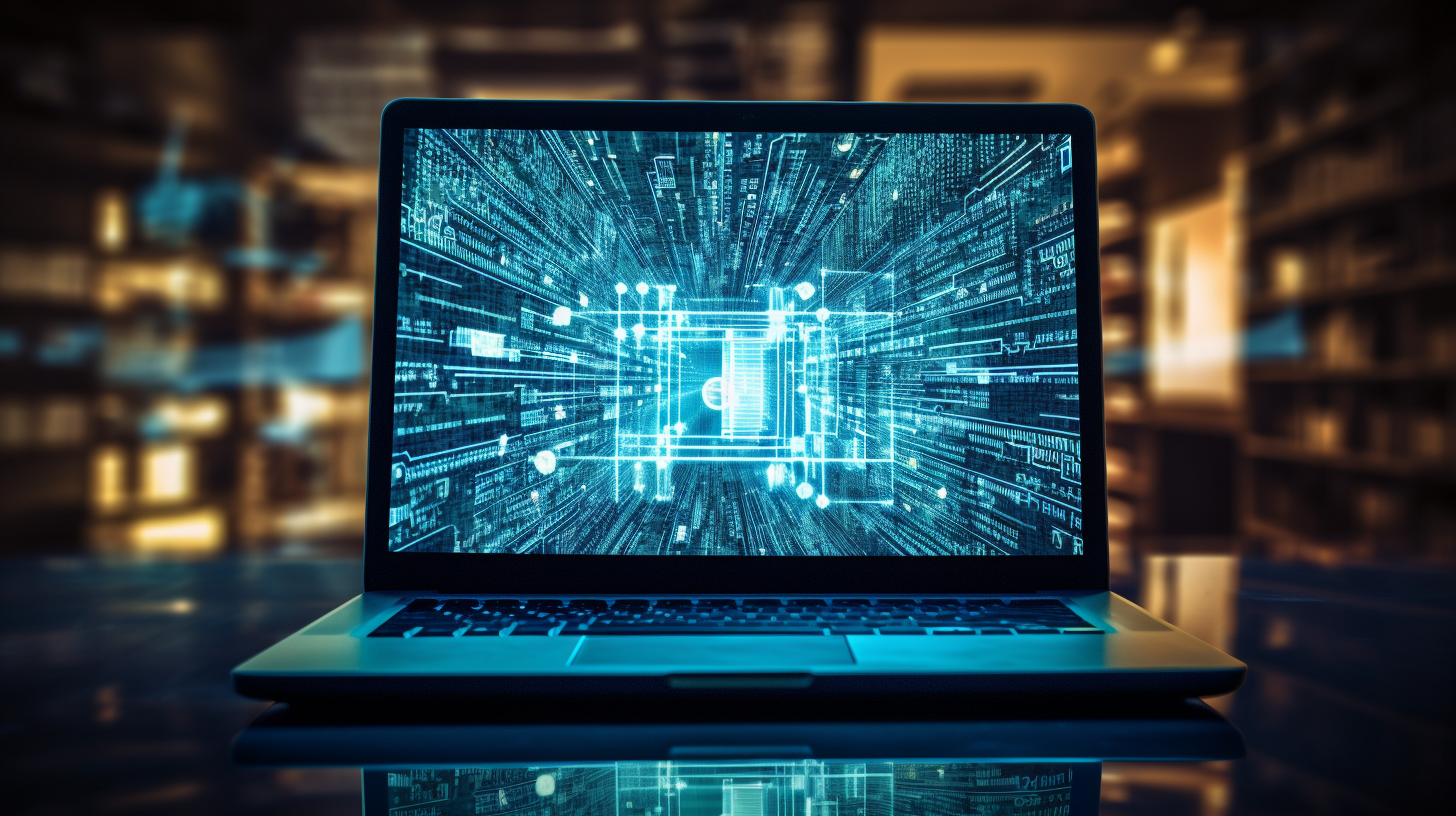
SQL and Subqueries for Data Analysis
Subqueries in SQL are a powerful feature that allows you to nest queries within other queries. This nested structure allows you to perform complex data retrieval and manipulation operations in a simpler manner. A subquery can return individual values, a list of values, or even an entire table that can be used in the main query. Typically classified as either single-row or multi-row, subqueries can significantly enhance your data analysis capabilities.
