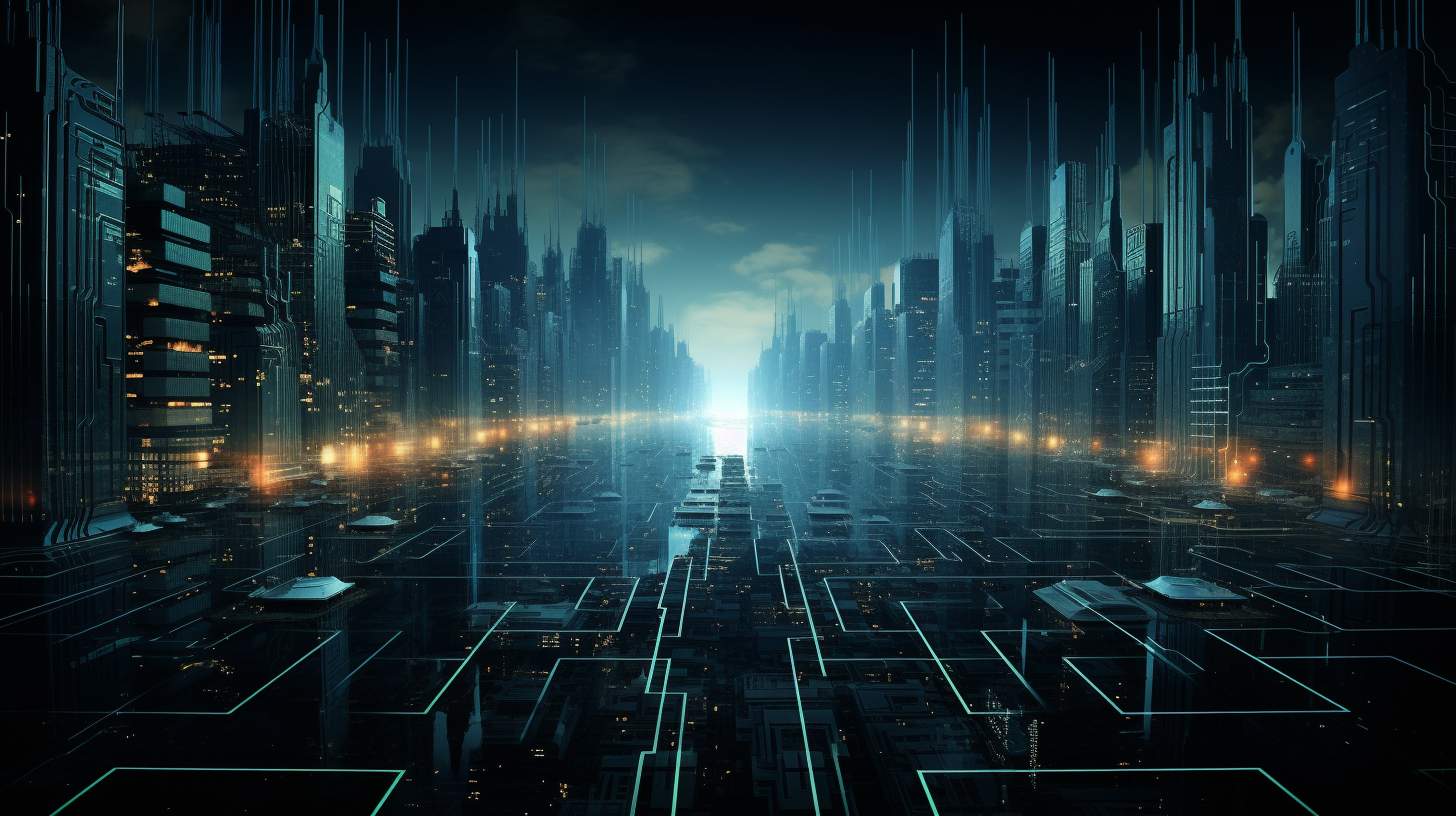
Advanced Swift: Protocol-Oriented Programming
In Swift, protocols play a pivotal role in defining a blueprint of methods, properties, and other requirements that suit a particular task or functionality. They serve as a powerful mechanism to create flexible and reusable code. At their core, protocols allow you to specify a set of functionalities that can be adopted by any class, structure, or enumeration, thus enabling a form of polymorphism.
