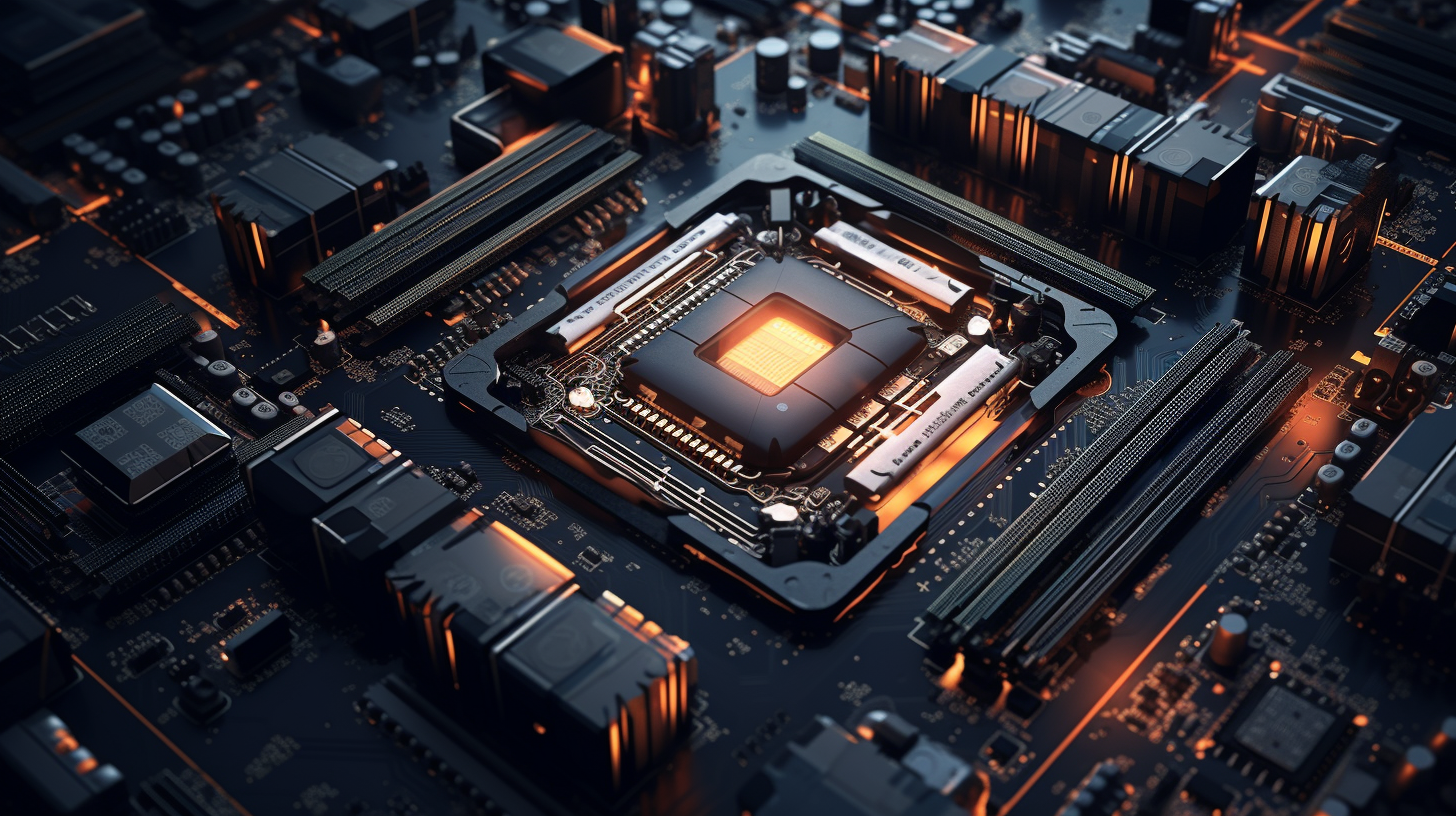
Bash for Beginners – Basic Scripts
The Bash shell is a powerful command-line interface that allows users to interact with the operating system through commands and scripts. It stands for “Bourne Again SHell,” a nod to its predecessor, the Bourne shell. Understanding the Bash shell is essential for anyone looking to harness the full potential of Unix-like environments.
At its core, the Bash shell interprets and executes commands entered by the user or contained within scripts. The shell provides a variety of features, including command history, tab completion, and scripting capabilities, making it a versatile tool for both simple tasks and complex automation.
