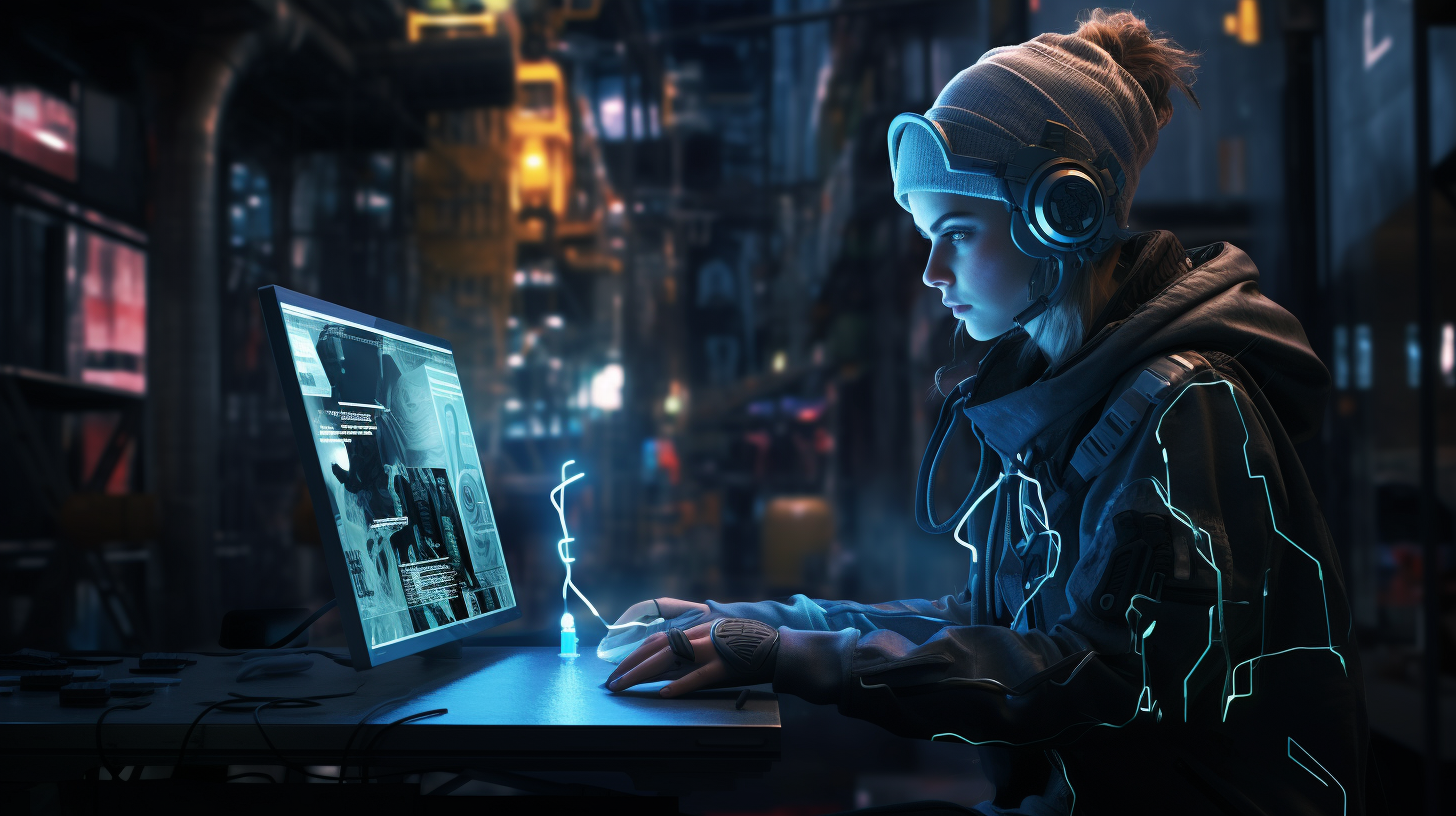
Bash and Kubernetes Integration
Kubernetes is a powerful platform designed for managing containerized applications across a cluster of machines. Understanding its architecture very important for effectively using its capabilities with Bash scripts. At its core, Kubernetes employs a master-slave architecture divided into several key components.
