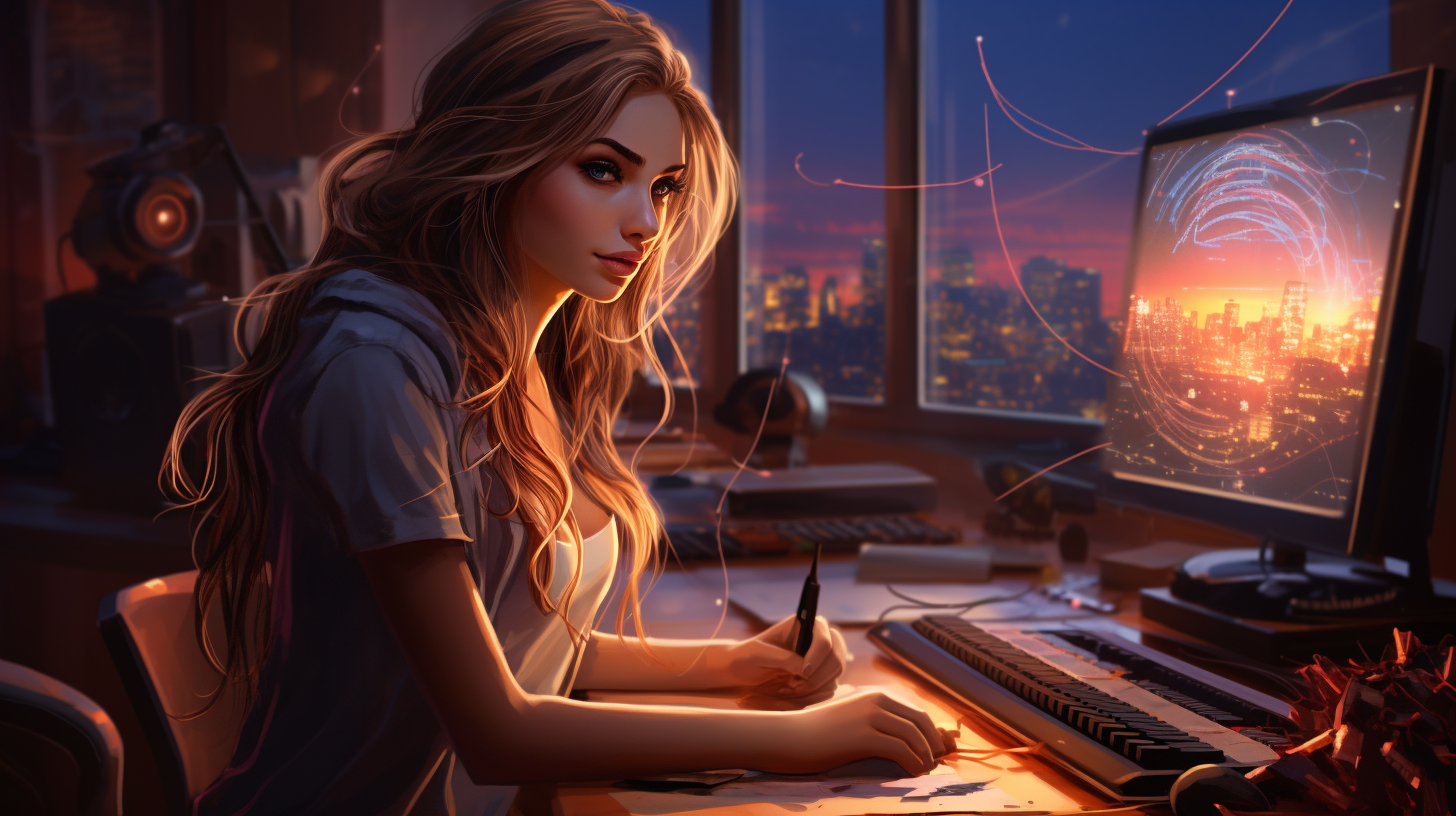
Advanced Bash Script Logging
Logging is an essential aspect of any robust Bash script, serving as a vital tool for monitoring execution, diagnosing issues, and understanding the behavior of your scripts. Various techniques can be employed for effective logging in Bash, each with its own advantages and use cases. A well-structured logging mechanism can greatly enhance the maintainability and reliability of your scripts.
