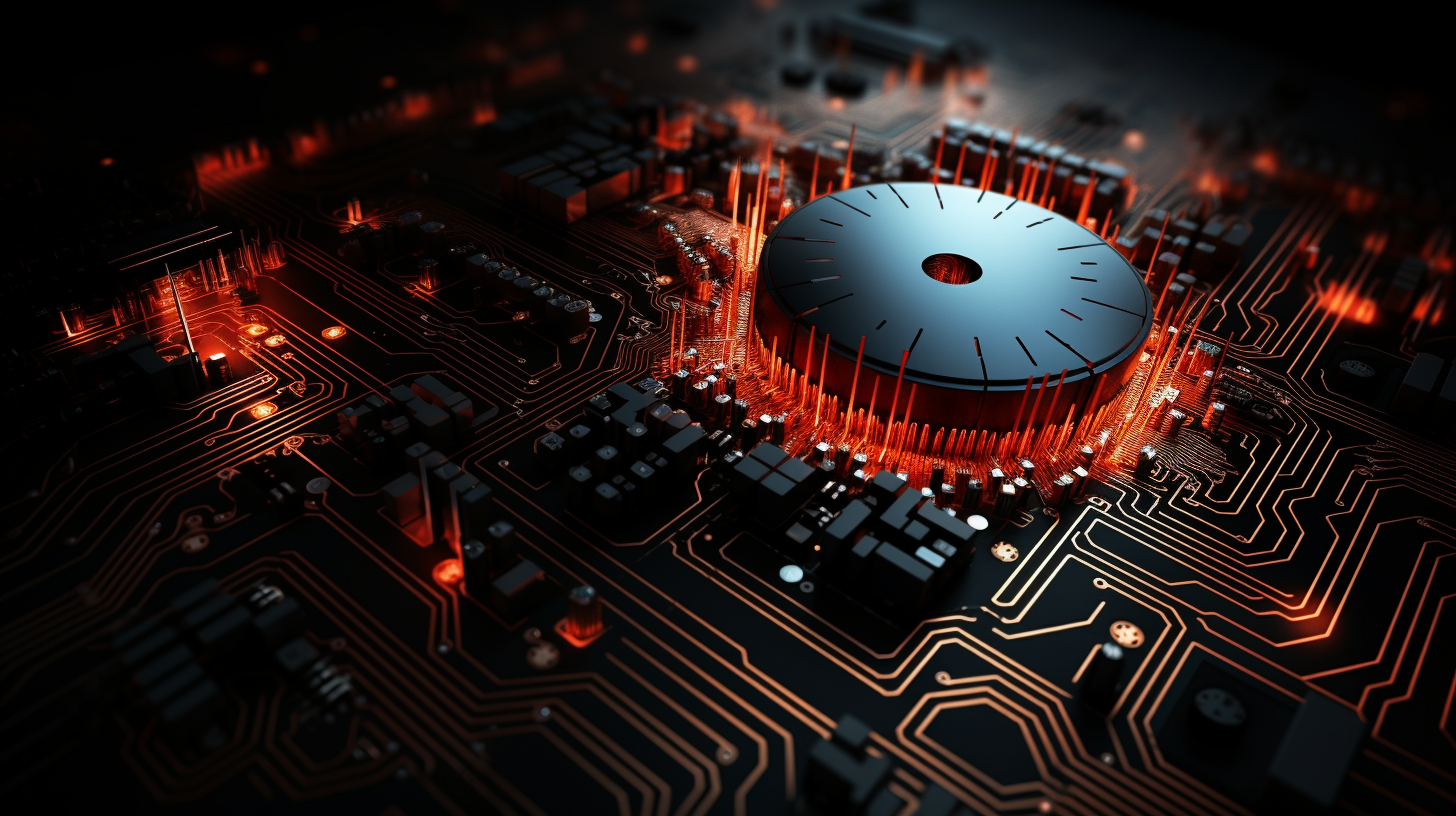
JavaScript for Digital Signage and Displays
Digital signage technology has transformed the way information is communicated in various environments, from retail to transportation. At its core, digital signage utilizes electronic displays to present multimedia content, such as images, videos, and text, to an audience. The integration of JavaScript enhances the interactivity and dynamism of these displays, allowing developers to create more engaging user experiences.
