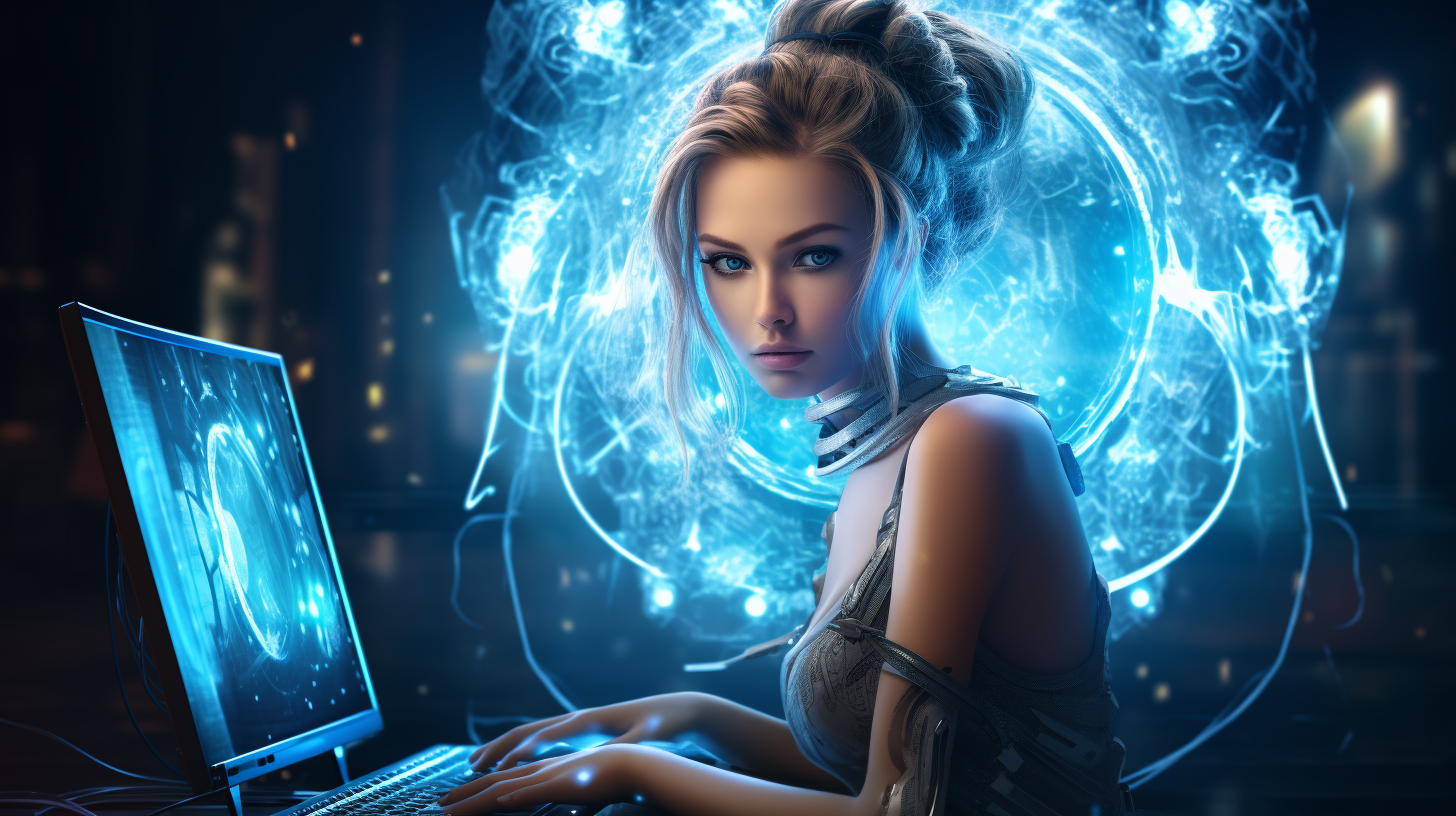
Java Access Modifiers Explained
In Java, access modifiers play an important role in encapsulation, an essential aspect of object-oriented programming. They control the visibility and accessibility of classes, methods, and variables within your code. By effectively using access modifiers, you can protect your data from unintended interference and create a clear boundary around your components, allowing for better maintainability and security.
Java provides four primary access modifiers: public, private, protected, and the default (package-private). Each modifier serves a unique purpose and offers varying levels of access control. Understanding how these modifiers work is key to designing robust and secure Java applications.
