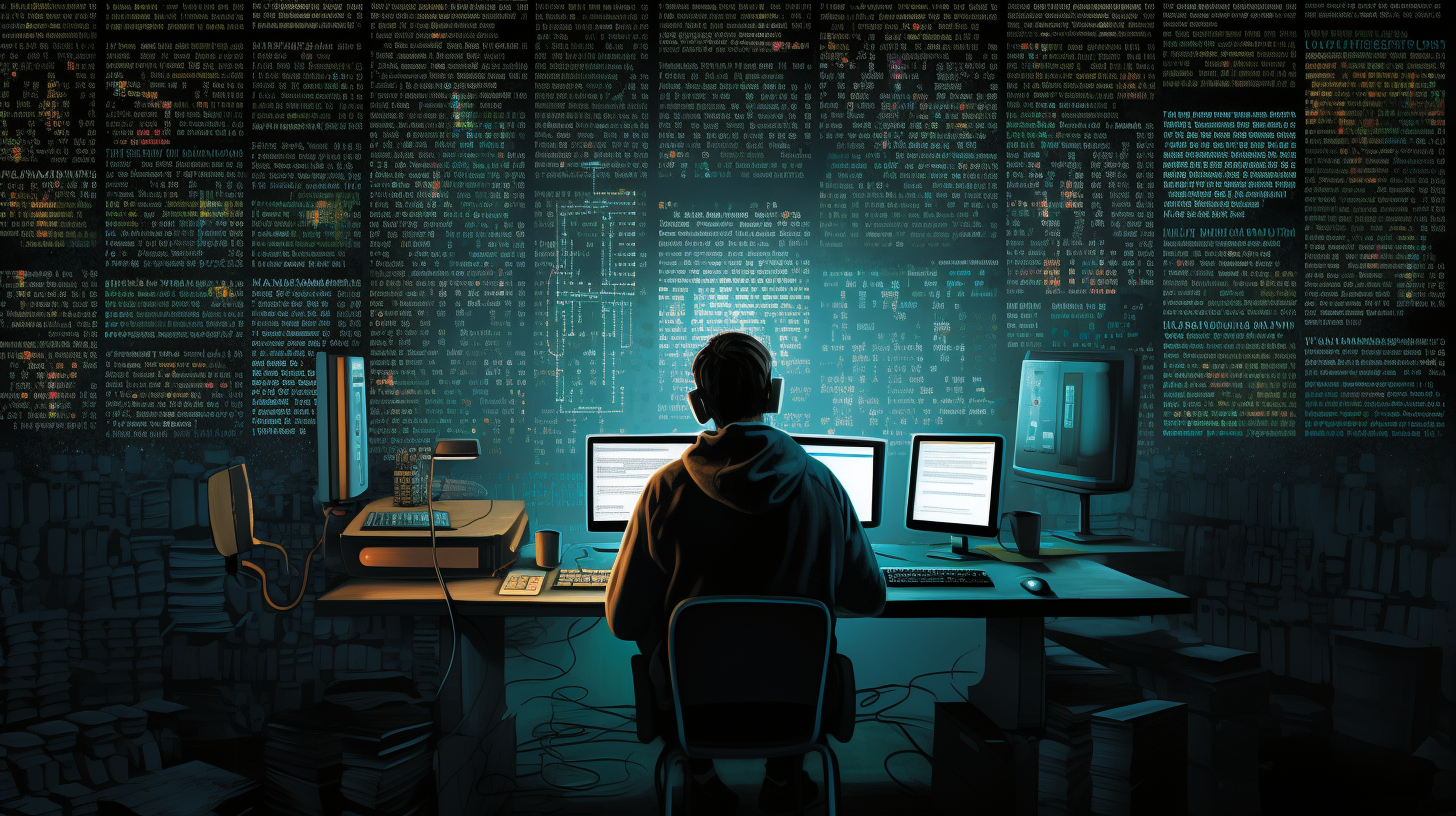
Python for Travel and Tourism: Data Analysis
Travel data, a multifaceted domain, encompasses a variety of information types sourced from numerous channels. Understanding these sources and types very important for effective data analysis in the travel and tourism industry. The data can be broadly categorized into several types, each offering distinct insights and opportunities for analysis.