Understanding how digital signage works involves recognizing a few key components: the display hardware, the content management system (CMS), and the network infrastructure. The display hardware can range from LED screens to projectors, while the CMS is responsible for scheduling and managing the content that is shown on these displays.
JavaScript plays a significant role in connecting these components. It can be used to dynamically update the content displayed on screens based on user interaction, time of day, or even external data sources. The ability to manipulate the Document Object Model (DOM) with JavaScript allows developers to create visually appealing and interactive interfaces.
For instance, think a retail environment where promotional videos need to be updated frequently. Using JavaScript, developers can fetch new video content from a server and update the display in real time without requiring a full reload. That is done using AJAX calls to fetch data asynchronously, minimizing downtime and optimizing user engagement.
fetch('https://api.example.com/latest-promo') .then(response => response.json()) .then(data => { const videoElement = document.getElementById('promo-video'); videoElement.src = data.videoUrl; videoElement.play(); }) .catch(error => console.error('Error fetching promo video:', error));
Furthermore, understanding the network infrastructure especially important for digital signage. Content is often delivered over local networks or the internet, meaning that the performance and reliability of these networks directly affect the viewer’s experience. JavaScript can be employed to monitor network conditions and adjust content delivery accordingly. For example, if a network lag is detected, JavaScript can switch to lower-resolution content to ensure smooth playback.
The intersection of digital signage technology and JavaScript creates a powerful platform for delivering dynamic content. By using the capabilities of JavaScript, developers can ensure that digital signage solutions are not only visually compelling but also responsive to the needs of the audience.
Key JavaScript Libraries for Digital Displays
In the sphere of digital signage, the choice of JavaScript libraries can significantly accelerate development and enhance functionality. Several libraries stand out for their features tailored to the unique requirements of display content management and real-time updates. These libraries can help developers create more engaging experiences without the need to reinvent the wheel.
D3.js is one of the most powerful libraries for data-driven documents. It allows developers to bind arbitrary data to a Document Object Model (DOM) and apply data-driven transformations to the document. That’s particularly useful for digital signage that needs to visualize data such as sales figures, traffic statistics, or social media trends. For example, a digital display could utilize D3.js to create dynamic visualizations that update in real-time based on incoming data:
const data = [10, 20, 30, 40, 50]; const svg = d3.select('svg'); const width = +svg.attr('width'); const height = +svg.attr('height'); const x = d3.scaleBand().domain(data.map((d, i) => i)).range([0, width]).padding(0.1); const y = d3.scaleLinear().domain([0, d3.max(data)]).range([height, 0]); svg.selectAll('.bar') .data(data) .enter().append('rect') .attr('class', 'bar') .attr('x', (d, i) => x(i)) .attr('y', d => y(d)) .attr('width', x.bandwidth()) .attr('height', d => height - y(d));
Three.js is another library worth mentioning, particularly for 3D graphics. In scenarios where a digital display aims to captivate viewers with three-dimensional content, Three.js enables developers to create stunning visual effects and animations. This can enhance the aesthetic appeal of digital signage, especially in environments like malls or exhibitions where catching the eye of passersby is important.