The access modifiers can be summarized as follows:
- Members declared as public are accessible from any other class in the same package or any other package.
- Members declared as private can only be accessed within the class they’re declared in.
- Members declared as protected can be accessed within the same package or subclasses in different packages.
- Members with no access modifier (default) can only be accessed within classes in the same package.
To illustrate these concepts, ponder the following Java code examples:
public class AccessModifierExample { // Public variable public int publicVar = 1; // Private variable private int privateVar = 2; // Protected variable protected int protectedVar = 3; // Default variable int defaultVar = 4; public void display() { System.out.println("Public Variable: " + publicVar); System.out.println("Private Variable: " + privateVar); System.out.println("Protected Variable: " + protectedVar); System.out.println("Default Variable: " + defaultVar); } }
In this example, we define a class AccessModifierExample
with four different types of variables. The display
method illustrates that all variables can be accessed within the same class. However, their accessibility from other classes will depend on the access modifiers applied to them.
Types of Access Modifiers in Java
To delve deeper into the implications of these access modifiers, let’s examine the specific characteristics and use cases for each type. The distinction between them is not merely academic; it has real-world consequences on how you design your systems and manage dependencies.
Public Access Modifier: When you declare a class member as public, you’re signaling that it is part of the API for that class. It’s open for access from anywhere in the application, which can be useful for methods or variables that you want to expose universally. However, this also means that they’re vulnerable to unintended manipulation or misuse, so careful consideration is required when determining what should be made public.
public class PublicExample { public void publicMethod() { System.out.println("This is a public method."); } }
In the above example, the publicMethod
can be called from any other class:
public class TestPublic { public static void main(String[] args) { PublicExample example = new PublicExample(); example.publicMethod(); // Accessible from any class } }
Private Access Modifier: This modifier is all about encapsulation. A private member can only be accessed within the class it belongs to. That is particularly useful for managing internal state or helper methods that should not be exposed outside of the class. It ensures that the class’s internal implementation can change without affecting other classes.
public class PrivateExample { private int secretValue = 42; private void printSecret() { System.out.println("Secret Value: " + secretValue); } public void revealSecret() { printSecret(); // Can call the private method from within the same class } }
In this case, you can only access secretValue
and printSecret
from within PrivateExample
. Attempting to access these from outside the class will result in a compile-time error.
public class TestPrivate { public static void main(String[] args) { PrivateExample example = new PrivateExample(); // example.secretValue; // Error: secretValue has private access in PrivateExample example.revealSecret(); // Works, as it is a public method } }
Protected Access Modifier: The protected modifier allows access within the same package and from subclasses, even if they are in different packages. This strikes a balance between public and private access. It is commonly used in inheritance scenarios where you want to allow subclasses to utilize certain features while keeping them hidden from the outside world.
public class ProtectedExample { protected void protectedMethod() { System.out.println("This is a protected method."); } } public class SubclassExample extends ProtectedExample { public void callProtectedMethod() { protectedMethod(); // Accessible in a subclass } }
Here, the protectedMethod
can be accessed in SubclassExample
, illustrating how inheritance can leverage protected access.
public class TestProtected { public static void main(String[] args) { SubclassExample subclass = new SubclassExample(); subclass.callProtectedMethod(); // Works } }
Default Access Modifier (Package-Private): If you do not specify any access modifier, Java applies the default access level, which means the member is accessible only within classes in the same package. This is useful for classes that are closely related and need to interact without exposing their members to the wider application. It encourages package-level encapsulation.
class DefaultExample { void defaultMethod() { System.out.println("This is a default method."); } }
As such, you might have another class in the same package that can access defaultMethod
:
class TestDefault { public static void main(String[] args) { DefaultExample example = new DefaultExample(); example.defaultMethod(); // Accessible because it's in the same package } }
However, trying to access defaultMethod
from a class in a different package will lead to a compile-time error. This characteristic can help in grouping related classes and methods while providing a layer of protection from the outside.
Default Access Modifier
The default access modifier, often referred to as “package-private,” is the most permissive access level in terms of allowing other classes within the same package to interact with a class’s members. When you omit an access modifier, Java automatically treats that class or member as package-private. This means that it can be accessed by any other class in the same package, but not from classes outside of that package.
This level of access is particularly useful when you have a set of classes that are closely related and need to collaborate without exposing their internals to the world outside their package. It promotes encapsulation at the package level while still allowing for a broader scope of interaction than private access would allow.
