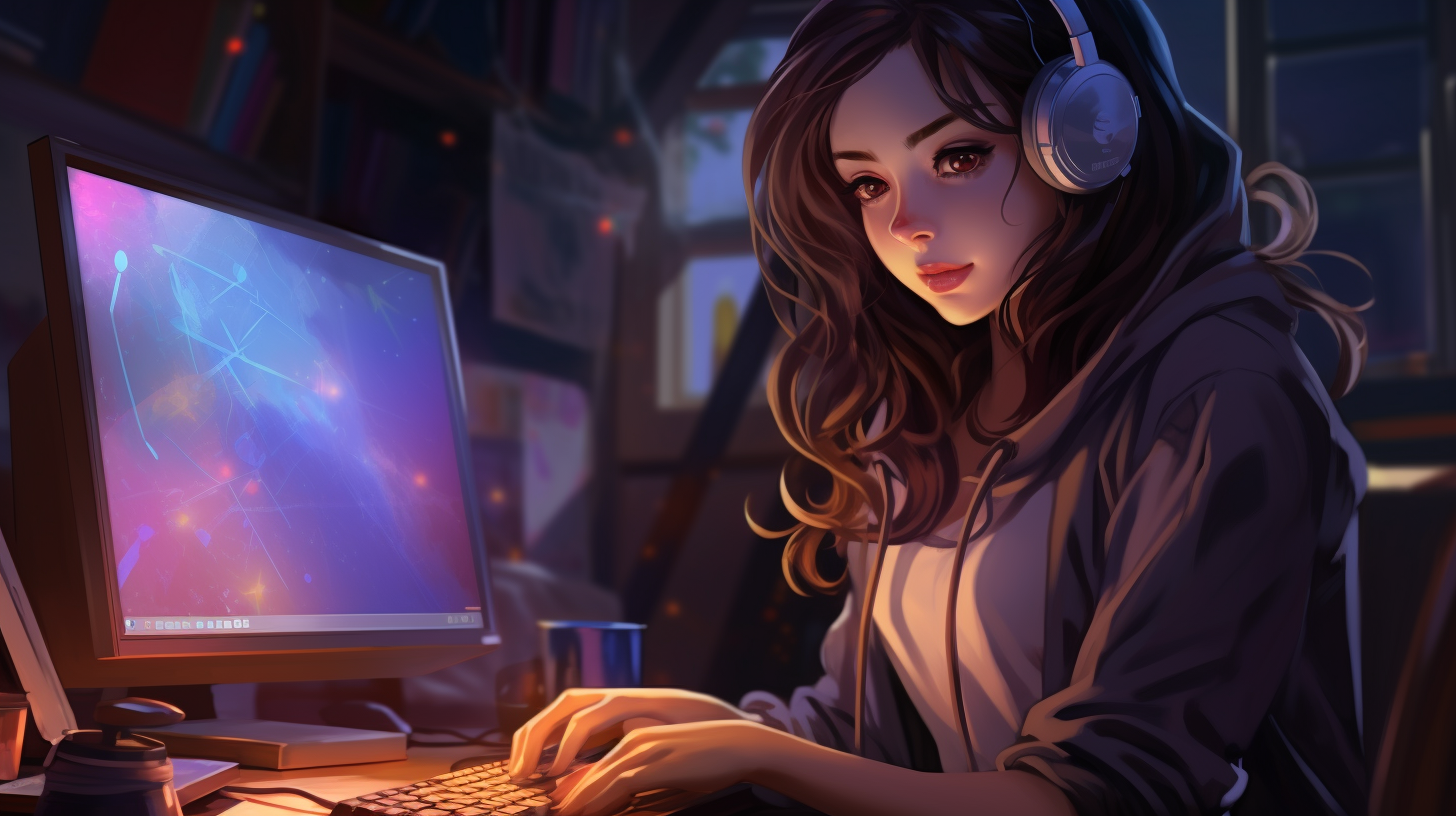
Advanced Bash Prompt Customization
In Bash, the prompt you see in your terminal is influenced primarily by a set of variables that define its appearance and behavior. The most significant of these is the PS1 variable, which dictates what your primary prompt looks like. Understanding how these variables work will empower you to create a prompt tailored to your specific needs.
