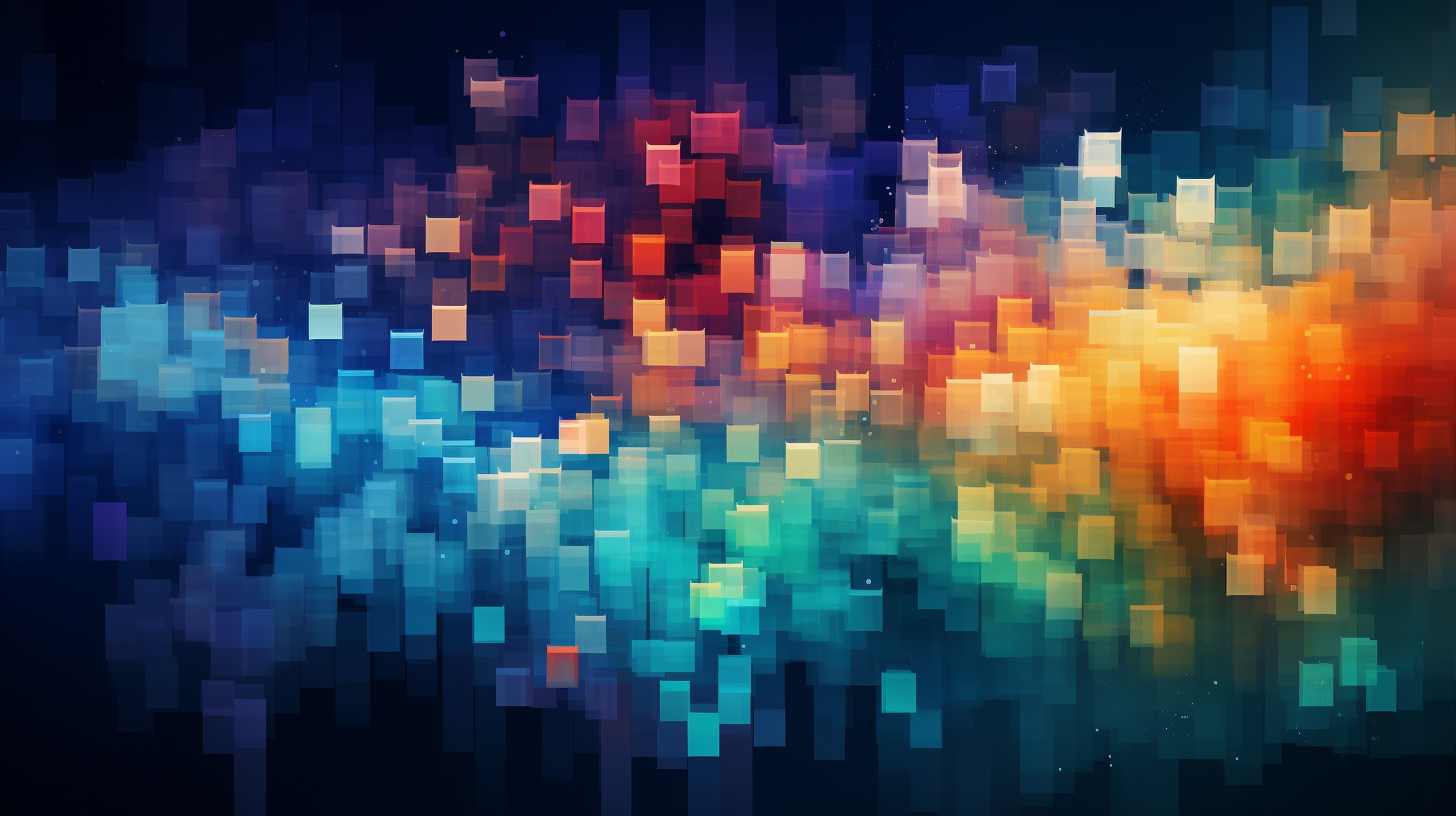
SQL and NoSQL: Working Together
Databases are fundamental components of modern applications, and understanding the two primary types—SQL (Structured Query Language) and NoSQL (Not Only SQL)—is crucial for developers and data architects alike. SQL databases are traditionally relational databases, characterized by their structured data format, which is organized into tables with predefined schemas. This structure allows for powerful querying capabilities and strict data integrity through the use of ACID (Atomicity, Consistency, Isolation, Durability) properties.
