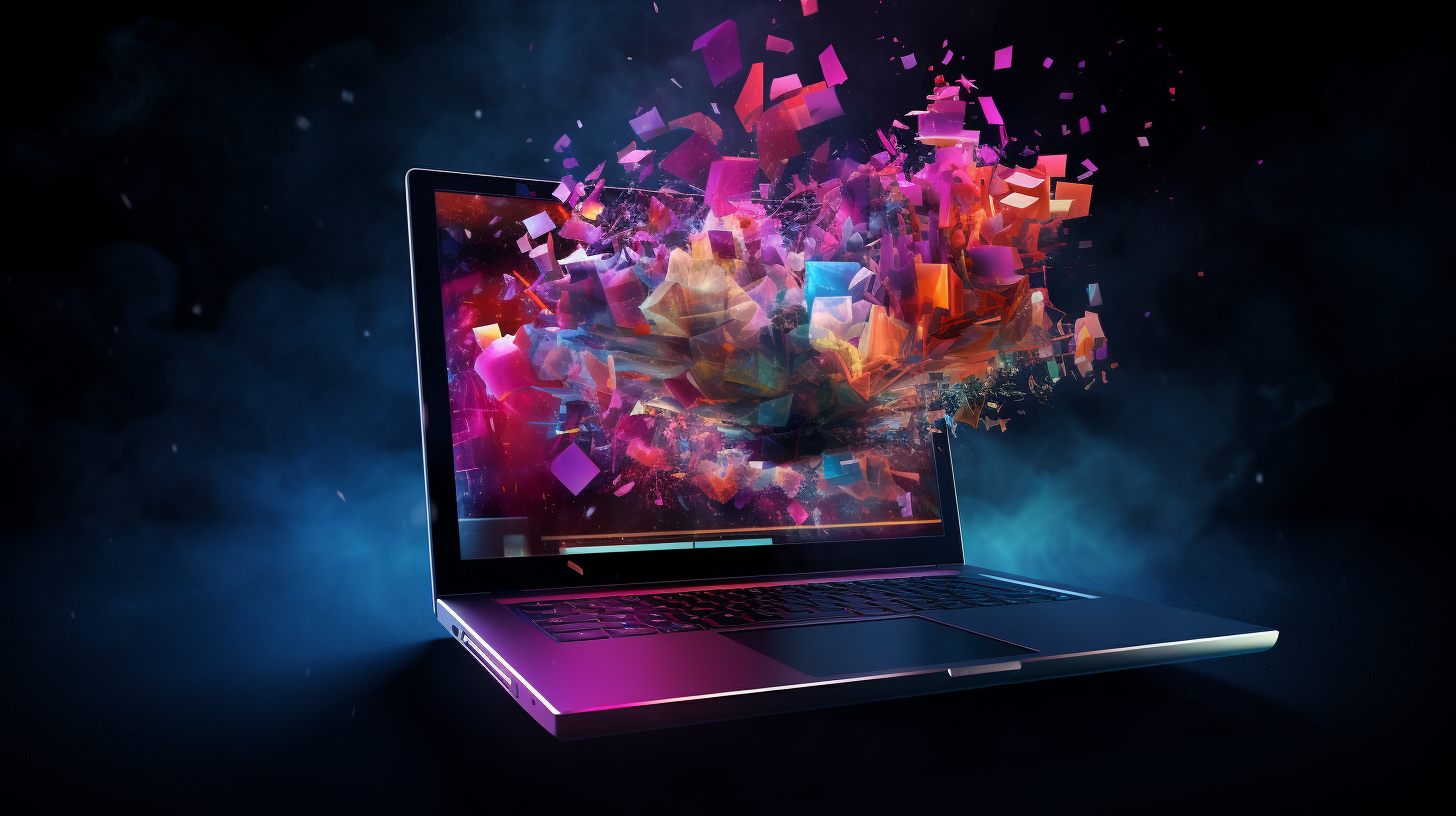
Bash and JSON – Parsing and Creating
When you are working with JSON in Bash, using the right tools can drastically simplify your tasks. There are several libraries and command-line utilities designed to handle JSON data effectively. One of the more popular tools is jq, a powerful command-line JSON processor that allows you to slice, filter, map, and transform structured data simply.
Another key utility is json.sh, a lightweight shell script that converts JSON to shell variables and back. It’s particularly useful when you want to operate directly with JSON data in your shell scripts without the complexity of a full parser.
For those who prefer to use native Bash without external dependencies, there are ways to manipulate JSON using built-in tools like grep, awk, and sed. However, this method can get cumbersome and is generally error-prone when handling complex JSON structures.