const scene = new THREE.Scene(); const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000); const renderer = new THREE.WebGLRenderer(); renderer.setSize(window.innerWidth, window.innerHeight); document.body.appendChild(renderer.domElement); const geometry = new THREE.BoxGeometry(); const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 }); const cube = new THREE.Mesh(geometry, material); scene.add(cube); camera.position.z = 5; function animate() { requestAnimationFrame(animate); cube.rotation.x += 0.01; cube.rotation.y += 0.01; renderer.render(scene, camera); } animate();
jQuery, though older, remains relevant due to its simplicity and ease of use. It simplifies DOM manipulation, which is a fundamental aspect of updating content on digital displays. For example, jQuery can be used to easily switch out videos, images, or text based on user input or scheduled events:
$('#update-button').on('click', function() { $('#display-area').fadeOut(500, function() { $(this).text('New Content Loaded!').fadeIn(500); }); });
Lastly, React.js and Vue.js have gained popularity for building user interfaces, especially when a digital signage solution requires complex state management and reactivity. Both libraries allow developers to create reusable components that can be easily updated in response to real-time data changes, ensuring that the displayed information remains current and relevant.
Using these libraries can dramatically reduce development time while enhancing the capabilities of digital signage solutions. By choosing the right tools, developers can create systems that are not only efficient but also capable of delivering rich, interactive user experiences that captivate audiences and effectively communicate messages in a variety of environments.
Creating Dynamic Content with JavaScript
Creating dynamic content with JavaScript is essential for modern digital signage solutions. The ability to change what is displayed on a screen in real time creates a more engaging experience for viewers. This flexibility can be leveraged to respond to various triggers such as user interactions, updates from external data sources, or even scheduled content changes. Understanding how to effectively implement these dynamic updates is key to maximizing the potential of digital displays.
One common approach is to utilize event listeners in JavaScript to detect user interactions. For example, in a retail setting, a touch screen display might allow users to browse through product categories. By using JavaScript to listen for user clicks or touches, the content displayed can be updated accordingly. Here’s a simple example that demonstrates this concept:
document.querySelectorAll('.category-button').forEach(button => { button.addEventListener('click', function() { const categoryId = this.dataset.categoryId; // Assuming each button has a data-category-id attribute loadCategoryContent(categoryId); }); }); function loadCategoryContent(categoryId) { fetch(`https://api.example.com/categories/${categoryId}`) .then(response => response.json()) .then(data => { const contentArea = document.getElementById('content-area'); contentArea.innerHTML = data.htmlContent; // Update the content area with new content }) .catch(error => console.error('Error loading category content:', error)); }
This code snippet sets up an event listener for each category button. When a button is clicked, it fetches the corresponding content from an API and updates the display area with that content. This kind of interactivity is what makes digital signage truly engaging.
In addition to user interactions, dynamic content can also be driven by external data sources. For instance, if a digital signage solution needs to display live updates such as news headlines or social media feeds, JavaScript can be used to poll these sources at regular intervals. Here’s an example of how one might implement a regularly updating news ticker:
function updateNewsTicker() { fetch('https://api.example.com/latest-news') .then(response => response.json()) .then(data => { const ticker = document.getElementById('news-ticker'); ticker.innerHTML = ''; // Clear the existing news data.articles.forEach(article => { const item = document.createElement('div'); item.textContent = article.title; // Assume each article has a title property ticker.appendChild(item); }); }) .catch(error => console.error('Error fetching news:', error)); } // Update the ticker every 10 seconds setInterval(updateNewsTicker, 10000);
In this example, the JavaScript code fetches the latest news from an API every ten seconds. The news ticker updates dynamically, providing viewers with fresh content without requiring any manual input or page reloads.
Another powerful feature of JavaScript is the ability to create animations that enhance the visual allure of the content. CSS animations can be combined with JavaScript to create smooth transitions when updating content. For instance, when new content is loaded, it can fade in while the old content fades out, creating a seamless user experience:
function loadContentWithAnimation(newContent) { const contentArea = document.getElementById('content-area'); contentArea.classList.add('fade-out'); setTimeout(() => { contentArea.innerHTML = newContent; // Update content after fade out contentArea.classList.remove('fade-out'); contentArea.classList.add('fade-in'); }, 500); // Match this timeout with the duration of the fade-out animation }
In this snippet, we assume that appropriate CSS classes for fading effects are already defined. The JavaScript code handles the logic for fading out the old content, updating the content area, and then fading in the new content. This kind of animation can significantly improve user perception of the content change, making it feel more fluid and professional.
