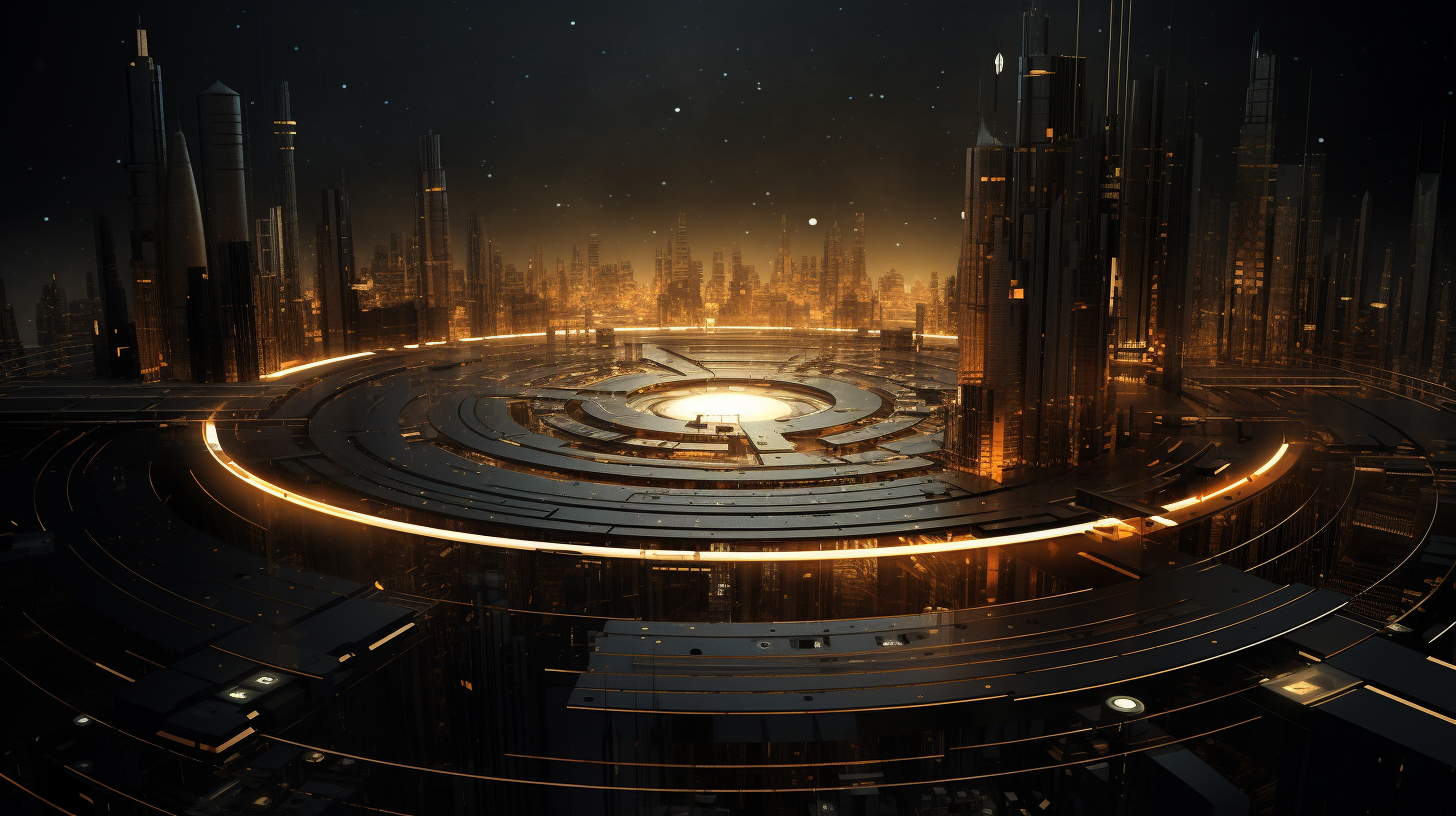
Bash Regular Expressions
Bash regular expressions serve as a powerful tool for pattern matching, allowing you to validate and manipulate text efficiently. They can be particularly useful when you want to search for specific strings within larger bodies of text or when filtering input data. Understanding how to leverage these regular expressions can significantly enhance your scripting capabilities.
Bash utilizes two types of regular expressions: basic and extended. The basic regular expressions (BRE) are the default in Bash. They include special characters that have special meanings, such as .* for matching any character zero or more times, and ^ for matching the start of a line. The extended regular expressions (ERE), on the other hand, offer additional capabilities and are enabled with the -E
option in certain commands.
