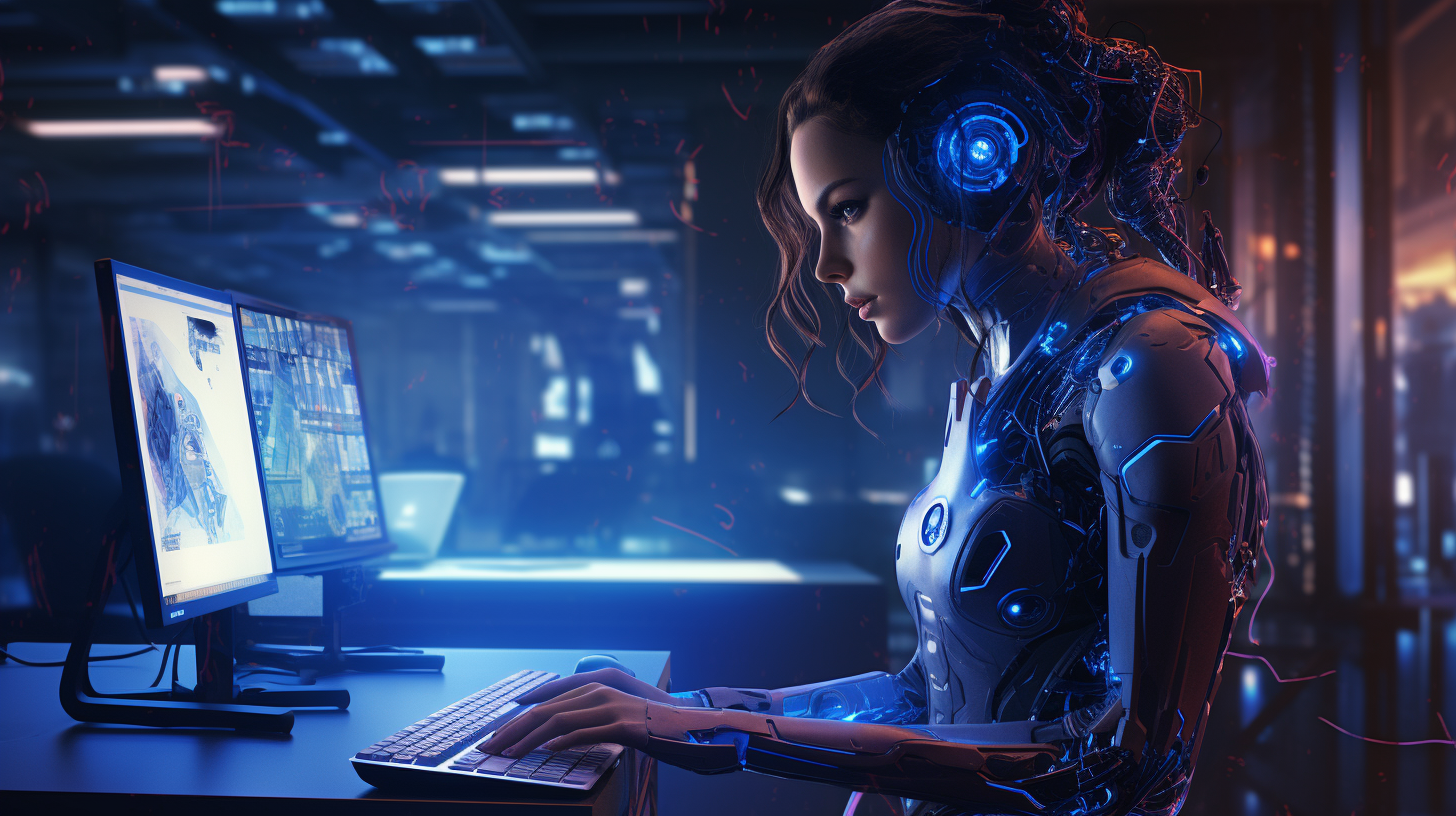
Automating Tasks with Bash Scripts
Bash scripting is a powerful way to automate tasks in Unix-based systems. At its core, a Bash script is simply a text file containing a sequence of commands that the Bash interpreter can execute. Understanding the basics of Bash scripting is essential for using its full potential.