One of the fundamental approaches to logging in Bash is to use the built-in echo
command to write messages to standard output or a designated log file. For instance:
echo "This is a log message" >> /path/to/logfile.log
However, this method lacks sophistication and can quickly become unmanageable in larger scripts. To ameliorate this, you can define a logging function that encapsulates the logging behavior, allowing for flexibility and standardization.
log_message() { local message="$1" local log_file="/path/to/logfile.log" echo "$(date '+%Y-%m-%d %H:%M:%S') - ${message}" >> "${log_file}" }
With this function, you can easily log messages with timestamps, which is invaluable for tracing script execution over time. Simply call the function with your message:
log_message "Script started."
Another technique involves using different log levels, which allows you to categorize messages based on their severity. For example, you may want to distinguish between info, warn, and error messages.
log() { local level="$1" local message="$2" local log_file="/path/to/logfile.log" echo "$(date '+%Y-%m-%d %H:%M:%S') [${level}] - ${message}" >> "${log_file}" }
You can then log messages by specifying the level:
log "INFO" "This is an informational message." log "WARN" "This is a warning message." log "ERROR" "This is an error message."
This structured approach not only streamlines your logging but also aids in filtering log entries based on severity when reviewing logs later.
For more advanced usage, ponder using a logging library or external tools that provide additional capabilities, such as rolling logs, logging to remote servers, or integrating with system logging daemons. However, for many Bash scripts, the methods outlined here will suffice to establish a robust logging framework.
Moreover, for scripts that are expected to run in various environments or for extended periods, adopting a logging technique that respects user privacy and adheres to system policies is important. Therefore, ensure that sensitive information is either not logged or is obfuscated appropriately.
Implementing Log Rotation
Implementing log rotation is a critical practice in maintaining the longevity and performance of your log files. As scripts execute, they can generate a vast amount of log data, which, if left unchecked, can consume significant disk space and potentially lead to system slowdowns or failures. Log rotation allows you to manage these log files effectively by archiving old logs and creating new ones, ensuring that your logging mechanism remains efficient and manageable.
One common approach to implement log rotation in Bash is to use the logrotate
utility, which is specifically designed for this purpose. It provides a flexible way to handle log files, allowing you to define various parameters such as frequency of rotation, number of retained backups, and compression options. To use logrotate
, you need to create a configuration file that specifies how your log files should be rotated.
# Create a logrotate configuration file for your script cat > /etc/logrotate.d/myscript << 'EOF' /path/to/logfile.log { daily # Rotate daily rotate 7 # Keep 7 days of backlogs compress # Compress old logs missingok # Don't throw an error if the log file is missing notifempty # Don't rotate the log if it is empty create 0640 user group # Create new log file with specified permissions } EOF
In this configuration, logrotate
will check your log file every day, rotating it and keeping the previous seven versions. The compress
option ensures that old logs are compressed to save disk space. Additionally, specifying missingok
and notifempty
provides safeguards against errors if the log file is unavailable or empty.
To ensure that your logs are being rotated as per the defined schedule, you can test the configuration with the following command:
logrotate -d /etc/logrotate.d/myscript
The -d
flag enables debug mode, so that you can see the actions that logrotate
would take without making any changes. This way, you can verify that your configurations are correctly set up. Once satisfied, you can run it without the debug flag to execute the rotation based on the schedule.
Another method of managing log rotation directly within your Bash script is to incorporate a simple rotation mechanism. For example, you can check the size of the log file and rename it if it exceeds a certain threshold. Here’s an example implementation:
# Simple log rotation in Bash log_file="/path/to/logfile.log" max_size=1048576 # 1MB if [[ -f "${log_file}" ]] && [[ $(stat -c%s "${log_file}") -ge ${max_size} ]]; then mv "${log_file}" "${log_file}.$(date '+%Y%m%d%H%M%S')" touch "${log_file}" fi
In this script snippet, we check if the log file exists and if its size exceeds 1MB. If both conditions are met, we rename the log file by appending a timestamp, which provides a clear record of when the log was rotated. We then create a new empty log file to continue logging.
By implementing log rotation techniques, you can ensure that your logging system remains efficient and manageable, preventing potential issues caused by excessive log file size. This practice is especially important for long-running scripts or those operating in production environments, where resource management is paramount.
Custom Log Formats and Levels
Custom log formats and levels can significantly enhance the usability and clarity of your logging system in Bash scripts. By defining specific formats for your log entries, you can ensure that they’re structured in a way that makes it easier to parse and analyze them later, whether manually or with automated tools. Tailoring log levels also provides the ability to filter messages according to their severity, allowing you to focus on critical issues while ignoring less important notices.
