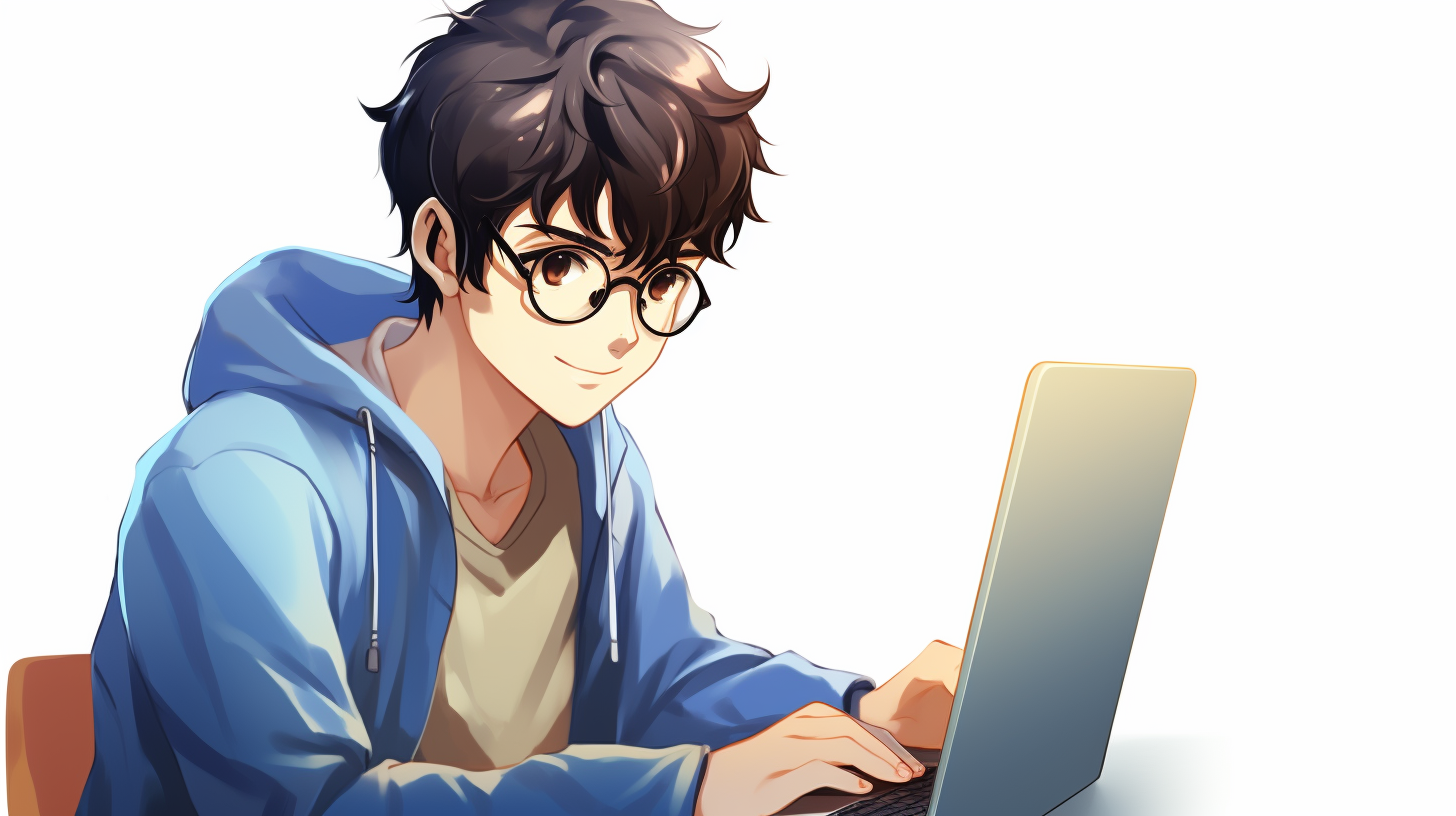
SQL Analytics with OLAP Functions
OLAP (Online Analytical Processing) functions in SQL are powerful tools designed to perform complex calculations across a set of rows that are related to the current row, making them invaluable for analytical queries. Unlike standard aggregate functions that return a single result for a group of rows, OLAP functions allow you to retain the details of each row while providing aggregated results. This dual capability enables deeper insights into data without sacrificing granularity.