The Master Node is the control plane that manages the Kubernetes cluster. It’s responsible for maintaining the desired state of the cluster, scheduling applications, and managing workloads. The master node consists of several components:
- The API server is the front end of the Kubernetes control plane. It exposes the Kubernetes API and processes REST requests, serving as the main access point for all the communication between components.
- This component manages controllers that regulate the state of the system, ensuring that the desired state of applications is maintained. Each controller watches the cluster state and makes necessary adjustments.
- The scheduler is responsible for placing pods onto the appropriate nodes based on resource availability and defined constraints.
- That’s a distributed key-value store used for persistent data storage. etcd holds all the configuration data and the state of the Kubernetes cluster.
On the other side, we have the Worker Nodes, which run the applications. Each worker node includes:
- An agent that runs on each worker node, the Kubelet ensures that containers are running in pods as per the specifications in the API server.
- That’s responsible for running the containers. Kubernetes supports several container runtimes, including Docker, containerd, and CRI-O.
- This component maintains network rules on nodes, allowing network communication to your pods from inside or outside the cluster.
Understanding how these components interact is fundamental for developing effective Bash scripts to manage Kubernetes resources. For instance, using the Kubernetes API, you can automate tasks such as scaling deployments or retrieving the status of services.
Here’s a simple example of how you can use Bash to interact with the Kubernetes API to list all pods in the current namespace:
#!/bin/bash # List all pods in the current namespace kubectl get pods --namespace=$(kubectl config view --minify -o jsonpath='{.context.namespace}')
This command leverages the kubectl
command-line tool, which communicates with the Kubernetes API server. The output provides a quick overview of the running pods, essential for monitoring application health and performance.
As you delve deeper into Bash scripting for Kubernetes management, grasping the architectural layout will significantly enhance your scripts’ effectiveness and your understanding of Kubernetes operations.
Bash Scripting for Kubernetes Management
Bash scripting is a powerful tool for managing Kubernetes resources, allowing for automation and efficiency in operations. With Bash, you can create scripts that interact with the Kubernetes API, automate repetitive tasks, and manage clusters effectively.
To harness the power of Bash with Kubernetes, it’s vital to become familiar with the kubectl command-line interface (CLI). This tool serves as a bridge between your Bash scripts and the Kubernetes API. Below, we’ll explore some fundamental operations you can automate using Bash, which streamline the workflow for both developers and system administrators.
One common task is to create a new deployment. The following Bash script demonstrates how to create a deployment using a specified container image:
#!/bin/bash # Create a new deployment DEPLOYMENT_NAME="my-deployment" IMAGE="nginx:latest" NAMESPACE="default" kubectl create deployment $DEPLOYMENT_NAME --image=$IMAGE --namespace=$NAMESPACE
In this script, we define the deployment name, the container image to be used, and the namespace where the deployment will reside. The kubectl create deployment command is then invoked to create the deployment in the specified namespace.
Another crucial aspect of Kubernetes management is monitoring the status of deployments. You can write a script that checks the health of your deployments and takes action based on their status:
#!/bin/bash # Check the status of a deployment DEPLOYMENT_NAME="my-deployment" NAMESPACE="default" STATUS=$(kubectl get deployment $DEPLOYMENT_NAME --namespace=$NAMESPACE -o jsonpath='{.status.conditions[?(@.type=="Available")].status}') if [ "$STATUS" == "True" ]; then echo "Deployment $DEPLOYMENT_NAME is available." else echo "Deployment $DEPLOYMENT_NAME is not available." fi
This script retrieves the status of the specified deployment and checks whether it’s available. Depending on the result, it echoes an appropriate message. Such scripts are invaluable for maintaining the health of your applications and ensuring uptime.
Scaling deployments is another area where Bash scripts prove beneficial. By using parameters, you can dynamically adjust the number of replicas in your deployments:
#!/bin/bash # Scale a deployment DEPLOYMENT_NAME="my-deployment" REPLICAS=3 NAMESPACE="default" kubectl scale deployment $DEPLOYMENT_NAME --replicas=$REPLICAS --namespace=$NAMESPACE
In this script, you can easily modify the REPLICAS variable to scale your application up or down, demonstrating the flexibility that Bash provides for managing Kubernetes resources efficiently.
