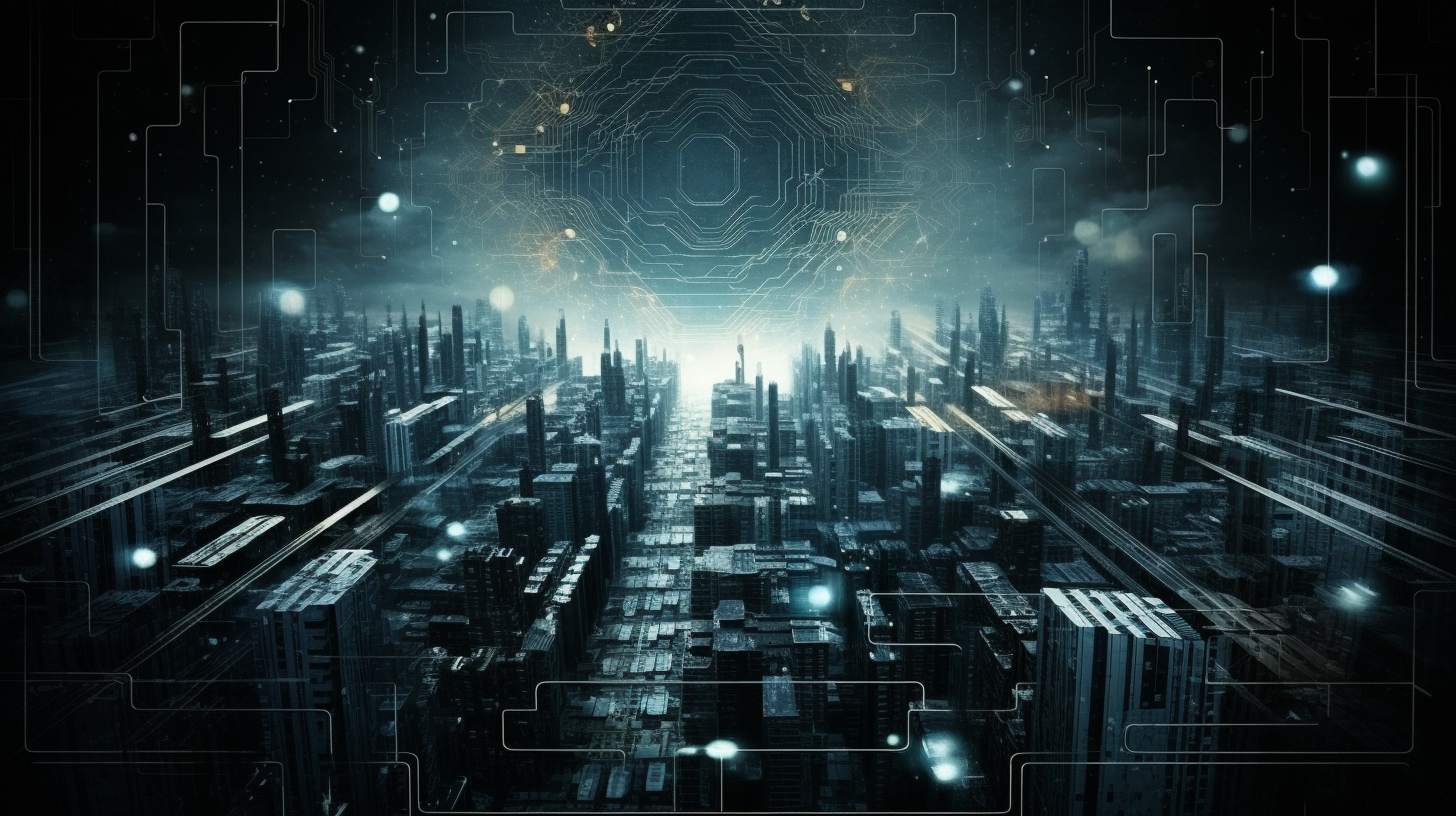
JavaScript Form Validation Techniques
Form validation is a critical aspect of web development that ensures the integrity and accuracy of the data submitted by users. It acts as the first line of defense against erroneous, incomplete, or malicious data entries. Without form validation, applications are vulnerable to a myriad of issues, ranging from minor inconveniences to significant security vulnerabilities.
