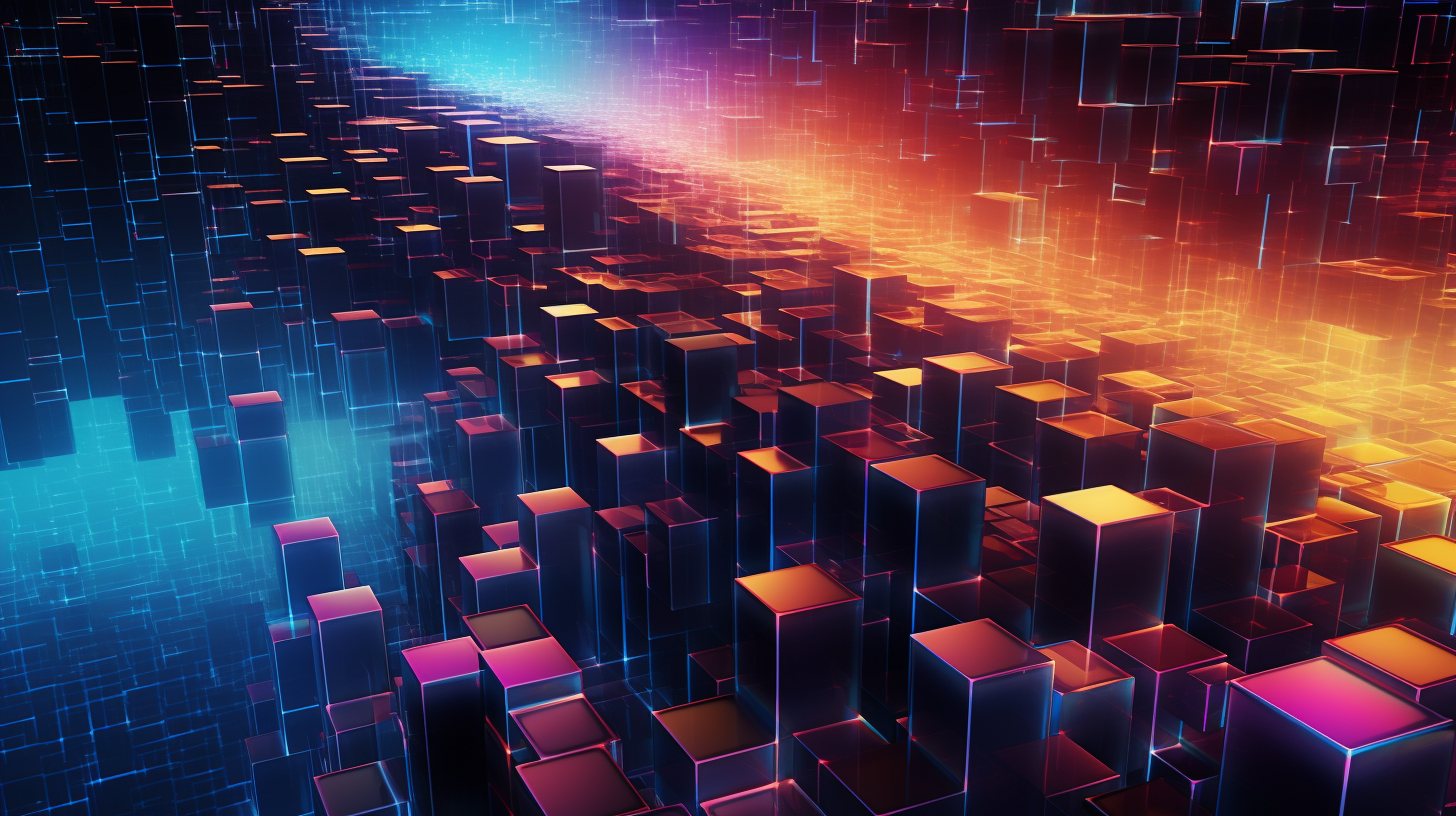
Advanced JavaScript Animation Techniques
The JavaScript animation pipeline is a fascinating mechanism, operating in the background to turn your code into fluid visuals. At its core, the process involves several key stages: preparation, rendering, and updating. Understanding these stages especially important for creating efficient animations that not only look good but also perform well.
When an animation is initiated, it often begins with the preparation phase, where the necessary data is collected and any state information is established. This phase may include calculating positions, determining styles, and making decisions about how elements will behave throughout the animation. Here, it’s essential to minimize the workload; gathering information in advance can significantly reduce overhead during the rendering phase.
Next comes the rendering phase. That is where the magic happens. The browser takes the prepared data and paints it onto the screen. It does so by using a deep understanding of the Document Object Model (DOM) and how to manipulate it effectively. At this stage, you must be conscious of reflows and repaints, as unnecessary updates can lead to performance hitches and visual stutters.