In contrast, NoSQL databases emerged in response to the limitations of SQL databases in handling vast amounts of unstructured data. They’re designed to provide flexibility in terms of data modeling and scalability. NoSQL encompasses a variety of database types, including document stores, key-value stores, wide-column stores, and graph databases. Each type caters to specific data storage needs and usage scenarios, allowing for a more agile development process.
SQL databases utilize a standardized language for defining and manipulating data, which facilitates complex queries across multiple tables using joins. This makes them perfect for applications where relationships between data points are crucial. For example, in an e-commerce application, a SQL database might contain separate tables for customers, orders, and products, allowing for detailed reporting and analytics:
SELECT customers.name, orders.order_date, products.product_name FROM customers JOIN orders ON customers.id = orders.customer_id JOIN products ON orders.product_id = products.id WHERE orders.order_date >= '2023-01-01';
NoSQL databases, on the other hand, offer a more flexible schema, allowing for the storage of various types of data formats without requiring a fixed structure. This flexibility is particularly advantageous for applications that need to scale horizontally across distributed systems. For instance, a document store like MongoDB allows you to store data in JSON-like documents, making it easier to handle hierarchical data structures:
db.products.insertOne({ name: "Wireless Mouse", price: 29.99, categories: ["electronics", "accessories"], stock: { warehouse: 150, store: 75 } });
Understanding the fundamental characteristics of SQL and NoSQL databases enables developers to make informed choices based on the specific needs of their applications. While SQL databases excel in scenarios where data relationships are paramount, NoSQL databases shine in flexibility and scalability, particularly in handling large volumes of diverse data. The decision between the two often involves evaluating the specific requirements of a project, including data consistency needs, the frequency of schema changes, and the performance expectations of the application.
Key Differences Between SQL and NoSQL
When comparing SQL and NoSQL databases, several critical differences emerge, each influencing how data is managed, accessed, and manipulated in various application scenarios. One of the primary distinctions lies in data structure. SQL databases are inherently structured, relying on a fixed schema that defines data types and relationships. This rigidity promotes data integrity but can hinder adaptability in dynamic environments. In contrast, NoSQL databases embrace schema-less design, allowing developers to store data in a more fluid manner, which can evolve over time without necessitating extensive migrations.
Another significant difference is the way both types of databases handle relationships between datasets. SQL databases leverage foreign keys and joins to maintain relationships, which can be queried efficiently using SQL’s powerful JOIN operations. This approach promotes relational integrity but can lead to complexity and performance issues as the size of the data grows. For instance, in a relational database, querying related data from multiple tables can be achieved as follows:
SELECT employees.name, departments.department_name FROM employees JOIN departments ON employees.department_id = departments.id;
Conversely, NoSQL databases often forgo traditional joins in favor of embedded documents or denormalized data storage. For example, in a document store, an employee’s department information might be embedded directly within their employee document. This design can greatly enhance read performance and reduce the complexity of queries, albeit at the expense of potential data redundancy:
{ "name": "Neil Hamilton", "department": { "department_name": "Engineering", "location": "Building A" } }
Scalability also presents a stark contrast between SQL and NoSQL databases. SQL databases traditionally scale vertically, meaning that to handle increased loads, one must upgrade the existing server’s hardware. This can become costly and impractical as demand grows. On the other hand, NoSQL databases are designed to scale horizontally, allowing for the addition of more servers to distribute the data load. This approach is particularly effective for applications requiring high availability and fault tolerance, such as social media platforms or real-time analytics.
