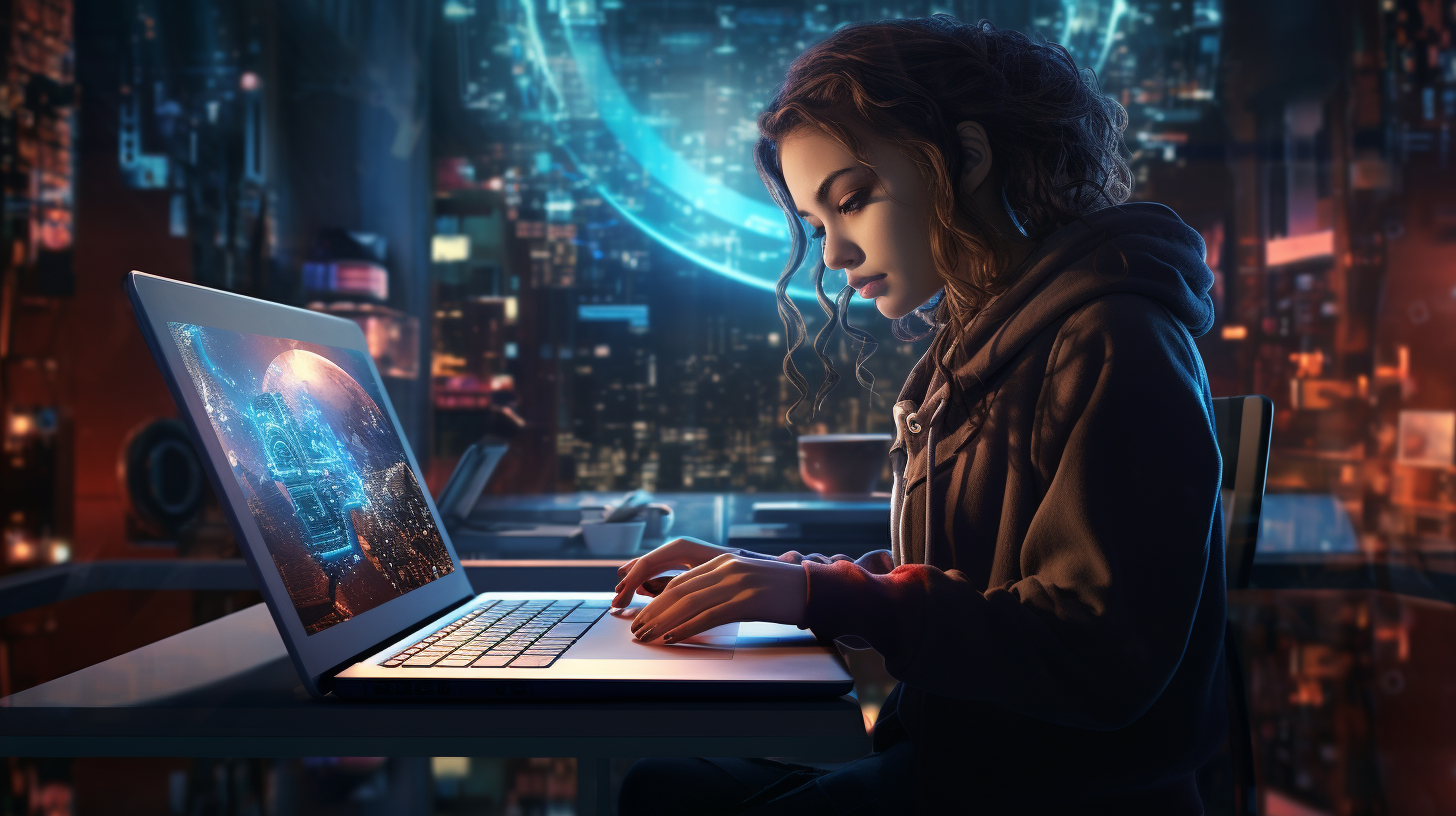
Bash in Cybersecurity – Scripting Tips
Bash scripting serves as a powerful ally in the sphere of cybersecurity, providing a versatile platform for automating tasks and managing system resources efficiently. Understanding the fundamentals of Bash scripting very important for cybersecurity professionals seeking to enhance their skill set. At its core, a Bash script is a file containing a series of commands that the Bash interpreter executes in sequence.
To begin scripting, you might want to create a simple script that can print text to the terminal. That is done by using the echo
command:
#!/bin/bash echo "Hello, Cybersecurity World!"