At the core of OLAP functions are the concepts of windowing and partitioning. Each OLAP function operates within a defined window of rows, which can be adjusted based on specific criteria. This feature allows for sophisticated analyses, such as running totals, moving averages, and ranking, all of which can be tailored to meet the specific needs of your data context.
Here are some key elements of OLAP functions:
- This defines the subset of rows over which the OLAP function will operate. You can specify the rows to include with
ROWS
orRANGE
clauses. - This divides the result set into partitions to which the OLAP function is applied separately. For example, you might partition data by a column such as
department
orregion
. - The order in which rows are processed can significantly affect results, especially for ranking functions. You can define the order using the
ORDER BY
clause within the window function.
To demonstrate how OLAP functions work, think the following SQL example that computes a running total of sales over time:
SELECT sale_date, sales_amount, SUM(sales_amount) OVER (ORDER BY sale_date) AS running_total FROM sales ORDER BY sale_date;
In this example, the SUM
function is used as an OLAP function with the OVER
clause. The result is a cumulative total of sales that updates with each row, providing a clear view of sales trends over time.
Another common use of OLAP functions is ranking. The ROW_NUMBER
, RANK
, and DENSE_RANK
functions allow you to assign ranks to rows within partitions. Here’s how to implement DENSE_RANK
to rank sales by amount within each department:
SELECT department, employee, sales_amount, DENSE_RANK() OVER (PARTITION BY department ORDER BY sales_amount DESC) AS sales_rank FROM employee_sales;
Here, the DENSE_RANK
function partitions the results by department
, ranking employees based on their sales_amount
in descending order. This is particularly useful for identifying top performers within individual departments.
Types of OLAP Functions: A Comprehensive Overview
When it comes to OLAP functions in SQL, there are primarily three types that stand out: aggregate functions, ranking functions, and analytical functions. Each serves a distinct purpose and can be used in various scenarios to extract meaningful insights from data. Understanding these categories will empower you to design more effective analytical queries.
1. Aggregate Functions: These functions compute a single result from a set of values, but when used as OLAP functions, they can provide results while still retaining the detail of individual rows. Common aggregate functions include SUM, AVG, COUNT, MIN, and MAX. What sets them apart when employed as OLAP functions is the ability to operate over a defined window of rows, allowing dynamic calculations that adjust contextually based on the partitioning and ordering specified.
SELECT employee_id, department, sales_amount, AVG(sales_amount) OVER (PARTITION BY department) AS avg_sales_by_department FROM employee_sales;
In this example, the AVG function calculates the average sales amount per department, providing insight into departmental performance while retaining individual sales records.
2. Ranking Functions: These functions assign a sequential rank to rows within a partition, enabling the identification of top performers or specific standings among a set of data. The primary ranking functions include ROW_NUMBER(), RANK(), and DENSE_RANK(). Each function has unique behaviors, particularly when handling ties.
SELECT employee_id, sales_amount, RANK() OVER (ORDER BY sales_amount DESC) AS sales_rank FROM employee_sales;
In this case, RANK assigns a rank based on sales_amount, where ties receive the same rank but may leave gaps in the ranking sequence. That’s particularly useful for scenarios where distinguishing among equally performing individuals is critical.
3. Analytical Functions: Analytical functions provide a more sophisticated means to perform calculations across sets of rows while still retaining the detail of each row. Functions such as LEAD and LAG fall into this category and allow for comparisons between rows. LEAD retrieves data from subsequent rows, while LAG pulls data from preceding rows. This capability is particularly useful for trend analysis and time series data.
SELECT sale_date, sales_amount, LAG(sales_amount, 1) OVER (ORDER BY sale_date) AS previous_sales, sales_amount - LAG(sales_amount, 1) OVER (ORDER BY sale_date) AS sales_difference FROM sales;
Here, the LAG function provides the previous day’s sales amount alongside the current day’s, allowing for direct comparison and calculation of sales differences. This insight can highlight trends in sales performance over time.
Practical Use Cases for OLAP Functions
When it comes to practical applications of OLAP functions in SQL, the possibilities are vast and versatile, aligning closely with common business analytics scenarios. Let’s explore several use cases where OLAP functions can significantly enhance data analysis and reporting capabilities.
One of the most prevalent use cases for OLAP functions is in sales analysis. Businesses often need to evaluate their sales performance across different dimensions, such as time, product categories, or geographical regions. For instance, calculating cumulative sales over a fiscal year can help stakeholders understand sales growth trends. The following SQL query demonstrates how to achieve this using the SUM function as an OLAP function:
SELECT sale_month, sales_amount, SUM(sales_amount) OVER (ORDER BY sale_month) AS cumulative_sales FROM monthly_sales ORDER BY sale_month;
In this example, the cumulative_sales column provides real-time insights into total sales up to each month, empowering the business to make informed decisions based on the observed trend.