Furthermore, the consistency models employed by SQL and NoSQL databases differ significantly. SQL databases generally adhere to ACID principles, ensuring that transactions are reliably processed in a manner that preserves data integrity. This strict adherence makes SQL ideal for applications where accuracy is paramount, such as financial systems. In contrast, many NoSQL databases adopt eventual consistency models, prioritizing availability and partition tolerance over immediate consistency. This paradigm allows for more responsive applications but can lead to brief periods of inconsistency, which may not be fits everyone use cases.
The key differences between SQL and NoSQL databases revolve around data structure, relationship handling, scalability, and consistency models. Understanding these distinctions is essential for architects and developers when selecting the appropriate database technology for their applications, ensuring that the chosen solution aligns with the project’s specific requirements and goals.
Use Cases for SQL Databases
SQL databases are particularly well-suited for a variety of use cases that demand structured data, complex queries, and robust transaction handling. One of the most prominent applications of SQL databases is in transactional systems, such as banking and financial applications. These systems require strict adherence to ACID properties to ensure that all transactions are processed reliably. For instance, a banking application might need to execute a transfer of funds between accounts, ensuring that both the debit and credit operations succeed or fail together. This could be represented in SQL as follows:
BEGIN; UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; UPDATE accounts SET balance = balance + 100 WHERE account_id = 2; COMMIT;
In addition to financial applications, SQL databases excel in managing Inventory Management Systems. These systems often require maintaining a detailed inventory of products, suppliers, and sales transactions. SQL’s relational capabilities enable complex queries that can track stock levels, generate sales reports, and analyze supplier performance effectively. A query to retrieve low-stock items from an inventory database could look like this:
SELECT products.product_name, inventory.stock_level FROM products JOIN inventory ON products.id = inventory.product_id WHERE inventory.stock_level < 20;
Customer Relationship Management (CRM) systems also benefit from SQL databases. They typically require the organization of customer data, interactions, and sales activities in a way that allows for detailed reporting and analytics. SQL’s ability to perform complex joins and aggregations is essential for generating insights, such as analyzing customer purchasing patterns or segmenting customers based on their interactions. For example, retrieving a summary of sales by customer would look like this:
SELECT customers.name, SUM(orders.total_amount) AS total_spent FROM customers JOIN orders ON customers.id = orders.customer_id GROUP BY customers.name ORDER BY total_spent DESC;
Another critical area where SQL databases shine is in Enterprise Resource Planning (ERP) solutions. These systems integrate various business processes, requiring a centralized repository of data that can be accessed and manipulated across departments. SQL’s structured data model allows for seamless integration of finance, human resources, and supply chain management data, facilitating cross-departmental reporting and decision-making. An example of querying employee data along with their respective departments in an ERP system might be:
SELECT employees.name, departments.department_name, employees.salary FROM employees JOIN departments ON employees.department_id = departments.id WHERE employees.salary > 60000;
SQL databases are indispensable in scenarios that demand rigorous data integrity, complex relationship handling, and sophisticated querying capabilities. Their structured nature, combined with ACID compliance, makes them the go-to choice for applications where data accuracy and consistency are critical. As industries evolve and new requirements emerge, the role of SQL databases remains central to managing structured data across various domains.
Use Cases for NoSQL Databases
NoSQL databases cater to a diverse range of use cases, particularly in scenarios that demand flexibility, scalability, and performance with unstructured or semi-structured data. One of the most prominent applications of NoSQL databases is in content management systems (CMS). Given that content can vary widely in format—from articles and images to videos and user-generated content—NoSQL databases allow developers to store and retrieve this content without the constraints of a rigid schema. For instance, in a document-oriented database like MongoDB, you can store a blog post in a JSON document as follows:
{ "title": "Exploring the Cosmos", "author": "Jane Doe", "content": "The universe is vast and full of mysteries...", "tags": ["space", "science", "exploration"], "comments": [ { "user": "User1", "comment": "Fascinating read!", "date": "2023-09-20" }, { "user": "User2", "comment": "I want to learn more!", "date": "2023-09-21" } ] }
This flexibility allows for rapid development and iteration, enabling content managers to adapt to changing requirements without complex migrations.
