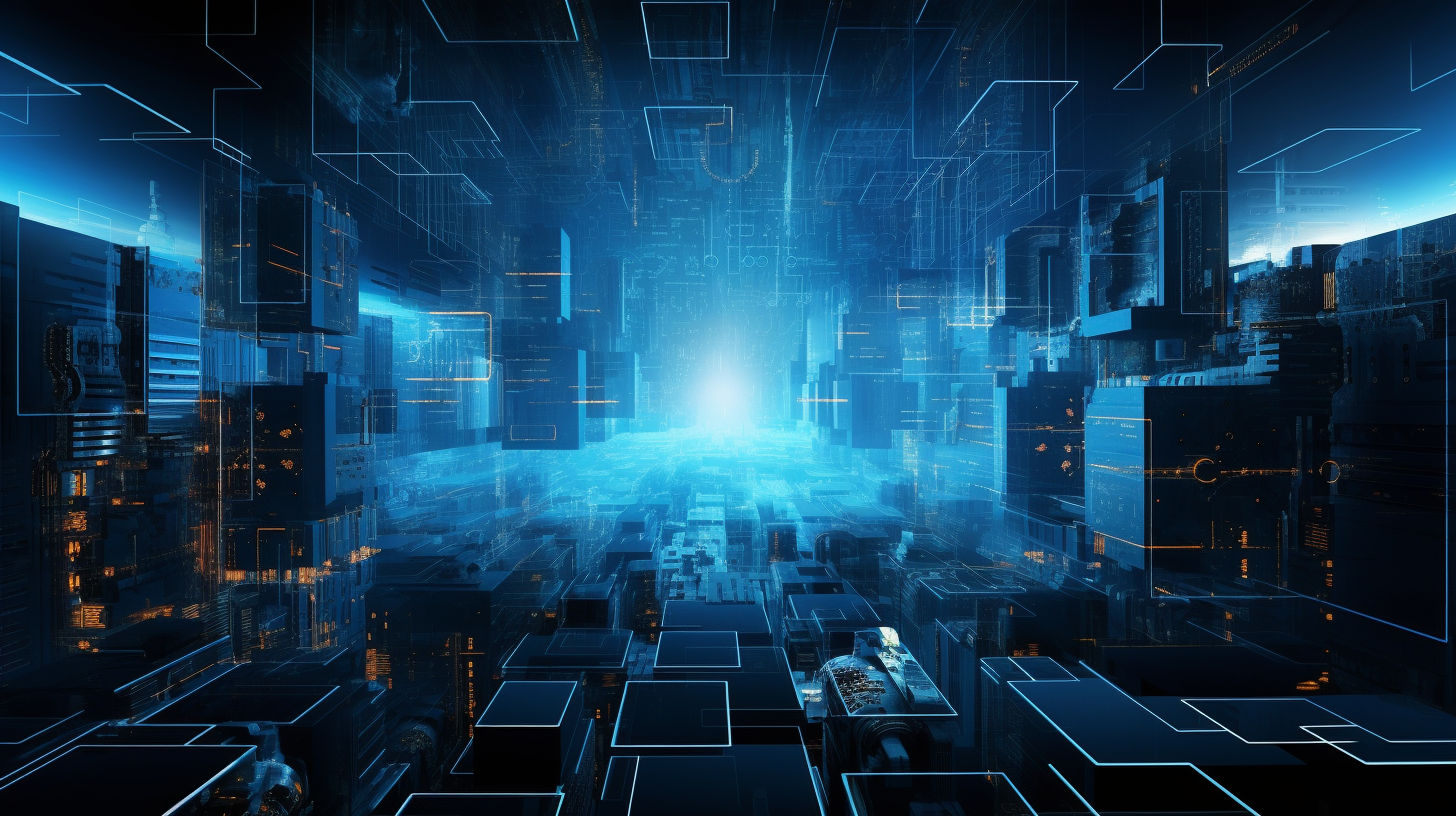
Advanced Text Manipulation in Bash
Regular expressions (regex) are an incredibly powerful tool for text manipulation in Bash. They offer a compact and efficient way to search, match, and manipulate strings based on patterns, making them an essential skill for any serious Bash developer. Let’s delve into the core concepts of mastering regular expressions in Bash.