Another compelling use case is employee performance tracking. Companies often wish to identify top performers within teams or departments. By employing ranking functions like RANK() or DENSE_RANK(), organizations can easily highlight employees who exceed sales targets. The following query ranks employees based on their sales performance within each department:
SELECT department, employee_name, sales_amount, RANK() OVER (PARTITION BY department ORDER BY sales_amount DESC) AS sales_rank FROM employee_sales;
This query partitions the results by department and ranks employees according to their sales_amount within those partitions. It effectively identifies who are the top salespeople, enabling targeted recognition or incentive programs.
Time series analysis is yet another area where OLAP functions find extensive application. Analysts often need to compare current performance against previous periods. Functions like LAG() can be incredibly useful here. For example, a business may want to analyze monthly sales growth:
SELECT sale_month, sales_amount, LAG(sales_amount, 1) OVER (ORDER BY sale_month) AS previous_month_sales, sales_amount - LAG(sales_amount, 1) OVER (ORDER BY sale_month) AS sales_growth FROM monthly_sales;
This query calculates the sales for the previous month and the difference in sales from that period. The resulting sales_growth gives insights into how well the business is doing compared to the previous month, allowing for timely adjustments in strategy or operations.
Moreover, customer behavior analysis can also leverage OLAP functions. By using window functions to analyze purchase patterns, businesses can ascertain customer loyalty and identify opportunities for cross-selling or upselling. Think a scenario where a company wants to assess how frequently customers make purchases over a specified period:
SELECT customer_id, purchase_date, COUNT(purchase_date) OVER (PARTITION BY customer_id ORDER BY purchase_date RANGE BETWEEN INTERVAL '30' DAY PRECEDING AND CURRENT ROW) AS purchases_last_30_days FROM customer_purchases;
This example uses a COUNT function to quantify the number of purchases made by each customer in the last 30 days. Such insights can inform marketing strategies and promotional efforts tailored to customer needs.
OLAP functions also shine in financial reporting, where businesses often need to calculate year-to-date figures or trailing twelve-month metrics. A typical approach would involve summing financial values over time while retaining detail for individual transactions:
SELECT transaction_date, transaction_amount, SUM(transaction_amount) OVER (ORDER BY transaction_date) AS year_to_date FROM financial_transactions WHERE transaction_date >= '2023-01-01';
This SQL statement calculates the year-to-date totals for financial transactions, providing a comprehensive view of performance within a fiscal year.
Performance Optimization Techniques for OLAP Queries
When it comes to optimizing performance for OLAP queries, several strategies can be employed to ensure that your SQL operations run efficiently, particularly when dealing with large datasets. The inherent complexity of OLAP functions can sometimes lead to performance bottlenecks, so understanding how to mitigate these challenges is essential for database administrators and developers alike.
One of the primary techniques for optimizing OLAP queries is to reduce the volume of data being processed. This can often be achieved through the use of filters or WHERE clauses to limit the dataset before applying OLAP functions. For instance, if you’re interested in analyzing sales data for the last quarter, you can filter the data beforehand:
SELECT sale_date, sales_amount, SUM(sales_amount) OVER (ORDER BY sale_date) AS running_total FROM sales WHERE sale_date >= '2023-07-01' AND sale_date < '2023-10-01' ORDER BY sale_date;
This query limits the dataset to only the relevant quarter, allowing the OLAP function to operate on a smaller, more manageable amount of data, which can significantly improve performance.
Additionally, indexing plays a vital role in enhancing the performance of OLAP queries. By creating indexes on columns that are frequently used in the ORDER BY or PARTITION BY clauses, you can expedite the retrieval process. For example, if your sales data is regularly queried by sale_date, consider creating an index on that column:
CREATE INDEX idx_sale_date ON sales(sale_date);
With this index in place, SQL can quickly locate the relevant rows, thus speeding up the execution of OLAP functions that rely on this ordering.
Another optimization technique is to evaluate the use of materialized views. Materialized views store the results of a query physically, which can save time on complex aggregations. When OLAP functions are frequently employed on a specific dataset, using a materialized view can provide quick access to pre-computed results:
CREATE MATERIALIZED VIEW mv_sales_summary AS SELECT sale_date, SUM(sales_amount) AS total_sales, AVG(sales_amount) AS avg_sales FROM sales GROUP BY sale_date;
By querying the materialized view instead of the base table, you can avoid the overhead of recalculating aggregates every time, thereby improving performance.
