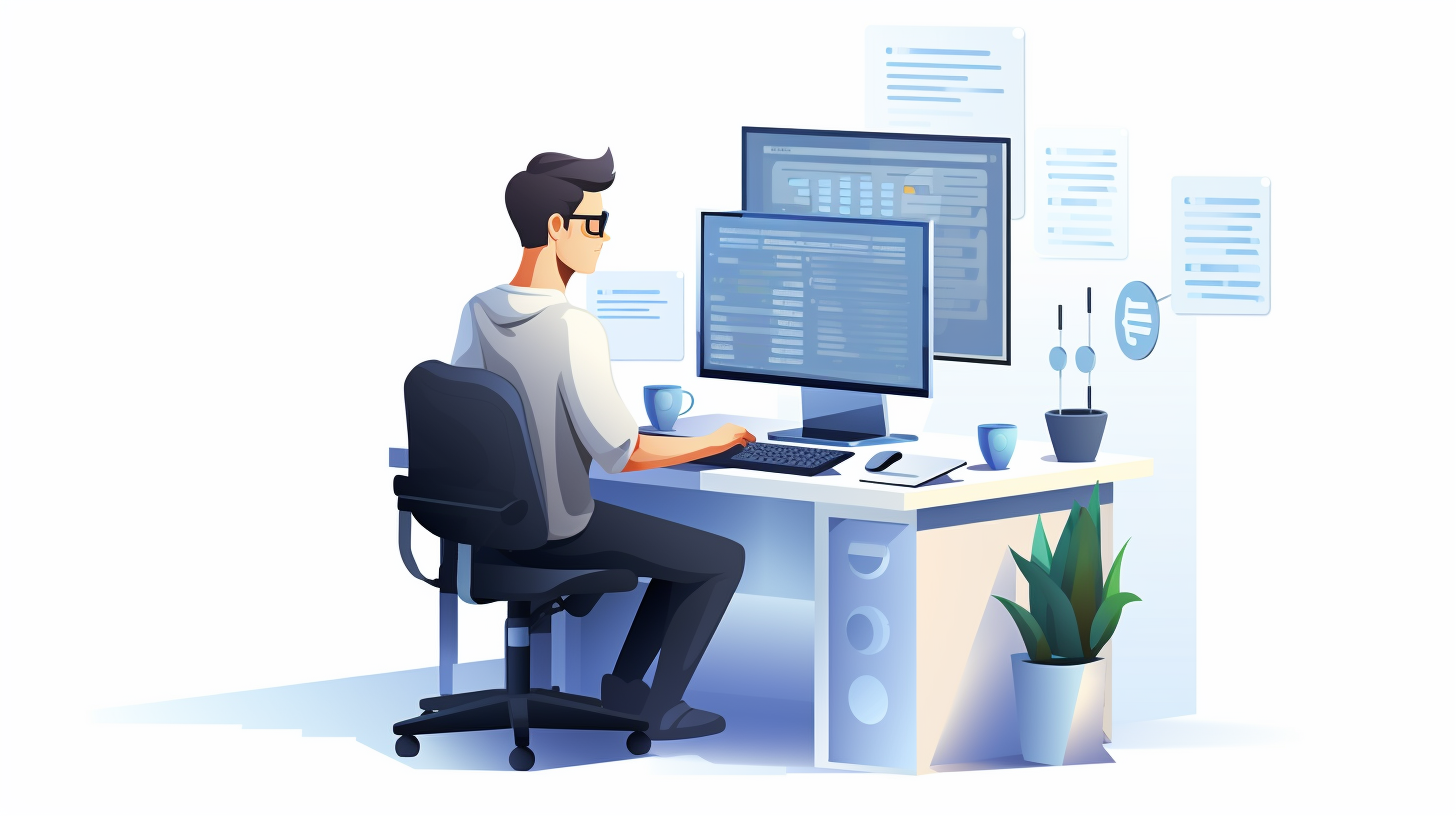
Advanced Swift: Generics
Generics in Swift provide a powerful way to write flexible and reusable code. By defining a function or type that works with any type, your code can adapt to a wide range of situations without sacrificing type safety. This is particularly useful in scenarios where you want to create data structures, algorithms, or functions that can operate on various data types without needing to duplicate code for each type.
At its core, generics allow you to define type parameters, which act as placeholders that can be replaced with actual types when the function or type is used. This means you can write code that is both generic and type-safe, ensuring that type errors are caught at compile-time rather than at run-time.
To illustrate the concept, think a simple example of a generic function that swaps two values:
func swap(_ a: inout T, _ b: inout T) { let temp = a a = b b = temp }
In this snippet, the function swap
takes two parameters of the same type T
, which is inferred when the function is called. This ensures that you can only swap values of the same type, maintaining type safety.