Another significant application of NoSQL databases is in big data analytics. With the explosion of data generated by user interactions, IoT devices, and social media, organizations need systems that can efficiently handle massive volumes of data. NoSQL databases, especially those optimized for analytical processing, like Apache Cassandra or HBase, can store vast amounts of data across distributed systems. For example, a business might use Cassandra to track user activity logs in real-time:
CREATE TABLE user_activity ( user_id UUID, event_time TIMESTAMP, event_type TEXT, PRIMARY KEY (user_id, event_time) );
This schema enables efficient querying of user activities over time, allowing companies to analyze behavior patterns and generate insights for targeted marketing strategies.
NoSQL databases also play an important role in applications requiring high availability and fault tolerance, such as social media platforms and real-time messaging applications. These applications often handle unpredictable spikes in traffic and need to maintain performance under load. The decentralized nature of many NoSQL databases allows them to distribute data across multiple nodes, ensuring that even if one node fails, the system remains operational. For instance, a key-value store like Redis can be utilized for caching user sessions in a web application:
SET session:12345 "user_data_goes_here" EXPIRE session:12345 3600
This example illustrates how NoSQL databases can quickly store and retrieve session data, significantly improving response times for users interacting with a web application.
Furthermore, NoSQL databases are particularly well-suited for managing data in applications that require rapid iteration and deployment cycles, such as agile development environments. As development teams adopt DevOps practices and continuous integration/continuous deployment (CI/CD) pipelines, the ability to modify data models easily becomes imperative. For example, developers can introduce new fields to user profiles in a document store without downtime or complex migrations, allowing for seamless feature enhancements:
{ "user_id": "12345", "name": "Alice", "email": "[email protected]", "preferences": { "newsletter": true, "notifications": false } }
This agility in data management allows teams to respond quickly to user feedback and evolving business requirements.
NoSQL databases excel in use cases where data variety, speed, and scalability are critical. By accommodating diverse data types and structures, they empower organizations to innovate rapidly while efficiently managing vast amounts of information.
Integrating SQL and NoSQL: Best Practices
Integrating SQL and NoSQL databases in a single application can provide a powerful approach that leverages the strengths of both technologies. This hybrid model allows developers to take advantage of SQL’s strong consistency and transactional support while also benefiting from NoSQL’s flexibility and scalability. To achieve a successful integration, several best practices should be considered.
First and foremost, it’s essential to clearly define the use cases for each database type within the application. SQL databases are best suited for structured data that requires complex queries and strong consistency, such as financial transactions or inventory management. Meanwhile, NoSQL databases excel in scenarios where unstructured or semi-structured data is prevalent, offering high performance for large volumes of data and accommodating rapid changes in schema. By identifying which data fits best into each system, developers can streamline workflows and optimize data access patterns.
When integrating both systems, a common approach is to use an application layer that abstracts the underlying database interactions. This layer can provide a unified API for the application while handling the specifics of data storage and retrieval in each database. For instance, an application might use a SQL database to manage user accounts and authentication while employing a NoSQL database to store user-generated content like comments and posts. This separation allows for clear responsibilities and performance optimization:
SELECT * FROM users WHERE username = 'johndoe';
In this scenario, user authentication data would reside in the SQL database, while user comments could be stored as documents in a NoSQL database:
{ "user_id": "12345", "comment": "Great article!", "timestamp": "2023-09-20T12:34:56Z" }
Another critical aspect of integrating SQL and NoSQL databases is ensuring data consistency across both systems. While SQL databases typically adhere to ACID properties, NoSQL databases may employ eventual consistency. To mitigate potential data inconsistency issues, developers can implement a change data capture (CDC) mechanism, which monitors changes in the SQL database and propagates those changes to the NoSQL database in real time. This approach ensures that both systems stay in sync and reduces the risk of discrepancies.