There are two primary types of subqueries: inline subqueries and correlated subqueries. Inline subqueries are executed only once, while correlated subqueries are executed for every row processed by the outer query. Understanding these distinctions especially important for writing efficient SQL code.
Here’s an example of an inline subquery:
SELECT employee_id, first_name, last_name FROM employees WHERE department_id = (SELECT department_id FROM departments WHERE department_name = 'Sales');
In this example, the inner query retrieves the department ID for ‘Sales’, which is then used in the outer query to fetch employee details.
On the other hand, a correlated subquery can be seen in the following example:
SELECT employee_id, first_name, last_name FROM employees e1 WHERE salary > (SELECT AVG(salary) FROM employees e2 WHERE e1.department_id = e2.department_id);
In this case, the inner query calculates the average salary for each department as the outer query processes each employee, comparing their salary to the department average.
Subqueries can be utilized in various clauses such as SELECT, FROM, and WHERE. Their flexibility allows analysts to write more readable and maintainable SQL code, encapsulating logic that would otherwise require more complicated joins or multiple queries.
However, while subqueries can simplify your queries, they can also lead to performance issues if not used judiciously. Therefore, understanding how to effectively use subqueries is essential for any SQL developer aiming to perform in-depth data analysis.
Types of Subqueries: Inline vs. Correlated
When diving deeper into the types of subqueries in SQL, it is essential to appreciate the implications of using inline versus correlated subqueries. Each type serves distinct purposes and can have varying performance impacts depending on how they are used.
Inline subqueries, as previously mentioned, execute only once and provide a static result set that the outer query can use. This makes them particularly advantageous when the inner query returns a consistent result that does not depend on the outer query’s current row context. They’re typically more efficient because the SQL engine optimizes the execution plan by evaluating the inline subquery just a single time.
For example, you might want to retrieve all products that are priced above the average price across all products. Here’s how an inline subquery would accomplish that:
SELECT product_id, product_name, price FROM products WHERE price > (SELECT AVG(price) FROM products);
In this case, the average price is calculated only once, and the outer query filters products based on that result.
In contrast, correlated subqueries depend on the outer query for their execution. They’re evaluated repeatedly, once for each row processed by the outer query. This characteristic can lead to performance bottlenecks, especially in scenarios involving large datasets, as the inner query is executed multiple times. Understanding this behavior is critical for optimizing query performance.
To illustrate a correlated subquery, consider the scenario where you want to find employees who earn more than the average salary within their respective departments:
SELECT employee_id, first_name, last_name FROM employees e1 WHERE salary > (SELECT AVG(salary) FROM employees e2 WHERE e1.department_id = e2.department_id);
Here, for each employee in the outer query, the inner query computes the average salary specific to their department. While this effectively filters employees based on their departmental context, it’s computationally expensive if the employee table is large, as the average salary calculation occurs multiple times.
To sum up, when deciding between inline and correlated subqueries, it especially important to analyze the context and requirements of your query. Inline subqueries can often lead to more efficient execution if the result does not change per row context. Conversely, correlated subqueries, while powerful in scenarios requiring row-by-row comparisons, should be used carefully to avoid performance degradation. Understanding the nuances between these types will help you write better SQL queries tailored to your data analysis needs.
Best Practices for Writing Efficient Subqueries
When using subqueries in SQL, efficiency is paramount. A well-optimized subquery can dramatically enhance query performance, while poorly constructed subqueries can lead to unnecessary overhead and slow responses. Here are several best practices to consider when writing subqueries.
1. Prefer Inline Subqueries When Possible
Inline subqueries are generally more efficient than correlated ones because they’re executed only once. Whenever you can use an inline subquery instead of a correlated subquery, you should do so. For example:
SELECT product_id, product_name FROM products WHERE price > (SELECT AVG(price) FROM products);
This inline subquery computes the average price once, allowing the outer query to filter products effectively without repeated calculations.