At the heart of regex is the notion of pattern matching. A regex pattern defines a search criteria that can match character sequences in strings. For example, consider the following simple regex:
^abc
This pattern matches any string that starts with “abc”. The caret (^) symbol asserts the position at the start of the string, making it quite useful for validating prefixes.
To see regex in action, you can use the grep
command, which is a quintessential tool for searching text. Here’s an example:
echo -e "abcnabdnxyz" | grep '^a'
This command outputs:
abc abd
Both “abc” and “abd” begin with “a”, so they match the regex pattern.
Regular expressions also support a plethora of special characters. For instance, the dot (.) matches any single character. To illustrate this:
echo -e "batncatndog" | grep 'c.t'
The output will be:
cat
Here, “cat” matches the pattern where any character can occupy the position of the dot.
Furthermore, quantifiers can modify the number of occurrences. For instance, the asterisk (*) means “zero or more” of the preceding element:
echo -e "anbnaaanabnabc" | grep 'a*'
This will yield:
a b aaa ab abc
As you can see, every line matches because “a*” includes zero instances of “a” as well.
For more complex patterns, you can use groups and alternation. Parentheses allow you to group patterns, and the pipe (|) serves as a logical OR. Here’s an example:
echo -e "catndognbat" | grep -E '(cat|bat)'
The output will be:
cat bat
This showcases matching either “cat” or “bat”.
When using Bash scripts, be mindful of the quoting mechanism, as certain characters in regex can have special meanings in the shell. Always enclose your regex patterns in single quotes to prevent the shell from interpreting them before passing them to the regex engine.
For a more robust handling of regex in Bash, the [[ ]]
construct supports regex matching natively:
string="hello world" if [[ $string =~ ^hello ]]; then echo "Matched!" fi
In this example, the condition checks if the variable string
begins with “hello”. If it does, it echoes “Matched!”.
By mastering these regex techniques, you can effectively harness the power of Bash to perform advanced text manipulation and processing tasks. With practice, you’ll find that regex can handle some of the most complex text processing challenges with elegance and efficiency.
Stream Processing with awk and sed
When it comes to stream processing in Bash, two tools reign supreme: awk and sed. Both are incredibly powerful for manipulating text in a flexible and efficient manner, which will allow you to perform complex operations on input streams or files. Each tool has its strengths, and understanding how to leverage them can significantly enhance your text processing capabilities in Bash.
awk is a versatile programming language primarily focused on pattern scanning and processing. It excels at handling structured data, such as CSV or TSV files, making it ideal for tasks that involve data analysis or extraction. The syntax is designed around the idea of pattern-action pairs, where you specify a pattern to match and an action to perform on the lines that match that pattern.
Consider the following example, where we want to extract the second column from a CSV file:
awk -F, '{print $2}' file.csv
In this case, -F,
tells awk to use a comma as the field separator. The {print $2}
action instructs awk to print the second field for each line that it processes. This command can be quite handy when dealing with structured text data.
Another powerful feature of awk is its ability to perform calculations. For instance, if you had a file that contained numerical data and you wanted to sum the values in the first column, you could do so as follows:
awk '{sum += $1} END {print sum}' file.txt
Here, we are accumulating the values of the first column into the variable sum
, and after processing all the lines, awk prints the total sum.
On the other hand, sed (short for stream editor) is primarily used for parsing and transforming text using a simple, compact language. It excels at basic text substitutions and deletions but can also handle more complex tasks through its scripting capabilities. A common use case for sed is to perform substitutions. For example, if you want to replace all occurrences of “apple” with “orange” in a file, you can use:
sed 's/apple/orange/g' file.txt
In this command, s/apple/orange/g
is a substitution command where s
stands for substitute, g
indicates that we want to replace all occurrences on each line, not just the first. This is an essential tool for text replacements in files or streams.
In addition to substitutions, sed can delete lines or sections of text that match a specified pattern. For instance, to delete all blank lines from a file, you could run:
sed '/^$/d' file.txt
Here, the /^$/d
syntax instructs sed to delete lines that match the regex for blank lines (those that start and end with nothing).