There are several prompt variables in Bash, each serving a distinct purpose:
- This is the primary prompt variable, shown before each command input. It can include placeholders for dynamic content, such as the username, hostname, and current working directory.
- This variable defines the secondary prompt, which appears when a command is continued on the next line. By default, it is set to >.
- Used in the
select
command, this variable sets the prompt for the user to make a selection from a list. - This prompt appears before each command in a trace when the
set -x
option is enabled, assisting in debugging scripts.
Each of these variables can be customized individually, but PS1 is where the most significant changes typically occur. By manipulating this variable, you can create rich, informative prompts that enhance your command-line experience.
To illustrate, here’s a basic example of how you might define a simple PS1 variable:
PS1="u@h:w$ "
In this example:
u
represents the username of the current user.h
represents the hostname of the machine.w
represents the current working directory.$
is a character that changes depending on whether the user is root or a normal user (it shows # for root and $ for others).
This prompt will display something like user@hostname:~/current/directory$, providing a clear context for your command input. Understanding these variables allows for a much deeper and more personalized shell experience, paving the way for further customization.
Customizing the PS1 Variable
Customizing the PS1 variable is where the true power of Bash prompt manipulation lies. By tweaking this variable, you can convey a wealth of information at a glance, making your terminal sessions more efficient and visually appealing. The customization possibilities are virtually limitless, as you can incorporate various escape sequences that represent different aspects of your environment.
To modify the PS1 variable, you can use the export command in your shell configuration file (like .bashrc or .bash_profile). This allows changes to persist across terminal sessions. Here’s an example of a slightly more complex PS1 configuration:
export PS1="[e[32m]u@h [e[34m]w [e[31m]$ [e[0m]"
In this configuration:
- [e[32m] sets the text color to green for the username and hostname.
- [e[34m] sets the text color to blue for the current working directory.
- [e[31m] sets the text color to red for the command prompt character.
- [e[0m] resets the text formatting to the terminal default after the prompt.
This setup results in a colorful prompt that not only looks attractive but also helps differentiate the various components at a glance. You can experiment with different color codes to find a combination that suits your taste.
Another powerful feature of the PS1 variable is its ability to include dynamic content. For instance, you might want to include the current time or the exit status of the last command. You can do this by using the appropriate escape sequences:
export PS1="[e[32m]u@h [e[34m]w [e[33m]$(date +'%H:%M:%S') [e[31m]$?[e[0m] $ "
In this example:
- %M:%S’) dynamically inserts the current time into the prompt.
- $? displays the exit status of the last command executed, helping quickly identify if the previous command ran successfully (0) or failed (non-zero).
With these techniques, the PS1 variable not only enhances the aesthetics of your command line but also increases its functionality. You can style it to your liking while ensuring that critical information remains readily accessible, all of which ultimately leads to a more productive command-line environment.
Adding Colors and Special Characters
Adding colors and special characters to your Bash prompt can significantly enhance its aesthetics and usability. By using ANSI escape codes, you can create a visually distinct prompt that stands out against the terminal background, making it easier to read and navigate. This section will explore how to incorporate various colors and special characters into your prompt for a more customized experience.
In Bash, colors are controlled using escape sequences that start with the escape character followed by specific codes. The general format is:
"[e[m]"
Here, `` represents the color code or formatting style you wish to apply. For instance:
- 30 - Black
- 31 - Red
- 32 - Green
- 33 - Yellow
- 34 - Blue
- 35 - Magenta
- 36 - Cyan
- 37 - White
Additionally, you can use codes like:
- 1 - Bold
- 4 - Underline
- 0 - Reset/Normal
To illustrate, consider the following example that uses a combination of colors and special characters:
export PS1="[e[1;32m]➜ [e[34m]u@h [e[33m]:w[e[0m] $ "
In this prompt configuration:
- ➜ is a special character that visually indicates the prompt's position, making it more engaging.
