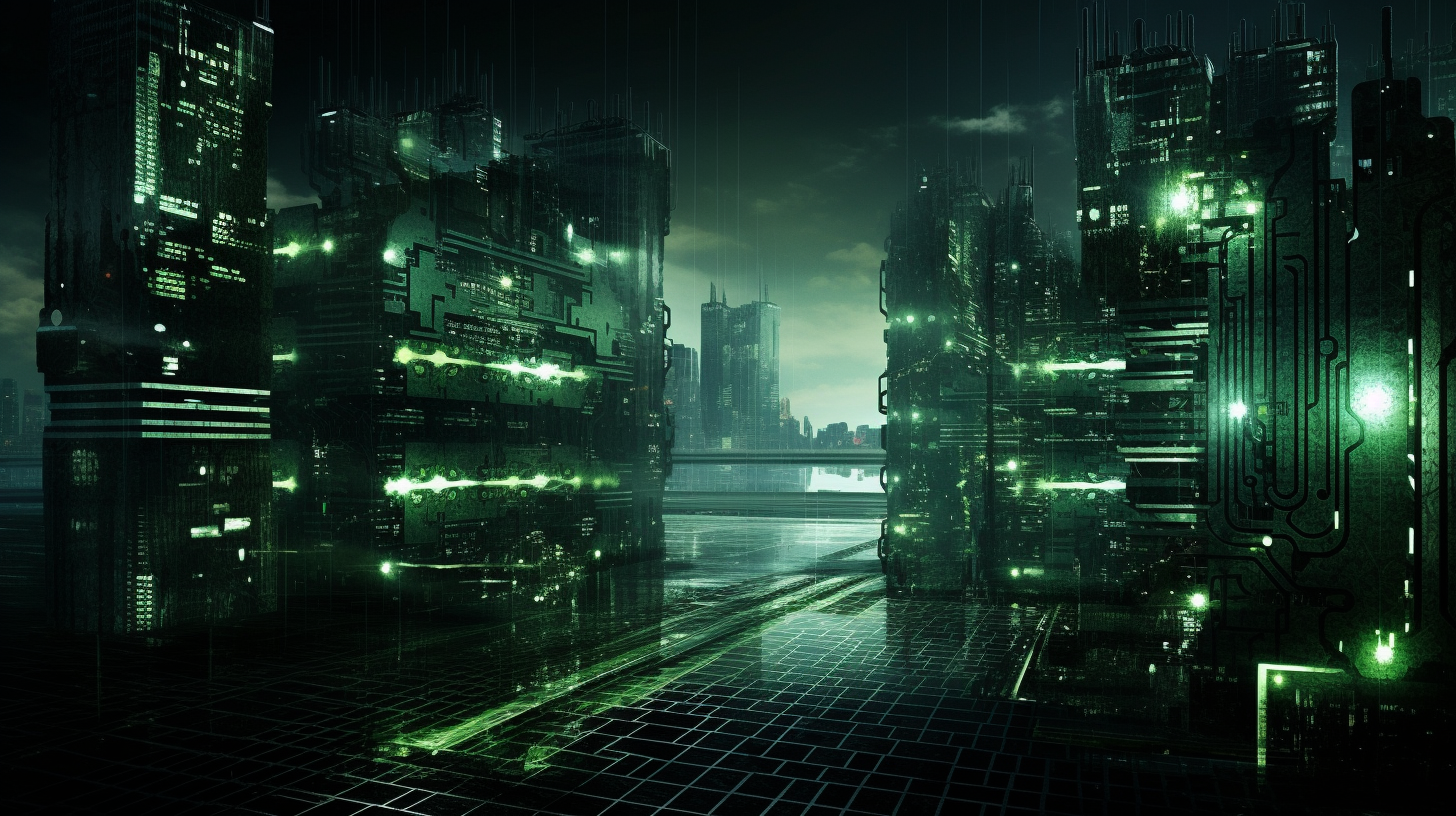
Bash and Machine Learning – Basic Scripts
When diving into the world of Bash scripting, it is essential to grasp its foundational concepts. Bash, or Bourne Again SHell, is a Unix shell that provides a command-line interface for interacting with the operating system. At its core, Bash scripting is about automating tasks by writing sequences of commands that the shell can execute.