Combining awk and sed in a single pipeline can further enhance your text processing capabilities. For example, you might want to process a file to replace specific terms and then extract certain fields:
sed 's/apple/orange/g' file.txt | awk -F, '{print $2}'
In this command, we first use sed to perform a substitution, and then pipe the output to awk to extract the second field from the modified text. This kind of chaining allows for sophisticated text processing workflows where you can leverage the strengths of both tools seamlessly.
For efficient stream processing, it is vital to understand the nuances of both awk and sed. Mastery of these tools will enable you to tackle a wide variety of text manipulation tasks, transforming raw data into meaningful insights with ease and precision.
Efficient String Operations and Substitutions
Efficient string operations and substitutions in Bash are fundamental aspects of text processing that can save you time and enhance your scripts’ performance. With a deep understanding of these operations, you can manipulate strings rapidly and effectively, allowing for streamlined workflows and cleaner code.
One of the most common string operations in Bash is concatenation, which allows you to combine multiple strings into one. This is done simply by placing the strings next to each other. For example:
string1="Hello" string2="World" combined="$string1 $string2" echo $combined
The output of this script would be:
Hello World
Next, let’s explore variable substitution, which is important for incorporating dynamic content into your strings. Bash allows for easy substitution of variable values within strings, making it highly versatile for scripting. For instance:
name="Alice" greeting="Hello, $name!" echo $greeting
This would print:
Hello, Alice!
Another important aspect of string manipulation is substring extraction. You can extract parts of a string using parameter expansion. For example, if you want to extract the first five characters of a string:
text="Bash Scripting" substring=${text:0:4} echo $substring
The output will be:
Bash
Bash also offers an efficient way to perform string replacement. Using parameter expansion, you can replace substrings without the need for external tools. Here is an example:
input="The quick brown fox jumps over the lazy dog." output=${input//fox/cat} echo $output
This will result in:
The quick brown cat jumps over the lazy dog.
For cases where you need to perform conditional replacements, using the `if` statement in conjunction with parameter expansion can be quite handy. For example:
text="Hello, World!" if [[ $text == *World* ]]; then text=${text/World/Bash} fi echo $text
This code checks if the string contains “World” and replaces it with “Bash”, yielding:
Hello, Bash!
Moreover, for bulk replacements across multiple files or extensive text streams, using the `sed` command is a powerful alternative. Here’s how you can use `sed` for efficient string substitutions:
Hello World
0
The `-i` flag edits the file in place, and the `g` flag ensures all occurrences in each line are replaced. This is notably useful in scripts that need to modify configuration files or large datasets.
In conclusion, mastering efficient string operations and substitutions in Bash empowers you to write cleaner, more effective scripts. With the right techniques and tools, you can streamline your text processing tasks, making your Bash scripting experience both powerful and enjoyable.
Parsing and Formatting Text Data
# Sample text data stored in a variable text_data="Name: Neil HamiltonnAge: 30nOccupation: DevelopernnName: Jane SmithnAge: 25nOccupation: Designer" # Parsing the text data using a while loop while IFS= read -r line; do # Check if the line contains 'Name' if [[ $line == Name:* ]]; then echo "Extracted Name: ${line#*: }" # Extracts the name by removing 'Name: ' fi done <<< "$text_data"
Parsing and formatting text data in Bash can be a daunting task, yet it’s essential for automating workflows and extracting meaningful information from structured or semi-structured text. With a bit of ingenuity and the right commands, you can transform piles of text into neatly formatted outputs that meet your needs.
Ponder data that comes in a format like free text or key-value pairs. The first step in parsing such data is often to read it line by line. Using a while loop combined with the read command, you can process each line one at a time. The example above illustrates this technique, where we use the ‘IFS’ (Internal Field Separator) variable to read lines from a multi-line string.
In the example, we examine each line for a specific pattern, in this case, lines that start with “Name:”. The use of parameter expansion ${line#*: } allows us to strip off the “Name: ” prefix and extract just the name itself. This simple yet powerful method of line processing can form the backbone of more complex parsing tasks.
# Using awk for parsing structured data echo -e "Name,Age,OccupationnAlex Stein,30,DevelopernJane Smith,25,Designer" | awk -F, '{printf "Name: %s, Age: %sn", $1, $2}'
When dealing with structured data, you may find tools like awk invaluable. In the example above, we read a CSV-like string and use awk to specify a comma as the field separator using the -F,
option. The printf
function allows for formatted output, enabling a clean presentation of extracted fields. This flexibility makes awk a powerful ally in parsing data quickly and effectively.
