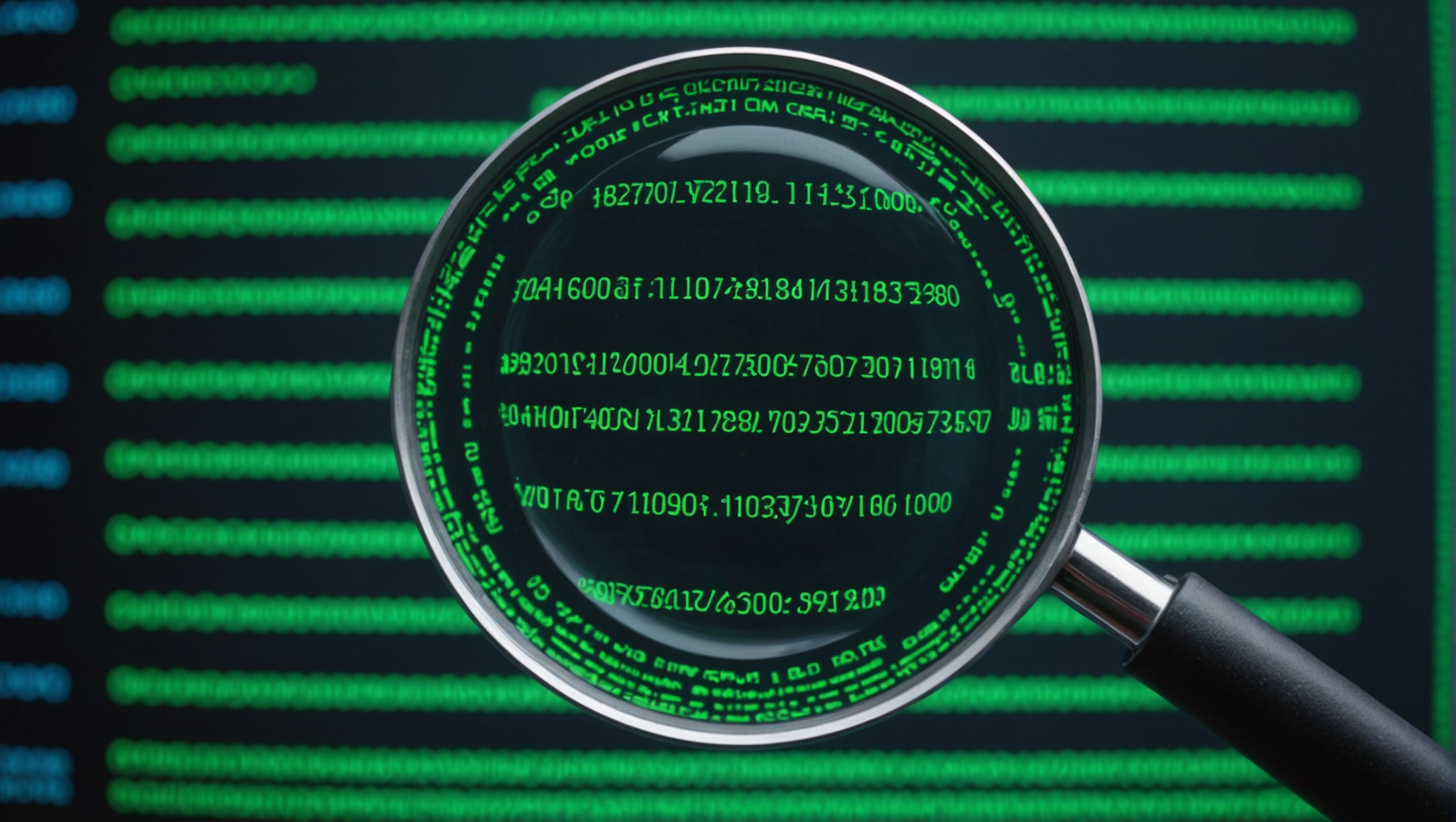
Bash Script Debugging Techniques
When diving into the world of Bash scripting, it’s crucial to familiarize yourself with the various debugging options available. Bash provides several built-in flags that can help you trace and identify issues within your scripts, making it easier to understand where things might be going awry.
One of the primary tools at your disposal is the -x
option, which enables a mode of debugging that prints each command and its arguments to the terminal before executing it. This is akin to having a magnifying glass over your script, providing insights into the execution flow.
#!/bin/bash set -x # Enable debugging echo "This is a test" ls /nonexistent_directory # This will trigger an error
By including set -x
at the beginning of your script, you can observe each command as it runs. When you run the above script, you’ll see output that reveals exactly what commands are being executed, which can be invaluable in pinpointing the source of errors.
Another essential debugging option is -e
. When set, this flag causes the script to immediately exit if any command fails (returns a non-zero exit status). That’s particularly useful for ensuring that your script does not continue executing when a critical command fails.