Variables are a fundamental part of Bash scripting. They allow you to store data that can be used throughout your script. To declare a variable, you simply assign a value without spaces around the equal sign. Here’s a simple example:
name="Machine Learning" echo "Welcome to $name!"
In this snippet, we declare a variable called name
and assign it a string. The echo
command then uses that variable to output a greeting.
Another important concept is control structures, which help in making decisions within your scripts. The most basic of these is the if
statement. Here’s how you can use it to check for a condition:
number=10 if [ $number -gt 5 ]; then echo "$number is greater than 5" else echo "$number is not greater than 5" fi
This example checks if the variable number
is greater than 5 and echoes a message based on the condition.
Loops are another key component in Bash scripting, so that you can execute a block of code multiple times. The for loop is particularly useful when you want to iterate over a list of items:
for i in {1..5}; do echo "Iteration $i" done
In this case, the loop prints the current iteration number from 1 to 5. This can be incredibly useful for processing batches of files or data entries.
Finally, functions in Bash provide a way to encapsulate code for reuse. Defining a function is straightforward:
function greet { local name=$1 echo "Hello, $name!" } greet "Bash User"
This function, greet
, takes a parameter and prints a greeting. By using functions, you can make your scripts cleaner and more modular.
Understanding these basics of Bash scripting especially important for anyone looking to leverage the power of the shell in the context of machine learning. With these foundational tools, you can start automating mundane tasks, preprocessing data, and integrating other tools effectively.
Setting Up Your Bash Environment
Before you can dive into the realm of Bash scripting, it is vital to establish a well-configured Bash environment. This setup ensures that you can run your scripts smoothly and make the most out of the features Bash has to offer. Here’s how to get started.
First and foremost, you need to have a terminal emulator installed on your machine. Most Unix-like systems come with Bash pre-installed, but if you are on Windows, you might want to use Windows Subsystem for Linux (WSL) or a terminal emulator like Git Bash or Cygwin.
Once you have your terminal ready, it is important to familiarize yourself with some key Bash commands that will be the backbone of your scripting. You can start by checking your Bash version with:
bash --version
This command will provide you with the version number of Bash currently installed, which is useful to know since some features may vary between versions.
Next, ensure that your environment variables are set correctly. These variables control the behavior of your shell and can be configured in the .bashrc or .bash_profile files located in your home directory. For example, you can set an important variable like PATH to include custom script directories:
export PATH=$PATH:~/my_bash_scripts
This command appends the directory `~/my_bash_scripts` to your existing PATH, enabling you to run scripts located there without needing to specify their entire paths.
Another essential step is to create a dedicated directory for your Bash scripts, keeping your workspace organized. You can create a directory called `bash_scripts` in your home directory with the following command:
mkdir ~/bash_scripts
Once created, you can navigate to this directory using:
cd ~/bash_scripts
Now, you’re ready to create your first Bash script. To do this, open a text editor of your choice. You can use `nano`, `vim`, or any other editor. For instance, to create a script called `hello.sh`, you can run:
nano hello.sh
Within this file, you can start your script with the shebang line that tells the system to use Bash to interpret the script:
#!/bin/bash
After the shebang line, you can add your commands. For example:
#!/bin/bash echo "Hello, World!"
Once you’ve saved your script, you must make it executable. This can be achieved with the chmod command:
chmod +x hello.sh
Finally, you can run your script by typing:
./hello.sh
Setting up your Bash environment is not just about getting the basics right; it’s about creating a structured and efficient workspace that allows you to harness the power of Bash scripting, especially in tasks related to machine learning. With your environment ready, you can start automating tasks and focusing on the more complex aspects of your projects.
Data Preprocessing with Bash Scripts
Data preprocessing is a critical step in any machine learning workflow, and Bash scripting provides powerful tools to handle it efficiently. By using Bash’s capabilities, you can automate the cleaning, transformation, and preparation of data, enabling you to focus on model building and evaluation. Here’s how you can utilize Bash scripts for data preprocessing.