2. Use EXISTS Instead of IN for Subqueries
When checking for existence in a subquery, using EXISTS
can often be more efficient than IN
. The EXISTS
clause returns true as soon as it finds a matching row, making it faster for large datasets.
SELECT employee_id, first_name FROM employees e WHERE EXISTS (SELECT * FROM departments d WHERE e.department_id = d.department_id AND d.department_name = 'Sales');
Here, the query checks for the existence of any matching department without needing to retrieve all results as IN
would require.
3. Limit Subquery Results
Whenever possible, filter the results of your subquery to include only the data needed for your outer query. This reduces the workload on the SQL engine and speeds up execution. For instance:
SELECT employee_id, first_name FROM employees WHERE department_id IN (SELECT department_id FROM departments WHERE location_id = 100);
This subquery limits its results to department IDs in a specific location, which optimizes the outer query’s performance.
4. Avoid Nested Subqueries
Deeply nested subqueries can lead to decreased readability and performance issues. If you find yourself creating multiple layers of subqueries, think whether a join or a Common Table Expression (CTE) would provide a clearer and more efficient solution. For example:
WITH AvgSalaries AS ( SELECT department_id, AVG(salary) AS avg_salary FROM employees GROUP BY department_id ) SELECT employee_id, first_name FROM employees e JOIN AvgSalaries a ON e.department_id = a.department_id WHERE e.salary > a.avg_salary;
Using a CTE not only improves readability but also enhances performance by calculating averages just once.
5. Analyze Execution Plans
Always analyze the execution plan of your queries to understand how SQL Server processes them. Look for performance bottlenecks and adjust your subqueries accordingly. Utilize tools like EXPLAIN
to gain insights into how your SQL queries are executed and to identify potential optimizations.
By following these best practices, you can harness the full potential of subqueries while minimizing the impact on database performance. The judicious use of subqueries will not only streamline your SQL code but also empower you to conduct more sophisticated data analysis with greater efficiency.
Common Use Cases for Subqueries in Data Analysis
Subqueries are an invaluable tool for SQL data analysis, enabling you to encapsulate complex logic within a single query statement. Their versatility enables a range of practical applications that can simplify data retrieval and enhance analytical capabilities. Understanding these common use cases helps in using subqueries for optimal results.
One of the primary use cases for subqueries is to filter results based on aggregates computed in the inner query. For instance, if you need to find all employees whose salaries exceed the average salary within their respective departments, a subquery can efficiently achieve this:
SELECT employee_id, first_name, last_name FROM employees e1 WHERE salary > (SELECT AVG(salary) FROM employees e2 WHERE e1.department_id = e2.department_id);
This query dynamically calculates the average salary for each department, which will allow you to isolate employees who earn more than this average without requiring a separate aggregation query.
Another common application of subqueries is to check for existence or non-existence of records in related tables. For example, if you wish to retrieve products that belong to categories currently active in the database, you can utilize a subquery with the EXISTS clause:
SELECT product_id, product_name FROM products p WHERE EXISTS (SELECT 1 FROM categories c WHERE p.category_id = c.category_id AND c.is_active = 1);
In this case, the subquery checks for active categories related to each product, ensuring only those products are returned that meet the specified condition.
Subqueries also shine when performing calculations based on another table’s results. For example, if you need to find customers whose orders exceed the average order total, you can achieve this with a subquery as follows:
SELECT customer_id, customer_name FROM customers WHERE (SELECT SUM(order_total) FROM orders WHERE customer_id = customers.customer_id) > (SELECT AVG(order_total) FROM orders);
This query compares each customer’s total orders to the overall average, thereby isolating those whose spending habits are notably above average.
Subqueries can also be utilized in the FROM clause, which will allow you to create temporary tables for further analysis. For example, if you need a summary of total sales by product category, you can use a subquery to aggregate the data first:
SELECT category_id, SUM(total_sales) AS total_sales FROM (SELECT p.category_id, o.order_total AS total_sales FROM products p JOIN orders o ON p.product_id = o.product_id) AS sales_summary GROUP BY category_id;
In this scenario, the inner subquery constructs a detailed view of sales that can then be grouped by category, providing a clean and effective means to analyze product performance across categories.