1. Transactional Data: This type of data originates from bookings, purchases, and reservations made by travelers. Sources can include online travel agencies (OTAs), airline ticketing systems, and hotel management software. Transactional data typically comprises attributes such as booking dates, travel itineraries, customer profiles, and payment information. It serves as a foundational dataset for evaluating consumer behavior and operational performance.
2. Social Media Data: With the rise of social media platforms, travelers often share their experiences, reviews, and photos online. Social media data, therefore, has become a goldmine for sentiment analysis and brand perception studies. By using APIs from platforms like Twitter, Instagram, or Facebook, analysts can gather real-time data to assess public opinion regarding destinations, services, and experiences.
3. Geolocation Data: Modern smartphones and GPS technology provide geolocation data that can be leveraged to understand travel patterns and behaviors. This data can reveal the most frequented areas, peak travel times, and demographic insights based on geographical regions. Using geolocation data enables businesses to optimize their offerings and create targeted marketing campaigns.
4. Survey and Feedback Data: Surveys conducted by travel agencies, hotels, and tour operators yield rich qualitative data. Feedback from customers about their experiences helps identify strengths and weaknesses in services. Analysis of this data can be performed using various statistical methods to glean actionable insights for improving customer satisfaction.
5. Economic and Demographic Data: External data sources, such as government databases and industry reports, provide valuable context for travel data analysis. Information on economic indicators, population demographics, and tourism trends helps businesses anticipate market changes and align their strategies accordingly.
To effectively analyze travel data, it’s important to aggregate these diverse data types into a cohesive dataset. Here’s a simple example of how one might begin gathering and processing travel data using Python:
import pandas as pd # Sample data frames for transactional and survey data transactional_data = pd.DataFrame({ 'BookingID': [1, 2, 3], 'CustomerID': [101, 102, 103], 'Destination': ['Paris', 'London', 'New York'], 'Date': ['2023-07-01', '2023-07-02', '2023-07-03'] }) survey_data = pd.DataFrame({ 'CustomerID': [101, 102, 103], 'SatisfactionScore': [5, 4, 3], 'Comments': ['Loved it!', 'Great experience', 'Average service'] }) # Merging datasets to analyze customer satisfaction by destination merged_data = pd.merge(transactional_data, survey_data, on='CustomerID') print(merged_data)
This code snippet demonstrates how to create basic data structures using pandas
, a powerful Python library for data manipulation. By merging transactional data with customer feedback, one can derive insights into satisfaction levels across different destinations. Such analyses can inform marketing strategies and operational improvements.
The myriad sources and types of travel data available provide a robust foundation for data analysis in the tourism sector. A thorough understanding of these data types is essential for deriving meaningful insights that drive strategic decision-making.
Exploring Data Visualization Techniques for Tourism
Data visualization is an indispensable component of data analysis, particularly in the travel and tourism sector, where the insights derived from data can significantly influence decision-making and strategic initiatives. By employing effective visualization techniques, analysts can transform complex datasets into accessible, intuitive representations that reveal trends, patterns, and anomalies at a glance. Here we will delve into several key visualization techniques that are particularly valuable for the tourism industry.
