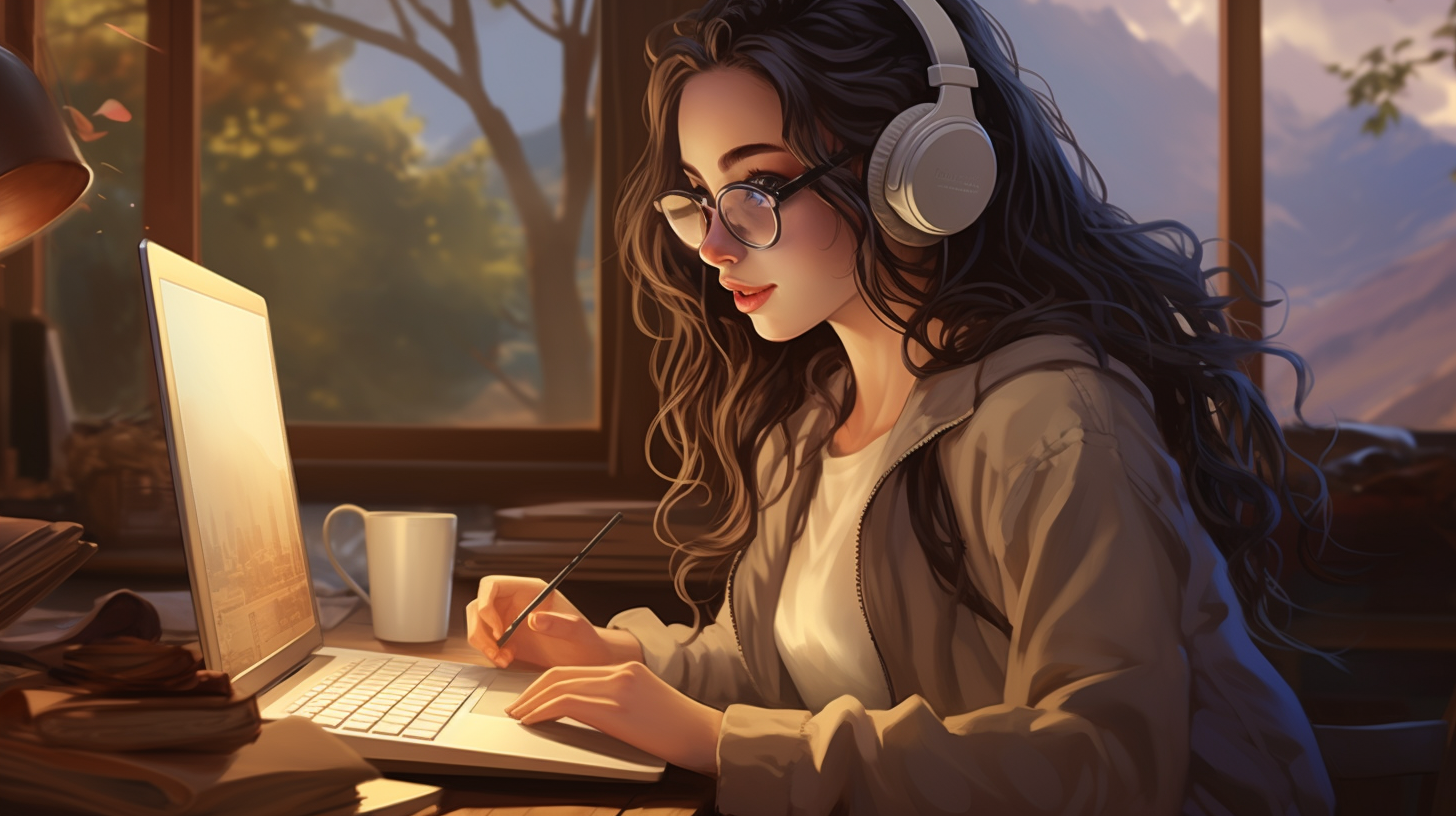
SQL Data Type Conversion and Casting
In SQL, data types are crucial as they define the nature of data that can be stored in a database. Understanding these types is fundamental for effective database design, ensuring data integrity, and optimizing query performance. SQL provides a variety of data types that can be broadly categorized into several groups:
- These include types like
INT
,FLOAT
,DECIMAL
, etc., which are used to store numeric values. - These types, such as
CHAR
,VARCHAR
, andTEXT
, are used to store string data. - Types like
DATE
,TIME
, andDATETIME
are utilized for storing date and time values. - The
BOOLEAN
type is used to store truth values, typically represented asTRUE
orFALSE
. - These types, such as
BINARY
andVARBINARY
, are for storing binary data like images or files.