Moreover, using data replication strategies can also be beneficial. In this approach, data can be duplicated across both databases to improve read performance and availability. For example, frequently accessed user profiles could be stored in both the SQL database for transactional integrity and the NoSQL database for quick retrieval. This setup allows for efficient reads while maintaining the ability to perform complex transactions on the SQL side:
SELECT * FROM users WHERE user_id = '12345';
{ "user_id": "12345", "name": "Alice", "email": "[email protected]" }
Additionally, monitoring and performance tuning are vital for a successful integration of SQL and NoSQL databases. Each database type has its own performance metrics and tuning parameters, and monitoring tools should be employed to track system performance, latency, and error rates. Setting up alerts for performance degradation allows teams to proactively address issues before they impact users.
Finally, training and documentation are essential for teams working with a hybrid database architecture. Developers should be familiar with the respective query languages and data models of both SQL and NoSQL databases. Adequate documentation about the integration architecture, data flow, and best practices will also help ensure smooth collaboration and support ongoing maintenance.
By following these best practices, organizations can successfully integrate SQL and NoSQL databases, using the unique strengths of each to build robust, scalable, and flexible applications that meet the diverse needs of their users.
Future Trends in Database Technologies
The landscape of database technologies is continually evolving, driven by the increasing demand for more efficient, scalable, and versatile data management solutions. As applications grow in complexity and the volume of data increases, the focus is shifting towards innovative database architectures that can better serve the diverse needs of modern applications. One of the most significant trends is the rise of multi-model databases, which provide the flexibility to handle different data types and structures within a single unified platform.
Multi-model databases allow developers to work with relational, document, graph, and key-value data models all within the same system, eliminating the need to maintain separate databases for different data types. This approach not only simplifies the architecture but also enhances data access and manipulation efficiency. An example of a multi-model database is ArangoDB, which enables queries across various data models using a single query language, thus streamlining the development process.
FOR user IN users LET posts = (FOR post IN posts FILTER post.user_id == user._id RETURN post) RETURN {user: user, posts: posts}
Another notable trend is the increased adoption of serverless databases, which abstract away the infrastructure management responsibilities from developers. This model allows developers to focus solely on application logic while the database automatically scales in response to demand. Such databases, like Amazon Aurora Serverless or Firebase, provide dynamic scaling capabilities and reasonable investment data storage solutions. In this paradigm, resource allocation is optimized based on actual usage patterns, thereby reducing operational costs while enhancing performance.
Artificial Intelligence (AI) and Machine Learning (ML) are also making significant inroads into database technology. Enhanced algorithms for data indexing, query optimization, and anomaly detection are being integrated into database systems, enabling smarter data management. For instance, AI-based query optimization can analyze historical query patterns and predict the most efficient execution plans, significantly improving query performance:
EXPLAIN SELECT * FROM sales WHERE region = 'North America' AND sale_date >= '2023-01-01';
Additionally, the integration of AI-driven analytics capabilities within databases allows organizations to derive insights more rapidly, making data-driven decision-making a streamlined process.
As organizations continue to embrace cloud-native architectures, the trend towards cloud databases is also gaining momentum. Cloud databases offer scalability, accessibility, and reduced infrastructure overhead. They enable organizations to deploy databases in the cloud at an unprecedented scale and with minimal operational burden. Solutions such as Google Cloud Spanner or Azure Cosmos DB exemplify how cloud databases can provide global scalability and high availability while supporting multiple consistency models.
Moreover, the concept of data mesh is emerging as an architectural approach to managing data at scale. This decentralized approach advocates for treating data as a product, promoting cross-functional teams to own their domain-specific data and manage it independently. By doing so, organizations can enhance agility and responsiveness to changing data needs, allowing for faster delivery of data-driven insights.
With the rapid pace of technological advancement, the future of database technologies will likely be characterized by a blend of these innovations. As the demand for agility, scalability, and performance in data handling increases, the shift towards more integrated, intelligent, and cloud-native solutions will shape the way developers and organizations approach data management, ensuring they remain competitive in an ever-evolving digital landscape.