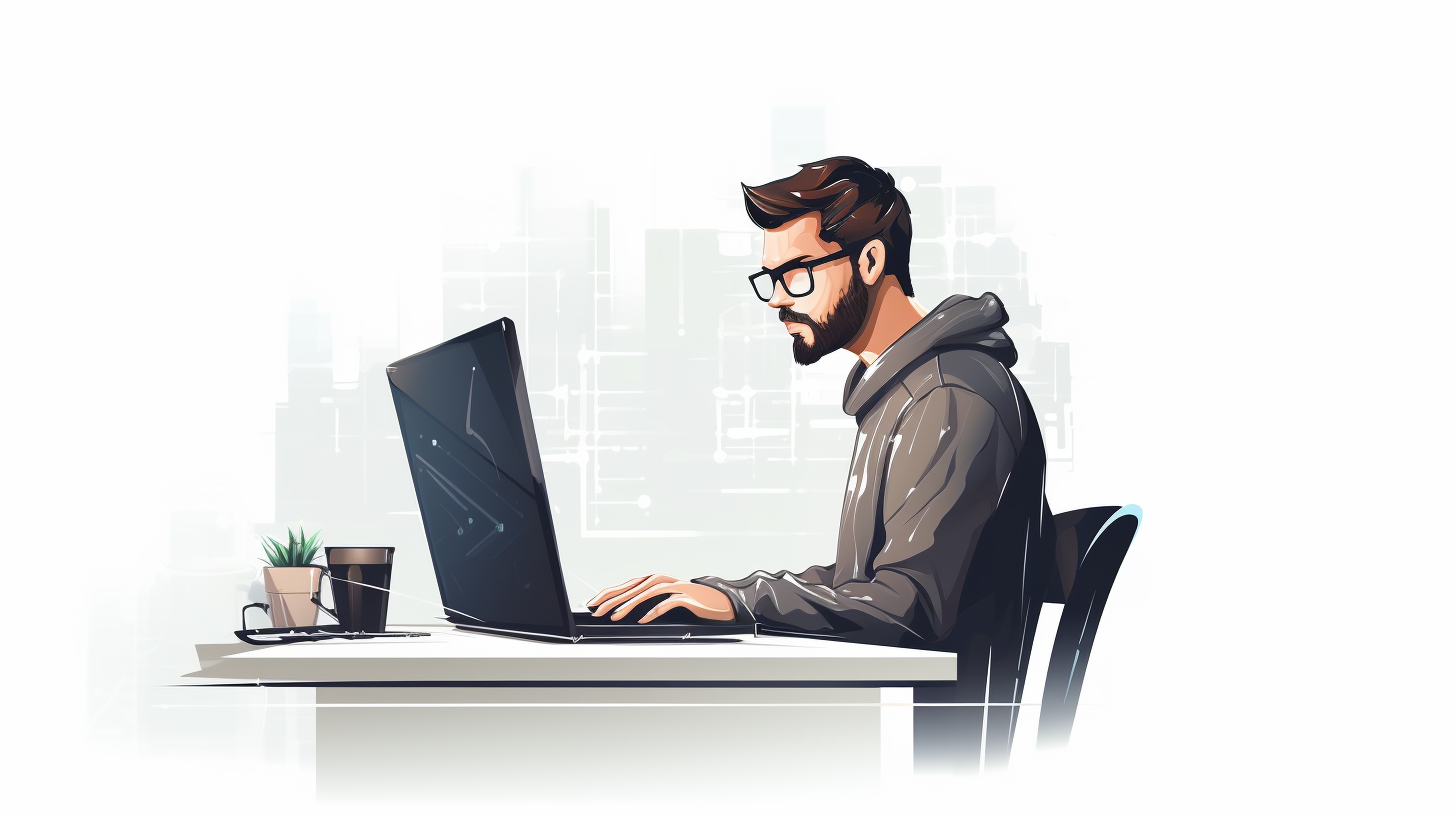
Inheritance in Swift
Inheritance in Swift is a powerful feature that allows one class to inherit the properties and methods of another class. This mechanism facilitates code reuse, making your applications more modular and maintainable. In Swift, classes can be subclassed, meaning that a new class can be created based on an existing class. The subclass can then extend or modify the behavior of the superclass, resulting in a hierarchy of classes that share common traits yet can exhibit unique functionalities.