Additionally, Bash can be utilized to clean up resources that are no longer needed. The following script deletes a specified deployment:
#!/bin/bash # Delete a deployment DEPLOYMENT_NAME="my-deployment" NAMESPACE="default" kubectl delete deployment $DEPLOYMENT_NAME --namespace=$NAMESPACE
This simplicity ensures that your Kubernetes environment remains organized and free of clutter, which is important for optimal resource management.
As you can see, Bash scripting offers a versatile approach to Kubernetes management, allowing for flexibility, automation, and control. By understanding how to leverage these scripts in conjunction with Kubernetes, you can enhance your operational efficiency and streamline your workflows, making your interactions with containerized applications smoother and more effective.
Automating Deployments with Bash
Bash scripts not only help in managing Kubernetes resources but also enable you to automate complex deployment workflows. Automating deployments with Bash can significantly reduce the overhead associated with manual operations and ensure consistency in deployment processes. The power lies in the ability to combine various kubectl commands within your scripts, allowing for a streamlined, repeatable, and error-reduced deployment strategy.
Consider a scenario where you need to deploy a multi-container application. You can automate the entire process, from creating the necessary deployments to applying specific configurations, all with a single Bash script. Below is an example of how you might structure such a script:
#!/bin/bash # Automate multi-container deployment NAMESPACE="my-app" DEPLOYMENT_NAME="my-multi-container-deployment" # Create a new namespace if it doesn't exist kubectl create namespace $NAMESPACE --dry-run=client -o yaml | kubectl apply -f - # Create the deployment with multiple containers kubectl create deployment $DEPLOYMENT_NAME --namespace=$NAMESPACE --image=app_image:latest --replicas=2 # Add a second container to the deployment kubectl set image deployment/$DEPLOYMENT_NAME app-container=app_image:latest db-container=db_image:latest --namespace=$NAMESPACE
In the above script, we first check for the existence of a namespace and create it if it does not exist. We then create a deployment with a specified container image and set the number of replicas. The kubectl set image
command is employed to add a second container to the deployment, demonstrating how you can extend and modify existing deployments seamlessly.
Moreover, in complex applications, you might also need to apply configuration files and secrets. Bash can help in templating these files based on environment variables, ensuring that each deployment is tailored to its environment. Here’s an example of how you might apply a configuration file to your deployment:
#!/bin/bash # Apply configuration from a YAML file CONFIG_FILE="config.yaml" NAMESPACE="my-app" # Ensure the namespace exists kubectl create namespace $NAMESPACE --dry-run=client -o yaml | kubectl apply -f - # Apply the configuration kubectl apply -f $CONFIG_FILE --namespace=$NAMESPACE
This script applies a configuration file to the specified namespace, showing how you can manage external configurations dynamically. Such flexibility is essential when managing different environments, such as staging or production.
Additionally, enhancing your Bash scripts with error handling can lead to more robust deployments. It’s essential to ensure that each step of your deployment process completes successfully before proceeding. Here’s how you can implement error handling in your deployment scripts:
#!/bin/bash # Deployment script with error handling set -e # Exit immediately if a command exits with a non-zero status NAMESPACE="my-app" DEPLOYMENT_NAME="my-deployment" # Create a namespace kubectl create namespace $NAMESPACE --dry-run=client -o yaml | kubectl apply -f - # Create the deployment kubectl create deployment $DEPLOYMENT_NAME --image=nginx:latest --namespace=$NAMESPACE # Check deployment status if ! kubectl rollout status deployment/$DEPLOYMENT_NAME --namespace=$NAMESPACE; then echo "Deployment failed. Please check the logs." exit 1 fi echo "Deployment $DEPLOYMENT_NAME created successfully."
In this example, the set -e
command ensures that the script terminates upon encountering an error, and the rollout status check confirms that the deployment was successful. Such measures are crucial for preventing partial deployments that may lead to inconsistent application states.