One of the primary tasks in data preprocessing is cleaning your dataset. This often involves handling missing values, removing duplicates, and filtering out irrelevant information. Let’s say you have a CSV file containing some data, and you need to remove any duplicate entries based on a specific column. You can use the awk
command for this purpose:
awk '!seen[$1]++' input.csv > output.csv
In this command, awk
processes the input CSV file, using an associative array to track seen values from the first column (specified by $1
). It writes only the unique entries to output.csv
.
Another common preprocessing task is replacing missing values. Suppose you want to replace any occurrence of “NA” in your dataset with the mean of that column. You can use the following snippet:
#!/bin/bash # Calculate mean of the column (assuming a numeric column in CSV) mean=$(awk -F',' '{if($2!="NA"){sum+=$2; count++}} END {print sum/count}' input.csv) # Replace NA with mean awk -F',' -v mean="$mean" '{gsub("NA", mean, $2)}1' OFS=',' input.csv > output.csv
This script first calculates the mean of the second column, ignoring “NA” entries. Then, it uses gsub
to replace “NA” with the calculated mean, outputting the results to a new CSV file.
Transforming data is also crucial. For instance, you might need to convert categorical variables into a numerical format. The sed
command is useful for this task. Assume you have a column with categorical labels and want to convert “yes” to 1 and “no” to 0:
sed 's/yes/1/g; s/no/0/g' input.csv > output.csv
This command substitutes “yes” with 1 and “no” with 0 throughout the CSV file.
Another essential aspect of data preprocessing is normalizing or standardizing numerical features. If your dataset contains features with different scales, it’s often beneficial to scale them. You can achieve this using a simple Bash script that computes the min-max normalization:
#!/bin/bash # Calculate min and max for a specific column min=$(awk -F',' 'NR>1 {if(NR==2 || $2 1 {if(NR==2 || $2 > max) max=$2} END {print max}' input.csv) # Normalize the column awk -F',' -v min="$min" -v max="$max" '{if(NR>1) $2=($2-min)/(max-min)}1' OFS=',' input.csv > output.csv
This script calculates the minimum and maximum values of the second column and normalizes it, outputting the transformed data to a new CSV file.
Bash scripting allows for a high degree of flexibility in data preprocessing tasks. By chaining commands using pipes and combining various tools, you can create powerful preprocessing pipelines. For more complex tasks, think breaking your script into functions to maintain clarity and reusability:
#!/bin/bash function clean_data { awk '!seen[$1]++' "$1" > temp.csv } function replace_missing { mean=$(awk -F',' '{if($2!="NA"){sum+=$2; count++}} END {print sum/count}' temp.csv) awk -F',' -v mean="$mean" '{gsub("NA", mean, $2)}1' OFS=',' temp.csv > "$2" } clean_data input.csv replace_missing temp.csv output.csv
This structure allows you to easily expand your script with more preprocessing functions as needed. By mastering these Bash scripting techniques, you will streamline your data preprocessing tasks, setting a solid foundation for the subsequent stages of your machine learning projects.
Integrating Bash with Machine Learning Libraries
Integrating Bash scripting with machine learning libraries can significantly enhance your workflow, enabling you to automate processes and manage data more efficiently. Whether you’re working with libraries like TensorFlow, scikit-learn, or PyTorch, you can use Bash to streamline the execution of scripts, manage datasets, and handle output seamlessly. Here’s how to effectively integrate Bash with these powerful machine learning libraries.
One of the most common ways to use Bash with machine learning libraries is by executing Python scripts from within Bash. This allows you to leverage the strengths of both languages—Bash for process automation and Python for machine learning. To run a Python script that utilizes a machine learning library, you can simply call the Python interpreter from your Bash script. For example:
#!/bin/bash # Run Python script for machine learning python3 train_model.py
This script will invoke the training process defined in `train_model.py`. You can also pass arguments to your Python script directly from Bash. Here’s how to do it:
#!/bin/bash # Define hyperparameters learning_rate=0.01 epochs=100 # Run Python script with arguments python3 train_model.py --learning_rate $learning_rate --epochs $epochs
In the above example, the Bash script sets hyperparameters and passes them to the Python script, allowing for dynamic configuration based on the Bash environment.