To start, Bash scripts usually begin with a shebang line, which indicates the script’s interpreter. For Bash scripts, this line is:
#!/bin/bash
This line must be the very first line in your script, and it tells the operating system to use the Bash shell to execute the following commands. After the shebang line, you can write your commands just as you would in the terminal.
Variables in Bash scripting allow you to store data that can be referenced later in your script. Declaring a variable is straightforward:
my_variable="Hello, World!"
To access the value of a variable, you precede it with a dollar sign:
echo $my_variable
This command outputs “Hello, World!” to the terminal. It’s important to note that there should be no spaces around the equal sign when assigning values to variables.
Bash also supports control structures such as loops and conditionals, which enable you to write more complex scripts. For example, a simple loop can be implemented with a for statement:
for i in {1..5}; do echo "Iteration $i" done
This loop will print “Iteration 1” through “Iteration 5” to the terminal. Similarly, conditionals allow you to execute commands based on certain conditions:
if [ "$my_variable" == "Hello, World!" ]; then echo "The variable contains the expected value." else echo "The variable does not contain the expected value." fi
In this example, the script checks if my_variable
holds the value “Hello, World!” and prints a message accordingly.
Error handling is another critical aspect of Bash scripting. By checking the exit status of commands, you can ensure that your scripts behave as expected. The exit status of the last command executed can be accessed using the variable $?
. A value of 0 indicates success, while any non-zero value indicates an error:
some_command if [ $? -ne 0 ]; then echo "An error occurred." fi
By mastering these foundational concepts of Bash scripting, you’ll be well on your way to creating effective automation scripts that can save time and streamline workflows.
Creating Your First Bash Script
Once you have grasped the fundamentals, it’s time to create your first Bash script. This process is simpler and can be done in a few simple steps. Let’s walk through them, so you can get your hands dirty with some scripting.
First, you need to open a text editor to write your script. You can use any text editor that you’re comfortable with, such as nano, vim, or even a graphical editor like gedit. For our example, we’ll use nano. To create a new file named my_first_script.sh, open your terminal and type:
nano my_first_script.sh
Inside nano, begin your script with the shebang line:
#!/bin/bash
Next, let’s add a simple command that outputs a message to the terminal. Below the shebang line, type the following:
echo "Hello, Bash scripting!"
Your complete script should now look like this:
#!/bin/bash echo "Hello, Bash scripting!"
Now save the file and exit nano by pressing CTRL + X, then Y to confirm changes, and Enter to exit.
Before you can run your script, you need to make it executable. You do this with the chmod command:
chmod +x my_first_script.sh
Now, you can execute your script by typing:
./my_first_script.sh
If everything is set up correctly, you should see the output:
Hello, Bash scripting!
Congratulations! You’ve just created and executed your first Bash script. This simple example demonstrates the process of writing, saving, making executable, and running a script. As you gain confidence, you can start incorporating more complex logic, variables, and control structures into your scripts.
To expand your scripting abilities, try modifying your script to include user input. For instance, you can prompt the user for their name and greet them accordingly. Update your script as follows:
#!/bin/bash read -p "What is your name? " name echo "Hello, $name! Welcome to Bash scripting!"
After saving your changes and running the script again, you will be prompted to enter your name, and it will greet you personally. This interactivity is one of the key features that makes Bash scripting both powerful and effortless to handle.
As you gain experience, think experimenting with various commands and structures. Remember, the world of Bash scripting is vast and full of possibilities, so don’t hesitate to explore and learn more advanced techniques at your pace.
Common Automation Tasks with Bash
Automating tasks with Bash can significantly enhance your productivity by so that you can execute repetitive commands or sequences of commands with ease. Let’s delve into some common automation tasks that can be efficiently handled using Bash scripts.
One of the most frequent tasks is file management. You can create scripts to automate file backups, organize files, or even clean up directories. For instance, imagine you want to back up your documents every night. You could create a script to copy your documents to a backup directory:
#!/bin/bash # Backup script for documents cp -r ~/Documents/* ~/Backup/Documents/ echo "Backup completed successfully!"