When you open a terminal window, you are greeted by a command prompt, usually displaying your username, the hostname, and the current directory. Here, you can execute commands such as ls
to list files, cd
to change directories, or echo
to display messages.
One of the most powerful aspects of the Bash shell is its ability to execute scripts. A script is simply a file containing a series of commands that can be run as a single program. To create a Bash script, you start with a shebang line, which tells the system which interpreter to use. The standard shebang for Bash scripts is:
#!/bin/bash
After the shebang, you can write any valid Bash commands in sequence. Here’s a simple example of a Bash script that prints “Hello, World!” to the terminal:
#!/bin/bash echo "Hello, World!"
To execute this script, you must first give it execute permissions using the chmod
command:
chmod +x hello.sh
Then, you can run your script by typing:
./hello.sh
The Bash shell excels at handling various data types, including strings and numbers, and supports a rich set of built-in functions for manipulating these types. Understanding how to work with these types very important for creating effective scripts.
Another important feature of the Bash shell is its support for command-line arguments. When you run a script, you can pass arguments to it, allowing for dynamic input. Within the script, you can access these arguments using special variables like $1
, $2
, and so on, where $1
represents the first argument, $2
the second, and so forth. This allows your scripts to be more flexible and reusable.
The Bash shell is a fundamental tool for anyone working with Linux or Unix-like operating systems. Understanding its features and capabilities opens the door to powerful scripting and automation tasks that can greatly enhance productivity.
Creating Your First Bash Script
Creating your first Bash script is an exciting enter the realm of command-line programming. It allows you to automate tasks that you might otherwise perform manually, saving both time and effort. The process of crafting a script begins with understanding its structure and the essential commands you can incorporate.
As previously mentioned, every Bash script starts with a shebang line:
#!/bin/bash
This line tells the system that the script should be executed using the Bash interpreter. Following the shebang, you will write the commands that you want to run in sequence.
Let’s create a slightly more complex script that greets a user based on their input. This script will prompt for the user’s name and then print a greeting. Here’s how it looks:
#!/bin/bash echo "What is your name?" read name echo "Hello, $name! Welcome to Bash scripting!"
In this example, we use echo
to display messages and read
to capture user input. The variable $name
holds the user’s input, which we then use in the greeting. To execute this script:
chmod +x greet.sh ./greet.sh
When you run this script, it will pause and wait for the user to enter their name. After the user inputs their name and presses Enter, the script continues and displays a personalized message.
Another important aspect of creating Bash scripts is using comments. Comments are crucial for making your scripts understandable, especially when you or someone else revisits them later. In Bash, you can add comments by starting a line with the #
symbol. For example:
#!/bin/bash # This script greets the user echo "What is your name?" read name echo "Hello, $name! Welcome to Bash scripting!"
Comments do not affect the execution of the script, but they serve as an excellent tool for documentation.
As you continue to write scripts, you’ll find that organizing your code into functions can be beneficial. Functions help modularize your script and can be reused throughout your script without duplication of code. Here’s a simple function example:
#!/bin/bash greet_user() { echo "Hello, $1! Welcome to Bash scripting!" } echo "What is your name?" read name greet_user $name
In this script, the greet_user
function takes one argument (the user’s name) and prints a greeting. This makes your script easier to read and maintain.
Creating your first Bash script involves understanding the basic structure, using user input, and effectively using comments and functions. As you gain more experience, these elements will become second nature, so that you can craft powerful scripts that can automate a wide range of tasks.
Variables and Data Types
The ability to handle variables is one of the cornerstones of effective Bash scripting. Variables allow you to store and manipulate data dynamically within your scripts, making them flexible and powerful. In Bash, declaring a variable is straightforward: simply assign a value without any spaces around the equal sign. For instance:
my_variable="Hello, Bash!"
In this example, we create a variable named my_variable
and assign it the string value “Hello, Bash!”. To retrieve or use the value of a variable, prepend it with a dollar sign ($
), like so:
echo $my_variable
This will output:
Hello, Bash!
