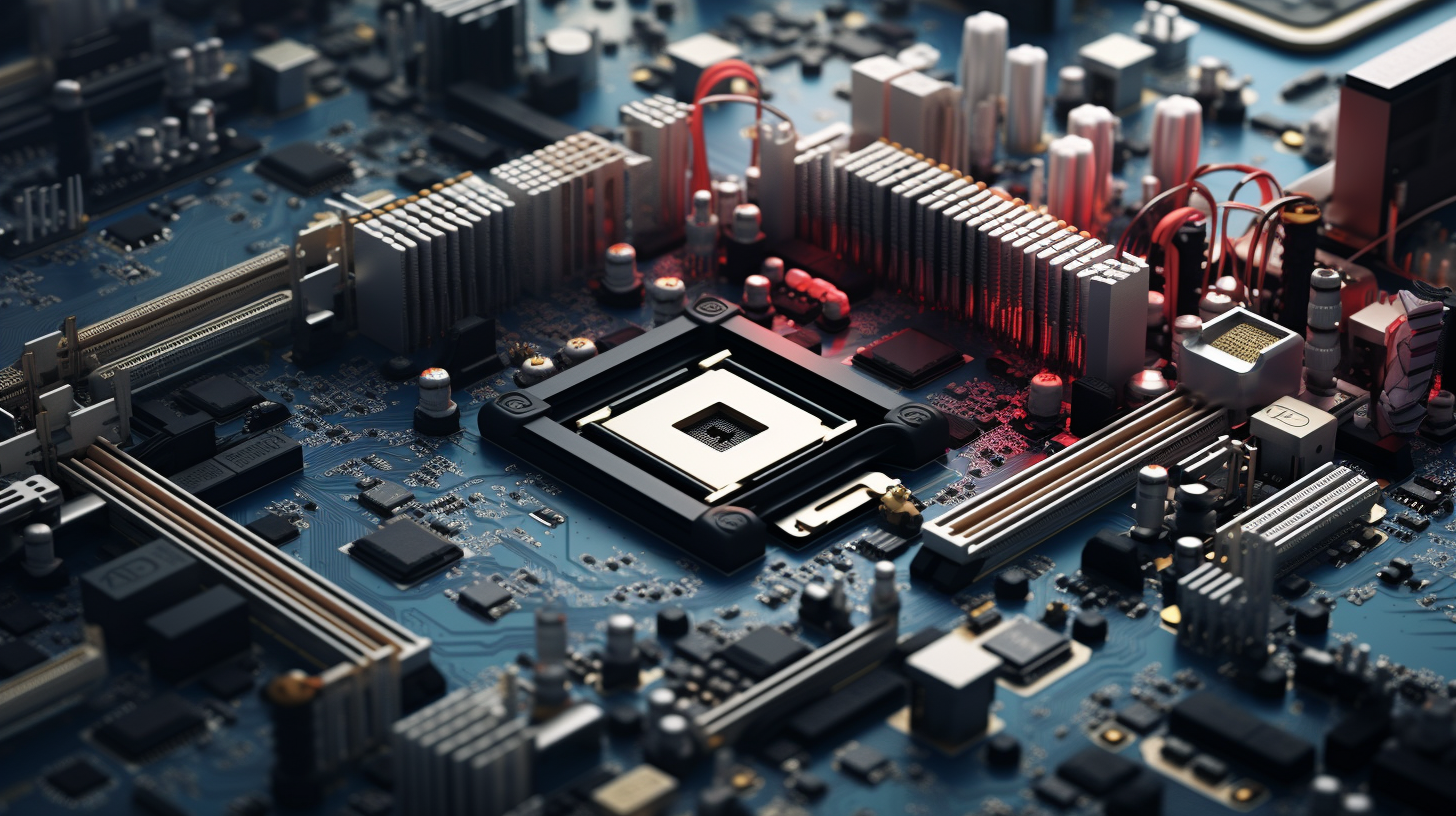
Automating System Updates with Bash
In the context of system management, understanding the underlying mechanisms that drive system updates is essential. At its core, a system update is a process that ensures your operating system and installed applications are running the latest versions, which can include security patches, bug fixes, and new features. Different operating systems employ various package management systems, and these systems play a vital role in how updates are fetched, installed, and managed.
Unix-like systems (such as Linux distributions) typically use package managers like Apt for Debian-based systems (like Ubuntu), Yum or Dnf for Red Hat-based systems (like Fedora), and Pacman for Arch Linux. Each of these tools abstracts the complexity of managing software packages, allowing users to perform updates with simple commands. For example, on a Debian-based system, you can check for available updates and apply them with:
sudo apt update && sudo apt upgrade
This command first refreshes the package index, ensuring you’re aware of the latest available packages, and then upgrades installed packages to their latest versions.
On the other hand, Windows systems utilize the Windows Update mechanism, which operates somewhat differently. Windows handles updates through a centralized service that automates the retrieval and installation of updates. However, it can be somewhat opaque, presenting a challenge for users who prefer more control.