1. Bar Charts: Bar charts are an excellent choice for comparing categories, such as the number of visitors to different destinations or the revenue generated from various services. They provide a clear visual representation of differences between discrete items. Below is a Python example using Matplotlib to create a bar chart showing the number of visitors to three popular tourist destinations.
import matplotlib.pyplot as plt # Sample data for destinations and their visitor counts destinations = ['Paris', 'London', 'New York'] visitor_counts = [1500, 1200, 1300] # Creating the bar chart plt.bar(destinations, visitor_counts, color=['blue', 'red', 'green']) plt.title('Visitor Counts to Popular Destinations') plt.xlabel('Destinations') plt.ylabel('Number of Visitors') plt.show()
2. Line Graphs: For visualizing trends over time, line graphs are particularly useful. They can effectively illustrate fluctuations in tourist arrivals or price changes over a given period. The following example demonstrates how to create a line graph depicting the trend of monthly tourist arrivals over a year.
import matplotlib.pyplot as plt import numpy as np # Sample data for monthly tourist arrivals months = np.arange(1, 13) tourist_arrivals = [100, 150, 200, 250, 300, 400, 350, 300, 450, 500, 600, 700] # Creating the line graph plt.plot(months, tourist_arrivals, marker='o') plt.title('Monthly Tourist Arrivals Over a Year') plt.xlabel('Months') plt.ylabel('Number of Arrivals') plt.xticks(months) plt.grid() plt.show()
3. Heatmaps: When analyzing geolocation data or patterns, heatmaps can provide an immediate visual cue regarding areas of high and low activity. They are particularly useful for demonstrating the density of visitors across different locations. The following snippet shows how to construct a heatmap using Seaborn, another powerful visualization library.
import seaborn as sns import pandas as pd # Sample data representing visitor density in different areas data = pd.DataFrame({ 'Area': ['Downtown', 'Uptown', 'Midtown', 'East Side', 'West Side'], 'VisitorDensity': [200, 150, 300, 250, 100] }) # Creating the heatmap heatmap_data = data.pivot("Area", "VisitorDensity", "VisitorDensity") sns.heatmap(heatmap_data, annot=True, cmap='YlGnBu') plt.title('Visitor Density Heatmap') plt.show()
4. Pie Charts: To represent proportionate data, such as the market share of various travel companies or the distribution of travel budgets, pie charts can be an effective visualization tool. However, they should be used sparingly, as they can become difficult to interpret with many categories. Here’s how to create a pie chart in Python.
# Sample data for market share of travel companies companies = ['Company A', 'Company B', 'Company C'] market_share = [30, 45, 25] # Creating the pie chart plt.pie(market_share, labels=companies, autopct='%1.1f%%', startangle=90) plt.title('Market Share of Travel Companies') plt.axis('equal') # Equal aspect ratio ensures the pie chart is circular plt.show()
Each of these visualization techniques holds the potential to convey insights in ways that raw data cannot. By implementing these strategies within Python, professionals in the travel and tourism sector can create compelling narratives from their data, facilitating informed decisions that enhance customer experiences and operational effectiveness.
Applying Python Libraries for Data Analysis in Travel
In the context of travel data analysis, Python libraries stand out as invaluable tools that streamline the process of extracting insights from complex datasets. With a rich ecosystem of libraries tailored for data manipulation, visualization, and statistical analysis, Python empowers analysts in the tourism industry to derive meaningful conclusions that can shape business strategies. Let’s explore some of the key libraries that are integral to this analytical landscape.
Pandas: This library is the cornerstone of data manipulation in Python, providing data structures like DataFrames that handle tabular data efficiently. With its intuitive syntax, analysts can easily clean, transform, and analyze large datasets. For instance, loading and processing CSV files, which are common formats for travel data, is simpler with Pandas.
import pandas as pd # Loading travel data from a CSV file travel_data = pd.read_csv('travel_data.csv') # Displaying the first few rows of the dataset print(travel_data.head()) # Cleaning the data by filling missing values travel_data.fillna(method='ffill', inplace=True)
In this code snippet, we see how simple it is to load and preprocess a dataset, making it ready for deeper analysis. The ability to handle missing data especially important in ensuring the integrity of any analysis derived from travel data.
NumPy: Often used in conjunction with Pandas, NumPy provides support for numerical operations and is particularly useful for handling large multidimensional arrays and matrices. For travel analysts, NumPy can be employed for complex calculations such as aggregating data or performing statistical analyses.
import numpy as np # Example: Calculating average spending per trip from an array of spending data spending_data = np.array([200, 300, 150, 400, 350]) average_spending = np.mean(spending_data) print(f'Average Spending per Trip: ${average_spending:.2f}')
Here, the use of NumPy simplifies the computation of averages, which can be a foundational analysis informing pricing strategies.