Another powerful capability is managing datasets directly using Bash commands before feeding them into your machine learning models. For instance, if you want to split your dataset into training and testing sets, you can use a combination of `head` and `tail` commands:
#!/bin/bash # Split dataset into training and testing sets split_ratio=0.8 total_lines=$(wc -l train.csv tail -n +$((train_lines + 1)) dataset.csv > test.csv
This script computes the number of lines in the dataset and splits it into training and testing sets based on the specified ratio. The output files, `train.csv` and `test.csv`, are then ready to be used in your machine learning workflows.
Additionally, you can manage output files and logs effectively through Bash scripting. For example, if you want to save the output of your model training progress to a log file, you can redirect output as follows:
#!/bin/bash # Run model training and save logs python3 train_model.py > training_log.txt 2>&1
This command captures both standard output and error output, saving them into `training_log.txt` for later review, which is invaluable for troubleshooting and debugging.
Furthermore, integrating Bash with tools like `cron` can help automate the scheduling of model training or evaluation tasks. By creating a cron job, you can set up regular intervals at which your scripts run, for example:
# Open crontab for editing crontab -e # Add a line to run the script daily at 2 AM 0 2 * * * /path/to/your_script.sh
This setup ensures your model is retrained daily, allowing for continuous learning with new data, thus enhancing the model’s performance over time.
By mastering the integration of Bash with machine learning libraries, you can create a robust and automated workflow that not only enhances productivity but also allows for more intricate and powerful data manipulation and model management. This synergy between Bash and machine learning can lead to significant advancements in your data science projects.
Automating Machine Learning Workflows
In the sphere of machine learning, automating workflows can save invaluable time and reduce the potential for human error. Bash scripting becomes a powerful ally in orchestrating these workflows, enabling you to chain together various tasks into a cohesive pipeline. Below, we will explore several techniques to automate machine learning workflows using Bash scripts, ensuring that your processes run smoothly and efficiently.
One of the simplest forms of workflow automation in Bash is the creation of a script that encompasses all the necessary steps of your machine learning model training and evaluation. Consider a scenario where you need to preprocess your data, train a model, and then evaluate its performance. You can encapsulate these steps in a single Bash script:
#!/bin/bash # Step 1: Preprocessing data echo "Preprocessing data..." python3 preprocess_data.py --input raw_data.csv --output clean_data.csv # Step 2: Training the model echo "Training model..." python3 train_model.py --data clean_data.csv --output model.pkl # Step 3: Evaluating the model echo "Evaluating model..." python3 evaluate_model.py --model model.pkl --test_data test_data.csv --output evaluation_results.txt echo "Workflow completed!"
This script can be executed with a single command, automating the entire process from data preprocessing through model evaluation. By breaking down the workflow into clear steps and using Python scripts for each task, you create an easy-to-manage automation tool.
Another important aspect of automating machine learning workflows is the management of environments and dependencies. You may want to use virtual environments to isolate your project’s dependencies. A Bash script can help automate the creation of virtual environments, installation of required packages, and execution of your main script:
#!/bin/bash # Step 1: Create a virtual environment echo "Creating virtual environment..." python3 -m venv venv # Step 2: Activate the environment source venv/bin/activate # Step 3: Install dependencies echo "Installing dependencies..." pip install -r requirements.txt # Step 4: Run the main script echo "Running the main script..." python main_script.py # Step 5: Deactivate the environment deactivate echo "Done!"
In this example, the script sets up an isolated environment for your machine learning project, ensuring that all dependencies are installed correctly before running the model. This approach significantly reduces conflicts that may arise from different package versions across projects.