To illustrate, consider the following protocol definition:
protocol Drawable { var color: String { get } func draw() }
This Drawable protocol defines a requirement for any conforming type to have a property called color, which is a String, and a method called draw(). Any class or struct that conforms to this protocol must implement these requirements, ensuring a consistent interface.
Here’s an example of a class and a struct that conform to the Drawable protocol:
class Circle: Drawable { var color: String init(color: String) { self.color = color } func draw() { print("Drawing a (color) circle.") } } struct Square: Drawable { var color: String func draw() { print("Drawing a (color) square.") } }
Both the Circle class and the Square struct provide specific implementations for the draw() method while adhering to the Drawable protocol’s contract. This illustrates how protocols allow different types to be treated uniformly based on the shared interface they implement.
Moreover, protocols can also inherit from one another, allowing for a hierarchical structure of requirements. For example:
protocol Shape: Drawable { var area: Double { get } }
In this example, the Shape protocol inherits from Drawable, meaning any conforming type must also implement the drawable requirements while adding a new requirement for an area property. This capability to compose protocols creates a rich interplay of behaviors and enforces a clear contract across different implementations.
Benefits of Protocol-Oriented Programming
Protocol-oriented programming (POP) in Swift introduces a paradigm shift that profoundly enhances the way we design and architect our applications. One of the standout benefits of this approach is its capacity to foster reusability and flexibility in code. By decoupling behavior from implementation, protocols allow developers to define clear and simple interfaces that can be adopted by various types, leading to a more modular codebase.
Another significant advantage is the encouragement of composition over inheritance. Traditional object-oriented programming often relies heavily on class hierarchies that can become unwieldy and hard to manage. In contrast, protocols enable you to compose behaviors in a way that promotes better separation of concerns. This means you can mix and match functionalities from different protocols without being constrained by a rigid inheritance chain.
Ponder this example where we have a protocol for logging:
protocol Loggable { func log(message: String) }
We can then create different types of loggers that conform to this protocol, each with its specialized behavior:
class ConsoleLogger: Loggable { func log(message: String) { print("Console log: (message)") } } class FileLogger: Loggable { func log(message: String) { // Imagine code that writes to a file print("File log: (message)") } }
This design allows us to easily switch between different logging mechanisms without altering the underlying code that utilizes these loggers. By depending on protocols rather than concrete implementations, we can change or extend functionality with minimal effort.
Moreover, protocol-oriented programming enhances type safety in Swift. Since protocols define exact requirements, any type conforming to a protocol guarantees that it will implement the specified methods and properties. This leads to fewer runtime errors, as many issues can be caught at compile time instead:
func performLogging(using logger: Loggable) { logger.log(message: "This is a log message.") }
Here, the function performLogging
only accepts parameters that conform to the Loggable
protocol, ensuring that any logger passed to it has the required log
method. This ensures a level of assurance that the code is operating on the expected interface, enhancing robustness significantly.
Additionally, protocol extensions further augment the benefits of protocol-oriented programming by allowing shared functionality to be defined in one place. This means you can add default implementations for methods in a protocol, thereby reducing duplication and promoting DRY (Don’t Repeat Yourself) principles:
extension Loggable { func log(message: String) { print("Default log: (message)") } }
With this extension in place, any type conforming to Loggable
that does not implement its own log
method will inherit this default behavior. This dramatically simplifies the implementation, especially for types that may not require a custom logging mechanism.
Defining and Implementing Protocols
Defining protocols in Swift is not only about stating what a type should do but also about forming a contract that details how it should behave. When you define a protocol, you are essentially establishing a set of rules that any conforming type must adhere to. This creates a layer of abstraction, allowing for various implementations without needing to know the specifics of how those implementations work.