Variables in Bash are untyped, which means they can hold any type of data, be it a string, a number, or even the output of a command. This lack of strict typing offers a lot of flexibility, though it can also lead to confusion if not handled carefully. For example, if you want to store the result of a command, you can use command substitution:
current_date=$(date)
This command assigns the current date and time to the variable current_date
. To see the result, you would again use:
echo $current_date
When it comes to data types, Bash primarily deals with strings and integers. While strings are the default type, Bash provides a couple of arithmetic evaluation syntaxes to handle numbers. The most common way to perform arithmetic is to use the let
command or the $((...))
syntax. Here’s how you can do some basic arithmetic:
number1=10 number2=5 let sum=number1+number2 echo "The sum is: $sum"
Alternatively, using the $((...))
syntax:
sum=$((number1 + number2)) echo "The sum is: $sum"
Both commands will output:
The sum is: 15
Another feature of variables in Bash is that they can be exported, allowing them to be available to child processes. This is done with the export
command:
export my_var="Accessible by child processes"
After exporting, any scripts or commands run from that shell session can access $my_var
as well. This is particularly useful for scripting scenarios where you want to maintain state across multiple scripts or processes.
Bash also supports arrays, which are indexed collections of values. Declaring an array involves parentheses:
my_array=(one two three four)
To access an element, use the syntax ${array_name[index]}
. For example:
echo $my_variable
0
This will output:
echo $my_variable
1
Understanding variables and their types opens up a world of possibilities in Bash scripting, enabling you to create dynamic and responsive scripts tailored to your needs. As you dive deeper into your scripting journey, mastering variables will be one of your most valuable skills.
Control Structures: Loops and Conditionals
Control structures are the backbone of any scripting language, allowing you to dictate the flow of execution based on certain conditions or to repeat tasks multiple times. In Bash, the two primary types of control structures are loops and conditionals. Mastering these constructs will empower you to write more complex and useful scripts.
Conditionals
Conditionals in Bash allow your scripts to execute different commands based on specific conditions. The most common form of conditional is the if
statement. Here’s a simple example:
#!/bin/bash read -p "Enter a number: " number if [ $number -gt 10 ]; then echo "The number is greater than 10." else echo "The number is 10 or less." fi
In this script, we prompt the user to enter a number and store it in the variable number
. The if
statement checks if number
is greater than 10 using the -gt
(greater than) comparison operator. If the condition is true, it executes the first echo
command; otherwise, it runs the echo
command in the else
block. Don’t forget to close the if
block with fi
.
Another useful conditional is the case
statement, which can simplify handling multiple possible values of a variable:
#!/bin/bash read -p "Enter a choice (a, b, c): " choice case $choice in a) echo "You chose option A." ;; b) echo "You chose option B." ;; c) echo "You chose option C." ;; *) echo "Invalid choice." ;; esac
In this example, the user’s input is matched against several defined cases. If the input matches one of the cases, the corresponding action is taken. The *
case acts as a default catch-all for invalid input, ensuring your script handles unexpected values gracefully.
Loops
Bash provides several types of loops that enable you to repeat commands. The most commonly used loops are for
, while
, and until
.
The for
loop is great for iterating over a list of items. Here’s how you can use it:
#!/bin/bash for fruit in apple banana cherry; do echo "I like $fruit" done
This loop iterates over a list of fruits and prints a message for each one. The loop variable fruit
takes on each value in the list, and the commands inside the loop are executed once for each value.
On the other hand, the while
loop continues executing as long as a specified condition is true. Here’s an example that counts down from 5:
#!/bin/bash count=5 while [ $count -gt 0 ]; do echo "Countdown: $count" ((count--)) done
In this script, we initialize count
to 5 and use a while
loop to print the countdown. The loop decrements the count with ((count--)
) until it reaches 0.
Finally, the until
loop is somewhat similar to while
, but it continues until a specified condition is true:
#!/bin/bash count=1 until [ $count -gt 5 ]; do echo "Count is: $count" ((count++)) done
This example will print the count from 1 to 5, demonstrating how the until
loop operates opposite to the while
loop.
By using control structures like loops and conditionals, you can create scripts that can handle a wide variety of tasks and respond dynamically to user input and data. This flexibility is what makes Bash such a powerful tool for automation and scripting in the command-line environment.
Functions in Bash Scripts
Functions in Bash scripts are a vital component that allows for code reusability and modularity. By defining functions, you can break down complex scripts into manageable pieces, making your code easier to read and maintain. Functions can take input parameters, perform operations, and return results, encapsulating functionality that can be invoked multiple times throughout your script.