When you define a class in Swift, you can specify a superclass from which it inherits. If no superclass is specified, the class implicitly inherits from the base class NSObject
. This establishes a chain of inheritance where properties and methods are accessible to subclasses, enhancing the overall organization of your code.
One fundamental aspect of inheritance in Swift is that it promotes the “is-a” relationship. This means that a subclass is a specialized version of its superclass. For example, if you have a superclass called Animal, you could have subclasses like Dog and Cat. Both subclasses inherit common properties and behaviors from Animal while also implementing their own specific characteristics.
class Animal { var name: String var sound: String init(name: String, sound: String) { self.name = name self.sound = sound } func makeSound() { print("(name) makes a (sound) sound.") } } class Dog: Animal { init(name: String) { super.init(name: name, sound: "bark") } } class Cat: Animal { init(name: String) { super.init(name: name, sound: "meow") } } let dog = Dog(name: "Buddy") let cat = Cat(name: "Whiskers") dog.makeSound() // Output: Buddy makes a bark sound. cat.makeSound() // Output: Whiskers makes a meow sound.
This structure not only allows for easy extension of functionality but also promotes encapsulation. Each class can encapsulate its specific behaviors while inheriting common functionality from its superclass. This allows for cleaner, more readable code.
It’s essential to understand that Swift supports single inheritance for classes, meaning a class can only inherit from one superclass. However, classes can adopt multiple protocols, which can help bridge the limitation of single inheritance by allowing classes to conform to various interfaces, thus achieving a form of multiple inheritance.
Types of Inheritance
In Swift, the types of inheritance can primarily be categorized into two main forms: single inheritance and multiple inheritance through protocol conformance. While Swift enforces single inheritance for classes, it allows for more flexibility in behavior through the use of protocols, which makes inheritance a versatile tool in your programming toolkit.
Single inheritance means that a class can inherit from only one superclass. This simplifies the inheritance hierarchy and reduces complexity, making it easier to follow the trail of method and property overrides. Ponder the following example:
class Vehicle { var numberOfWheels: Int init(numberOfWheels: Int) { self.numberOfWheels = numberOfWheels } func drive() { print("Driving a vehicle with (numberOfWheels) wheels.") } } class Car: Vehicle { var trunkSize: Int init(numberOfWheels: Int, trunkSize: Int) { self.trunkSize = trunkSize super.init(numberOfWheels: numberOfWheels) } override func drive() { print("Driving a car with (numberOfWheels) wheels and a trunk size of (trunkSize) liters.") } } let myCar = Car(numberOfWheels: 4, trunkSize: 500) myCar.drive() // Output: Driving a car with 4 wheels and a trunk size of 500 liters.
In this example, the Car
class inherits from the Vehicle
superclass. It can access and modify the behavior of the drive()
method, demonstrating the flexibility of single inheritance. The Car
class not only inherits characteristics from Vehicle
but also introduces new ones, like trunkSize
.
On the other hand, while Swift limits classes to single inheritance, it allows a class to adopt multiple protocols. This enables a form of multiple inheritance whereby a class can conform to several different interfaces, effectively inheriting behaviors and properties from multiple sources. Here’s an illustration:
protocol Drivable { func drive() } protocol Flyable { func fly() } class FlyingCar: Vehicle, Drivable, Flyable { func drive() { print("Driving a flying car with (numberOfWheels) wheels.") } func fly() { print("Flying high in the sky!") } } let myFlyingCar = FlyingCar(numberOfWheels: 4) myFlyingCar.drive() // Output: Driving a flying car with 4 wheels. myFlyingCar.fly() // Output: Flying high in the sky!