# Formatting text output with sed echo "Hello World!" | sed 's/[[:space:]]+/ /g'
Formatting the output is just as important as parsing the data. To achieve cleaner output, you can employ sed to manipulate whitespace. The command above uses a regular expression to replace multiple spaces with a single space, resulting in a more readable format. Such string manipulations are often necessary when dealing with poorly formatted input data.
Moreover, when you encounter JSON or XML data, you can still utilize tools like jq or xmlstarlet for parsing and formatting, though these require external dependencies. Always think the nature of your input data and choose the right tools accordingly.
# Example of parsing JSON using jq json_data='{"employees":[{"name":"Vatslav Kowalsky","age":30},{"name":"Jane Smith","age":25}]}' echo $json_data | jq '.employees[] | {Name: .name, Age: .age}'
This command uses jq, a lightweight command-line JSON processor, to extract and format employee names and ages from a JSON string. The syntax is concise yet powerful, illustrating how specialized tools can simplify complex parsing tasks.
Mastering text parsing and formatting in Bash involves a combination of using built-in commands, regular expressions, and external utilities. The key to success lies in knowing your data format, selecting the appropriate tools, and using their capabilities to achieve the desired output. With practice, you will be able to handle any text manipulation challenge with confidence and efficiency.
Advanced Techniques with Bash Arrays and Loops
When it comes to manipulating data in Bash, arrays and loops are indispensable tools, allowing you to manage collections of items and automate repetitive tasks with ease and precision. By mastering these techniques, you’ll gain the ability to handle complex data structures and perform iterative operations efficiently.
Bash supports one-dimensional and associative arrays, each serving distinct purposes. One-dimensional arrays are indexed by numerical values, while associative arrays use strings as indices. Let’s start with a basic example of a one-dimensional array:
fruits=("apple" "banana" "cherry") echo ${fruits[1]} # Outputs: banana
Here, we declare an array called fruits
and access its second element by using its index, which is zero-based. To loop through all elements of an array, a for
loop can be employed:
for fruit in "${fruits[@]}"; do echo $fruit done
This loop iterates over each item in the fruits
array and prints it. Note the use of quotes around ${fruits[@]}
to handle elements containing spaces properly.
Now, if you need to utilize an associative array, you can declare it using the declare
command. Think the following example where we store fruit colors:
declare -A fruit_colors fruit_colors=( ["apple"]="red" ["banana"]="yellow" ["cherry"]="red" ) echo "The color of an apple is ${fruit_colors[apple]}." # Outputs: The color of an apple is red.
In this snippet, we define an associative array fruit_colors
that maps fruit names to their respective colors. This allows for more meaningful data representation and quick lookups.
To iterate through an associative array, you can use a for
loop as well:
for fruit in "${!fruit_colors[@]}"; do echo "$fruit is ${fruit_colors[$fruit]}." done
This loop utilizes the !fruit_colors[@]
syntax to retrieve all keys, iterating over them to print each fruit with its associated color.
When it comes to processing data in a structured manner, loops are invaluable. Consider a scenario where you want to process a list of file names. You can read the file names into an array and apply operations in a loop:
files=("file1.txt" "file2.log" "file3.conf") for file in "${files[@]}"; do if [[ -f $file ]]; then echo "$file exists." else echo "$file does not exist." fi done
This example checks if each file in the files
array exists, outputting the result accordingly. The ability to combine conditional statements with loops allows for sophisticated data processing.
Another powerful loop construct in Bash is the while
loop, which can be particularly useful when reading lines from a file or processing input until a condition is met:
counter=0 while [[ $counter -lt 5 ]]; do echo "Counter is at: $counter" ((counter++)) done
This loop will print the value of counter
from 0 to 4. The use of ((counter++))
demonstrates how to increment the counter variable, showcasing the power of arithmetic expressions in Bash.
Combining arrays with loops allows you to manage data structures and automate repetitive tasks effectively. As you become proficient in these techniques, you’ll find yourself equipped to handle an array of text manipulation challenges with confidence and finesse, transforming your Bash scripting capabilities into a formidable toolset.