At its core, form validation helps maintain data quality. By verifying input before it reaches the server, developers can significantly reduce the chances of database corruption or the introduction of harmful scripts. This not only safeguards the application but also enhances the user experience by providing immediate feedback, thus preventing frustration.
Moreover, form validation is essential for maintaining the integrity of business logic. For instance, when a user fills out a registration form, ensuring that fields like email addresses follow the correct format protects the application’s workflow. A well-validated form can help in preventing further errors down the line, such as failed logins or erroneous data processing.
It is not just about preventing errors, though. Form validation also plays a pivotal role in enhancing security. Applications that fail to validate user input can be susceptible to attacks like SQL injection or cross-site scripting (XSS). By implementing stringent validation rules, developers can create a barrier that helps mitigate these risks and preserves the safety of user data.
As we delve deeper into the various validation approaches, remember that effective form validation strikes a balance between being thorough and maintaining a smooth user experience. Too many restrictions may deter users from completing their tasks. Therefore, striking the right chord in your validation logic is paramount.
Think the following simple example of a basic form validation function in JavaScript:
function validateForm() { var email = document.getElementById("email").value; var password = document.getElementById("password").value; if (!email.includes("@") || email.length < 5) { alert("Please enter a valid email address."); return false; } if (password.length < 6) { alert("Password must be at least 6 characters long."); return false; } return true; }
This snippet demonstrates how a simpler validation function can provide immediate feedback to users, ensuring that the data submitted adheres to the expected formats and lengths. Thus, understanding and implementing form validation is vital for any web application aiming for reliability and security.
Client-Side vs. Server-Side Validation
When considering form validation, it is essential to differentiate between client-side and server-side validation, as both play crucial roles in ensuring data integrity and application security. Client-side validation occurs in the user’s browser, while server-side validation takes place on the server after the data has been submitted. Each approach has its advantages and limitations, which developers must understand to implement an effective validation strategy.
Client-side validation is primarily about providing instant feedback to users. By using JavaScript, developers can check user input in real time, preventing erroneous submissions before they ever reach the server. This not only enhances user experience but also reduces unnecessary server load. For example, a simple JavaScript function can check that all required fields are filled out and that the input formats are correct. Here’s an example:
function validateEmail(email) { const regex = /^[w-]+(.[w-]+)*@([w-]+.)+[a-zA-Z]{2,7}$/; return regex.test(email); } function validateForm() { const email = document.getElementById("email").value; if (!validateEmail(email)) { alert("Please enter a valid email address."); return false; } return true; }
This method improves user engagement by providing immediate prompts for correction, thus fostering a more interactive and responsive experience. However, while client-side validation is beneficial, it should never be the sole line of defense. Since users can manipulate client-side code, relying exclusively on it can lead to security vulnerabilities.
On the other hand, server-side validation is important for safeguarding data integrity. It ensures that all incoming data adheres to the specified rules, regardless of the source. Since server-side validation occurs after the data has been submitted, it acts as a safety net against malicious inputs or errors that may bypass client-side checks. This involves revalidating data on the server and applying business logic checks, such as ensuring that usernames are unique or that passwords meet strength criteria. Here’s a simplified example:
app.post("/submit-form", (req, res) => { const { email, password } = req.body; if (!validateEmail(email)) { return res.status(400).send("Invalid email format."); } if (password.length < 6) { return res.status(400).send("Password must be at least 6 characters long."); } // Proceed to handle valid data });
In this example, even if the client-side validation is bypassed, the server will still enforce the necessary checks, ensuring that only valid data is processed. This redundancy is critical in protecting the application from various attacks, including XSS and SQL injection.