In this script, we use the cp
command to copy files from the Documents
directory to a Backup
directory. The -r
option allows the copying of directories recursively.
Another common automation task is monitoring system performance. You can write scripts that check disk usage, memory consumption, or CPU load, alerting you when certain thresholds are exceeded. Here’s a basic example that checks disk usage and alerts you if usage exceeds 90%:
#!/bin/bash # Check disk usage and alert if over 90% usage=$(df / | grep / | awk '{ print $5 }' | sed 's/%//g') if [ "$usage" -gt 90 ]; then echo "Warning: Disk usage is above 90%! Current usage: $usage%" else echo "Disk usage is at a safe level: $usage%" fi
This script utilizes the df
command to check the disk space usage and processes the output with grep
, awk
, and sed
to extract and manipulate the relevant data.
Additionally, Bash scripting is excellent for scheduling tasks. Using cron
, you can set up your scripts to run at specific intervals. For instance, if you want your backup script to run every day at midnight, you can add a cron job:
0 0 * * * /path/to/your/backup_script.sh
This line in your crontab file specifies that the backup_script.sh
will execute at 12:00 AM every day.
Data manipulation is another area where Bash excels. You can automate the processing of text files, such as logs or CSV files, by using tools like awk
or sed
. For instance, if you want to extract specific columns from a CSV file, you can do something like this:
#!/bin/bash # Extract specific columns from CSV awk -F, '{ print $1, $3 }' data.csv > extracted_columns.txt echo "Columns extracted to extracted_columns.txt"
This script uses awk
to specify a comma as the field separator (-F,
) and prints the first and third columns from data.csv
into a new file called extracted_columns.txt
.
As you explore automation tasks with Bash, you’ll uncover a wealth of capabilities that can streamline your workflows. From file management and system monitoring to scheduling and data manipulation, the versatility of Bash scripting allows you to automate numerous tasks that would otherwise take considerable time and effort if done manually. With practice, you’ll develop scripts tailored to your specific needs, enhancing efficiency in your daily routines.
Debugging and Testing Your Scripts
Debugging and testing your scripts is an important phase in the development of Bash scripts, as it ensures that your automated tasks run smoothly and deliver the expected results. The process of debugging can be simpler, but it requires an understanding of how Bash processes commands and handles errors.
One of the first steps you should take when debugging is to run your script with the -x option. This option enables a mode of the shell where all executed commands are printed to the terminal before they are run. It provides a clear view of the flow of execution and can help identify where things might be going wrong. You can invoke this by running:
bash -x my_script.sh
Incorporating echo statements throughout your script is another effective debugging technique. By strategically placing echo
commands, you can track the values of variables and the flow of control structures. For instance:
#!/bin/bash my_variable="10" echo "Value of my_variable is: $my_variable" if [ "$my_variable" -gt 5 ]; then echo "my_variable is greater than 5" else echo "my_variable is not greater than 5" fi
This will help you confirm the variable’s value and show which branch of the conditional is being executed.
Another useful debugging strategy is using the set built-in command to control the shell’s behavior. You can use set -e
to make your script exit immediately upon encountering an error. That is particularly helpful in preventing cascading failures from subsequent commands that rely on the successful execution of previous ones. Conversely, you can use set -u
to treat unset variables as errors and exit immediately, which helps catch typos and other mistakes. Here’s how you can implement these options:
#!/bin/bash set -e set -u echo "This script will exit on error or unset variable." my_variable="Hello" echo $my_variable
In this script, if my_variable
were not set, it would terminate with an error, allowing you to catch issues early.
Testing your script in a controlled environment is equally important. Use small test cases to validate the logic in your scripts before deploying them in a live environment. Create a separate directory for testing scripts and any associated files to avoid accidental modification of your production data. This way, you can freely experiment without fear of causing disruptions.
Finally, take advantage of tools and frameworks tailored for testing Bash scripts. For instance, Bats (Bash Automated Testing System) is a testing framework that allows you to write tests for your Bash scripts in a simple, readable format. You can write tests that check if your functions return the expected output, handle errors correctly, and more. Here’s a basic example of what a Bats test might look like:
# my_test.bats @test "Test my_function" { run bash my_script.sh [ "$status" -eq 0 ] [ "${output}" = "Expected output" ] }
This test asserts that when you run my_script.sh
, the exit status is 0, indicating success, and the output matches the expected result.