Finally, we have the updating phase. This is where the animation’s progression is managed. Typically, you’ll find yourself using a loop to repeatedly update the animation state—this is where techniques like requestAnimationFrame
come into play. By using this API, you can ensure your animations are synchronized with the display refresh rate, leading to a smoother experience.
To illustrate this pipeline, think the following example of a simple animation using requestAnimationFrame
:
let start = null; const element = document.getElementById('animateMe'); function animate(timestamp) { if (!start) start = timestamp; const progress = timestamp - start; // Change the position of the element element.style.transform = 'translateX(' + Math.min(progress / 10, 200) + 'px)'; if (progress < 2000) { requestAnimationFrame(animate); } } requestAnimationFrame(animate);
In this example, we set up a basic animation that translates an element horizontally. The timestamp
provided by requestAnimationFrame
helps track the elapsed time since the animation began, allowing for smooth transitions based on the frame rate.
Using CSS Transitions and Animations
When it comes to enhancing animations in your web applications, using CSS transitions and animations can offer an incredible advantage. The integration of CSS with JavaScript allows developers to harness hardware acceleration and optimize rendering performance seamlessly. CSS transitions allow you to change property values smoothly over a specified duration, while CSS animations provide more complex sequences that can be triggered via JavaScript or events.
CSS transitions are defined using the transition property in your stylesheet. By specifying which properties should transition, along with duration and timing functions, you can create engaging animations with minimal effort. For example, to animate the background color of an element on hover, you could use the following CSS:
.box { width: 100px; height: 100px; background-color: blue; transition: background-color 0.5s ease; } .box:hover { background-color: red; }
With this simple implementation, when a user hovers over the box, it smoothly transitions from blue to red over half a second. This not only enhances the user experience but also offloads animation tasks to the browser’s optimized rendering engine, leading to better performance.
CSS animations, on the other hand, allow for more complex sequences of animations defined with the @keyframes rule. This gives you the capacity to create intricate animations that can loop or run in specific directions. Here’s an example of how you might animate a bouncing ball:
@keyframes bounce { 0%, 20%, 50%, 80%, 100% { transform: translateY(0); } 40% { transform: translateY(-30px); } 60% { transform: translateY(-15px); } } .ball { width: 50px; height: 50px; background-color: yellow; border-radius: 50%; animation: bounce 2s infinite; }
This CSS animation causes the ball to bounce up and down continuously. The @keyframes rule specifies the animation sequence, which is then applied to the element using the animation property. Such animations are not only visually appealing but also maintain performance efficiency as browsers are optimized for handling CSS animations.
However, combining CSS transitions and animations with JavaScript can further extend the capabilities of your animations. For instance, you might want to trigger a CSS animation based on user interaction. Below is an example of how to toggle a CSS class with JavaScript to initiate the animation:
const ball = document.querySelector('.ball'); ball.addEventListener('click', () => { ball.classList.toggle('bounce'); });
In this example, when the ball is clicked, it toggles the bounce animation on and off. This simple integration between JavaScript and CSS can lead to highly interactive and engaging user experiences.
Advanced Techniques with RequestAnimationFrame
One of the key advantages of using requestAnimationFrame is its ability to create a seamless animation experience by synchronizing animations with the browser’s refresh rate. This means that animations will appear smooth and fluid, as they’re executed right before the browser repaints the screen. But there are additional advanced techniques you can implement to optimize animations further and unlock new creative possibilities.
For instance, while using requestAnimationFrame, you can introduce a more sophisticated approach to control the timing and pacing of animations through the use of easing functions. Easing functions dictate the acceleration and deceleration of an animation, allowing for more natural movements compared to linear transitions. By incorporating easing, you can make animations feel more organic, akin to real-world physics.
Here’s an example that demonstrates how to apply easing functions to an animation using requestAnimationFrame:
const element = document.getElementById('animateMe'); let start = null; function easeInOutQuad(t) { return t < 0.5 ? 2 * t * t : -1 + (4 - 2 * t) * t; } function animate(timestamp) { if (!start) start = timestamp; const progress = timestamp - start; const duration = 2000; // Duration in milliseconds const timeFraction = Math.min(progress / duration, 1); const easedProgress = easeInOutQuad(timeFraction); // Change the position of the element using the eased progress element.style.transform = 'translateX(' + (easedProgress * 200) + 'px)'; if (timeFraction < 1) { requestAnimationFrame(animate); } } requestAnimationFrame(animate);
In this example, we use the easeInOutQuad function to create a smooth transition effect. The function adjusts the timing of the animation so that it starts slow, accelerates, and then decelerates before reaching the endpoint. This provides a visually engaging experience, making it feel more lifelike.