Both client-side and server-side validations are necessary components of a robust form validation framework. They serve different purposes but are complementary in ensuring that the data entering an application is both valid and secure. Understanding and implementing both types of validation allow developers to create a seamless user experience while protecting their applications from potential threats.
Using HTML5 Built-in Validation Features
HTML5 has introduced several built-in validation features that greatly simplify the process of ensuring user input meets specific criteria. These features leverage the HTML5 specification, allowing developers to create forms that automatically validate user input without requiring extensive JavaScript code. By using these attributes, developers can minimize the amount of custom validation code they need while still providing a robust user experience.
One of the most significant advantages of HTML5 form validation is that it integrates seamlessly with standard form controls. For instance, the required attribute ensures that a user cannot submit a form without filling out specific fields. This is an effective way to enforce mandatory inputs without additional scripting:
In the above form, users will receive a browser-generated message if they attempt to submit the form without completing the username or email fields. This built-in validation behavior enhances usability, allowing users to quickly correct their input without waiting for a server response.
Another powerful feature is the pattern attribute, which allows developers to specify a regular expression that input must match for the form to be submitted. For example, if you want to ensure that a user enters a specific format for a phone number, you could implement it as follows:
In this snippet, the pattern attribute enforces a phone number format of three digits, followed by a hyphen, three more digits, another hyphen, and finally four digits. If the format doesn’t match, the browser will prompt the user to correct it before submission.
The type attribute enhances validation by which will allow you to specify the expected data type. Using types like email, url, and number can provide intrinsic validation checks based on the input type. For instance:
Here, the input type url ensures that users can only submit valid URLs. If a user attempts to submit an invalid URL format, the browser will display an error message and prevent submission, which can save developers time and effort in handling incorrect inputs.
HTML5 validation also provides visual feedback through pseudo-classes such as :valid and :invalid. This allows developers to style form elements based on their validation state. For example, you could highlight valid fields in green and invalid fields in red:
input:valid { border-color: green; } input:invalid { border-color: red; }
This approach enriches user experience by visually guiding users towards valid input, thereby reducing frustration and enhancing form usability.
Using HTML5’s built-in validation features not only simplifies the development process but also enhances user experience by providing immediate, client-side feedback. This integration of validation directly within the markup reduces the need for extensive JavaScript, making forms cleaner and easier to maintain. As web developers, embracing these features is essential for building efficient, uncomplicated to manage applications that adhere to the latest web standards.
Regular Expressions for Advanced Validation
Regular expressions (regex) are an essential tool in the arsenal of a JavaScript developer, particularly when it comes to advanced form validation. They provide a powerful way to define complex patterns for input validation, allowing developers to ensure that user inputs conform to expected formats. Using regex can help catch a wide range of errors that basic string checks might miss, enhancing both the robustness and security of your applications.
When working with forms, regex can validate inputs like email addresses, phone numbers, passwords, and even custom formats. The key advantage of using regular expressions is their flexibility; a single regex pattern can replace multiple lines of validation logic. This conciseness helps keep your code clean and maintainable.
Let’s look at an example of how to use regex for validating email addresses. The following pattern checks if an email follows standard conventions, accounting for the presence of an ‘@’ symbol and a valid domain:
function validateEmail(email) { const regex = /^[w-]+(.[w-]+)*@([w-]+.)+[a-zA-Z]{2,7}$/; return regex.test(email); }
The regex used here consists of several components:
- ^ asserts the start of a line.
- [w-]+ matches one or more word characters (letters, digits, or underscores) and hyphens.
- (.[w-]+)* permits zero or more occurrences of a period followed by word characters or hyphens, allowing for email addresses with subdomains.
- @ is a literal character that separates the local part from the domain.
- [w-]+ matches the domain name, which can contain word characters and hyphens.