While understanding these mechanisms is essential, so is grasping the implications of updates. When updates are applied, they can introduce changes that might affect system stability or compatibility with existing applications. Consequently, automating updates should be approached with caution.
Furthermore, many systems allow for the configuration of update settings. For instance, on Debian-based systems, you can set up unattended upgrades to handle updates automatically without user intervention. This feature can significantly enhance security by ensuring that critical updates are applied promptly.
To configure unattended upgrades, you may need to install the unattended-upgrades package and then modify its configuration. A simple command to install it would look like this:
sudo apt install unattended-upgrades
After installation, you can edit the configuration file located at /etc/apt/apt.conf.d/50unattended-upgrades
to specify which updates to apply automatically.
Understanding these systems and their configurations will empower you to create effective Bash scripts that can automate updates while maintaining control over your system’s health and security.
Setting Up a Bash Script for Updates
Now that we have a solid grasp of the update mechanisms in various operating systems, it’s time to delve into the practical aspects of automating these updates with a Bash script. The goal here is to create a script that can efficiently check for and install updates, while also providing options for logging and monitoring the update process.
To set up a basic Bash script for system updates, you need to start with the shebang line, which tells the system that this script should be executed with Bash. Follow this with commands relevant to your package manager. Here’s a simple example for a Debian-based system:
#!/bin/bash # Update the package index sudo apt update # Upgrade the installed packages sudo apt upgrade -y # Optionally, you can also include autoremove to clean up unnecessary packages sudo apt autoremove -y # Log the update process echo "Updates applied on $(date)" >> /var/log/update-script.log
This script begins by updating the package index to ensure you have the latest information about available packages. The `apt upgrade -y` command applies all available upgrades automatically, where the `-y` flag confirms any prompts that may arise. Finally, it appends a log entry to a designated log file, which can be helpful for tracking changes over time.
If you are using a different Linux distribution, you will need to modify the package management commands accordingly. For example, a script for a Red Hat-based system using Dnf might look like this:
#!/bin/bash # Update the package index and upgrade installed packages sudo dnf upgrade -y # Clean up unnecessary packages sudo dnf autoremove -y # Log the update process echo "Updates applied on $(date)" >> /var/log/update-script.log
In this script, `dnf upgrade -y` performs the same function as the `apt upgrade` command in the previous example, but adapted to the Dnf package manager.
Once you have crafted your Bash script, ensure that it’s executable. You can make your script executable by running:
chmod +x /path/to/your-script.sh
After making your script executable, you can run it manually to verify that it performs as expected. Keep an eye on the log file to check for any issues that arise during the update process.
This foundational script can serve as a springboard for more advanced features, such as error handling, notifications, and conditional logic to check for specific types of updates. As you build on this script, remember that the goal is not just to automate the updates, but to do so in a way that keeps your system secure and stable.
Scheduling Automated Updates with Cron
Automating the execution of your update script is where the power of Cron, the time-based job scheduler in Unix-like operating systems, comes into play. Cron allows you to schedule commands or scripts to run at specified intervals, which is particularly useful for system maintenance tasks like applying updates. Setting up a Cron job is relatively simpler, but it requires an understanding of Cron’s syntax and how to customize it to fit your needs.
The first step in scheduling your update script is to open the crontab file for editing. You can do this with the following command:
crontab -e
This command opens the crontab file in your default text editor, so that you can add your scheduled tasks. Cron jobs are specified using a specific syntax that defines when and how often they’re executed. The basic structure is:
* * * * * /path/to/your-script.sh
Each asterisk represents a time field, which corresponds to:
- Minute (0-59)
- Hour (0-23)
- Day of the month (1-31)
- Month (1-12)
- Day of the week (0-7) (Sunday is both 0 and 7)
For example, to schedule your update script to run every day at 2 AM, you would add the following line to your crontab:
0 2 * * * /path/to/your-script.sh
This line specifies that the script will be executed at the 0th minute of the 2nd hour of every day, regardless of the day of the month, month, or day of the week.
In addition to scheduling daily updates, you might want to think running your script weekly or monthly, depending on your system’s requirements and the nature of the updates. For instance, to execute the script every Sunday at 3 AM, you would use:
0 3 * * 0 /path/to/your-script.sh
Once you’ve added your desired Cron jobs, save and exit the editor. Cron will automatically pick up the changes, and the scheduled tasks will begin executing at the specified times.
It’s essential to monitor your script’s execution, especially when automating updates. You can check the system’s mail (usually accessible via the mail
command) for cron output, or you can redirect the output and errors of your script to a log file. You can modify your crontab entry like this:
0 2 * * * /path/to/your-script.sh >> /var/log/update-script.log 2>&1
In this command, >>
appends standard output to the log file, and 2>&1
ensures that any errors are also captured in the log. This way, you can conveniently check the log file for both successful updates and any issues that occurred during execution.
Finally, keep in mind that your automated updates should be subjected to scrutiny. Depending on the environment, you may not want to perform updates automatically without first reviewing what changes are being made. Consider setting up notifications or manual checks if your updates could potentially disrupt critical services or applications.
