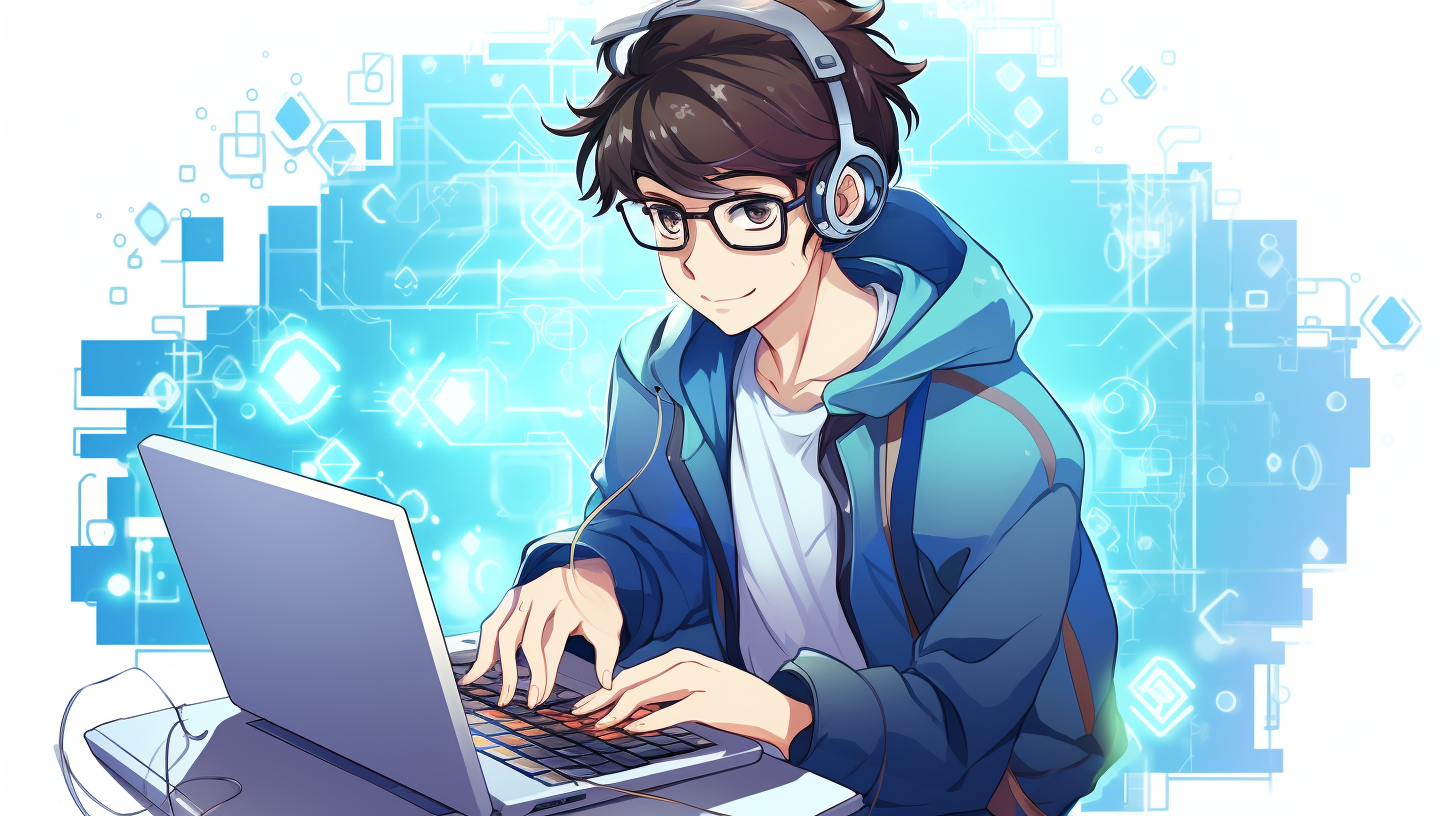
Bash and Git Integration
Understanding the Bash command line is essential for any Git user aiming to navigate their repository efficiently. Bash is a powerful shell that allows you to interact with your system through command-line instructions. By mastering some fundamental concepts, you can significantly enhance your Git workflow.
First, let’s familiarize ourselves with the basic structure of Bash commands. A typical command consists of the command name followed by options and arguments. For instance:
git status
In this example, git
is the command name, and status
is the argument that tells Git what action to perform.
Another crucial aspect is the use of options, which modify the behavior of commands. Options usually begin with a dash (-
) or double dash (--
). Here’s how you might use an option with the git log
command:
git log --oneline
This command displays the commit history in a compact format, making it easier to read at a glance.