In this case, FlyingCar
inherits from Vehicle
and conforms to both Drivable
and Flyable
protocols. This allows FlyingCar
to implement the drive()
and fly()
methods, showcasing how Swift’s protocol-oriented programming can effectively serve as a workaround for single inheritance limitations.
Overriding Methods and Properties
Overriding methods and properties is an important aspect of inheritance in Swift. When a subclass inherits from a superclass, it can provide its own implementation of methods and properties defined in the superclass. This ability to override allows for specialization and customization of inherited behaviors, tailoring them to the specific needs of the subclass.
To override a method or property, you use the override keyword in the subclass. This explicitly indicates that you’re providing a new implementation that will replace the behavior defined in the superclass. It’s important to note that the method or property in the superclass must be marked with the open or public access modifier to allow overriding; otherwise, a compilation error will occur.
Here’s a simple example that illustrates method overriding:
class Shape { func area() -> Double { return 0.0 } } class Circle: Shape { var radius: Double init(radius: Double) { self.radius = radius } override func area() -> Double { return Double.pi * radius * radius } } class Square: Shape { var side: Double init(side: Double) { self.side = side } override func area() -> Double { return side * side } } let circle = Circle(radius: 5) let square = Square(side: 4) print("Area of the circle: (circle.area())") // Output: Area of the circle: 78.53981633974483 print("Area of the square: (square.area())") // Output: Area of the square: 16.0
In this example, the Shape class defines a method called area() that returns a default value. Both Circle and Square subclasses override this method to provide their specific implementations, calculating the area based on their respective geometries. When you call the area() method on instances of these subclasses, you get the appropriate area calculation, demonstrating how overriding allows subclasses to customize inherited methods.
Properties can also be overridden in a similar fashion. You can override both stored properties and computed properties. Here’s how you might do this with a computed property:
class Vehicle { var topSpeed: Double { return 0.0 } } class Car: Vehicle { override var topSpeed: Double { return 150.0 } } class Bicycle: Vehicle { override var topSpeed: Double { return 20.0 } } let myCar = Car() let myBicycle = Bicycle() print("Car's top speed: (myCar.topSpeed) km/h") // Output: Car's top speed: 150.0 km/h print("Bicycle's top speed: (myBicycle.topSpeed) km/h") // Output: Bicycle's top speed: 20.0 km/h
In this case, the Vehicle class has a computed property topSpeed that returns a default value. The subclasses Car and Bicycle override this property to return their respective top speeds. This allows for polymorphic behavior, where the same property name produces different results based on the instance type.
It’s also worth mentioning that if you want to call the superclass’s implementation of a method or property within the overridden implementation in the subclass, you can do so using the super keyword. This allows you to extend the behavior of the superclass rather than completely replacing it.
class Animal { var sound: String { return "Generic animal sound" } func makeSound() { print(sound) } } class Dog: Animal { override var sound: String { return "Woof!" } override func makeSound() { super.makeSound() // Calls sound property from superclass print("Dogs bark loudly!") } } let myDog = Dog() myDog.makeSound() // Output: Woof! // Dogs bark loudly!
This example shows how the Dog subclass overrides both the sound property and the makeSound() method. The super.makeSound() call within the overridden method allows the subclass to utilize the superclass functionality while also adding its own unique behavior.
Using Superclass and Subclass
In Swift, working with superclasses and subclasses is central to using the full power of inheritance. When you define a subclass, it inherently gains access to the properties and methods of its superclass. This access allows subclasses to utilize common logic while also providing the capability to specialize or override certain behaviors as needed. Understanding how to effectively use superclasses and subclasses allows you to build well-structured, modular, and reusable code.