Handling Update Notifications and Logs
In the context of automated updates, effective handling of notifications and logs is paramount. Simply applying updates without oversight can lead to unforeseen issues, making it vital to implement a system that alerts you to changes and records detailed information about each update process. This allows you to maintain a comprehensive view of your system’s health while providing the transparency necessary for troubleshooting.
When it comes to logging, the first step is to ensure that your Bash script captures all relevant output—both successes and errors. Modify your script to append detailed log entries, including timestamped messages that indicate the status of the update operations. Below is an enhanced example demonstrating how to implement this:
#!/bin/bash LOG_FILE="/var/log/update-script.log" { echo "Starting update process on $(date)" # Update the package index sudo apt update # Upgrade the installed packages sudo apt upgrade -y # Clean up unnecessary packages sudo apt autoremove -y echo "Updates applied successfully on $(date)" } >> "$LOG_FILE" 2>&1
In this script, we use braces `{}` to group commands, allowing us to redirect all output to the log file. The `2>&1` part ensures that both standard output and standard error messages are captured, providing a complete picture of the update process.
To improve the utility of notifications, you can integrate email alerts or desktop notifications to inform you when updates are applied. For example, using the `mail` command, you can notify yourself of the update results by adding the following lines to your script:
EMAIL="[email protected]" if [ $? -eq 0 ]; then echo "Updates were successful" | mail -s "Update Notification" $EMAIL else echo "Updates failed" | mail -s "Update Notification" $EMAIL fi
This snippet checks the exit status of the last command executed (which is the update command) and sends an appropriate email based on whether it succeeded or failed. You can also customize the subject and body of the email as needed.
In addition to email notifications, ponder implementing log rotation to prevent log files from consuming excessive disk space. Use tools such as `logrotate` to manage log files efficiently. Setting this up can be as simple as creating a configuration file in `/etc/logrotate.d/` for your update logs:
/var/log/update-script.log { daily rotate 7 compress missingok notifempty create 0640 root root }
This configuration will rotate the log file daily, keeping seven days of archived logs, while compressing older versions to save space. The `missingok` option tells logrotate to ignore the log file if it isn’t present, and `notifempty` prevents rotation if the log file is empty.
By effectively managing notifications and logs, you can create a robust automated update system that not only applies updates but also keeps you informed about the process. This level of transparency aids in maintaining system integrity and allows for swift troubleshooting when necessary. The combination of detailed logging, alert notifications, and log management is key to mastering automated system updates.
Testing and Troubleshooting Your Update Scripts
When it comes to automating system updates with Bash, testing and troubleshooting your scripts is an indispensable part of the process. Even the most meticulously written scripts can encounter unexpected issues, whether due to system configurations, network problems, or package manager idiosyncrasies. Thus, developing a systematic approach to testing your update scripts can save you headaches down the line.
Start by testing your Bash script manually in a controlled environment. This can be as simple as executing the script in a terminal and observing the output for errors. For instance, if you run your update script without automating it, you can see firsthand any issues that arise:
bash /path/to/your-script.sh
Monitor the terminal output carefully. Any errors, warnings, or prompts that appear can provide insight into what may need adjusting. If your script fails, you can capture the exit status of the last command run using:
echo $?
A non-zero exit status typically indicates an error. Integrating checks for the exit status into your script will help you catch issues preemptively. For example, you can include a conditional statement to exit the script if a command fails:
#!/bin/bash sudo apt update if [ $? -ne 0 ]; then echo "Failed to update package index." >&2 exit 1 fi sudo apt upgrade -y if [ $? -ne 0 ]; then echo "Failed to upgrade packages." >&2 exit 1 fi
In this snippet, after each command, we check the exit status and print an error message to standard error if something goes wrong, ultimately terminating the script early if a critical failure occurs.
Another practical approach for troubleshooting is to implement debug logging. It can be extremely beneficial to gain a deeper understanding of what your script is doing at each stage of execution. You can modify your script to include verbose logging by simply adding the `set -x` command at the beginning:
#!/bin/bash set -x # Your update commands here
This command causes Bash to print each command before executing it, providing a detailed trace of the script’s execution. This debugging output can be redirected to a separate debug log file for easier inspection, as follows:
bash /path/to/your-script.sh >> /var/log/update-debug.log 2>&1
Additionally, consider running your script in a test environment—a virtual machine or a container where you can safely execute updates without affecting a production system. This isolated environment allows you to trial different scenarios and configurations without risk. If you encounter an issue, you can quickly revert to a snapshot or a clean state.
Once you feel confident that your script operates correctly, you can introduce it into your scheduled automation. However, remain vigilant: even automated processes require ongoing oversight. Continue to check your logs regularly, and think setting up notifications for script execution failures, as mentioned previously. This proactive approach will enable you to catch issues before they escalate into larger problems.
Overall, the key to effective scripting lies in thoroughly testing and troubleshooting your scripts as they evolve. By implementing error checking, debug logging, and using controlled environments, you can ensure that your automated updates run smoothly and reliably, keeping your system secure and up to date.