Lastly, subqueries can aid in comparative analysis, such as finding employees who earn more than the average salary. A well-structured subquery can simplify the complexity of such comparisons:
SELECT employee_id, first_name FROM employees WHERE salary > (SELECT AVG(salary) FROM employees);
This example directly compares each employee’s salary to the overall average, streamlining the query and enhancing clarity.
Subqueries are not merely a syntactic convenience but a strategic asset in data analysis, allowing for effective filtering, existence checks, aggregations, and comparative operations. Mastering their use opens up a world of possibilities for insightful data exploration and refined analytical capabilities.
Troubleshooting Subqueries: Common Pitfalls and Solutions
When working with subqueries in SQL, developers often encounter challenges that can lead to unexpected results or performance issues. Understanding these common pitfalls and knowing how to troubleshoot them very important for writing efficient SQL code. Here, we will explore several frequent subquery-related problems and provide solutions to help you navigate through them effectively.
One of the primary issues with subqueries arises from their placement within the SQL statement. For example, if a subquery is used in a WHERE clause without proper context, it may return unexpected results. Consider the following situation:
SELECT first_name, last_name FROM employees WHERE department_id IN (SELECT department_id FROM departments WHERE location_id = 100 AND department_name = 'Sales');
This query may yield no results if there are no departments named ‘Sales’ in location 100. To troubleshoot, ensure that your inner query is correctly returning the desired values and that the conditions align with your expectations. Testing the inner query independently can help confirm its logic before integrating it into the main query.
Another common pitfall is the misuse of NULL values. Subqueries often return NULL when no matching result is found, which can cause issues in comparison operations. For instance:
SELECT employee_id, first_name FROM employees WHERE salary > (SELECT AVG(salary) FROM employees WHERE department_id = NULL);
The comparison to NULL will not yield any results because NULL is not equal to any value, including itself. To resolve this, ensure that your subquery’s conditions prevent NULL results, or handle nulls appropriately using IS NULL checks or COALESCE functions:
SELECT employee_id, first_name FROM employees WHERE salary > COALESCE((SELECT AVG(salary) FROM employees WHERE department_id IS NOT NULL), 0);
Performance issues can also arise with correlated subqueries, especially when they’re executed repeatedly for each row processed by the outer query. This can lead to significant slowdowns. A classic example of this is:
SELECT employee_id, first_name FROM employees e1 WHERE salary > (SELECT AVG(salary) FROM employees e2 WHERE e1.department_id = e2.department_id);
To optimize this, think using a JOIN instead of a correlated subquery. A JOIN allows for a single pass through the data, which can improve performance significantly:
SELECT e1.employee_id, e1.first_name FROM employees e1 JOIN (SELECT department_id, AVG(salary) AS avg_salary FROM employees GROUP BY department_id) e2 ON e1.department_id = e2.department_id WHERE e1.salary > e2.avg_salary;
Another common issue is failing to account for the data types that subqueries may return. For example, attempting to compare a VARCHAR to an INT can lead to errors or unexpected behavior. Always ensure that the data types align correctly between the main query and the subquery. You can use explicit type conversions if necessary:
SELECT first_name FROM employees WHERE department_id = (SELECT CAST(department_id AS INT) FROM departments WHERE department_name = 'Sales');
Finally, be cautious of subqueries that could potentially return more than one row when the outer query expects a single value. This can lead to errors that interrupt query execution. For instance:
SELECT first_name FROM employees WHERE department_id = (SELECT department_id FROM departments);
This will throw an error if the subquery returns more than one department ID. To prevent this, ponder using the LIMIT clause if appropriate, or redesign the query to ensure a single result is returned:
SELECT first_name FROM employees WHERE department_id IN (SELECT department_id FROM departments);
By understanding these common pitfalls and employing the suggested solutions, you can enhance your ability to write robust and efficient SQL queries. Remember that testing subqueries independently, checking for NULL values, optimizing performance, ensuring data type consistency, and managing expected return sizes are all critical practices to develop as you work with subqueries in SQL.