Another advanced technique involves layering multiple animations. By managing several animations at the same time, you can create complex interactions that feel cohesive. For instance, you might animate an element’s position while at the same time changing its scale or opacity. Here’s how you could achieve this:
const element = document.getElementById('animateMe'); let start = null; function animate(timestamp) { if (!start) start = timestamp; const progress = timestamp - start; const duration = 2000; const timeFraction = Math.min(progress / duration, 1); const easedProgress = easeInOutQuad(timeFraction); // Change the position, scale, and opacity of the element element.style.transform = 'translateX(' + (easedProgress * 200) + 'px) scale(' + (1 + easedProgress) + ')'; element.style.opacity = 1 - easedProgress; if (timeFraction < 1) { requestAnimationFrame(animate); } } requestAnimationFrame(animate);
In this case, the element not only moves to the right but also scales up while fading out, delivering a richer visual experience. The combination of transformations adds depth and enhances user engagement.
Creating Smooth Animations with Easing Functions
Creating smooth animations in JavaScript often hinges on the use of easing functions. Easing functions determine how an animation progresses over time, allowing for movements that feel more natural and visually appealing compared to simple linear transitions. Rather than moving at a constant speed from start to finish, easing functions can create the illusion of weight and fluidity, mimicking the physical behavior of objects in the real world.
There are several common easing functions, each offering different timing characteristics. For instance, a linear easing function moves at a constant speed, while others like ease-in or ease-out start slowly and then speed up or slow down at the end, respectively. A more sophisticated easing function, such as ease-in-out, combines both effects, starting and ending the animation slowly while moving faster in the middle. This nuance can significantly enhance user experience.
To demonstrate how easing functions can be applied in an animation, let’s think an example where we create a simple animation of a ball moving across the screen with an ease-in-out effect:
const ball = document.getElementById('ball'); let start = null; function easeInOutQuad(t) { return t < 0.5 ? 2 * t * t : -1 + (4 - 2 * t) * t; } function animate(timestamp) { if (!start) start = timestamp; const progress = timestamp - start; const duration = 2000; // Duration in milliseconds const timeFraction = Math.min(progress / duration, 1); const easedProgress = easeInOutQuad(timeFraction); // Change the position of the ball ball.style.transform = 'translateX(' + (easedProgress * 500) + 'px)'; if (timeFraction < 1) { requestAnimationFrame(animate); } } requestAnimationFrame(animate);
In this code snippet, we define an easeInOutQuad
function that modifies the animation’s timing. As the animation progresses, the ball moves 500 pixels to the right, but the movement is affected by the easing function, making it feel more organic. The animation starts slowly, speeds up in the middle, and then slows down as it reaches its destination.
Beyond simple animations, easing functions can be applied to complex sequences where multiple properties are animated concurrently. For instance, you could animate an element’s position while also changing its opacity to create a fade-in effect:
const element = document.getElementById('animatedElement'); let start = null; function animate(timestamp) { if (!start) start = timestamp; const progress = timestamp - start; const duration = 2000; const timeFraction = Math.min(progress / duration, 1); const easedProgress = easeInOutQuad(timeFraction); // Change position and opacity of the element element.style.transform = 'translateY(' + (easedProgress * 300) + 'px)'; element.style.opacity = easedProgress; if (timeFraction < 1) { requestAnimationFrame(animate); } } requestAnimationFrame(animate);
This example demonstrates an element that moves vertically while at once fading in from transparency to full visibility. The use of the easing function ensures that both the translation and opacity transitions feel harmonious, further enhancing the overall animation quality.
Integrating Canvas for Complex Animation Projects
Integrating the HTML5 Canvas element into your animation projects opens up a wealth of opportunities for creating complex and visually stunning graphics that surpass the capabilities of standard DOM-based animations. Unlike traditional animations which manipulate HTML elements, the canvas gives you direct control over pixels, allowing for intricate visual effects that can respond dynamically to user input and other stimuli.
The canvas works through a 2D drawing context, which provides a set of methods for rendering shapes, images, and text. This flexibility makes it perfect for a range of applications—from simple animations to full-fledged games or data visualizations. The first step is to ensure you have a canvas set up in your HTML:
<canvas id="myCanvas" width="800" height="600"></canvas>
Once the canvas is in place, you can access its drawing context in JavaScript and start creating animations. Here’s a basic example of animating a bouncing ball:
const canvas = document.getElementById('myCanvas'); const ctx = canvas.getContext('2d'); let x = canvas.width / 2; let y = canvas.height / 2; let dx = 2; // Change in x (speed) let dy = 2; // Change in y (speed) const radius = 20; function drawBall() { ctx.clearRect(0, 0, canvas.width, canvas.height); // Clear the canvas ctx.beginPath(); ctx.arc(x, y, radius, 0, Math.PI * 2); ctx.fillStyle = 'blue'; ctx.fill(); ctx.closePath(); } function animate() { drawBall(); // Bounce off the walls if (x + radius > canvas.width || x - radius canvas.height || y - radius < 0) { dy = -dy; } x += dx; // Update x position y += dy; // Update y position requestAnimationFrame(animate); // Call animate again } animate();
In this example, we create a simple animation of a blue ball bouncing inside the canvas. The requestAnimationFrame method is used here to ensure smooth animations, synchronizing with the display refresh rate. The ball is drawn using the arc method, and we check for collisions with the canvas borders to reverse its direction as necessary.