In this example, the first line, known as the shebang, indicates that the script should be run using the Bash interpreter. The echo
command outputs the specified string to the terminal.
Next, you’ll often find the need to use variables. Variables in Bash are defined without a preceding dollar sign and can be referenced by prefixing them with a dollar sign:
#!/bin/bash greeting="Welcome to Bash Scripting!" echo $greeting
Control structures, such as loops and conditional statements, allow for more complex scripts. A common construct is the if
statement, useful for making decisions based on the output of a command:
#!/bin/bash if [ -d "/etc" ]; then echo "/etc directory exists." else echo "/etc directory does not exist." fi
In this snippet, the script checks for the existence of the /etc
directory and outputs a message depending on the result.
Loops, such as for
and while
, enable the execution of a block of code multiple times:
#!/bin/bash for i in {1..5}; do echo "Iteration number: $i" done
This loop will iterate from 1 to 5, printing the iteration number each time.
Another essential aspect of Bash scripting in cybersecurity is working with command line arguments. This allows scripts to be more dynamic by accepting input from the user:
#!/bin/bash echo "The first argument is: $1" echo "The second argument is: $2"
When you run this script, you can pass arguments from the command line, enhancing the script’s utility.
Lastly, incorporating comments using the #
symbol is a good practice to document your code, making it easier for others (or yourself) to understand the purpose and functionality of different sections of the script:
#!/bin/bash # This script demonstrates basic Bash scripting concepts. echo "Learning Bash scripting!"
Common Bash Commands for Security Tasks
In the context of cybersecurity, proficiency with common Bash commands is pivotal for executing security-related tasks efficiently. These commands form the backbone of typical operations ranging from file handling to process management. Familiarizing oneself with these commands can dramatically increase a cybersecurity professional’s ability to respond to incidents and automate routine tasks.
One of the most frequently used commands is ls, which lists files and directories. In a security context, it can help you quickly inspect the contents of sensitive directories:
ls -l /etc
The grep command is another powerhouse, allowing you to search for specific patterns within files. That’s particularly useful when analyzing logs for signs of malicious activity:
grep "ERROR" /var/log/syslog
To monitor real-time logs, you can combine tail and grep to filter output dynamically:
tail -f /var/log/auth.log | grep "Failed password"
When it comes to file manipulation, the cp and mv commands are indispensable. They allow you to copy and move files, respectively. For example, to create a backup of a configuration file, you might use:
cp /etc/ssh/sshd_config /etc/ssh/sshd_config.bak
Permissions are a critical aspect of system security, and the chmod command allows you to change file permissions. For instance, if a script should be executable only by the owner, you would use:
chmod 700 myscript.sh
In scenarios where you need to check running processes or system resource usage, the ps and top commands become invaluable. ps lets you see a snapshot of current processes:
ps aux | grep apache2
For real-time monitoring of system performance, top provides a dynamic view of processes and resource utilization:
top
Network security tasks also heavily rely on Bash commands. The netstat command can provide insights into active connections, while curl is used for making network requests. To check for open ports, you might use:
netstat -tuln
To fetch a webpage and examine its response, curl can be employed as follows:
curl -I http://example.com
Finally, automating repetitive tasks is a hallmark of effective Bash usage. By chaining commands with && or using pipes, you can create powerful one-liners. For instance, to clean up old log files while at the same time backing them up, you could craft:
cp /var/log/*.log /path/to/backup/ && rm /var/log/*.log
Automating Security Audits with Bash Scripts
Automating security audits with Bash scripts can streamline the process of evaluating system vulnerabilities and compliance with security policies. By using the power of scripting, security professionals can create efficient workflows that systematically check for potential issues across multiple systems. This not only saves time but also enhances the accuracy of audits, minimizing the chances of human error.
A typical security audit script might focus on checking user accounts, installed packages, and system configurations. Below is an example of a Bash script that performs a simple audit of user accounts, ensuring that only expected users have access to the system:
#!/bin/bash # Audit user accounts on the system # Define expected users expected_users=("admin" "user1" "user2") # Get a list of actual users actual_users=$(cut -d: -f1 /etc/passwd) # Check for unexpected users for user in $actual_users; do if [[ ! " ${expected_users[@]} " =~ " ${user} " ]]; then echo "Unexpected user found: $user" fi done
In the above script, we define an array of expected users. The script then retrieves the list of actual users from the /etc/passwd file and checks for any discrepancies. If an unexpected user is found, it outputs a warning, enabling quick identification of potentially unauthorized accounts.