To start, you should define a standard format for your log entries. A common practice is to include the timestamp, log level, and the actual message. This structure not only helps in identifying when an event occurred but also categorizes it by its importance. Here’s an enhanced logging function that incorporates a custom format:
log() { local level="$1" local message="$2" local log_file="/path/to/logfile.log" # Format the log entry with timestamp, level, and message echo "$(date '+%Y-%m-%d %H:%M:%S') [${level}] - ${message}" >> "${log_file}" }
With the logging function defined, you can categorize your log entries by severity levels such as INFO, WARN, ERROR, and DEBUG. This categorization becomes particularly useful when you want to filter logs. For instance, if you only want to review warning and error messages, you can use tools like grep
to quickly sift through the log file:
grep '[WARN]|[ERROR]' /path/to/logfile.log
To further enhance your logs, think implementing additional log levels such as DEBUG and TRACE. This can be especially helpful during the development phase of your scripts, enabling you to include detailed information about the script’s execution flow:
log "DEBUG" "Starting the process with parameters: $1, $2" log "TRACE" "Entering function foo with argument: $1"
This distinction between log levels enables better control over what gets logged based on the script’s current state or a specific runtime flag. For example, you might want to allow for a verbosity flag that enables or disables DEBUG logs:
VERBOSITY=0 # 0 = normal, 1 = verbose if [[ "$VERBOSITY" -eq 1 ]]; then log "DEBUG" "More detailed logging enabled." fi
Another aspect of custom logging is the ability to format your logs in different styles. For instance, you might want JSON-formatted logs, which are easier to parse programmatically. Consider the following adaptation of the logging function to produce JSON output:
log_json() { local level="$1" local message="$2" local log_file="/path/to/logfile.log" local timestamp="$(date '+%Y-%m-%d %H:%M:%S')" # Format the log entry as JSON echo "{"timestamp": "${timestamp}", "level": "${level}", "message": "${message}"}" >> "${log_file}" }
This JSON format not only maintains the essential components of your log entry but also enhances compatibility with log management systems that can ingest JSON data. Consequently, whether you are working in a debugging context or monitoring production systems, adopting custom log formats and levels can lead to more effective tracking and analysis of events within your Bash scripts.
Error Handling and Debugging with Logs
Error handling and debugging in Bash scripts are critical components that ensure your scripts can gracefully handle unexpected situations while providing useful feedback for troubleshooting. By integrating logging into your error handling strategy, you can capture essential information that aids in diagnosing issues and understanding the context of failures.
One effective way to manage errors in Bash is through the use of exit statuses. Every command in Bash returns an exit status, which can be checked to determine whether it succeeded or failed. The exit status of the last command executed is stored in the special variable $?
. By evaluating this value, you can make informed decisions on how to proceed. Here’s a simple example:
command_that_might_fail if [[ $? -ne 0 ]]; then log "ERROR" "Command failed with exit status $?" fi
In this case, if command_that_might_fail
does not execute successfully, the error message will be logged, providing a clear indication of what went wrong.
For more complex error handling, consider using a trap to catch errors globally. You can set a trap on the ERR
signal to execute a specific function whenever a command fails. This can simplify the process of logging errors across your entire script:
trap 'log "ERROR" "An error occurred in line $LINENO"' ERR
With this trap in place, any command that fails will trigger the logging of an error message that includes the line number where the failure occurred. That’s particularly useful for identifying issues in longer scripts.
When it comes to debugging, logging can provide a wealth of information about the state of your script at various points in its execution. You can enhance your logging functions to include variable states, which will help you trace the flow of data and identify where things might be going awry. For example:
debug() { local message="$1" local variables=$(declare -p) # Capture all current variables log "DEBUG" "${message}. Current variables: ${variables}" }
This debug
function can be called at strategic points in your script to log the current state of all variables, providing context that can be crucial for debugging complex logic. For instance:
my_variable="some value" debug "Before processing my_variable"
Additionally, ponder implementing a verbosity flag that allows you to control the level of detail in your logs. This way, you can toggle between minimal logging and verbose logging as needed:
VERBOSITY=1 # Set to 1 for verbose, 0 for normal if [[ "$VERBOSITY" -eq 1 ]]; then debug "Verbose logging is enabled." fi
By systematically incorporating logging into your error handling and debugging processes, you can create scripts that not only manage errors effectively but also provide valuable insights during development and maintenance. This approach allows you to pinpoint issues swiftly, making your scripts more robust and easier to troubleshoot.