When working with regular expressions in Bash, syntax plays a critical role in how patterns are defined and matched. For example, you can use the [[ ]]
conditional expression for pattern matching. Here’s a simple example demonstrating the use of basic regular expressions:
#!/bin/bash string="Hello, World!" if [[ $string =~ ^Hello ]]; then echo "The string starts with 'Hello'." fi
In this example, the ^Hello
pattern checks if the variable string
begins with the word “Hello”. If the condition is satisfied, it echoes a confirmation message.
Additionally, you can utilize parentheses for grouping and the pipe character | for logical OR operations. This extends the flexibility of your patterns, allowing for more complex matching scenarios. Think this example:
#!/bin/bash input="cat" if [[ $input =~ ^(cat|dog)$ ]]; then echo "Input is either 'cat' or 'dog'." else echo "Input is something else." fi
This snippet checks if the input
variable contains either “cat” or “dog”, thanks to the use of parentheses to group the options. Mastering these details can transform your approach to text processing in Bash scripts.
Understanding the fundamental concepts of Bash regular expressions will empower you to create more efficient scripts. As you experiment with different patterns and scenarios, you’ll find that the ability to parse and manipulate strings is not only a skill but an art form in itself.
Syntax and Structure of Regular Expressions
Regular expressions in Bash follow a specific syntax that defines how patterns are constructed. This syntax includes a combination of literal characters and special characters that allow for flexible and powerful pattern matching.
At the core of Bash regular expressions, you will find a set of operators and metacharacters. The period (.) matches any single character, while the asterisk (*) matches zero or more occurrences of the preceding element. For instance, the pattern abc.*
matches any string that begins with “abc” followed by any number of characters.
Anchors are also vital in defining where a match can occur within a string. The caret symbol (^) is used to signify the start of a string, whereas the dollar sign ($) indicates the end of a string. For example, the pattern ^abc$
exclusively matches the string “abc” and nothing else.
Character classes provide another layer of specificity by which will allow you to define a set of characters to match against. By enclosing characters in square brackets, you can specify acceptable characters. For instance, the pattern [aeiou]
matches any single vowel, while [0-9]
matches any single digit. You can also define ranges, such as [a-z]
for any lowercase letter.
To define sequences or repetitions, you can use curly braces to specify how many times a character or group should occur. For example, the pattern ba{2,4}n
matches “ban”, “baaan”, “baaaan”, or “baaaaan”. This construct offers great flexibility in matching repeated patterns.
Grouping is accomplished using parentheses, which will allow you to apply operators to entire sub-patterns. This is particularly useful when working with alternatives or when you want to apply quantifiers to a group of characters. The pattern (abc|def)
matches either “abc” or “def”.
Here’s a practical demonstration of various syntax elements in a single script:
#!/bin/bash input="Hello, 123" if [[ $input =~ ^Hello ]]; then echo "Input starts with 'Hello'." fi if [[ $input =~ [0-9]+ ]]; then echo "Input contains numbers." fi if [[ $input =~ ^[A-Za-z]+ ]]; then echo "Input starts with letters." fi if [[ $input =~ (Hello|Hi) ]]; then echo "Greeting detected." fi if [[ $input =~ ba{2,4}n ]]; then echo "Pattern with repetitions matched." fi
In this example, we utilize a variety of syntax elements to check different conditions against the variable input
. Each if
statement leverages different aspects of regular expressions, showcasing the versatility and power of this tool in Bash scripting.
As you delve deeper into the syntax and structure of Bash regular expressions, you’ll discover a world of possibilities that can significantly streamline your text processing tasks. Each character, each operator, contributes to a rich tapestry of pattern matching that, once mastered, can transform the way you approach string manipulation in your scripts.
Practical Examples and Use Cases
Bash regular expressions open the door to a multitude of practical applications that can streamline your scripting tasks. From validating user input to parsing log files, the ability to leverage regex effectively can vastly improve the efficiency and clarity of your scripts. Here are some compelling use cases that demonstrate the power and versatility of Bash regular expressions.
One of the most common applications of regular expressions is input validation. For example, if you’re writing a script that requires user input in a specific format, regex can help ensure that the input meets your criteria. Ponder a scenario where you need to validate an email address:
#!/bin/bash read -p "Enter your email: " email if [[ $email =~ ^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+.[a-zA-Z]{2,}$ ]]; then echo "Valid email address." else echo "Invalid email address." fi
The pattern used here checks for a standard email format, ensuring that it contains a local part, the ‘@’ symbol, and a domain. This kind of validation is important in many scripts where user input must conform to specific formats.