Another common audit task is checking for outdated packages that may pose security risks. Using the package manager, we can automate the process of identifying packages that need updates:
#!/bin/bash # Audit for outdated packages # Update package list apt-get update # Check for upgrades outdated_packages=$(apt list --upgradable 2>/dev/null | tail -n +2) if [ -z "$outdated_packages" ]; then echo "No outdated packages found." else echo "Outdated packages found:" echo "$outdated_packages" fi
This script first updates the package list and then checks for any available upgrades. It suppresses error messages and only outputs relevant information. Engaging with the package management system in this way ensures that systems are running the latest software, reducing vulnerability risks.
System configuration audits, particularly for critical files such as /etc/ssh/sshd_config, are also vital. A simple script can check whether certain parameters are set correctly. For instance, to ensure that password authentication is disabled, the following script can be used:
#!/bin/bash # Audit SSH configuration config_file="/etc/ssh/sshd_config" if grep -q "^PasswordAuthentication no" "$config_file"; then echo "Password authentication is disabled." else echo "Warning: Password authentication is enabled." fi
This script utilizes grep to search for the “PasswordAuthentication no” line within the SSH configuration file. If the setting is correct, it confirms compliance; otherwise, it issues a warning.
Additionally, automating the collection of system logs can provide invaluable insight during an audit. A script can be crafted to gather log entries from various sources and compress them for analysis:
#!/bin/bash # Collect system logs for audit log_directory="/var/log" output_file="/path/to/audit_logs_$(date +%F).tar.gz" tar -czf "$output_file" $log_directory/*.log echo "Logs collected and archived to $output_file"
This script archives all log files from the specified directory into a compressed tar.gz file, organizing the logs for easier review and analysis.
Error Handling and Debugging in Bash Scripting
Error handling and debugging are critical components of effective Bash scripting, especially in the context of cybersecurity. When automating security tasks, it’s essential to ensure that scripts behave predictably and handle unexpected situations gracefully. A robust script not only performs its designated functions but also anticipates potential errors and responds accordingly.
One fundamental technique in error handling is checking the exit status of commands. In Bash, every command returns an exit status, where a status of zero indicates success, and any non-zero value indicates failure. By examining this status, you can control the flow of your script based on the success or failure of commands.
#!/bin/bash # Check if a directory exists dir="/path/to/directory" if [ -d "$dir" ]; then echo "Directory exists." else echo "Error: Directory does not exist." exit 1 fi
In this example, the script checks for the existence of a directory and provides a clear error message if it does not exist, exiting the script with a non-zero status. This practice not only alerts users to issues but also allows for better integration of scripts into larger workflows, where downstream processes can react to errors appropriately.
Another useful technique involves using the trap command to catch errors and cleanup resources when a script encounters a problem. For example, if your script creates temporary files, you can ensure these files are deleted, even if the script exits unexpectedly:
#!/bin/bash # Cleanup temporary files on error temp_file="/tmp/tempfile" trap 'rm -f "$temp_file"; echo "Error occurred, cleaning up."; exit 1;' ERR # Simulate an error echo "Creating a temporary file..." touch "$temp_file" echo "Temporary file created." # Intentional error false echo "This line will not execute."
In this script, if the command false fails (which it always does), the trap command is triggered, ensuring that the temporary file is removed and a message is displayed. This adds a layer of reliability to your scripts, especially in scenarios involving critical tasks.
Debugging is another crucial aspect of Bash scripting. When troubleshooting issues, you can utilize the set command to enable debugging options. For instance, using set -x will print each command before it’s executed, so that you can trace the script’s execution flow:
#!/bin/bash # Enable debugging set -x echo "Starting script..." # Simulated commands mkdir /some/directory touch /some/directory/file.txt echo "File created." # Disable debugging set +x echo "Script completed."
By enabling debugging, each command will be displayed in the terminal, providing insight into the script’s execution and making it easier to identify where things may have gone wrong. Once debugging is no longer needed, you can turn it off with set +x.
Best Practices for Secure Bash Scripting
When crafting secure Bash scripts, it’s essential to follow best practices that minimize security risks and ensure the integrity of your code. Bash scripts can often run with elevated privileges, making them a potential vector for security vulnerabilities if not written carefully. Here are some key best practices to ponder.
Use Absolute Paths: Always use absolute paths for commands and files. This prevents ambiguity and ensures that your script always references the intended files or commands, regardless of the current working directory.
#!/bin/bash # Using absolute paths for security /bin/echo "Hello, secure world!"