Matplotlib and Seaborn: These libraries are essential for data visualization. Matplotlib provides a robust framework for creating static, animated, and interactive visualizations, while Seaborn builds on Matplotlib by offering a high-level interface for attractive statistical graphics. Together, they enable analysts to present their findings in a visually engaging manner.
import matplotlib.pyplot as plt import seaborn as sns # Example: Visualizing the correlation between travel expenditure and satisfaction data = pd.DataFrame({ 'Expenditure': [200, 300, 150, 400, 350], 'Satisfaction': [5, 4, 3, 5, 4] }) # Creating a scatter plot sns.scatterplot(data=data, x='Expenditure', y='Satisfaction') plt.title('Travel Expenditure vs. Customer Satisfaction') plt.xlabel('Expenditure ($)') plt.ylabel('Satisfaction Score') plt.show()
This snippet showcases how easily one can visualize relationships between different variables within travel data, enhancing the interpretability of results.
Scikit-learn: When it comes to applying machine learning techniques, Scikit-learn is the go-to library for data analysis in travel. It provides a plethora of tools for model building, evaluation, and selection. Whether predicting customer behavior or segmenting market data, Scikit-learn facilitates sophisticated analyses that can drive strategic decisions.
from sklearn.cluster import KMeans # Sample data for clustering clustering_data = np.array([[1, 2], [1, 4], [1, 0], [4, 2], [4, 0], [4, 4]]) # Applying KMeans clustering kmeans = KMeans(n_clusters=2, random_state=0).fit(clustering_data) print(kmeans.labels_)
In this example, KMeans clustering is applied to group data points, which can be particularly useful for market segmentation or identifying travel trends.
Statsmodels: For those interested in rigorous statistical analysis, Statsmodels provides a comprehensive suite of tools for estimating statistical models. It allows analysts to conduct hypothesis testing, regression analysis, and other statistical computations, vital for making data-driven decisions in tourism.
import statsmodels.api as sm # Sample data for regression analysis X = np.array([[1, 1], [1, 2], [2, 2], [2, 3]]) y = np.array([1, 1, 2, 2]) # Adding a constant for the intercept X = sm.add_constant(X) # Fitting the regression model model = sm.OLS(y, X).fit() print(model.summary())
This snippet demonstrates how to apply ordinary least squares (OLS) regression to analyze relationships between multiple variables, a common task in assessing factors that influence travel behavior.
By using these powerful libraries, analysts can perform complex data analyses that drive insights in the travel and tourism sector. Each library serves a distinct purpose, yet when combined, they create a formidable toolkit that enhances the analytical capabilities of professionals in this field. With Python as a central component of their analytical arsenal, travel businesses can stay ahead of trends and make informed decisions that enhance customer experiences and operational efficiencies.
Case Studies: Successful Data-Driven Strategies in Tourism
In the dynamic landscape of travel and tourism, data-driven strategies have emerged as pivotal in shaping business decisions and enhancing customer experiences. A high number of case studies illustrate how various organizations have effectively utilized data analytics to optimize their operations, improve marketing strategies, and elevate overall service quality. Below are some noteworthy examples that illustrate the power of data in the travel industry.