When you call this function, it could look like this:
var x = 10 var y = 20 swap(&x, &y) // After this call, x will be 20 and y will be 10
Moreover, generics do not only apply to functions; they also extend to types. You can define a generic struct or class. For instance, a simple stack implementation can be created as follows:
struct Stack<Element> { private var items: [Element] = [] mutating func push(_ item: Element) { items.append(item) } mutating func pop() -> Element? { return items.isEmpty ? nil : items.removeLast() } }
This Stack
struct is now type-agnostic; it can work with any type, be it Int
, String
, or any custom type. You simply specify the type when you create an instance of the stack:
var intStack = Stack<Int>() intStack.push(1) intStack.push(2) print(intStack.pop()) // Prints Optional(2) var stringStack = Stack<String>() stringStack.push("Hello") stringStack.push("World") print(stringStack.pop()) // Prints Optional("World")
As you can see, generics provide a means of developing code that’s both versatile and robust, using Swift’s strong type system to ensure that not only is your code reusable but also safe from type-related errors.
Benefits of Using Generics
One of the most compelling benefits of using generics in Swift is the ability to create code this is not only reusable but also highly efficient. By writing functions, methods, and types that can work with any type while maintaining type safety, you avoid code duplication and reduce the risk of errors. This leads to cleaner codebases that are easier to maintain and extend.
Generics also allow you to implement algorithms and data structures that are inherently type-agnostic. This means you can write a single implementation that works across multiple data types, as opposed to writing separate implementations for each specific type. This not only saves development time but also reduces the potential for bugs that can arise from having multiple similar implementations.
Furthermore, generics can improve performance. When you use specific types instead of generic placeholders, the Swift compiler can optimize code more effectively. The compiler generates specialized versions of your generic code for each type that is used, which can lead to performance gains compared to using protocols or any type erasure mechanism.
Ponder a generic sorting function, which can sort arrays of any type that conforms to the Comparable protocol:
func genericSort<T: Comparable>(_ array: [T]) -> [T] { return array.sorted() }
In this example, the genericSort function takes an array of any type T that conforms to the Comparable protocol. You can use this function with different types seamlessly:
let intArray = [3, 1, 2] let sortedInts = genericSort(intArray) // Returns [1, 2, 3] let stringArray = ["banana", "apple", "cherry"] let sortedStrings = genericSort(stringArray) // Returns ["apple", "banana", "cherry"]
This demonstrates not only the reusability of generics but also how they can be used to write more abstract and high-level code. The same function handles both integers and strings without any modifications, showcasing the flexibility that generics afford developers.
The benefits of generics in Swift are manifold. They allow for reusable and type-safe code, enhance performance through type specialization, and enable the creation of generic algorithms and data structures that can operate across various types. This makes generics an essential feature of the Swift programming language, significantly enriching the developer’s toolkit.
Generic Functions and Types
In Swift, generic functions and types empower developers to create components that are highly flexible and reusable while maintaining the integrity of type safety. The key to understanding how these generics work lies in their structure. A generic function can be thought of as a blueprint that can work with any data type by using type parameters.
Let’s delve deeper into the structure of generic functions. The declaration of a generic function begins with the use of angle brackets to define one or more type parameters. These parameters can then be used throughout the function as if they were concrete types. For example, a simple generic function to get the first element of an array can be defined as follows:
func firstElement<T>(_ array: [T]) -> T? { return array.isEmpty ? nil : array[0] }
This function, `firstElement`, accepts an array of any type `T` and returns the first element of that array. The use of a generic type parameter `T` allows this function to work seamlessly with arrays of any data type. Calling this function is equally straightforward:
let intArray = [1, 2, 3] let firstInt = firstElement(intArray) // Returns Optional(1) let stringArray = ["Hello", "World"] let firstString = firstElement(stringArray) // Returns Optional("Hello")
In both cases, the function adapts to the type of the array passed to it, demonstrating the utility of generics in eliminating redundancy while ensuring type safety.
Now, let’s look at generic types, which can be employed in a similar fashion. A generic class or struct allows you to define a data structure that can hold values of various types. Ponder a more complex example involving a generic container class:
class Container<Item> { private var items: [Item] = [] func add(_ item: Item) { items.append(item) } func retrieve(at index: Int) -> Item? { return index < items.count ? items[index] : nil } }
Here, `Container` is a class that can hold items of any type specified by the type parameter `Item`. This allows for great flexibility when using the `Container` class. You can instantiate it with different types as shown:
let intContainer = Container<Int>() intContainer.add(10) intContainer.add(20) print(intContainer.retrieve(at: 0)) // Prints Optional(10) let stringContainer = Container<String>() stringContainer.add("Swift") stringContainer.add("Generics") print(stringContainer.retrieve(at: 1)) // Prints Optional("Generics")
The `Container` class above demonstrates how generics allow the creation of a versatile data structure that can handle different types of items without sacrificing the type safety that Swift provides. Each instance of `Container` is tailored to hold a specific type, rendering it effortless to manage collections of various data types within the same context.