- (.)+[a-zA-Z]{2,7}$ ensures that the domain ends with a dot followed by a valid top-level domain (TLD) consisting of 2 to 7 letters.
Next, let’s implement a regex pattern for validating phone numbers. Assuming we want to validate a North American phone number format, we can use the following regex:
function validatePhoneNumber(phone) { const regex = /^(d{3}) d{3}-d{4}$/; return regex.test(phone); }
Here’s how this regex breaks down:
- ^ asserts the start of the string.
- (d{3}) matches an area code enclosed in parentheses, which consists of exactly three digits.
- s matches a whitespace character to account for the space after the area code.
- d{3} matches the next three digits of the phone number.
- – is a literal hyphen separating the next set of digits.
- d{4}$ matches the final four digits of the phone number, asserting the end of the string.
In many cases, you will also want to provide user feedback on what constitutes valid input. This can be done by integrating validation logic directly into your form handling functions. Here’s how you might implement email and phone validation in a single form submission handler:
function validateForm() { const email = document.getElementById("email").value; const phone = document.getElementById("phone").value; if (!validateEmail(email)) { alert("Please enter a valid email address."); return false; } if (!validatePhoneNumber(phone)) { alert("Please enter a valid phone number (e.g., (123) 456-7890)."); return false; } return true; }
By using regex in this manner, developers can create more sophisticated and simple to operate validation processes. However, it’s crucial to remember that while regex is an incredibly powerful tool, it’s also complex and prone to error if not used correctly. Testing your regex patterns thoroughly is essential to ensure they behave as expected across various input scenarios.
The application of regular expressions can greatly enhance the depth and reliability of form validations in JavaScript. They allow developers to enforce precise input formats, which can significantly reduce the likelihood of erroneous or malicious data submissions. As you continue to explore form validation techniques, mastering regex will undoubtedly expand your capabilities in crafting secure and robust web applications.
Implementing Custom Validation Functions
Custom validation functions provide developers with the flexibility to enforce specific rules and logic tailored to their application’s requirements. While HTML5 built-in features and regex offer robust options for general input validation, there are scenarios where bespoke validation logic becomes necessary. This can include complex business rules, conditional validation based on other input fields, or unique user requirements that cannot be addressed through standard methods.
When implementing custom validation functions, it’s essential to maintain clean, readable code while ensuring that your validation logic is robust and efficient. A good practice is to create modular functions that can be reused across different forms or input fields. This not only streamlines your validation process but also enhances maintainability.
Here’s a basic example of a custom validation function that checks a user’s age based on their date of birth input. This function ensures that the user is at least 18 years old:
function validateAge(dob) { const today = new Date(); const birthDate = new Date(dob); const age = today.getFullYear() - birthDate.getFullYear(); const monthDifference = today.getMonth() - birthDate.getMonth(); // Check if the user is 18 years or older if (age > 18 || (age === 18 && monthDifference > 0) || (age === 18 && monthDifference === 0 && today.getDate() >= birthDate.getDate())) { return true; } else { alert("You must be at least 18 years old."); return false; } }
In this function, we calculate the user’s age by comparing the current date with their date of birth. The function checks if the age is 18 or older, providing immediate feedback if the user does not meet the requirement. Note that the alert message can be replaced with more effortless to handle feedback mechanisms, such as displaying validation messages next to the input field.
Another instance where custom validation functions shine is in ensuring that passwords meet specific criteria. For example, a function could enforce that passwords contain a mix of uppercase letters, lowercase letters, numbers, and special characters. Here’s an example:
function validatePassword(password) { const regex = /^(?=.*[a-z])(?=.*[A-Z])(?=.*d)(?=.*[@$!%*?&])[A-Za-zd@$!%*?&]{8,}$/; if (!regex.test(password)) { alert("Password must be at least 8 characters long and include an uppercase letter, a lowercase letter, a number, and a special character."); return false; } return true; }
This function uses a regex pattern that requires a combination of character types, thereby enforcing a strong password policy. If the provided password fails to match the pattern, it alerts the user with detailed information on the requirements.