1. Airline Revenue Management: Airlines have long recognized that optimal pricing strategies are crucial for maximizing revenue. By employing sophisticated data analytics, airlines can analyze historical booking patterns, seasonality, and customer demographics to forecast demand accurately. For instance, a leading airline implemented machine learning algorithms to analyze past ticket sales and predict future demand. The resultant pricing model adjusted fares dynamically based on real-time data, resulting in a significant increase in revenue. The following Python code demonstrates a simple linear regression model that could be used to predict ticket prices based on historical demand data.
import pandas as pd from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression # Sample data: historical sales data data = pd.DataFrame({ 'demand': [100, 150, 200, 250, 300], 'price': [150, 145, 140, 135, 130] }) # Splitting the dataset X = data[['demand']] y = data['price'] X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Fitting the linear regression model model = LinearRegression() model.fit(X_train, y_train) # Predicting prices based on demand predicted_prices = model.predict(X_test) print(predicted_prices)
2. Hotel Guest Personalization: Many hotels utilize data analytics to enhance guest personalization and improve customer satisfaction. By analyzing guest preferences, past bookings, and feedback, hotels can tailor their offerings to meet individual needs. A prominent hotel chain developed a data-driven customer relationship management (CRM) system to track guest interactions and preferences. This system enabled the hotel to personalize marketing messages, recommend services, and even adjust room features based on individual guest profiles. The following code snippet illustrates how to analyze guest feedback data to identify common themes and preferences.
import pandas as pd from collections import Counter import matplotlib.pyplot as plt # Sample guest feedback data feedback_data = pd.DataFrame({ 'GuestID': [1, 2, 3, 4, 5], 'Feedback': ['Great service', 'Loved the pool', 'Great service', 'Room was clean', 'Loved the pool'] }) # Analyzing common feedback themes feedback_counter = Counter(feedback_data['Feedback']) common_feedback = feedback_counter.most_common() # Visualizing the common feedback labels, values = zip(*common_feedback) plt.bar(labels, values) plt.title('Common Guest Feedback Themes') plt.ylabel('Frequency') plt.xticks(rotation=45) plt.show()
3. Tour Operator Market Segmentation: Tour operators are also using data analytics to segment their markets effectively. By analyzing demographic data, booking patterns, and customer preferences, they can create targeted marketing campaigns that resonate with specific audience segments. One successful case involved a tour operator that used clustering algorithms to identify distinct customer segments based on travel preferences and budget levels. This segmentation allowed them to tailor their offerings and marketing messages, ultimately leading to increased bookings. The following example demonstrates how KMeans clustering can be applied to identify customer segments.
import numpy as np from sklearn.cluster import KMeans # Sample data representing customer budgets and preferences customer_data = np.array([[2000, 1], [1500, 2], [3000, 1], [4000, 2], [3500, 1], [4500, 2]]) # Applying KMeans clustering kmeans = KMeans(n_clusters=2, random_state=0).fit(customer_data) segments = kmeans.labels_ print(segments)
4. Destination Marketing Analysis: To improve destination marketing strategies, tourism boards are using data analytics to assess traveler sentiment and behavior. By analyzing social media data and online reviews, they can gauge public perception of various destinations and adjust their marketing efforts accordingly. A particular tourism board employed sentiment analysis techniques to analyze tweets and reviews, identifying key factors that attract or deter visitors. This analysis facilitated the development of targeted marketing campaigns that addressed specific concerns and highlighted popular attractions. The following code illustrates how to use the Natural Language Toolkit (NLTK) for basic sentiment analysis on reviews.
import pandas as pd from nltk.sentiment import SentimentIntensityAnalyzer # Sample review data reviews = pd.DataFrame({ 'Review': ['Amazing experience', 'Not what I expected', 'Beautiful place', 'Will not return', 'Loved the food'] }) # Performing sentiment analysis analyzer = SentimentIntensityAnalyzer() reviews['Sentiment'] = reviews['Review'].apply(lambda x: analyzer.polarity_scores(x)['compound']) print(reviews)
Through these case studies, it’s evident that data-driven strategies can lead to significant improvements in various aspects of travel and tourism. By using the power of data analytics, organizations can optimize their operations, enhance customer experiences, and ultimately achieve greater success in a competitive marketplace.
Future Trends: The Role of Data Analytics in Travel and Tourism
As we look towards the future of travel and tourism, data analytics is poised to play an increasingly vital role in shaping the industry. With the continuous evolution of technology and the accumulation of vast amounts of data, organizations must adapt to leverage insights that can drive innovation and improve customer experiences. Here, we explore several emerging trends that highlight the significance of data analytics in the travel sector.