#!/bin/bash set -e # Exit immediately if a command exits with a non-zero status echo "Starting script" cp nonexistent_file.txt /tmp/ # This will cause the script to exit echo "This will not execute"
In the example above, because the cp
command fails, the script exits immediately, and the subsequent echo
command is never executed. This helps in preventing cascading failures within your script.
Additionally, you can combine these options to maximize your debugging capabilities. Using set -ex
together allows you to see both the commands being executed and ensures that the script stops on errors.
#!/bin/bash set -ex # Enable debugging and exit on error echo "Starting script" mkdir /tmp/test_directory cd /nonexistent_directory # This will exit the script echo "This will not execute either"
By understanding and effectively using these debugging options, you can gain a clearer view of your script’s operations and quickly identify areas that may require attention. Debugging in Bash is not just about fixing errors; it’s about gaining insights into your scripts’ behavior and improving their reliability.
Using Debugging Tools and Commands
Once you’ve armed yourself with the fundamental debugging options, now, let’s delve deeper into more sophisticated tools and commands that can significantly enhance your debugging prowess. Tools such as `trap`, `set`, and `declare` can offer insightful perspectives into the behavior of your scripts during execution.
The trap command allows you to catch signals and execute specific commands when they’re received. This is especially useful for debugging, as you can set traps to log error messages or clean up resources in case your script exits unexpectedly. For example, you can trap the EXIT signal to perform actions just before your script terminates, providing an opportunity to inspect output or state.
#!/bin/bash trap 'echo "Script exited with status $?"; exit' EXIT echo "This is a test script" false # This command will fail and trigger the trap
In the script above, when `false` executes and fails, the trap catches the EXIT signal, so that you can log the exit status before the script terminates. This provides useful feedback and can help you understand the point of failure.
Another powerful debugging tool is the set command, which provides a way to enable or disable certain script behaviors. The command set -u
can be particularly useful; it forces the script to treat unset variables as an error and exit. This helps catch situations where a variable might not have been initialized, which can lead to unexpected behaviors.
#!/bin/bash set -u # Exit if any variable is unset echo "Value of unset variable: $UNSET_VAR" # This will cause the script to exit
In this case, the script will terminate with an error message when it attempts to access the unset variable UNSET_VAR
, so that you can catch the issue early on.
Additionally, the declare command can be employed to help debug variable types and attributes. By using declare -p
, you can print the attributes and values of variables, which can assist in verifying that your script is handling data types correctly.
#!/bin/bash declare -i num=5 # Declare an integer variable declare -a arr=(1 2 3) # Declare an array declare -p num # Print the variable attributes and value declare -p arr # Print the array attributes and value
Understanding the attributes of your variables can be crucial for debugging complex scripts, especially when dealing with arrays or arithmetic operations.
Finally, don’t overlook the importance of using echo or printf strategically throughout your script. Simple debugging output can provide clarity on variable states and execution flow. For example, using echo to display the current value of a variable at different points can reveal where things go wrong.
#!/bin/bash my_var="Hello, World!" echo "Before modification: $my_var" my_var="Goodbye, World!" echo "After modification: $my_var"
With these tools and commands in your arsenal, you enhance your ability to debug Bash scripts effectively. Each tool serves a unique purpose, providing further granularity and insight into the nuances of script execution. The right combination of these debugging techniques can make the difference between a script that silently fails and one that performs reliably and predictably.
Common Debugging Techniques
When it comes to debugging in Bash, certain techniques stand out for their effectiveness and simplicity. Understanding and employing these techniques can significantly ease the process of identifying issues within your scripts. A few common debugging techniques include the use of echo statements, conditional breakpoints, and logging output to files.
One of the most simpler and widely used methods for debugging is the insertion of echo
statements throughout your script. By strategically placing echo statements, you can output variable values or messages that indicate the script’s progress. This allows you to track how far the script has executed and the state of various variables at different points in time.
#!/bin/bash some_var="Initial Value" echo "Starting script with some_var: $some_var" some_var="Modified Value" echo "Now some_var is: $some_var" # Simulating a conditional error if [ "$some_var" == "Unknown Value" ]; then echo "This is an unexpected state" fi
In this example, the echoes provide feedback on the state of some_var
at key moments, so that you can see how its value changes as the script progresses. This technique can be especially helpful when troubleshooting logic errors or unexpected behavior.
Conditional breakpoints are another powerful debugging technique, particularly when combined with the set -x
option. By incorporating conditions into your debug output, you can isolate specific cases that may lead to errors. This approach allows for targeted debugging without cluttering your output with too many messages.