Here’s how you can install jq on various systems:
# On Debian/Ubuntu sudo apt-get install jq # On macOS using Homebrew brew install jq # On CentOS/RHEL sudo yum install jq
Once installed, jq can be utilized to parse and transform JSON data with ease. Here’s a simple example demonstrating how to extract a specific value from a JSON object:
json_data='{"name": "John", "age": 30}' echo $json_data | jq '.name'
This command will output:
"John"
Additionally, json.sh can be employed to convert JSON data into a Bash-friendly format. First, ensure you have json.sh saved as a script:
#!/bin/bash # Save this as json.sh and make it executable # Usage: ./json.sh < json_file
Then you can run it like so:
cat data.json | ./json.sh
This will parse the JSON content and provide output that can be used as shell variables. While these tools streamline the process, always consider the complexity of your JSON data and choose the right approach based on your specific requirements.
Parsing JSON with jq
Parsing JSON with jq is not just straightforward; it’s an art that allows a deeper interaction with complex data structures. The syntax is designed to make the extraction of information crisp and intuitive. With jq, you can traverse JSON objects and arrays, applying filters and transformations as needed.
Ponder a JSON file containing user information:
{ "users": [ { "id": 1, "name": "Alice", "age": 28, "email": "[email protected]" }, { "id": 2, "name": "Bob", "age": 34, "email": "[email protected]" } ] }
To extract all user names from this JSON structure, you could use the following jq command:
cat users.json | jq '.users[].name'
This would yield:
"Alice" "Bob"
jq also supports more complex queries. For instance, if you wanted to filter users based on age, you could execute:
cat users.json | jq '.users[] | select(.age > 30) | .name'
The output for this command would be:
"Bob"
In addition to filtering, jq allows you to shape your output in various ways. If you want to create a new JSON object that includes only the names and emails of users, you could do:
cat users.json | jq '{users: [.users[] | {name: .name, email: .email}]}')
The result would be neatly structured:
{ "users": [ { "name": "Alice", "email": "[email protected]" }, { "name": "Bob", "email": "[email protected]" } ] }
It’s important to note that jq can also handle nested structures. For example, if your JSON had nested objects, you could access elements using the dot notation. If we had a JSON structure like this:
{ "users": [ { "id": 1, "info": { "name": "Alice", "age": 28 } }, { "id": 2, "info": { "name": "Bob", "age": 34 } } ] }
You could retrieve Alice’s age using:
cat users.json | jq '.users[0].info.age'
This would output:
28
jq offers a multitude of features, including the ability to sort arrays, map values, and even perform arithmetic operations. Each of these capabilities enhances your ability to work seamlessly with JSON data. By mastering jq, you not only optimize your data processing tasks but also empower your scripting with a level of finesse that can transform mundane data interactions into elegant solutions.
Creating JSON Data in Bash
Creating JSON data in Bash can be just as simpler as parsing it, especially with tools like jq. This utility allows you to construct JSON objects programmatically, facilitating dynamic data generation right from your shell scripts. The syntax for creating JSON structures is intuitive, making it accessible for even those who may not be deeply familiar with JSON.
To begin crafting a JSON object, you can use jq’s `–null-input` option, which permits you to create JSON data from scratch without needing to read from a file. For example, if you wanted to create a simple JSON object representing a person, you could use the following command:
jq -n '{"name": "John", "age": 30}'
This command will output:
{"name":"John","age":30}
For more complex structures, such as arrays or nested objects, jq allows you to build those seamlessly as well. For instance, if you want to create a JSON object that includes a list of users, you might do the following:
jq -n '{ "users": [ {"name": "Alice", "age": 28}, {"name": "Bob", "age": 34} ] }'
The output will be formatted nicely as:
{ "users": [ { "name": "Alice", "age": 28 }, { "name": "Bob", "age": 34 } ] }
Creating JSON data dynamically can also involve incorporating variables from your Bash script. This means you can generate JSON based on runtime data. For example:
name="Charlie" age=25 jq -n --arg name "$name" --argjson age "$age" '{"name": $name, "age": $age}'
This will yield:
{"name":"Charlie","age":25}
Moreover, if you need to build more intricate JSON structures with nested objects and arrays, you can continue to use the `–arg` and `–argjson` options to insert Bash variables into your JSON structure efficiently. Consider a scenario where you want to include hobbies in the user object:
hobbies='["reading", "cycling"]' jq -n --arg name "Charlie" --argjson age 25 --argjson hobbies "$hobbies" '{ "name": $name, "age": $age, "hobbies": $hobbies }'
The output will be:
{ "name": "Charlie", "age": 25, "hobbies": [ "reading", "cycling" ] }
By using jq, Bash not only becomes a powerful tool for data parsing, but it also allows you to conveniently create well-structured JSON data. The combination of variable manipulation and JSON generation opens up a realm of possibilities for scripting, enabling you to build complex data-driven applications directly from the command line.