Additionally, logging is essential in any automated workflow, especially when training machine learning models. It can help you track the performance of your model over time. You can enhance your automation by appending timestamps and status messages to log files:
#!/bin/bash log_file="workflow.log" # Function to log messages log() { echo "$(date '+%Y-%m-%d %H:%M:%S') - $1" >> $log_file } log "Starting the workflow..." # Preprocessing log "Preprocessing data..." python3 preprocess_data.py --input raw_data.csv --output clean_data.csv # Training log "Training model..." python3 train_model.py --data clean_data.csv --output model.pkl # Evaluation log "Evaluating model..." python3 evaluate_model.py --model model.pkl --test_data test_data.csv --output evaluation_results.txt log "Workflow completed!"
With the logging function in place, your script will create a comprehensive record of each step, including the date and time of execution. This can be extremely helpful when diagnosing issues or evaluating the effectiveness of changes over time.
Moreover, integrating external scheduling tools like `cron` further enhances your ability to automate workflows. You can set up a cron job to run your entire automation script at specified intervals, so that you can retrain models or update datasets without manual intervention. For example, to run your workflow script every day at midnight, you can add the following line to your crontab:
0 0 * * * /path/to/your_automation_script.sh
This capability ensures your machine learning models are always up-to-date with the latest data, leading to better performance and accuracy over time.
By using Bash scripting for automating machine learning workflows, you empower yourself to focus on more complex tasks such as model tuning and feature engineering. The automation you craft will not only save time but also lead to more robust and reproducible results in your machine learning projects.
Troubleshooting and Debugging Bash Scripts
Debugging and troubleshooting Bash scripts can be a daunting task, especially when these scripts are integral to machine learning workflows that often involve complex data processing. The key to effective debugging lies in understanding how to identify and rectify errors efficiently. Here are several techniques to help you navigate through the challenges of troubleshooting Bash scripts.
First and foremost, using the built-in debugging options available in Bash can significantly aid in identifying issues. One of the simplest methods to start debugging is by using the -x
option when executing your script. This enables a trace of the commands being executed, which will allow you to see the values of variables and the flow of execution:
bash -x your_script.sh
By running your script with this option, you’ll get a detailed output of each command executed, along with its arguments, which can help pinpoint where things go wrong.
Another useful technique is to incorporate error checking within your script. This involves checking the exit status of commands using the $?
variable or using set -e
at the beginning of your script. The set -e
command instructs Bash to immediately exit the script if any command has a non-zero exit status, which indicates an error:
#!/bin/bash set -e # Example command cp file_that_does_not_exist.txt destination_folder/
In this case, if the copy command fails, the script will terminate, and you’ll be notified of the error. This can help you catch issues early on instead of letting them propagate through your script.
Using trap
is another powerful method for handling errors gracefully. You can set traps to catch errors and execute cleanup commands or log messages when an error occurs:
#!/bin/bash trap 'echo "An error occurred. Exiting..."; exit 1;' ERR # Commands here that may fail rm non_existent_file.txt
In this example, if any command results in an error, the script will print a message and exit, so that you can handle the situation without causing further issues downstream.
Proper logging also plays an important role in troubleshooting Bash scripts. Redirecting output and error messages to a log file provides a history of events that transpired during execution. You can accomplish this with output redirection:
#!/bin/bash log_file="script.log" # Redirect stdout and stderr to log file exec > >(tee -i "$log_file") 2>&1 echo "Starting the script..." # Your script logic here
This setup captures both the output and any errors, making it easier to review what happened during the script’s execution.
Moreover, isolating components of your script can simplify the debugging process. Rather than running your entire script, start with smaller sections of code. This allows you to focus on specific functionality without the distraction of other operations. For instance, test individual functions or commands to verify that each part behaves as expected:
# Function to process data function process_data { # Simulate processing echo "Processing data..." # Add potential error here for testing } # Test the function in isolation process_data
Lastly, don’t underestimate the value of community and external resources. If you encounter a particularly perplexing issue, forums like Stack Overflow or dedicated scripting communities can provide insights from others who have likely faced similar challenges.
By using these debugging techniques, you can enhance your proficiency in troubleshooting Bash scripts. This not only streamlines your development process but also ensures that your machine learning workflows remain robust and reliable, allowing you to focus on building and refining your models instead of getting bogged down by script errors.