To illustrate this, let’s ponder a simple example involving a superclass called `Vehicle` and a subclass called `Car`. The `Vehicle` class defines some common attributes and methods that all vehicles would share, such as `numberOfWheels` and the method `drive()`. On the other hand, the `Car` subclass can add specific attributes like `trunkSize` while using the inherited properties and methods from `Vehicle`.
class Vehicle { var numberOfWheels: Int init(numberOfWheels: Int) { self.numberOfWheels = numberOfWheels } func drive() { print("Driving a vehicle with (numberOfWheels) wheels.") } } class Car: Vehicle { var trunkSize: Int init(numberOfWheels: Int, trunkSize: Int) { self.trunkSize = trunkSize super.init(numberOfWheels: numberOfWheels) } override func drive() { print("Driving a car with (numberOfWheels) wheels and a trunk size of (trunkSize) liters.") } } let myCar = Car(numberOfWheels: 4, trunkSize: 500) myCar.drive() // Output: Driving a car with 4 wheels and a trunk size of 500 liters.
In this example, the `Car` class not only inherits the `numberOfWheels` property and the `drive()` method from the `Vehicle` superclass but also overrides the `drive()` method to incorporate its own details regarding trunk size. This illustrates how subclasses can build upon the foundation set by their superclasses while also customizing certain behaviors to fit their specific context.
It’s also important to note that the `super` keyword plays an important role in scenarios where a subclass needs to access the properties or methods of its superclass. By calling `super.init()`, you ensure that the superclass’s initializer is executed, setting up the inherited properties correctly. Similarly, within an overridden method, calling `super.methodName()` allows you to invoke the superclass’s implementation, which can be particularly useful if you want to extend rather than completely replace the behavior.
class ElectricCar: Car { var batteryLife: Int init(numberOfWheels: Int, trunkSize: Int, batteryLife: Int) { self.batteryLife = batteryLife super.init(numberOfWheels: numberOfWheels, trunkSize: trunkSize) } override func drive() { super.drive() // Calls the drive method from Car print("Battery life: (batteryLife) hours.") } } let myElectricCar = ElectricCar(numberOfWheels: 4, trunkSize: 400, batteryLife: 24) myElectricCar.drive() // Output: Driving a car with 4 wheels and a trunk size of 400 liters. // Battery life: 24 hours.
In the `ElectricCar` subclass, we see another layer of inheritance. It not only inherits from `Car` but also adds a new property called `batteryLife`. When the `drive()` method is called on the `ElectricCar` instance, it first invokes the `drive()` method from the `Car` superclass and subsequently adds its own unique behavior. This kind of structured layering allows for clear relationships and behavior specialization across your class hierarchy.
Protocol Inheritance in Swift
In Swift, protocol inheritance provides a powerful mechanism that allows protocols to inherit from one or more other protocols. This kind of inheritance is distinct from class inheritance, as it focuses on defining a set of methods and properties that can be adopted by any conforming types, regardless of their class hierarchy. This feature enhances the flexibility of your code and promotes a protocol-oriented design, which is a cornerstone of Swift programming.
When you define a protocol in Swift, you can specify that it inherits from one or more other protocols. This means that any type conforming to the derived protocol will also be required to implement the methods and properties of its parent protocols. That is particularly useful for defining common interfaces that can be shared across different types while still allowing for specialized behavior in each conforming type.
protocol Vehicle { var numberOfWheels: Int { get } func drive() } protocol Electric { var batteryLife: Int { get } } protocol ElectricVehicle: Vehicle, Electric { func charge() }
In this example, we define a base Vehicle
protocol that outlines the properties and methods associated with vehicles. The Electric
protocol specifies an additional requirement related to battery life. The ElectricVehicle
protocol inherits from both Vehicle
and Electric
. Consequently, any type conforming to ElectricVehicle
must implement all requirements from the three protocols.
struct Tesla: ElectricVehicle {
var numberOfWheels: Int
var batteryLife: Intfunc drive() {
print("Driving a Tesla with (numberOfWheels) wheels.")
}