To define a protocol, you use the `protocol` keyword followed by the name of the protocol. Inside the protocol, you declare properties and methods that conforming types must implement. For example, consider the following protocol that represents a vehicle:
protocol Vehicle { var numberOfWheels: Int { get } func startEngine() -> String }
In this example, the `Vehicle` protocol specifies that any conforming type must have a `numberOfWheels` property and a `startEngine` method. The property is read-only, as indicated by the absence of `set`, which means any conforming type cannot modify it directly. This encapsulation of behavior is a key feature of protocols.
Now, let’s implement this protocol with a couple of different types:
class Car: Vehicle { var numberOfWheels: Int { return 4 } func startEngine() -> String { return "Car engine started." } } class Motorcycle: Vehicle { var numberOfWheels: Int { return 2 } func startEngine() -> String { return "Motorcycle engine started." } }
The `Car` and `Motorcycle` classes both conform to the `Vehicle` protocol, each providing specific implementations for the required properties and methods. Notice how encapsulation promotes the differentiation of behavior while adhering to a common interface.
Furthermore, protocols can also define initializers. This allows you to enforce that specific initial setup code is implemented in the conforming types. Here’s how you can define a protocol with an initializer:
protocol Initializable { init(name: String) }
Any type conforming to `Initializable` must implement the `init(name:)` initializer. Here’s an example of how a struct could adopt this protocol:
struct User: Initializable { var name: String init(name: String) { self.name = name } }
The `User` struct now has a guaranteed initializer that accepts a name, thus solidifying the contract established by the `Initializable` protocol. This ensures that any code relying on `Initializable` can safely create instances of conforming types with the required properties.
Implementing protocols in this manner not only promotes modular design but also enhances readability and maintainability. By enforcing a structured approach to defining types, Swift’s protocol system empowers developers to create more flexible and reliable code. It allows for easily extensible systems where new types can be integrated seamlessly, provided they conform to the established protocols.
To further illustrate the power of defining and implementing protocols, ponder a scenario in which multiple types need to be processed through a common interface. By using protocols, you can create functions that act on these types without being aware of their specific implementations:
func activateVehicle(vehicle: Vehicle) { print("Activating vehicle with (vehicle.numberOfWheels) wheels.") print(vehicle.startEngine()) } let myCar = Car() let myMotorcycle = Motorcycle() activateVehicle(vehicle: myCar) activateVehicle(vehicle: myMotorcycle)
Protocol Extensions and Default Implementations
extension Drawable { func drawBorder() { print("Drawing a border in color: (color)") } }
By using protocol extensions, we can enrich the capabilities of a protocol without altering the types that conform to it. This ability to extend protocols allows developers to provide default implementations for methods or computed properties, which can be incredibly beneficial in reducing code duplication across multiple conforming types.
Let’s return to our earlier `Drawable` protocol. By adding an extension, we can give every type that conforms to `Drawable` a default implementation of a new method called `drawBorder`, which will print out a border in the drawing color. This lets any conforming type leverage this new functionality without needing to explicitly implement it:
extension Drawable { func drawBorder() { print("Drawing a border in color: (color)") } }
With this addition, both `Circle` and `Square` can now call `drawBorder()` directly:
let circle = Circle(color: "red") circle.draw() // Output: Drawing a red circle. circle.drawBorder() // Output: Drawing a border in color: red let square = Square(color: "blue") square.draw() // Output: Drawing a blue square. square.drawBorder() // Output: Drawing a border in color: blue
Notice how `Circle` and `Square` automatically gain the `drawBorder()` method provided by the `Drawable` protocol extension without requiring any further code in their implementations. This not only adheres to the DRY principle but also enhances the expressiveness of our types.
Moreover, protocol extensions can also provide default implementations for properties. By using computed properties, we can extend these protocols to include additional, derived information. For instance, let’s extend our `Drawable` protocol to include a computed property that defines the shape type:
extension Drawable { var shapeType: String { return String(describing: type(of: self)) } }
Now, every conforming type can access the `shapeType` property without explicitly implementing it:
print(circle.shapeType) // Output: Circle print(square.shapeType) // Output: Square
This demonstrates how protocol extensions allow you to enrich and evolve protocol interfaces over time. It allows you to introduce new features to all conforming types with minimal effort and maximum consistency.