Ultimately, the power of automating deployments with Bash lies in its simplicity and versatility. By integrating Bash scripts with Kubernetes operations, you can create efficient, repeatable processes that enhance your deployment strategies and improve overall productivity in managing containerized applications.
Integrating Bash with Kubernetes CLI Tools
Integrating Bash with Kubernetes CLI tools is a game changer for developers and operators alike. The kubectl command-line tool is a potent interface for interacting with Kubernetes clusters, and using it within Bash scripts opens up a world of automation possibilities. With Bash, you can create scripts that not only simplify repeated tasks but also enhance operational workflows by integrating various Kubernetes operations seamlessly.
One of the most common ways to utilize Bash in conjunction with kubectl is through chaining commands and using command substitution. This allows you to create dynamic and responsive scripts based on the current state of your Kubernetes cluster. For example, consider a scenario where you want to deploy an application only if no other deployment is currently running. A Bash script could check for existing deployments before proceeding:
#!/bin/bash # Check for existing deployments before creating a new one DEPLOYMENT_NAME="my-app" NAMESPACE="default" if kubectl get deployments --namespace=$NAMESPACE | grep -q $DEPLOYMENT_NAME; then echo "Deployment $DEPLOYMENT_NAME already exists in $NAMESPACE." else kubectl create deployment $DEPLOYMENT_NAME --image=nginx:latest --namespace=$NAMESPACE echo "Deployment $DEPLOYMENT_NAME created successfully." fi
This script uses grep to check the existence of a deployment before attempting to create it, providing a safeguard against conflicts. Such checks can prevent deployment failures and maintain resource integrity within your cluster.
Another powerful feature of integrating Bash with kubectl is the ability to process and manipulate JSON or YAML outputs directly in your scripts. Kubernetes resources typically provide detailed information in these formats, and you can use tools like jq or yq to parse this data effectively. For instance, if you want to retrieve the IP address of a running pod, you could use the following Bash script:
#!/bin/bash # Retrieve the IP address of a running pod POD_NAME="my-pod" NAMESPACE="default" POD_IP=$(kubectl get pod $POD_NAME --namespace=$NAMESPACE -o json | jq -r '.status.podIP') if [ -n "$POD_IP" ]; then echo "The IP address of pod $POD_NAME is $POD_IP." else echo "Pod $POD_NAME not found or not running." fi
In this script, jq is harnessed to extract the pod’s IP address from the JSON output provided by kubectl. This capability enables scripting solutions that can react dynamically to the current state of resources, making your management tasks more efficient and responsive.
Furthermore, environment variables can be employed to improve the reusability of your scripts. By defining parameters such as the namespace or image name at the top of your script, you can quickly adjust their values without modifying your entire script. Here’s how you might set this up:
#!/bin/bash # Deploy an application using environment variables NAMESPACE="default" DEPLOYMENT_NAME="my-app" IMAGE="nginx:latest" kubectl create deployment $DEPLOYMENT_NAME --image=$IMAGE --namespace=$NAMESPACE
This approach encourages clean and maintainable code, enabling reuse across different environments or applications with minimal effort.
To further streamline operations, you can create wrapper scripts that aggregate multiple kubectl commands into cohesive workflows. For example, a script that sets up a complete environment by creating a namespace, deploying applications, and exposing services might look as follows:
#!/bin/bash # Setup a Kubernetes environment NAMESPACE="my-app" # Create namespace kubectl create namespace $NAMESPACE --dry-run=client -o yaml | kubectl apply -f - # Deploy the application kubectl create deployment my-app --image=nginx:latest --namespace=$NAMESPACE # Expose the application kubectl expose deployment my-app --type=LoadBalancer --port=80 --namespace=$NAMESPACE
This script methodically creates a namespace, deploys an application, and exposes it to external traffic—all with a single execution. Such comprehensive scripts reduce the risk of human error and ensure that environments are consistently set up.
By integrating Bash with Kubernetes CLI tools, you gain the ability to automate and streamline your workflows, making it easier to manage your containerized applications effectively. The combination of Bash scripting with kubectl empowers developers and operators to improve productivity, minimize errors, and maintain robust Kubernetes environments with confidence.