Quote Variables: Quoting variables is important to prevent word splitting and globbing by the shell. This practice protects against command injection attacks and unexpected behavior.
#!/bin/bash user_name="admin" echo "Welcome, $user_name" # Correct: "$user_name" is quoted
Validate Input: Always validate user inputs and command-line arguments. That’s vital for maintaining control over what your script accepts, especially when executing commands that could affect system security.
#!/bin/bash # Input validation if [[ "$1" =~ ^[a-zA-Z0-9_]+$ ]]; then echo "Valid input: $1" else echo "Invalid input!" exit 1 fi
Set the Script to Fail on Errors: Use the set -e command to ensure that your script exits immediately if any command fails. This prevents further execution that could lead to unintended consequences.
#!/bin/bash set -e # Example script that exits on error cp /some/nonexistent/file /tmp/
Limit Script Privileges: Avoid running scripts with root privileges unless absolutely necessary. Use the principle of least privilege to limit the potential impact of a compromised script.
Implement Logging: Incorporate logging within your scripts to track their execution and identify potential issues. That is particularly useful for auditing and incident response.
#!/bin/bash log_file="/var/log/myscript.log" echo "Script execution started at $(date)" >> "$log_file"
Regularly Review and Update Scripts: Cybersecurity is a constantly evolving field. Regularly review your scripts for vulnerabilities and update them as necessary to adapt to new threats and best practices.
Use Version Control: Storing your scripts in a version control system such as Git allows you to track changes, collaborate with others, and roll back to earlier versions if necessary. This adds an extra layer of security and accountability to your scripting practices.
Integrating Bash Scripts with Security Tools and Frameworks
Integrating Bash scripts with security tools and frameworks is an essential skill for cybersecurity professionals. By using the power of existing security tools, you can enhance your scripting capabilities, automate repetitive tasks, and create comprehensive security solutions that are both efficient and effective. Bash scripts can serve as the glue that binds various tools together, allowing for streamlined workflows that leverage the strengths of each tool.
One common integration is with network scanning tools like Nmap. By scripting Nmap scans, you can automate the process of identifying open ports and services on a target system. Here’s a simple example of a Bash script that performs a basic Nmap scan:
#!/bin/bash # Script to perform a network scan using Nmap target="$1" if [[ -z "$target" ]]; then echo "Usage: $0 " exit 1 fi echo "Scanning $target for open ports..." nmap -sS "$target"
In this script, the user specifies a target IP address as a command-line argument, which the script then uses to perform a stealth SYN scan. Automating this task ensures that you can quickly gather information about your network without manual intervention.
Another powerful integration is with log analysis tools, such as AWK or Logwatch. When analyzing logs for suspicious activity, you can use Bash scripts to filter and summarize log entries. Here’s an example of a script that uses AWK to extract failed login attempts from an SSH log:
#!/bin/bash # Analyze SSH log for failed login attempts log_file="/var/log/auth.log" echo "Failed login attempts:" awk '/Failed password/ {print $1, $2, $3, $9}' "$log_file" | sort | uniq -c | sort -nr
This script searches the auth.log file for lines that contain “Failed password”, extracting relevant information and providing a count of unique failed attempts. This allows security professionals to quickly identify potential brute-force attacks.
Integration with configuration management tools like Ansible can also be beneficial. You can create Bash scripts that serve as modules or playbooks for Ansible, allowing for dynamic execution of tasks based on the current state of the system. For instance, a script can be used to ensure that essential security updates are applied:
#!/bin/bash # Check and install security updates echo "Checking for security updates..." apt-get update apt-get -y upgrade --only-upgrade echo "Security updates applied."
This script can be invoked by Ansible to ensure that all managed systems are up to date, reducing the attack surface by eliminating vulnerabilities associated with outdated software.
Finally, integrating with incident response frameworks such as TheHive or MISP can enhance your ability to respond to security incidents. By constructing Bash scripts that automate the retrieval of indicators of compromise (IOCs) and pushing them to these platforms, you can streamline your incident response workflow:
#!/bin/bash # Push IOCs to TheHive api_url="https://your-thehive-instance/api" api_key="your_api_key" ioc="malicious.com" curl -X POST "$api_url/ioc" -H "Authorization: $api_key" -H "Content-Type: application/json" -d '{ "dataType": "domain", "iocs": ["'"$ioc"'"], "description": "Malicious domain observed" }'
This script uses the curl command to send IOCs to TheHive, integrating your detection capabilities with your incident response processes. Automating such tasks ensures that critical information is transmitted quickly and accurately, facilitating a timely response to threats.