Choosing the correct data type is pivotal as it affects both storage requirements and performance. For example, using TINYINT
instead of INT
for a value that only ranges from 0 to 255 can significantly reduce storage space. Conversely, using a larger type when not necessary can lead to inefficient data handling and processing.
Here are some common numeric data types and their storage characteristics:
-- Numeric Types CREATE TABLE example_numeric ( small_num TINYINT, -- 1 byte medium_num SMALLINT, -- 2 bytes large_num INT, -- 4 bytes very_large_num BIGINT -- 8 bytes );
Character data types, on the other hand, allow for flexible string handling:
-- Character Types CREATE TABLE example_character ( fixed_length CHAR(10), -- Fixed length of 10 variable_length VARCHAR(255) -- Variable length up to 255 );
Date and time types are indispensable for any application that deals with time-stamped data:
-- Date and Time Types CREATE TABLE example_datetime ( event_date DATE, event_time TIME, event_timestamp DATETIME );
Understanding SQL data types also extends to their compatibility rules and how they interact with one another during operations. For instance, if you attempt to add a string to a number, SQL will trigger either implicit or explicit conversion based on the context, which can lead to unexpected results if not managed properly.
By grasping the nuances of each data type and using them effectively, SQL developers can create more robust, efficient, and maintainable databases.
Implicit vs. Explicit Conversion
Within the scope of SQL, understanding the distinction between implicit and explicit conversion is essential, as it directly influences how data is interpreted and manipulated during operations. Implicit conversion, also referred to as automatic conversion, is when SQL automatically converts one data type into another without requiring any explicit instruction from the developer. This occurs in scenarios where the system recognizes that a conversion is necessary to perform a specific operation.
For example, when a numeric type is combined with a string type in an arithmetic operation, SQL may implicitly convert the string to a number if the string is a valid representation of a number. Here’s a simpler illustration:
SELECT 10 + '20'; -- Implicitly converts '20' to 20
The result of this operation would be 30, as SQL seamlessly converts the string ’20’ into the integer 20. However, while implicit conversion can simplify coding, it may also introduce subtle bugs if the developer is unaware of the conversion behavior.
On the other hand, explicit conversion requires developers to specify the conversion command themselves, often using functions like CAST() or CONVERT(). This approach grants greater control over the conversion process and helps avoid ambiguity. For instance, if you want to ensure that a numeric value is treated as a string, you would use explicit conversion as follows:
SELECT CAST(10 AS VARCHAR(10)) + '20'; -- Explicitly converts 10 to '10'
In this case, the result would be ‘1020’, as the numeric 10 is explicitly converted to a string before concatenation with ’20’. Explicit conversions can also prevent unexpected outcomes that arise from implicit conversions, making them a preferred choice in many scenarios.
SQL’s handling of implicit and explicit conversions is not uniform across all databases. Different SQL dialects might have varying rules and behaviors. For example, in SQL Server, implicit conversion is more permissive, while in stricter systems, it may throw an error if the types are incompatible. Understanding these nuances is critical for writing portable and robust SQL code.
Ultimately, the key takeaway is to be aware of which type of conversion is taking place in your queries. Relying on implicit conversion can sometimes lead to unforeseen issues, while explicit conversion provides clarity and control over data handling, helping maintain the integrity of your operations.
Using CAST and CONVERT Functions
When it comes to converting data types in SQL, the CAST and CONVERT functions are the primary tools at a developer’s disposal. Both functions allow you to transform one data type into another explicitly, thus affording you the precision necessary for effective data manipulation.
The CAST function is used to change a data type into another, providing a simpler syntax. The general form of the CAST function is as follows:
CAST(expression AS target_data_type)
For example, if you have a decimal value and you want to convert it to an integer, you would use the CAST function like this:
SELECT CAST(123.45 AS INT) AS ConvertedValue;
This would return 123, as the decimal portion is truncated in the conversion process. It’s essential to note that the CAST function can handle a variety of data types, including numeric, string, and date types, making it highly versatile.