Next, let’s look at how to navigate directories using Bash. The cd
command changes your current directory:
cd /path/to/your/repo
Once you are in the appropriate directory, you can start executing Git commands relevant to that repository. You can list files in the current directory using the ls
command:
ls
To create a new directory, use the mkdir
command:
mkdir new-feature
After creating a new branch, you might want to switch to it. The git checkout
command lets you change branches:
git checkout new-feature
With these basic commands, you’re well on your way to mastering Git in your Bash environment. The real power comes from combining these commands into more complex operations, which can streamline your development process significantly.
Remember, the command line is an interface designed for efficiency and speed. As you become more comfortable with these basics, you’ll find yourself navigating through Git repositories with ease.
Setting Up Git in Your Bash Environment
Setting up Git in your Bash environment is an important step towards using the full potential of version control in your projects. The setup process involves ensuring that Git is installed and properly configured for your needs, which can significantly improve your workflow.
First, you need to verify if Git is installed on your system. You can do this by running the following command in your Bash terminal:
git --version
If Git is installed, you’ll see the version number displayed. If it’s not installed, you can install it using your package manager. For example, on Ubuntu, you would execute:
sudo apt-get install git
Once Git is installed, the next step is to configure your user information, which is essential for identifying the author of the commits. You can set your name and email address with the following commands:
git config --global user.name "Your Name" git config --global user.email "[email protected]"
The –global flag means that these settings will apply to all repositories on your machine. If you want to set user information for a specific repository only, navigate to that repository and omit the –global flag when running the commands.
Another important configuration is setting your default text editor for Git. This editor will be used for writing commit messages and other inputs. For instance, to set Nano as your default editor, you can use:
git config --global core.editor "nano"
Additionally, it’s useful to enable color output in Git commands, making it easier to read the output. This can be done by running:
git config --global color.ui auto
With these configurations, your Bash environment is now primed for Git usage. You can verify all your settings by executing:
git config --list
The output will display all the configurations you’ve set, which will allow you to ensure everything is in order.
As you work with Git, consider creating aliases for frequently used commands to streamline your workflow. For example, you can create an alias for git status for quicker access:
git config --global alias.st status
Now, instead of typing git status, you can simply type:
git st
By following these steps, you’ll establish a robust foundation for using Git within your Bash environment, setting the stage for effective version control and collaboration on your projects.
Common Git Commands and Their Bash Equivalents
Having set up your Bash environment for Git, it’s time to delve into some common Git commands and their Bash equivalents. Knowing how to execute these commands efficiently in the command line will enhance your productivity and streamline your workflows.
Let’s start with the git init command, which initializes a new Git repository in your current directory. Here’s how you would execute it:
git init
This command creates a new subdirectory named .git that contains all the necessary files for version control. It is the first step for turning a regular directory into a Git repository.
Next, you’ll often want to add files to your staging area before committing them. That is done using the git add command. You can add a specific file:
git add filename.txt
Or to add all changed files in the current directory, you can simply use:
git add .
Once the files are staged, the next step is to create a commit. That is accomplished using the git commit command. You can include a message describing the changes with the -m option:
git commit -m "Your commit message here"
To check the current status of your Git repository, the git status command provides a summary of changes made, staged files, and untracked files:
git status
When looking to view the commit history, the git log command is indispensable. It displays all the commits made in the current branch:
git log
If you want a concise view of your commit history, you can apply the –oneline option:
git log --oneline
As you progress with your project, you might need to create branches to work on different features or fixes. The git branch command lists existing branches, while the git checkout command lets you switch between them:
git branch
git checkout branch-name
Alternatively, you can create and switch to a new branch in one step using:
git checkout -b new-branch-name
When you are ready to merge changes from one branch into another, the git merge command is what you need. To merge changes from feature-branch into your current branch:
git add filename.txt
0
Finally, if you need to share your changes with others, the git push command uploads your local commits to a remote repository:
git add filename.txt
1
Conversely, to fetch changes from a remote repository, you can use:
git add filename.txt
2
These are just a few of the essential Git commands that you’ll frequently use in conjunction with Bash. Mastering these will not only enhance your efficiency but also give you a better understanding of version control processes.
Automating Git Tasks with Bash Scripts
Automating tasks in Git using Bash scripts can greatly enhance your productivity by enabling you to perform repetitive actions with a single command. That is especially useful in scenarios where you have a series of Git commands that you run frequently. By encapsulating these commands in a script, you can save time and minimize the risk of human error.