It is also vital to handle validation in a way that enhances the user experience. Instead of relying solely on alert boxes, ponder using inline error messages or highlighting invalid fields. This not only improves usability but also reduces user frustration. Here’s how you might integrate inline error handling:
function validateForm() { const dob = document.getElementById("dob").value; const password = document.getElementById("password").value; let isValid = true; // Clear previous error messages document.getElementById("dobError").textContent = ""; document.getElementById("passwordError").textContent = ""; if (!validateAge(dob)) { document.getElementById("dobError").textContent = "Invalid age. You must be at least 18 years old."; isValid = false; } if (!validatePassword(password)) { document.getElementById("passwordError").textContent = "Password is not strong enough."; isValid = false; } return isValid; }
In this implementation, we clear any previous error messages before performing the validations. If a validation fails, we display a corresponding message next to the relevant input field. This approach keeps the user informed and engaged, making the form-filling process smoother and more intuitive.
Ultimately, custom validation functions empower developers to create tailored validation rules that enhance the overall robustness of web applications. By using JavaScript’s capabilities and focusing on user experience, you can ensure that your forms not only safeguard against erroneous submissions but also provide users with the guidance they need to complete their tasks successfully.
Best Practices for Easy to use Validation Messages
When it comes to form validation, how you communicate errors to users can significantly impact their experience. Well-crafted, easy to use validation messages can guide users seamlessly through the form-filling process, reducing frustration and enhancing usability. To achieve this, developers should adhere to several best practices when designing validation feedback.
Be Clear and Concise: The primary goal of validation messages is to inform users about what went wrong. Messages should be simpler and easy to understand. Avoid jargon or overly technical language. Instead, use simple phrases that clearly explain the issue. For instance, instead of saying “Input format is invalid,” you might say, “Please enter a valid email address.” This clarity helps users quickly grasp the necessary corrections.
Provide Contextual Feedback: Feedback should be relevant to the specific input field causing the issue. Position error messages close to the related input field to provide immediate context. This visual proximity allows users to easily identify what they need to change. For example:
function validateEmail(email) { const regex = /^[w-]+(.[w-]+)*@([w-]+.)+[a-zA-Z]{2,7}$/; if (!regex.test(email)) { document.getElementById("emailError").textContent = "Please enter a valid email address."; return false; } document.getElementById("emailError").textContent = ""; // Clear previous error return true; }
Use Positive Language: Frame messages positively whenever possible. Rather than saying “This field cannot be empty,” you could say “Please fill out this field.” Positive language can make the interaction feel less punitive and more supportive, encouraging users to correct their errors.
Use Visual Cues: In addition to textual feedback, consider using visual indicators to highlight errors. Changing the border color of an input field to red or adding an icon can visually communicate that something is wrong. This dual approach of visual and textual feedback reinforces the message and helps grab the user’s attention effectively.
input:invalid { border-color: red; } input:valid { border-color: green; }
Limit Error Messages: While it’s important to provide feedback, bombarding users with too many error messages at once can be overwhelming. Focus on the most critical errors first, allowing users to correct one issue at a time. Once they address the highlighted error, you can validate the form again and present additional feedback as necessary.
Encourage Positive Actions: After resolving an error, guide users on what to do next. For instance, after correcting an invalid email address, you might prompt them to “Continue to the next step.” This helps maintain momentum and keeps users engaged in the process.
Test with Real Users: Finally, it’s essential to test your validation messages with real users. Gathering feedback on clarity and usefulness can help identify areas for improvement. Observing how users interact with your forms can provide valuable insights into how they interpret validation messages and what adjustments might enhance their experience.
Incorporating these best practices into your form validation strategy not only improves usability but also fosters a more positive user experience. By prioritizing clear, supportive, and contextual feedback, developers can guide users through form completion with confidence, leading to higher satisfaction and successful submissions.