In contrast, the CONVERT function is more specific to SQL Server and provides additional options, such as formatting for date and time types. The syntax for the CONVERT function is as follows:
CONVERT(target_data_type, expression [, style])
The style parameter is optional and indicates how the conversion should be performed, particularly useful for formatting date and time data. For instance, to convert a datetime to a string in a specific format, you could do the following:
SELECT CONVERT(VARCHAR(10), GETDATE(), 101) AS FormattedDate;
This would yield a date formatted as MM/DD/YYYY, using the style parameter that defines the output format. The power of the CONVERT function lies in its ability to handle complex transformations with formatting options, which is valuable in reporting and data presentation.
While both CAST and CONVERT serve the purpose of data type conversion, the choice between them often boils down to the specific requirements of your SQL environment and the desired outcome. If you seek simplicity and broad compatibility, CAST might be your best bet. However, if you’re working within SQL Server and need more control, especially over date formatting, CONVERT could be the preferred option.
In practice, using either function requires careful consideration of the data types involved. Converting incompatible types can lead to errors or unexpected results, so it’s prudent to validate your expressions before executing conversions. For example:
SELECT CAST('ABC' AS INT); -- This will fail!
This statement will throw an error since ‘ABC’ cannot be converted to an integer. Being aware of the nuances of each function and the data types at play will help you write more resilient SQL code.
Ultimately, mastering the use of CAST and CONVERT functions enhances your ability to manipulate data effectively, paving the way for cleaner, more efficient database operations.
Handling Null Values in Conversion
Handling NULL values during data type conversion is an important aspect of SQL programming, as NULL represents the absence of a value and can complicate operations that involve conversion. When dealing with NULL values, it is essential to understand how SQL behaves during conversions, as this can lead to unexpected results if not carefully managed.
In SQL, when a NULL value is encountered, the result of most expressions involving that NULL will also be NULL. This behavior extends to conversions as well. For instance, if you attempt to convert a NULL value to another data type, the result remains NULL. Here’s a simple example to illustrate this:
SELECT CAST(NULL AS INT) AS ConvertedValue;
In this example, the output will yield a NULL result. It is important to note that that is the expected behavior, as NULL signifies that there is no value to convert. Therefore, any conversion attempt involving NULL will not produce a valid output.
To handle NULL values effectively during conversions, you can utilize SQL’s COALESCE or ISNULL functions. These functions allow you to provide a default value that will be used when a NULL is encountered. This approach can help to avoid NULLs in your results and ensure that you have meaningful data to work with. Here’s how you can use COALESCE:
SELECT COALESCE(CAST(NULL AS INT), 0) AS ConvertedValue;
In this case, if the NULL is encountered, it will be replaced with 0, resulting in the output of 0 rather than NULL. This can be particularly useful in scenarios where NULL values might disrupt your calculations or reporting.
Another important consideration when dealing with NULL values is the context of data type conversion. If you’re performing operations that involve both NULL and non-NULL values, the presence of NULL can change the outcome of your query. For instance:
SELECT NULL + 5 AS Result;
The result of this operation will be NULL, demonstrating that adding NULL to any numeric value results in NULL rather than a numeric result. To mitigate such issues, you can utilize the same COALESCE function to provide defaults:
SELECT COALESCE(NULL, 0) + 5 AS Result;
Here, the NULL would be replaced by 0 before the addition, yielding a result of 5.
Moreover, it is important to be aware of the underlying data types when dealing with NULLs. Each database management system may have slightly different behavior regarding NULL handling, especially in type conversion contexts. Hence, consulting the documentation specific to your SQL dialect is recommended to fully understand how NULLs will be treated during operations.
Effective handling of NULL values during data type conversion is vital for maintaining the integrity of your SQL queries. By using functions like COALESCE or ISNULL, you can ensure that your data manipulations yield meaningful results while avoiding the pitfalls of unforeseen NULL occurrences.
Common Errors and Pitfalls
When working with SQL data types and their conversions, developers often encounter a variety of common errors and pitfalls that can lead to unexpected behaviors or results. Recognizing and understanding these issues is essential for writing robust SQL code that reliably produces the desired outcomes.
One prevalent pitfall is attempting to convert incompatible data types without proper checks. For instance, when a string that cannot be numerically interpreted is converted to an integer, an error will occur. Think the following example:
SELECT CAST('XYZ' AS INT); -- This will fail!
This statement will throw an error, as ‘XYZ’ cannot be converted into an integer. Developers must ensure that the data being converted is valid for the target data type to prevent runtime errors.