Moreover, consider the execution plan of your queries. Understanding how SQL Server or your database management system processes your queries can provide valuable insights into potential inefficiencies. Use the EXPLAIN command to analyze how your OLAP queries are executed and identify areas for improvement.
EXPLAIN SELECT department, employee_name, sales_amount, DENSE_RANK() OVER (PARTITION BY department ORDER BY sales_amount DESC) AS sales_rank FROM employee_sales;
This analysis can reveal whether indexes are being utilized effectively or if any full table scans are occurring, which can slow down performance.
Lastly, consider the impact of partitioning strategies on your OLAP queries. Properly partitioning your tables can make OLAP calculations more efficient. For instance, if you have a large sales table, partitioning it by year or by month can help SQL engines operate on smaller subsets of data, improving query response times:
CREATE TABLE sales ( sale_id INT, sale_date DATE, sales_amount DECIMAL(10, 2) ) PARTITION BY RANGE (YEAR(sale_date)) ( PARTITION p2023 VALUES LESS THAN (2024), PARTITION p2024 VALUES LESS THAN (2025) );
This approach not only enhances performance but also simplifies data management, allowing for efficient archiving and purging of old data.
Integrating OLAP Functions with Other SQL Features
Integrating OLAP functions with other SQL features can dramatically enhance your analytical capabilities, allowing for more sophisticated and nuanced data analysis. One of the primary benefits of OLAP functions is their ability to work seamlessly with various SQL constructs, such as joins, subqueries, and common table expressions (CTEs). This integration empowers users to leverage the full power of SQL for comprehensive data analysis.
One powerful combination is the use of OLAP functions with joins. By joining multiple tables, you can enrich the dataset upon which OLAP functions operate, providing deeper insights. Think a scenario where you want to analyze sales data in conjunction with customer demographics. Here’s how you would structure the query:
SELECT c.customer_id, c.customer_name, s.sale_date, s.sales_amount, SUM(s.sales_amount) OVER (PARTITION BY c.customer_id ORDER BY s.sale_date) AS cumulative_sales FROM customers c JOIN sales s ON c.customer_id = s.customer_id ORDER BY c.customer_id, s.sale_date;
In this example, the cumulative sales for each customer are calculated while also reflecting the customer details. The integration of OLAP functions with joins provides a holistic view of customer behavior over time, which is essential for targeted marketing strategies.
Another effective integration involves using OLAP functions with subqueries to perform advanced calculations. Subqueries can pre-aggregate data or refine the dataset that OLAP functions will analyze. For instance, if you want to calculate the average sales per department and then rank those averages, you could structure your query like this:
SELECT department, avg_sales, RANK() OVER (ORDER BY avg_sales DESC) AS sales_rank FROM (SELECT department, AVG(sales_amount) AS avg_sales FROM employee_sales GROUP BY department) AS department_sales;
Here, the subquery computes the average sales for each department, and the outer query applies the RANK() OLAP function to rank the departments based on their average sales. This layered approach allows for clean and efficient calculations.
Common Table Expressions (CTEs) can also be a valuable tool when integrating OLAP functions. CTEs can simplify complex queries and improve readability. For example, to analyze sales data and calculate a moving average, you could structure your query as follows:
WITH SalesData AS ( SELECT sale_date, sales_amount FROM sales ) SELECT sale_date, sales_amount, AVG(sales_amount) OVER (ORDER BY sale_date ROWS BETWEEN 6 PRECEDING AND CURRENT ROW) AS moving_average FROM SalesData ORDER BY sale_date;
This approach uses a CTE to isolate the sales data, allowing the main query to cleanly apply the moving average calculation over a defined window. The moving average effectively smooths out short-term fluctuations, providing clearer insights into sales trends.
Moreover, combining OLAP functions with conditional logic, such as CASE statements, can yield even more powerful analytics. For instance, you might want to categorize sales performance while also calculating cumulative totals:
SELECT employee_id, sales_amount, SUM(sales_amount) OVER (ORDER BY sale_date) AS running_total, CASE WHEN sales_amount >= 10000 THEN 'High Performer' WHEN sales_amount >= 5000 THEN 'Moderate Performer' ELSE 'Low Performer' END AS performance_category FROM employee_sales ORDER BY sale_date;
In this query, the sales amounts are categorized based on performance thresholds while at the same time calculating a running total. This dual functionality allows for a more dynamic analysis of employee performance against their sales contributions.