1. Predictive Analytics for Demand Forecasting: One of the most promising applications of data analytics in the travel industry is predictive analytics. By analyzing historical data, companies can forecast future travel demand with remarkable accuracy. This capability allows airlines, hotels, and tour operators to optimize pricing strategies, manage inventory effectively, and tailor promotional campaigns. For instance, using time series analysis and regression models can yield precise predictions about peak travel periods. The following Python code exemplifies a simple time series analysis using ARIMA to forecast future demand.
import pandas as pd from statsmodels.tsa.arima_model import ARIMA import matplotlib.pyplot as plt # Sample historical travel demand data data = pd.Series([200, 220, 250, 300, 280, 320, 360], index=pd.date_range('2023-01-01', periods=7, freq='M')) # Fitting the ARIMA model model = ARIMA(data, order=(1, 1, 1)) model_fit = model.fit(disp=0) # Forecasting future demand forecast = model_fit.forecast(steps=3)[0] print('Forecasted Demand:', forecast) # Visualizing the forecast plt.plot(data.index, data, label='Historical Demand') plt.plot(pd.date_range('2023-08-01', periods=3, freq='M'), forecast, label='Forecasted Demand', color='red') plt.legend() plt.show()
2. Enhanced Personalization through Machine Learning: As customer expectations evolve, personalization is becoming a key competitive differentiator in travel. Machine learning algorithms can analyze customer data to identify preferences and behaviors, allowing travel companies to offer tailored recommendations and personalized experiences. For example, hotels can use collaborative filtering techniques to suggest amenities and services that align with individual guest profiles. Here’s a simple implementation using collaborative filtering for hotel recommendations.
from sklearn.metrics.pairwise import cosine_similarity import pandas as pd # Sample guest preference data preferences = pd.DataFrame({ 'GuestID': [1, 2, 3], 'Pool': [1, 0, 1], 'Spa': [0, 1, 0], 'Gym': [1, 1, 0] }) # Calculating cosine similarity between guests similarity_matrix = cosine_similarity(preferences.iloc[:, 1:]) print('Similarity Matrix:n', similarity_matrix)
3. Real-Time Analytics for Dynamic Operations: The ability to analyze data in real-time is becoming crucial for tourism businesses that need to react quickly to changing conditions. Whether it’s adjusting flight schedules due to weather disruptions or modifying hotel availability based on last-minute bookings, real-time analytics empowers organizations to make informed operational decisions. Streamlining operations through real-time data can significantly enhance efficiency and customer satisfaction.
4. Sustainability Analytics: As environmental concerns become more pronounced, travel companies are increasingly focusing on sustainability through data analytics. By tracking carbon footprints, resource consumption, and waste generation, organizations can identify areas for improvement and implement sustainable practices. Data analytics can also aid in promoting eco-friendly travel options, allowing customers to make informed choices. A simple example is calculating the carbon emissions of different travel modes:
# Sample data for travel modes and their emissions modes = {'Airplane': 0.2, 'Car': 0.12, 'Train': 0.05} # emissions in kg CO2 per km distance = 500 # in km # Calculating emissions for each mode for mode, emission in modes.items(): total_emissions = emission * distance print(f'Total CO2 emissions for {mode} over {distance} km: {total_emissions} kg')
5. Integration of Augmented and Virtual Reality: As technology advances, the integration of augmented reality (AR) and virtual reality (VR) into travel analytics is gaining traction. These technologies can provide immersive experiences that enhance customer engagement while enabling companies to gather data on user interactions and preferences. Analyzing this interaction data will provide insights into consumer behavior in ways that traditional analytics cannot.
As the travel industry embraces these emerging trends, the role of data analytics will undoubtedly expand, driving innovation and fostering a deeper connection between companies and their customers. By using the power of data, travel organizations can navigate the complexities of the modern landscape and thrive in a competitive market.