In addition to flexibility and type safety, using generics helps in writing cleaner and more maintainable code. By abstracting the type details, you can focus on the logic of your functions and data structures without cluttering your code with type-specific implementations.
Generics in Swift open up a world of possibilities, allowing developers to write functions and types that are not only efficient and reusable but also adaptable to a multitude of scenarios. As you continue to harness the power of generics, you’ll find your Swift code evolving into a more sophisticated and elegant form, capable of handling diverse requirements with ease.
Constraints on Generics
Constraints on generics in Swift allow you to impose certain restrictions on the types that can be used as arguments for your generic functions and types. That’s essential for ensuring that the generic code operates correctly and efficiently by limiting the acceptable types to those that meet specified criteria. By applying constraints, you can work with types that conform to certain protocols or inherit from specific classes, thus using the capabilities of those types within your generic implementations.
At its simplest, you can constrain a generic type parameter to conform to a protocol using a syntax that resembles this:
func performAction<T: SomeProtocol>(on item: T) { item.someMethod() // Methods of SomeProtocol can be called }
In this example, the type parameter T is constrained to types that conform to SomeProtocol. This means you can only pass instances of types that meet this protocol’s requirements to the `performAction` function. Here’s a practical illustration using the Comparable protocol, allowing us to implement a function that can compare two values:
func compare<T: Comparable>(a: T, b: T) -> Bool { return a < b }
With this `compare` function, we can compare any two values of a type that conforms to Comparable:
let result1 = compare(a: 5, b: 10) // true let result2 = compare(a: "apple", b: "banana") // false
Moreover, constraints can be combined. You can require that a type conforms to multiple protocols by separating them with commas. For instance, if you want to ensure that a type conforms to both Comparable and Equatable protocols, you would write:
func areEqual<T: Comparable & Equatable>(a: T, b: T) -> Bool { return a == b }
This allows you to use the `areEqual` function with types that are both comparable and equatable, providing greater flexibility and functional rigor.
Another vital aspect of constraints involves associated types within protocols. When a protocol has an associated type, you can specify that the generic type parameter must conform to that protocol, which allows you to work with associated types seamlessly. Ponder the following example with a custom protocol:
protocol Container { associatedtype Item func add(_ item: Item) func get(at index: Int) -> Item? } func processContainer<C: Container>(container: C) where C.Item == Int { container.add(10) let item = container.get(at: 0) print(item) // Prints Optional(10) }
In this `processContainer` function, C is constrained to be of a type that conforms to the `Container` protocol, and it is further specified that the associated type `Item` must be of type Int. This level of specificity ensures that the function operates safely with the expected types.
Constraints on generics enhance your code’s safety and clarity by ensuring that only the appropriate types can be used in specific contexts. They enable you to define precise requirements for type parameters, leading to more robust and maintainable code. As you become adept at employing constraints, your generics will not only be powerful but also precisely tailored to your program’s needs, providing the right balance between flexibility and safety.
Type Erasure and Any
Type erasure is a concept in Swift that allows you to hide the specific type of a value while still being able to interact with it in a type-safe manner. This can be particularly useful when you want to use generics in situations where the exact type is not known ahead of time or when you need to work with heterogeneous collections of types that share some common protocol but may not share a common superclass. The `Any` type serves as a fundamental mechanism in Swift that facilitates type erasure.
In Swift, the `Any` type can represent an instance of any type at all. Thus, when you want to create a collection or a data structure that can hold different types that conform to a protocol, you often need to use `Any`. However, using `Any` comes with trade-offs, particularly related to type safety and the inability to directly call methods of the underlying types without casting.
Think a simple example where we define a protocol and then create a type-erased wrapper around it:
protocol Drawable { func draw() } struct AnyDrawable { private let _draw: () -> Void init(_ drawable: T) { _draw = drawable.draw } func draw() { _draw() } }
In this example, `Drawable` is a protocol that requires a `draw` method. The `AnyDrawable` struct acts as a type-erased wrapper for any type conforming to `Drawable`. The initializer of `AnyDrawable` accepts any `Drawable` type and stores a closure that calls the `draw` method. When you invoke the `draw` method on an instance of `AnyDrawable`, it calls the original `draw` method of the underlying type without needing to know what that type is.