To illustrate this, think the following example:
class DefaultAccessExample { void display() { System.out.println("This is a default access method."); } }
Here, we define a class DefaultAccessExample
with a method display
. Since we haven’t specified any access modifier, display
has default access.
Next, we can create another class within the same package to test this:
class TestDefaultAccess { public static void main(String[] args) { DefaultAccessExample example = new DefaultAccessExample(); example.display(); // Accessible because TestDefaultAccess is in the same package } }
In this example, TestDefaultAccess
can successfully create an instance of DefaultAccessExample
and invoke the display
method because both classes reside in the same package. If we were to attempt this from a class in a different package, the compiler would throw an error, indicating that display
has default access and cannot be accessed.
package differentPackage; import somePackage.DefaultAccessExample; // Assume this is the package of DefaultAccessExample class TestDifferentPackage { public static void main(String[] args) { DefaultAccessExample example = new DefaultAccessExample(); // example.display(); // This would cause a compile-time error } }
Protected Access Modifier
The protected access modifier in Java offers a unique blend of visibility that caters specifically to the needs of inheritance. Members declared as protected can be accessed not only within the same package but also by subclasses residing in different packages. This characteristic makes the protected modifier particularly useful for building class hierarchies where you want to expose certain functionality to subclasses while still encapsulating those details from the rest of the application.
When designing a class hierarchy, using protected access allows subclasses to inherit and utilize methods or variables from their parent classes, promoting code reuse and flexibility. However, it’s vital to remember that while you achieve greater accessibility for subclasses, you must also be cautious to prevent unintended exposure of sensitive implementation details to the outside world.
Consider the following example that illustrates how the protected modifier operates:
public class Animal { protected void makeSound() { System.out.println("Animal sound"); } } public class Dog extends Animal { public void bark() { makeSound(); // Accessible due to protected access System.out.println("Bark!"); } } public class TestProtectedAccess { public static void main(String[] args) { Dog dog = new Dog(); dog.bark(); // Works, calling the protected method through a subclass } }
In this example, the Animal
class has a method makeSound
that is marked as protected. The Dog
class, which extends Animal
, can access makeSound
directly within its own method bark
. When you run the TestProtectedAccess
class, it creates an instance of Dog
and calls bark
, which in turn calls the protected makeSound
method. This demonstrates the effective use of protected access to share functionality between a parent class and its subclass.
However, attempting to access a protected member from a non-subclass in a different package would result in a compile-time error. This encapsulation strategy allows you to maintain a structured approach to how classes interact, emphasizing the importance of visibility and accessibility in your code architecture.
Public and Private Access Modifiers
The public and private access modifiers serve as the cornerstones of visibility control in Java, each catering to distinct needs within the language’s design paradigm. Understanding the nuances between these two modifiers is essential for crafting secure and maintainable applications.
Public Access Modifier: Declaring a member as public is akin to throwing open the doors of your class, allowing any other part of your application to interact with it freely. This unrestricted access is beneficial when you want to expose certain functionalities or data to the entire world. For instance, ponder a utility class that provides widely used helper methods.
public class Utility { public static void printHello() { System.out.println("Hello, World!"); } } public class TestUtility { public static void main(String[] args) { Utility.printHello(); // Accessible from anywhere } }
In this example, the printHello method is public, meaning it can be called from any class in any package. However, while public members can facilitate easy interaction, they also open up the potential for misuse. A poorly designed public API can lead to unexpected behaviors if users are allowed to manipulate internal states indiscriminately.
Private Access Modifier: In stark contrast, the private modifier effectively locks away a member, restricting access solely to the class in which it resides. This encapsulation is vital for safeguarding the integrity of your class’s internal state. By using private members, you can ensure that only the class itself can modify or interact with these members directly.
public class SecretKeeper { private String secret = "Top Secret"; private void revealSecret() { System.out.println("Secret: " + secret); } public void showSecret() { revealSecret(); // Accessing private method within the same class } } public class TestSecretKeeper { public static void main(String[] args) { SecretKeeper keeper = new SecretKeeper(); keeper.showSecret(); // Works fine // keeper.revealSecret(); // Error: revealSecret has private access } }
In this case, secret and revealSecret are private, preventing any external class from accessing them directly. Instead, the public showSecret method acts as a controlled access point, allowing external classes to retrieve the secret without exposing the underlying implementation. This encapsulation strategy is fundamental in maintaining clean interfaces and reducing dependencies within your codebase.