Defining a function in Bash is simpler. You typically start with the function keyword, followed by the function name, parentheses, and then the code block enclosed in curly braces. Here’s an example:
function greet { echo "Hello, World!" }
To call the function, simply use its name followed by parentheses, like this:
greet
This will output:
Hello, World!
Functions can also accept arguments, which allows you to pass data into them when you call them. Inside a function, you can access these arguments using special variables: $1, $2, and so forth, corresponding to the first, second argument, etc. Here’s an example of a function that takes a name as an argument and greets the user:
function greet_user { echo "Hello, $1! Welcome to Bash scripting!" } greet_user "Alice"
This will output:
Hello, Alice! Welcome to Bash scripting!
Bash also allows you to return values from functions, although the return statement is limited to integer exit statuses. To return a value from a function, you can set a variable and then echo it. For example:
function add { local sum=$(( $1 + $2 )) echo $sum } result=$(add 5 7) echo "The sum is: $result"
This script defines an add function that takes two arguments, calculates their sum, and echoes the result. The output will be:
The sum is: 12
Using the local keyword, you can limit the scope of variables within a function. That’s important to avoid variable name conflicts and unintended side effects in your scripts. Always ponder making your variables local unless you specifically need them to be global.
Functions can also be defined without the function keyword, using just the name followed by parentheses, though that is less common. Here’s an example:
greet_user() { echo "Hello, $1! Welcome to Bash scripting!" }
This approach works identically to the previous function definition.
Functions in Bash scripts enhance your ability to write clean, maintainable code by encapsulating logic into reusable blocks. By incorporating functions into your scripts, you can streamline operations, reduce redundancy, and facilitate debugging. As you become more comfortable with scripting, using functions will undoubtedly elevate the sophistication and efficiency of your Bash programs.
Debugging and Error Handling
Debugging in Bash is an essential skill that can save you from hours of frustration. Scripts may not always work as expected, and learning how to effectively troubleshoot and handle errors especially important for becoming a proficient Bash programmer. In this section, we will explore various techniques for debugging Bash scripts and how to implement error handling to create robust and reliable code.
One of the simplest methods for debugging a script is to run it with the -x
option. This option enables a mode where each command is printed to the terminal before it’s executed. This can help you trace the execution of your script step by step. You can invoke this mode directly from the command line as follows:
bash -x your_script.sh
Alternatively, you can enable debugging within the script itself by adding the following line at the top:
set -x
When you’re done debugging, you can disable this mode by adding:
set +x
These commands will help you identify the flow of your script and pinpoint where things might be going wrong.
Another useful debugging technique is to insert echo
statements throughout your code. By printing variable values and messages at strategic points, you can gain insight into the state of your script at various stages of execution. For example:
#!/bin/bash var1="Hello" var2="World" echo "var1 is: $var1" echo "var2 is: $var2" echo "Combining variables..." combined="$var1 $var2" echo "Combined value is: $combined"
This approach allows you to confirm that your variables hold the expected values and that your logic is working as intended.
Error handling is another critical aspect of writing robust Bash scripts. You can use the trap
command to catch and handle errors gracefully. The trap
command allows you to specify commands to execute when your script receives specific signals or exits. For example, you can set up a trap to catch errors and perform cleanup actions:
#!/bin/bash cleanup() { echo "An error occurred. Cleaning up..." # Add cleanup commands here } trap cleanup ERR # Simulating an error echo "Running some commands..." command_that_might_fail echo "This line won't execute if the previous command fails."
In this script, if command_that_might_fail
fails, the cleanup
function is triggered, allowing you to execute any necessary cleanup commands before the script exits.
Additionally, you can check the exit status of commands using the special variable $?
. This variable holds the exit status of the last executed command, where 0
indicates success and any non-zero value indicates an error. You can use this in conjunction with conditional statements to handle errors appropriately:
#!/bin/bash mkdir my_directory if [ $? -ne 0 ]; then echo "Failed to create directory." exit 1 fi echo "Directory created successfully."
In this example, if the mkdir
command fails, the script will print an error message and exit with a non-zero status, signaling that something went wrong.
In summary, mastering the art of debugging and error handling in Bash is indispensable for writing efficient scripts. Using features like the -x
option, echo
statements, the trap
command, and checking exit statuses will enhance the reliability of your scripts and streamline the troubleshooting process. These techniques not only help catch errors but also aid in understanding your code better, making you a more effective Bash programmer.