Another issue arises with implicit conversions, which can lead to unexpected results if not carefully managed. For example, if you mistakenly perform mathematical operations involving a string and an integer, SQL may implicitly convert the string, but the results may not match your expectations:
SELECT '10' + 5; -- This will concatenate to '105' instead of adding
Here, SQL treats the integer 5 as a string, resulting in a concatenation rather than an arithmetic addition. Such unexpected behaviors highlight the need for developers to be vigilant about the data types involved in their operations.
Data truncation is another common error during conversions. When converting from a larger data type to a smaller one, the potential for data loss exists. For instance, converting a decimal to an integer will truncate the decimal portion:
SELECT CAST(123.456 AS INT) AS TruncatedValue; -- This will yield 123
This behavior can lead to discrepancies in the database if not anticipated, particularly in scenarios where precision especially important. It’s advisable to carefully evaluate the implications of such truncations and handle them appropriately, perhaps by rounding or adjusting the data beforehand.
Furthermore, errors may arise from the handling of NULL values during conversions. As previously noted, when NULL is involved, the result will typically also be NULL, which can disrupt calculations:
SELECT NULL + 10 AS Result; -- This will yield NULL
To mitigate this, developers should use functions like COALESCE to provide default values, thereby ensuring calculations yield meaningful results even in the presence of NULLs:
SELECT COALESCE(NULL, 0) + 10 AS Result; -- This will yield 10
Additionally, cross-compatibility between different SQL dialects can lead to confusion. Behavior that’s standard in one system may not hold true in another. For instance, how certain databases handle implicit conversions or string formatting can vary, leading to unexpected errors when moving between environments. Developers should familiarize themselves with the specific behaviors of the SQL dialect they’re using to avoid surprises.
Ultimately, being aware of these common errors and pitfalls during data type conversion is vital for any SQL developer. Through cautious coding practices, thorough testing, and a solid grasp of data type behavior, developers can navigate these challenges and build more resilient SQL applications.
Best Practices for Data Type Conversion
When it comes to ensuring effective data type conversion in SQL, employing best practices can significantly enhance the reliability and performance of your database operations. Here are key recommendations that every developer should consider:
1. Understand Your Data: Before undertaking any conversion, it is crucial to have a comprehensive understanding of the data you are working with. This includes knowing the source data type, the target data type, and the nature of the data itself. For example, if you’re converting a VARCHAR containing dates, ensure that the format is consistent to avoid conversion errors.
2. Use Explicit Conversions: While SQL provides implicit conversion capabilities, relying on them can lead to unexpected results. To maintain clarity in your code and avoid ambiguity, always use explicit conversions using the CAST or CONVERT functions. This practice not only makes your intent clear but also helps prevent errors due to implicit type conversions.
SELECT CAST('2023-01-01' AS DATE); -- Explicit conversion to DATE
3. Handle NULL Values Gracefully: As discussed earlier, NULL values can complicate conversions. Always account for NULL values by using functions such as COALESCE or ISNULL. This approach allows you to provide default values during conversions, thereby avoiding NULL-related issues in your results.
SELECT COALESCE(CAST(NULL AS INT), 0) AS SafeValue; -- Defaults NULL to 0
4. Be Mindful of Data Truncation: When converting from larger to smaller data types, be aware of possible data loss through truncation. Always validate that the converted value meets your expectations. If precision is important, ponder rounding or adjusting the data before conversion to mitigate loss.
SELECT ROUND(123.456, 0) AS RoundedValue; -- Rounds to prevent truncation
5. Test for Incompatibilities: Before executing a conversion, especially in a production environment, run tests to ensure that the data being converted is compatible with the target type. This proactive approach can save you from runtime errors that could disrupt your applications.
SELECT CASE WHEN ISNUMERIC('ABC') = 1 THEN CAST('ABC' AS INT) ELSE 'Invalid' END AS Result; -- Checks compatibility before conversion
6. Think Performance Implications: Data type conversions can have performance implications, especially in large datasets. Use appropriate data types from the outset to minimize the need for conversion later on. For example, using INT for an integer value rather than VARCHAR can improve performance by reducing the overhead of conversion during data retrieval.
7. Document Your Conversions: Always document any conversions you perform in your SQL code. This practice not only aids in maintaining clarity for yourself but also assists other developers who may work with your code in the future. Being explicit about the reasons for conversions can save time and confusion down the line.
By adhering to these best practices, SQL developers can ensure that data type conversions are handled efficiently and effectively, leading to more robust and reliable database applications.