Default implementations also serve as a safety net. If a conforming type does not require a specialized behavior for a specific method, it can simply inherit the default implementation. This characteristic significantly reduces the overhead of implementing boilerplate code across multiple types.
Think this practical example: imagine you have a protocol for network request handling:
protocol NetworkRequest { func fetchData() } extension NetworkRequest { func fetchData() { print("Fetching data from a default endpoint.") } }
Any type conforming to `NetworkRequest` can now utilize the built-in `fetchData()` implementation, unless it specifically overrides it:
class UserRequest: NetworkRequest { func fetchData() { print("Fetching user data from a specific endpoint.") } }
Here, `UserRequest` overrides the default behavior, while other conforming types can just use the protocol’s implementation, showcasing how protocol extensions can streamline the implementation process while allowing for customization when necessary.
Real-World Use Cases of Protocol-Oriented Programming
In real-world applications, protocol-oriented programming (POP) in Swift can significantly enhance the design and architecture of software systems. By allowing different types to conform to the same protocol, developers can implement a more flexible and reusable code base. Let’s delve into some practical scenarios where POP shines.
Think the domain of user authentication. Imagine you have different authentication methods, such as password-based, token-based, and biometric authentication. By defining a protocol for authentication, you can create interchangeable authentication mechanisms without modifying the core logic of your application.
protocol Authenticatable { func authenticate() -> Bool } class PasswordAuthenticator: Authenticatable { private var password: String init(password: String) { self.password = password } func authenticate() -> Bool { // Simulate a password check. return password == "securePassword" } } class TokenAuthenticator: Authenticatable { private var token: String init(token: String) { self.token = token } func authenticate() -> Bool { // Simulate a token validation. return token == "validToken" } } class BiometricAuthenticator: Authenticatable { func authenticate() -> Bool { // Simulate biometric authentication. return true // Assume authentication is successful. } }
In this example, we have defined an Authenticatable
protocol with an authenticate()
method. Different classes, such as PasswordAuthenticator
, TokenAuthenticator
, and BiometricAuthenticator
, implement this protocol, allowing for various authentication strategies. This decouples the authentication logic from the specifics of each method, enabling flexibility in managing authentication mechanisms.
Next, think a scenario involving payment processing. You may need to integrate multiple payment gateways, such as credit card, PayPal, and Apple Pay. By employing protocols, you can design a uniform interface for handling payments.
protocol PaymentProcessor { func processPayment(amount: Double) -> Bool } class CreditCardProcessor: PaymentProcessor { func processPayment(amount: Double) -> Bool { // Simulate credit card processing. print("Processing credit card payment of $(amount).") return true } } class PayPalProcessor: PaymentProcessor { func processPayment(amount: Double) -> Bool { // Simulate PayPal processing. print("Processing PayPal payment of $(amount).") return true } } class ApplePayProcessor: PaymentProcessor { func processPayment(amount: Double) -> Bool { // Simulate Apple Pay processing. print("Processing Apple Pay payment of $(amount).") return true } }
The PaymentProcessor
protocol serves as the foundation for various payment methods. Each payment processor class implements the processPayment(amount:)
method, allowing the application to process payments without needing to know the specifics of each gateway.
Another compelling use case for protocol-oriented programming is within the scope of networking. By defining a protocol for making network requests, you can easily swap out different implementations, such as mocking for tests or using different networking libraries.
protocol NetworkService { func fetch(url: String, completion: @escaping (Data?) -> Void) } class URLSessionService: NetworkService { func fetch(url: String, completion: @escaping (Data?) -> Void) { guard let url = URL(string: url) else { completion(nil) return } let task = URLSession.shared.dataTask(with: url) { data, _, _ in completion(data) } task.resume() } } class MockNetworkService: NetworkService { func fetch(url: String, completion: @escaping (Data?) -> Void) { // Simulate a network call with mock data. let mockData = "Mock data".data(using: .utf8) completion(mockData) } }
In this example, NetworkService
serves as an interface for fetching network data. The URLSessionService
handles actual network requests, while the MockNetworkService
provides a mock implementation for testing purposes. This approach allows for easy testing and flexibility in swapping out networking strategies without disrupting the application’s architecture.