By incorporating these techniques, developers can create digital signage this is not only dynamic but also interactive and visually appealing. The strategic use of JavaScript allows for a more responsive content management system, catering to the needs of the audience and enhancing engagement. As digital signage continues to evolve, mastering these dynamic content creation strategies will prove invaluable for developers looking to push the boundaries of what’s possible in this space.
Integrating APIs for Real-Time Data
Integrating APIs for real-time data is a critical aspect of modern digital signage, allowing displays to present fresh, relevant information that can adapt to changing circumstances. JavaScript serves as the glue that binds these disparate data sources to the dynamic visual outputs on digital displays. By tapping into various APIs, developers can enrich their content with live data, creating a more engaging user experience. This integration can range from fetching simple text updates to complex data visualizations, all driven by the capabilities of JavaScript.
One common use case is displaying real-time information such as weather updates, stock prices, or social media feeds. For example, ponder a digital signage solution that provides live weather updates for a public transport station. By using a weather API, JavaScript can continuously fetch and display the latest weather conditions. Here’s how you can implement that:
fetch('https://api.weatherapi.com/v1/current.json?key=YOUR_API_KEY&q=London') .then(response => response.json()) .then(data => { const weatherDisplay = document.getElementById('weather-display'); weatherDisplay.innerHTML = `Current temperature in ${data.location.name}: ${data.current.temp_c}°C`; }) .catch(error => console.error('Error fetching weather data:', error));
This code snippet fetches data from a weather API and updates the display with the current temperature and the location. The use of promises ensures that the display is updated only after the data has been successfully retrieved, maintaining a responsive interface.
Another powerful application of API integration is for displaying social media feeds. For instance, a digital signage solution could showcase the latest tweets or Instagram posts relevant to a brand. By using the Twitter API or Instagram Graph API, developers can pull in user-generated content that promotes engagement and interaction. Here’s a basic example of fetching tweets:
fetch('https://api.twitter.com/2/tweets?ids=YOUR_TWEET_IDS', { headers: { 'Authorization': `Bearer YOUR_ACCESS_TOKEN` } }) .then(response => response.json()) .then(data => { const tweetsDisplay = document.getElementById('tweets-display'); tweetsDisplay.innerHTML = ''; // Clear existing tweets data.data.forEach(tweet => { const tweetElement = document.createElement('div'); tweetElement.textContent = tweet.text; // Display tweet text tweetsDisplay.appendChild(tweetElement); }); }) .catch(error => console.error('Error fetching tweets:', error));
In this example, the developer retrieves tweets based on specified tweet IDs. The resulting tweets are then displayed in a designated area of the digital signage. Note the use of an authorization token, which is necessary for accessing the Twitter API.
Real-time data integration is not just about fetching data but also about handling updates efficiently. Using techniques such as long polling or WebSocket connections allows for instant updates without the need for repeated fetch calls. For example, WebSockets can be used to receive real-time notifications, which can be particularly useful for urgent announcements in environments like airports or retail locations:
const socket = new WebSocket('wss://your-websocket-url'); socket.onmessage = function(event) { const message = JSON.parse(event.data); const alertsDisplay = document.getElementById('alerts-display'); alertsDisplay.innerHTML += `${message.alert}`; };
In this WebSocket example, the JavaScript listens for incoming messages from the server. When a new message arrives, it updates the alert display area dynamically, ensuring that viewers receive timely information.
Integrating APIs into digital signage solutions goes beyond merely fetching data; it requires careful consideration of how that data is presented and updated. Using JavaScript effectively in this context allows developers to create rich, interactive experiences that keep audiences informed and engaged. Furthermore, with the increasing demand for personalized content, the ability to incorporate real-time data will only enhance the relevance and impact of digital signage in various environments.