To get started, you need to create a new Bash script file. You can do this using the touch command followed by the name of your script, such as git-automation.sh
:
touch git-automation.sh
Next, open the script in your preferred text editor. If you are using Nano, you can start editing the file by running:
nano git-automation.sh
At the top of your script, you should specify the shell that will interpret the script. This is done with the shebang line:
#!/bin/bash
Now, let’s say you often find yourself creating a new branch, switching to it, and then pushing it to the remote repository. You can automate this sequence of commands. Below is an example of how to do this within your script:
#!/bin/bash # Check if the branch name is supplied if [ -z "$1" ]; then echo "Please provide a branch name." exit 1 fi # Create a new branch git checkout -b "$1" # Push the new branch to the remote repository git push -u origin "$1"
In this script, we first check if a branch name was provided as an argument. If not, the script exits with a message. If a name is given, it creates a new branch using git checkout -b
and then pushes that branch to the remote repository with git push -u origin
.
To make your script executable, you need to change its permissions. Use the following command:
chmod +x git-automation.sh
Now you can run your script from the command line by providing the branch name as an argument:
./git-automation.sh new-feature-branch
This will create a new branch called new-feature-branch
, switch to it, and push it to your remote repository, all with a single command.
Another example of automation could involve batch processing files within a repository. Suppose you want to add all files, commit them with a message, and push changes. You could create a script like this:
#!/bin/bash # Check if a commit message is supplied if [ -z "$1" ]; then echo "Please provide a commit message." exit 1 fi # Add all changes git add . # Commit the changes git commit -m "$1" # Push to the remote repository git push
In this script, we again verify if a commit message was supplied. If it is, we add all changes, commit them, and push them to the remote repository in one command.
To execute this script, use the following command, passing your commit message:
./git-automation.sh "Your commit message here"
Automating Git tasks with Bash scripts not only saves time but also ensures that your commands are executed consistently, minimizing the potential for errors in your workflow. As you become more comfortable with scripting, you can create increasingly complex scripts that cater to your specific development needs.
Troubleshooting Git Issues Using Bash
Troubleshooting Git issues using Bash can be an invaluable skill for developers looking to maintain a smooth workflow. Whether you’re encountering problems with commits, merges, or repository states, using Bash commands can help diagnose and fix these issues efficiently. Here, we’ll explore some common troubleshooting scenarios and illustrate how Bash can be used effectively to resolve them.
One of the first steps in troubleshooting Git issues is to understand the current state of your repository. The git status
command provides a comprehensive overview, indicating which files are staged, which are modified, and which are untracked. This information is essential for identifying where things might have gone awry.
git status
If you encounter merge conflicts, the output of git status
will also indicate which files are in conflict. Bash can be used to view these files quickly. By using the ls
command in conjunction with grep
, you can filter for files with conflict markers.
ls | grep -E '.orig$'
Once you’ve identified the conflicting files, you can open them in your preferred text editor to resolve the issues. Once resolved, you can stage the changes using:
git add filename.txt
After staging the resolved files, commit the changes. This process clears the conflict and finalizes your merge:
git commit -m "Resolved merge conflict"
Another common issue arises from committing changes to the wrong branch. If you realize that you’ve made commits intended for a different branch, you can use git reflog
to view a log of your actions and find the commit hash of the misplaced commit.
git reflog
Once you identify the correct commit, you can cherry-pick it onto the intended branch:
git checkout target-branch
git cherry-pick commit-hash
If you find yourself facing an uncommitted change that you want to discard, you can reset your working directory with:
git checkout -- filename.txt
For more extensive changes that need to be undone, git reset
can be utilized. Using git reset --hard
will revert your entire working directory to the last commit, so proceed with caution:
git reset --hard
In situations where you are not sure about the changes made in your commits, you can view a difference between the current state and the last commit using:
git diff
Should you wish to review the differences between two branches, you can do so with:
ls | grep -E '.orig$'
0
Finally, if you ever need to recover lost commits after a rebase or reset, the git reflog
command becomes your best friend again. It keeps a track of all the changes made to your HEAD, allowing you to restore commits that might seem permanently lost.
Using Bash to troubleshoot Git issues provides you with a powerful toolkit to diagnose and resolve problems efficiently. Understanding the commands available and how to combine them effectively can make a significant difference in maintaining your workflow and repository health.
The article provides a solid foundation for using Git with Bash, but it misses a discussion on error handling and logging within scripts. When automating Git tasks, incorporating error checks after each command can prevent cascading failures. For example, after a `git push`, you could add a check to confirm that the push was successful before proceeding. Additionally, capturing logs of script executions can be invaluable for troubleshooting. Using `echo` statements or redirecting outputs to a log file can provide insights into the script’s execution flow and any issues that arise, enhancing the overall robustness of automated processes in Git.