As you venture deeper into canvas animations, consider using additional features such as image manipulation, transformation functions (like scaling and rotation), and event handling for interactivity. The following example illustrates how to create an interactive bouncing ball that changes color when clicked:
function changeColor() { ctx.fillStyle = 'red'; // Change color when clicked } canvas.addEventListener('click', changeColor); function animate() { drawBall(); // Similar boundary checks as before if (x + radius > canvas.width || x - radius canvas.height || y - radius < 0) { dy = -dy; } x += dx; y += dy; requestAnimationFrame(animate); } animate();
In this version, we add an event listener for mouse clicks on the canvas. When the ball is clicked, its color changes to red, demonstrating how simple interactivity can enhance your animations. The flexibility of the canvas in conjunction with JavaScript allows you to create not just simpler animations but also rich, engaging experiences that can captivate users.
Performance Optimization Strategies for JavaScript Animations
When it comes to optimizing JavaScript animations for performance, several strategies can be employed to ensure your animations run smoothly while maintaining visual fidelity. The goal is to achieve a balance between aesthetic quality and resource efficiency, allowing animations to be rendered seamlessly across different devices and browsers.
One of the most significant performance bottlenecks in animations arises from manipulating the DOM. Frequent layout changes, such as altering styles or adding/removing elements, can lead to reflows and repaints that are costly in terms of performance. To mitigate this, consider minimizing DOM interactions and batch your updates. Instead of changing styles one at a time, you can group changes together, like so:
const element = document.getElementById('myElement'); element.style.transition = 'none'; // Disable transitions temporarily element.style.transform = 'translateX(100px)'; // Update position element.style.opacity = '0'; // Update opacity element.offsetHeight; // Trigger reflow element.style.transition = ''; // Re-enable transitions
This technique allows you to apply multiple style changes efficiently before the browser calculates the layout. By triggering a reflow only when necessary, you can significantly enhance the performance of your animations.
Another important aspect of performance optimization is using hardware acceleration. When you use CSS properties like transform and opacity, the browser can offload the rendering to the GPU (Graphics Processing Unit), which is designed to handle complex visual effects more efficiently than the CPU. Here’s an example of how to take advantage of GPU acceleration:
const element = document.getElementById('myAnimatedElement'); let start = null; function animate(timestamp) { if (!start) start = timestamp; const progress = timestamp - start; // Utilize transform for GPU acceleration element.style.transform = 'translateY(' + (Math.sin(progress / 500) * 100) + 'px)'; requestAnimationFrame(animate); } requestAnimationFrame(animate);
In this code snippet, the transform property is utilized to create a smooth vertical oscillation for the element. By relying on this property, the browser can optimize rendering, resulting in a more fluid animation.
Additionally, be aware of the number of elements being animated simultaneously. Animating multiple elements can quickly consume resources and lead to dropped frames. Instead, consider using techniques such as sprite sheets or canvas to manage animations with a lower impact on performance. For example, when using the canvas for sprite animations, you can draw multiple frames in a single canvas update, effectively reducing the overhead associated with DOM manipulation:
const canvas = document.getElementById('spriteCanvas'); const ctx = canvas.getContext('2d'); const spriteSheet = new Image(); spriteSheet.src = 'sprites.png'; // Your sprite sheet source let frameIndex = 0; function draw() { ctx.clearRect(0, 0, canvas.width, canvas.height); ctx.drawImage(spriteSheet, frameIndex * frameWidth, 0, frameWidth, frameHeight, x, y, frameWidth, frameHeight); frameIndex = (frameIndex + 1) % totalFrames; // Loop through frames requestAnimationFrame(draw); } spriteSheet.onload = draw;
In this example, the drawImage method is used to render frames from a sprite sheet, significantly improving performance compared to animating individual DOM elements.
Finally, always keep an eye on the frame rate of your animations. Tools like the browser’s performance profiler can help you identify bottlenecks in your animation code. Strive for a target frame rate of 60 frames per second (fps) to ensure that animations appear fluid. If performance dips, analyze your code for potential optimizations, such as reducing the complexity of animations or simplifying calculations performed during rendering.