Handling JSON Arrays and Objects
When handling JSON arrays and objects in Bash, it’s crucial to understand how to navigate their structure effectively. JSON arrays are essentially ordered lists, while objects are unordered collections of key-value pairs. Both types can be manipulated using jq, which provides a robust way to parse and create JSON data.
Imagine you have a JSON structure containing a list of products, each with attributes, including name, price, and tags. Here’s how such a structure looks:
{ "products": [ { "name": "Laptop", "price": 999.99, "tags": ["electronics", "computers"] }, { "name": "Smartphone", "price": 499.99, "tags": ["electronics", "mobile"] } ] }
With jq, you can extract specific details from this JSON array. For example, if you want to retrieve all product names, you could execute the following command:
cat products.json | jq '.products[].name'
This will yield:
"Laptop" "Smartphone"
To extract more complex data, such as the names of products that are tagged as “electronics”, you can use jq’s filtering capabilities:
cat products.json | jq '.products[] | select(.tags | index("electronics")) | .name'
The output will be:
"Laptop" "Smartphone"
When it comes to JSON objects, accessing an object’s properties is done using dot notation. For instance, to get the price of the Laptop, you would run:
cat products.json | jq '.products[0].price'
This command will produce:
999.99
Creating JSON arrays and objects can also be done on the fly. If you want to create a new JSON object representing a product, you can build it using jq as follows:
jq -n '{"name": "Tablet", "price": 299.99, "tags": ["electronics", "mobile"]}'
This outputs:
{"name":"Tablet","price":299.99,"tags":["electronics","mobile"]}
Furthermore, if you need to assemble an array of products dynamically, you can do so using jq’s array construction syntax:
jq -n '[{"name": "Laptop", "price": 999.99}, {"name": "Smartphone", "price": 499.99}]'
The result will be:
cat products.json | jq '.products[].name'
0
Handling JSON arrays and objects in Bash becomes a seamless experience when using jq. It empowers you to traverse, filter, and create structured data with precision and ease, which is invaluable in modern scripting environments. Whether you’re extracting specific fields or building complex structures, mastering these skills allows you to manipulate JSON data efficiently and effectively.
Common Pitfalls and Troubleshooting
When dealing with JSON in Bash, even seasoned scripters can occasionally stumble upon pitfalls that can lead to frustration and inefficiency. Understanding these common issues can dramatically improve your workflow and enhance the reliability of your scripts.
One of the primary pitfalls when parsing JSON is not accounting for the intricacies of JSON syntax. For instance, JSON keys and string values must always be enclosed in double quotes. If you mistakenly use single quotes or omit them altogether, you’ll encounter parsing errors. Here’s an example of a common mistake:
# Incorrect JSON syntax json_data='{name: "John", age: 30}' echo $json_data | jq '.name'
The above command will result in an error because the key name
is not properly enclosed in double quotes. The correct JSON syntax should look like this:
# Correct JSON syntax json_data='{"name": "John", "age": 30}' echo $json_data | jq '.name'
Another common issue arises from the misinterpretation of JSON data structures. JSON can include nested objects and arrays, and navigating these structures can be tricky. It’s crucial to use the correct jq syntax to access these nested elements. For example, if you have a JSON object like the following:
json_data='{"user": {"name": "Alice", "age": 28, "address": {"city": "Wonderland", "zip": "12345"}}}'
To access the city within the address object, you would need to use:
echo $json_data | jq '.user.address.city'
Failing to understand the structure can lead to errors where no output is produced or, worse, the wrong data is retrieved.
Moreover, when handling JSON arrays, it is essential to remember that indexing starts at zero. This might not always be intuitive, especially for those used to different data structures. For instance, if you want to access the first element of an array:
json_data='{"users": [{"name": "Alice"}, {"name": "Bob"}]}' echo $json_data | jq '.users[0].name'
This will correctly output "Alice"
. However, trying to access an out-of-bounds index will lead to nothing being returned, which can be confusing.
Another frequent challenge is dealing with special characters in JSON data, such as quotes or backslashes. If your data includes these characters, they must be properly escaped. For example:
json_data='{"quote": "He said, "Hello, World!""}' echo $json_data | jq '.quote'
Not escaping the double quotes will break your JSON syntax, leading to errors when parsing.
Finally, when it comes to creating JSON data, be cautious of the types of data you’re inserting. jq distinguishes between strings and numbers, and incorrect types can result in misleading or erroneous output. For example:
# Correctly using string jq -n '{name: "Charlie", age: 25}' # Incorrectly mixing types jq -n '{name: "Charlie", age: "25"}'
In the latter case, the age is treated as a string rather than a number, which could lead to unexpected behavior if you’re performing arithmetic operations later.
By being aware of these common pitfalls and troubleshooting techniques, you can avoid many of the headaches associated with JSON processing in Bash. Mastery of jq’s capabilities combined with an understanding of JSON syntax will equip you to handle JSON data with confidence and precision.