Finally, the integration of OLAP functions with windowing techniques enhances the ability to perform time-based analyses. For example, using LEAD or LAG functions in conjunction with date functions can reveal trends over time.
SELECT sale_date, sales_amount, LAG(sales_amount, 1) OVER (ORDER BY sale_date) AS previous_sales, sales_amount - LAG(sales_amount, 1) OVER (ORDER BY sale_date) AS sales_difference FROM sales ORDER BY sale_date;
This example illustrates how OLAP functions can be used to compare current sales figures to those from the previous period, facilitating an understanding of growth or decline in sales performance.
Best Practices for Implementing OLAP Solutions in SQL
Implementing OLAP solutions in SQL requires a strategic approach to ensure that you maximize the benefits of these powerful analytical tools while maintaining clarity and performance in your queries. Here are some best practices to guide you through the implementation process:
1. Clearly Define Your Analytical Requirements: Before writing any SQL code, spend time understanding the specific analytical questions you need to answer. Whether it’s calculating averages, identifying trends, or ranking items, being clear about your requirements will help you choose the right OLAP functions and structure your queries effectively.
2. Use Appropriate Window Frames: OLAP functions operate over a set of rows defined by a window frame. It especially important to define this frame correctly to ensure that your calculations yield meaningful results. Use the ROWS or RANGE clauses wisely to specify how many preceding or following rows to include in your calculations. For example:
SELECT sale_date, sales_amount, SUM(sales_amount) OVER (ORDER BY sale_date ROWS BETWEEN 6 PRECEDING AND CURRENT ROW) AS moving_average FROM sales ORDER BY sale_date;
This query calculates a moving average for the last six months, keeping the analysis robust and aligned with your data context.
3. Partition Your Data Strategically: Partitioning is a powerful way to enhance the performance of OLAP functions. By dividing your data into smaller segments, you can apply OLAP functions more efficiently. Consider partitioning based on logical groupings such as department, region, or time period. For example:
SELECT department, sales_amount, DENSE_RANK() OVER (PARTITION BY department ORDER BY sales_amount DESC) AS sales_rank FROM employee_sales;
Here, the sales data is partitioned by department, making the analysis more relevant and efficient.
4. Leverage Indexing: Indexing can significantly accelerate the performance of OLAP queries. When you create indexes on columns used in your ORDER BY or PARTITION BY clauses, SQL can retrieve the necessary rows much faster. For example:
CREATE INDEX idx_sales_date ON sales(sale_date);
This index on the sale_date column will improve performance for queries that analyze sales over time.
5. Avoid Overly Complex Queries: While OLAP functions are powerful, creating overly complex queries can lead to performance issues and make your SQL hard to read and maintain. Break down complicated calculations into simpler components using Common Table Expressions (CTEs) or nested queries. This not only enhances clarity but also helps in debugging and optimizing performance.
WITH SalesData AS ( SELECT sale_date, sales_amount FROM sales ) SELECT sale_date, sales_amount, AVG(sales_amount) OVER (ORDER BY sale_date ROWS BETWEEN 6 PRECEDING AND CURRENT ROW) AS moving_average FROM SalesData ORDER BY sale_date;
Using a CTE in this example simplifies the query structure and assists in isolating the sales data for analysis.
6. Test and Optimize Queries: It’s essential to run performance tests on your OLAP queries, especially when dealing with large datasets. Utilize execution plans to analyze how SQL processes your queries. Look for any potential bottlenecks, such as full table scans, suggesting areas for optimization. The EXPLAIN command can provide valuable insights:
EXPLAIN SELECT department, employee_name, sales_amount, RANK() OVER (PARTITION BY department ORDER BY sales_amount DESC) AS sales_rank FROM employee_sales;
By understanding the execution plan, you can adjust your indexes, partitioning, or query structure for better performance.
7. Document Your Queries: Good documentation is vital for maintaining OLAP solutions over time. Include comments within your SQL code to explain the purpose and logic of each part of the query. This practice not only aids in future modifications but also helps other team members understand your analytical processes.
8. Monitor Performance Post-Implementation: After deploying your OLAP solutions, continuously monitor their performance and usage. Keep an eye on the execution times and adjust your strategies based on real-world usage patterns. This ongoing evaluation will help ensure that your OLAP functions remain efficient and relevant.