Another practical application is log file analysis. When analyzing logs, you might want to filter out entries that match certain patterns. For instance, if you are interested in capturing error messages from a log file, you can use the following script:
#!/bin/bash log_file="system.log" grep -E "ERROR|WARNING" $log_file
In this example, the use of grep with the -E flag allows for extended regular expressions, enabling us to search for both “ERROR” and “WARNING” in the log file. This makes it easy to quickly identify critical issues that need attention.
Regular expressions can also be instrumental in text manipulation tasks. Suppose you need to extract specific data from a structured text file, such as CSV. You can parse and modify the data with regex like so:
#!/bin/bash input_file="data.csv" while IFS=, read -r name age location; do if [[ $age -gt 30 ]]; then echo "$name, $location" fi done < $input_file
This script reads a CSV file line by line, extracting the name, age, and location. It uses a conditional check to filter out entries where age is greater than 30, demonstrating how regex can be combined with other Bash constructs to manipulate and process data effectively.
Additionally, regular expressions can aid in renaming files based on specific patterns. If you have a batch of files with inconsistent naming conventions and you want to standardize their names, regex can help you achieve that. Here’s an example of how to rename files ending in “.txt” to have a “.bak” extension:
#!/bin/bash for file in *.txt; do mv "$file" "${file%.txt}.bak" done
This loop leverages parameter expansion to remove the “.txt” extension and replace it with “.bak”, effectively renaming all matching files in the current directory.
As you can see, the practical applications of Bash regular expressions are vast, encompassing user input validation, log analysis, data extraction, and file manipulation. By mastering these regex techniques, you can create more robust, efficient, and maintainable scripts that significantly enhance your productivity as a Bash programmer.
Common Pitfalls and Troubleshooting Tips
When working with Bash regular expressions, encountering pitfalls is a common experience, especially for those new to the realm of pattern matching. Recognizing and troubleshooting these issues can save considerable time and frustration. One frequent mistake is misunderstanding the context in which regular expressions operate. In Bash, the double square brackets ([[ ]]) are used for regex matching, while single brackets ([ ]) are for string comparisons. This distinction very important. Attempting to use regex in single brackets will not yield the desired results.
Another common pitfall lies in the nuances of character classes and anchors. For instance, if you intend to match the entire string, failing to use both the caret (^) and dollar sign ($) can result in unexpected matches. A pattern like ‘abc’ will match any string containing ‘abc’, while ‘^abc$’ will only match the exact string ‘abc’. This subtlety can lead to logical errors in scripts, especially when validating input or processing text.
Escape characters also play an important role in regular expressions. Many characters, such as the period (.), asterisk (*), and parentheses, possess special meanings. If you need to match these characters literally, you must escape them using a backslash (). For example, to match a period, you should use ‘.’ instead of just ‘.’. Forgetting to escape these characters can lead to misinterpretation of the intended pattern, often resulting in incorrect matches or no matches at all.
In addition, it is essential to be mindful of the regex engine’s behavior regarding greedy versus lazy matching. By default, quantifiers like * and + are greedy, meaning they will match as much text as possible. If you want to limit the match to the shortest possible string, you need to employ lazy quantifiers, which are appended with a ‘?’ (e.g., .*?). Understanding this behavior is vital when dealing with complex patterns, as it can significantly affect the output of your scripts.
Here’s a practical example demonstrating some of these pitfalls:
#!/bin/bash input="abc123" # Common mistake: using single brackets for regex if [ $input =~ ^abc ]; then echo "This won't work as expected!" fi # Correct usage with double brackets if [[ $input =~ ^abc ]]; then echo "Input starts with 'abc'." fi # Forgetting to escape a special character if [[ $input =~ .123 ]]; then echo "This won't match anything." fi # Correctly escaping if [[ $input =~ .123 ]]; then echo "You need to escape the period." fi # Greedy vs. Lazy matching string="X123X456" # Greedy match if [[ $string =~ X.*X ]]; then echo "Greedy match: $BASH_REMATCH" fi # Lazy match if [[ $string =~ X.*?X ]]; then echo "Lazy match: $BASH_REMATCH" fi
In this example, we illustrate mistakes and their corrections. The first check highlights the importance of using double brackets for regex matching. The second section emphasizes escaping special characters correctly. Finally, we compare greedy and lazy matching, demonstrating how both can yield different results depending on your patterns. By being aware of these common pitfalls and refining your understanding of regular expressions in Bash, you can significantly enhance your text processing skills.