Here’s how you can use `AnyDrawable` to store different types that conform to `Drawable`:
struct Circle: Drawable { func draw() { print("Drawing a circle") } } struct Square: Drawable { func draw() { print("Drawing a square") } } let shapes: [AnyDrawable] = [AnyDrawable(Circle()), AnyDrawable(Square())] for shape in shapes { shape.draw() }
In this code, we create two structs, `Circle` and `Square`, both of which conform to `Drawable`. We then create an array of `AnyDrawable` that holds instances of both shapes. When we iterate over the array and call `draw`, each shape’s respective `draw` method executes, demonstrating the power of type erasure to handle diverse types uniformly.
Using `Any` and type erasure comes with its implications. One significant downside is that you lose compile-time type checking for the specific types contained within `Any`. This means you must be cautious when performing operations that depend on the specific type, as you may encounter runtime errors if you attempt to cast to an incorrect type. Therefore, it’s essential to provide a thoughtful design around where and how you use type erasure to ensure safety and clarity in your code.
Type erasure and `Any` are potent tools in the Swift programmer’s arsenal, enabling flexible designs that can accommodate various concrete types while maintaining type safety and encapsulation. By understanding and applying these concepts judiciously, you can leverage the full strength of Swift’s type system, unlocking new possibilities for code organization and reuse.
Advanced Patterns with Generics
Advanced patterns with generics in Swift reveal the depth and flexibility that generics provide beyond basic use cases. By using advanced patterns, developers can implement sophisticated APIs and data structures that promote code reuse and maintainability while embracing the power of static typing.
One of the most intriguing patterns is the use of generics in combination with associated types within protocols. This allows you to design protocols that can operate over generic types, enhancing the extensibility of your codebase. For instance, think a protocol representing a data source that can provide elements of a specific type:
protocol DataSource { associatedtype Element func fetch() -> Element }
The `DataSource` protocol defines an associated type `Element`, which allows conforming types to specify what type of elements they provide. That’s particularly powerful for building abstractions over various data storage systems, such as network services or databases, without tying your code to specific implementations.
To see this in action, let’s implement a concrete type conforming to the `DataSource` protocol for an array:
struct ArrayDataSource<T>: DataSource { private var items: [T] init(items: [T]) { self.items = items } func fetch() -> T { return items.removeFirst() // Just an example; would need to handle empty array } }
In this case, `ArrayDataSource` can operate on any type specified during its initialization, using the associated type `Element`. This design pattern not only accommodates different data sources but also emphasizes the reusable nature of your code.
Another advanced pattern involves combining generics with the builder pattern. This pattern is particularly useful when you need to create complex objects step-by-step while ensuring that the final object is constructed correctly. By using generics, you can enforce type constraints at compile time. Here’s a simple example:
class Builder<T> { private var element: T? func setElement(_ element: T) -> Self { self.element = element return self } func build() -> T { guard let result = element else { fatalError("Element must be set before building.") } return result } }
This `Builder` class allows you to set an element of type `T` and ensures that once you call `build`, the element has been provided. This guarantees that the client code is aware of the need to set the value, leading to fewer runtime errors and promoting a clear API.
Using the `Builder` pattern, you can create instances of different types easily:
let intBuilder = Builder<Int>() let builtInt = intBuilder.setElement(42).build() // Outputs 42 let stringBuilder = Builder<String>() let builtString = stringBuilder.setElement("Hello").build() // Outputs "Hello"
Generics can also enhance the use of functional programming paradigms in Swift. For example, ponder creating generic higher-order functions that can accept other functions as parameters. That is immensely powerful when dealing with collections. A common use case is to implement a `map` function for any collection type:
func map<T, U>(_ array: [T], transform: (T) -> U) -> [U] { var result: [U] = [] for item in array { result.append(transform(item)) } return result }
The `map` function above takes an array of type `T` and a transformation function that converts `T` into `U`. This pattern allows you to work with collections in a type-safe manner while applying transformations flexibly:
let numbers = [1, 2, 3] let strings = map(numbers) { "($0)" } // ["1", "2", "3"]
Advanced patterns with generics are not just about writing flexible and reusable code; they also revolve around creating APIs and structures that are clean and semantically clear. Using generics effectively can lead to APIs that not only serve a wide range of use cases but also enforce proper usage patterns at compile time.
Moreover, generics enhance code readability and intent. When used judiciously, they can help signal the programmer’s intent more clearly than if using concrete types. This adds to the maintainability of the code, as future developers can quickly grasp the functionality without excessive documentation.
These advanced patterns can significantly improve your Swift programming experience, allowing you to build robust, type-safe, and reusable components that are tailored to meet complex requirements. As you experiment with generics and integrate them into your code, you will uncover new opportunities for elegant solutions and efficient designs.