- [e[1;32m] sets the color to bold green for the special character.
- [e[34m] sets the color to blue for the username and hostname.
- [e[33m] uses yellow for the current working directory.
- [e[0m] resets the formatting back to the terminal's default color after the prompt.
As you customize your prompt, consider the implications of color choices and symbols. A harmonious color palette can make your terminal more appealing and less straining on the eyes during long coding sessions. Additionally, using distinct symbols can help delineate different elements of the prompt, providing clarity and improving overall usability.
To take it a step further, you can add more complexity to your prompt by incorporating additional dynamic elements, such as the time, weather, or even Git branch status for developers working with version control. Here’s an advanced example:
export PS1="[e[1;32m]➜ [e[34m]u@h [e[33m]:w [e[36m]$(git branch 2>/dev/null | grep '*' | sed 's/* //')[e[0m] $ "
In this example:
- The prompt shows the current Git branch (if available) in cyan.
- 2>/dev/null suppresses any error messages if not inside a Git repository.
- The sed command extracts only the branch name to keep the prompt clean and concise.
This level of customization not only enhances the look of your terminal but also provides essential information at a glance, ultimately leading to a more productive and enjoyable command-line experience. By experimenting with different colors, special characters, and dynamic content, you can craft a prompt that's uniquely your own.
Dynamic Content in the Bash Prompt
Dynamic content in your Bash prompt is one of the most exciting features to leverage, transforming a static command line into a responsive and informative workspace. By inserting real-time information into your prompt, you can create a more interactive and context-aware environment. This capability is particularly useful for monitoring the state of your system or keeping track of the progress of your work.
To illustrate how to incorporate dynamic content, consider using commands that output real-time data. For example, you might display the current time or system load, or even the result of the last executed command. Each of these can enhance your prompt by providing critical context directly where you need it. Here’s how to include some of these elements.
One common approach is to use command substitution with the dollar sign and parentheses syntax, as in $(command). This allows you to run a command and insert its output into your PS1 prompt. Here's a simpler example that shows the current time:
export PS1="[e[32m]u@h:w [e[36m]$(date +'%H:%M:%S')[e[0m] $ "
In this example, $(date +'%H:%M:%S') retrieves the current time formatted as hours, minutes, and seconds and displays it in cyan (thanks to [e[36m]). This small addition can significantly improve your awareness of the time while working in the terminal.
Another useful dynamic addition is the exit status of the last command executed. This can be done using the special variable $?. By including this in your prompt, you get immediate feedback on the success or failure of the previous command. Think the following example:
export PS1="[e[32m]u@h:w [e[31m] Exit: $?[e[0m] $ "
Here, the exit status is displayed in red, so that you can quickly ascertain if the last command succeeded (0) or failed (any non-zero value).
For those working in collaborative environments or projects involving version control, displaying the current Git branch can be incredibly useful. You can achieve this by incorporating Git commands into your prompt. The following example demonstrates how to include the current Git branch, if applicable:
export PS1="[e[32m]u@h:w [e[36m]$(git branch 2>/dev/null | grep '*' | sed 's/* //')[e[0m] $ "
In this command, $(git branch 2>/dev/null | grep '*' | sed 's/* //') checks for the current Git branch. The 2>/dev/null part suppresses any error messages when you're not in a Git repository. This allows your prompt to adjust dynamically based on your current context, giving you instant access to vital information without cluttering your workspace.
The key takeaway when adding dynamic content to your Bash prompt is to ensure that it serves a purpose without overwhelming you with information. A well-thought-out prompt can lead to a more efficient workflow, providing both aesthetic allure and functional utility. Experimenting with various commands and their output can uncover a wealth of possibilities for enhancing your command line experience.