By employing these debugging and testing techniques, you’ll enhance the reliability of your Bash scripts, making them robust and easier to maintain. Each failure is a learning opportunity, and as you refine your debugging skills, you will find yourself writing scripts that not only automate efficiently but also stand the test of time.
Best Practices for Writing Maintainable Scripts
When it comes to writing maintainable Bash scripts, adopting best practices is essential for ensuring that your scripts remain readable, reliable, and easy to modify in the future. As with any programming language, clarity and structure in your code are paramount, especially when scripts can become complex with time. Here are some key best practices to ponder.
1. Use Descriptive Variable and Function Names
Choosing meaningful names for your variables and functions can significantly improve the readability of your scripts. Rather than using single-letter or cryptic names, opt for descriptive identifiers that convey the purpose of each variable or function. For example:
# Poor naming a="Hello" b="World" # Better naming greeting="Hello" recipient="World"
By using names like greeting
and recipient
, anyone looking at your code can quickly understand its intent without needing extensive comments.
2. Comment Generously
Incorporating comments throughout your code can provide context and explanations for complex logic or decisions made in your scripts. However, avoid stating the obvious. Instead, focus on clarifying the intent behind your code. Here’s an example:
#!/bin/bash # This script backs up user documents to a specified backup directory backup_dir=~/Backup cp -r ~/Documents/* "$backup_dir/" echo "Backup completed successfully!"
In this example, the comment at the beginning clearly outlines the purpose of the script, allowing anyone reading it to grasp its functionality at a glance.
3. Structure Your Scripts Logically
Organizing your script into clear sections can improve readability. Ponder grouping related commands and using functions to encapsulate specific tasks. Functions not only make your script easier to navigate, but they also enable code reuse. Here’s how you might structure a script with functions:
#!/bin/bash function backup_documents { local backup_dir="$1" cp -r ~/Documents/* "$backup_dir/" echo "Backup completed successfully!" } function main { local backup_dir=~/Backup backup_documents "$backup_dir" } main
In this structure, the main
function serves as the entry point, while backup_documents
encapsulates the backup logic, enhancing clarity.
4. Handle Errors Gracefully
Incorporating error handling in your scripts is essential for maintainability. When something goes wrong, your script should provide meaningful feedback rather than failing silently. Using conditional statements and checking exit statuses can help manage errors effectively:
#!/bin/bash backup_dir=~/Backup if cp -r ~/Documents/* "$backup_dir/"; then echo "Backup completed successfully!" else echo "Error: Backup failed!" >&2 exit 1 fi
Here, the script checks if the cp
command executed successfully and provides an error message if it did not. This practice aids in diagnosing issues when they arise.
5. Avoid Hardcoding Values
Hardcoding values in your scripts can lead to difficulties when changes are needed. Instead, consider using variables or configuration files to store values that might change frequently. This not only makes your script more flexible but also reduces the risk of introducing errors when updates are required:
#!/bin/bash backup_dir=~/Backup document_dir=~/Documents if cp -r "$document_dir/"* "$backup_dir/"; then echo "Backup completed successfully!" else echo "Error: Backup failed!" >&2 exit 1 fi
In this example, the script uses variables for both backup_dir
and document_dir
, rendering it effortless to change the directories in one place if needed.
6. Format and Indent Your Code
Proper indentation and formatting can significantly enhance the readability of your Bash scripts. Consistent indentation helps identify blocks of code, such as loops and conditionals, making it easier to follow the script’s logic. Here’s a well-formatted example:
#!/bin/bash for file in ~/Documents/*; do echo "Processing $file" cp "$file" ~/Backup/ done
Using proper indentation allows the reader to quickly discern the flow of logic and understand the hierarchy of commands.
By implementing these best practices, you can create maintainable Bash scripts that are not only functional but also easy to read and understand. This approach will ultimately save you time in the long run by simplifying the process of updating and troubleshooting your scripts, fostering a smoother automation experience.