#!/bin/bash set -x # Enable debugging for i in {1..5}; do echo "Iteration: $i" if [ $i -eq 3 ]; then echo "Condition met at iteration 3" break fi done
Here, the script outputs each iteration of the loop, but when it reaches the condition for i
being equal to 3, it logs a special message and breaks out of the loop. This succinct output can help pinpoint when specific conditions arise during execution.
Logging output to files is another beneficial technique, particularly for longer scripts that may produce a lot of output. Instead of flooding your terminal with messages, you can redirect output to a log file. This practice not only keeps your terminal clean but also provides a historical record of the script’s execution, which can be invaluable for post-mortem analysis.
#!/bin/bash log_file="script.log" exec > $log_file # Redirect all output to the log file echo "Script started at $(date)" echo "Performing operations..." # Simulating operations echo "Operation 1 complete." echo "Operation 2 complete." echo "Script finished at $(date)"
In this example, all output from the script is redirected to script.log
. This allows you to review the entire output after execution, making it easier to spot errors or unexpected behavior that may have occurred during the run.
Finally, don’t underestimate the power of commenting out sections of your script. When you suspect an area of your code is problematic, you can comment it out and re-run your script to see if the issue persists. This method helps isolate the part of the code that may be causing trouble.
#!/bin/bash echo "Starting script" # Commenting out the following line for testing # echo "This line may cause issues" echo "Continuing with the rest of the script"
By applying these common debugging techniques, you can enhance your ability to troubleshoot and resolve issues in your Bash scripts. Each technique offers a different perspective on the execution flow, helping you to uncover the root causes of errors and improve the overall reliability of your scripts.
Best Practices for Writing Debug-Friendly Scripts
Writing debug-friendly Bash scripts is an essential skill for any developer. By following best practices, you can create scripts that are easier to troubleshoot and maintain. A few of the most effective strategies include adopting a consistent coding style, using clear variable names, and incorporating thorough comments throughout your code.
First and foremost, adopting a consistent coding style can greatly enhance the readability of your scripts. Using indentation and spacing uniformly helps in visually parsing the structure of your script, making it easier to locate potential issues. For example, think the following code segment:
#!/bin/bash function my_function() { local var1="Value1" local var2="Value2" echo "Var1: $var1" echo "Var2: $var2" } my_function
In this snippet, the function and its variables are neatly organized with consistent indentation. This clarity can be invaluable during debugging, allowing you to quickly see where each part of your script resides.
Next, using descriptive and clear variable names very important. Avoid cryptic abbreviations and opt for names that convey the purpose of the variable. For instance:
#!/bin/bash user_count=10 max_users=20 if [ "$user_count" -gt "$max_users" ]; then echo "User limit exceeded." else echo "User limit is within safe range." fi
Here, the variables user_count
and max_users
provide immediate context, making it easier to understand the logic without having to trace back their purpose. This is particularly helpful when you or someone else revisits the script later.
Comments are another vital aspect of writing debug-friendly scripts. They allow you to annotate your code, explaining the reasoning behind certain choices or detailing what each section does. For example:
#!/bin/bash # This script checks if the user count exceeds the maximum allowed. user_count=10 max_users=20 # Compare user count to maximum users if [ "$user_count" -gt "$max_users" ]; then echo "User limit exceeded." else echo "User limit is within safe range." fi
In this example, the comments clarify the script’s overall purpose and the specific function of each segment. They act as a roadmap for anyone reviewing the code, reducing the time spent deciphering logic.
Additionally, think modularizing your scripts into functions. This strategy not only enhances readability but also allows for easier testing and debugging of individual components. Functions encapsulate specific behavior, making it simpler to identify where an issue may lie. Here’s how you might implement this:
#!/bin/bash # Function to check user count check_user_count() { local user_count=$1 local max_users=$2 if [ "$user_count" -gt "$max_users" ]; then echo "User limit exceeded." return 1 # Indicating an error fi return 0 # Indicating success } # Main script execution max_users=20 check_user_count 10 $max_users
This modular approach allows you to test check_user_count
independently, making it far easier to pinpoint errors without wading through unrelated code.
Lastly, maintain a habit of thorough testing. Whenever you add new features or make changes, run your scripts in a controlled environment to ensure that they behave as expected. Employing unit tests can also be beneficial; they help validate that individual functions behave correctly. For instance:
#!/bin/bash test_check_user_count() { check_user_count 10 20 # This should return success if [ $? -ne 0 ]; then echo "Test failed: User limit check" fi check_user_count 30 20 # This should return failure if [ $? -eq 0 ]; then echo "Test failed: User limit exceeded check" fi } # Run tests test_check_user_count
By integrating these best practices into your Bash scripting, you create a strong foundation that not only facilitates debugging but also enhances the maintainability and clarity of your code. As you grow more comfortable with these principles, you’ll find that debugging becomes a much smoother and less daunting process.