Best Practices for Performance and Optimization in Digital Signage
When it comes to optimizing digital signage for performance and ensuring a seamless user experience, several best practices need to be adhered to. The primary goal is to maintain a fluid and responsive interface while delivering rich content. This involves understanding how JavaScript, as well as the overall architecture of the digital signage setup, can be effectively utilized to achieve these objectives.
One of the first steps in optimizing performance is to minimize the amount of JavaScript that needs to be executed on the client side. This can be done by using techniques such as code splitting and lazy loading. Code splitting allows you to break down your JavaScript files into smaller chunks, which can be loaded on demand. This ensures that users only download the essential code required for the initial rendering of the display. Here’s an example of how you might implement lazy loading for a module that handles heavy computations:
document.getElementById('load-heavy-module').addEventListener('click', async () => { const module = await import('./heavyModule.js'); module.initialize(); });
In this example, the heavy module is only loaded when the user clicks a button, reducing the initial load time of the application. This technique not only decreases loading times but also minimizes memory usage for devices that may not have the capability to handle large JavaScript files.
Another crucial aspect of optimization is efficient DOM manipulation. The DOM is notoriously slow to update, so minimizing direct interactions with it can lead to significant performance gains. Rather than updating the DOM in response to every single change, you can batch updates and manipulate the DOM once with all necessary changes. This can be illustrated with the following example, which demonstrates how to batch multiple updates together:
function updateDisplay(data) { const displayArea = document.getElementById('display-area'); const fragment = document.createDocumentFragment(); // Create a document fragment data.forEach(item => { const div = document.createElement('div'); div.textContent = item.text; fragment.appendChild(div); // Append to fragment instead of directly to the DOM }); displayArea.appendChild(fragment); // Append the entire fragment simultaneously }
Using a document fragment allows you to reduce reflows and repaints by performing a bulk update, significantly enhancing the rendering speed of the digital signage content.
Additionally, caching strategies can play a pivotal role in optimization. By caching static assets such as images, scripts, and stylesheets, you can reduce the amount of data that needs to be fetched from the server. Implementing service workers is an excellent way to achieve this, as they enable background caching and can serve cached content while offline. Here’s a simple example of a service worker that caches assets:
self.addEventListener('install', event => { event.waitUntil( caches.open('v1').then(cache => { return cache.addAll([ '/index.html', '/styles.css', '/script.js', '/image.jpg' ]); }) ); });
This service worker intercepts network requests after installation, allowing the application to serve cached responses and improving load times significantly, especially in environments with unstable internet connections.
It’s also essential to perform regular audits of your JavaScript code to identify bottlenecks or areas that can be optimized further. Tools such as Google Lighthouse can help assess the performance of your digital signage applications, providing insights and recommendations for enhancements.
Finally, when dealing with real-time data updates, it’s crucial to implement throttling or debouncing techniques to manage how often updates occur. This can prevent overwhelming the display with rapid changes that could degrade performance. For example, when fetching data from an API, you might want to throttle the calls to only occur once every few seconds:
let lastFetchTime = 0; function fetchData() { const now = Date.now(); if (now - lastFetchTime > 5000) { // Only fetch every 5 seconds fetch('https://api.example.com/data') .then(response => response.json()) .then(data => updateDisplay(data)); lastFetchTime = now; } } // Fetch data every second setInterval(fetchData, 1000);
This simple check ensures that the fetch operation is not overused, thereby conserving resources and keeping the display responsive.
By adhering to these best practices in performance optimization, developers can significantly enhance the efficiency and user experience of digital signage applications. The use of JavaScript in conjunction with thoughtful architectural decisions will ensure that digital displays not only convey information effectively but do so in a manner that captivates and holds the audience’s attention.