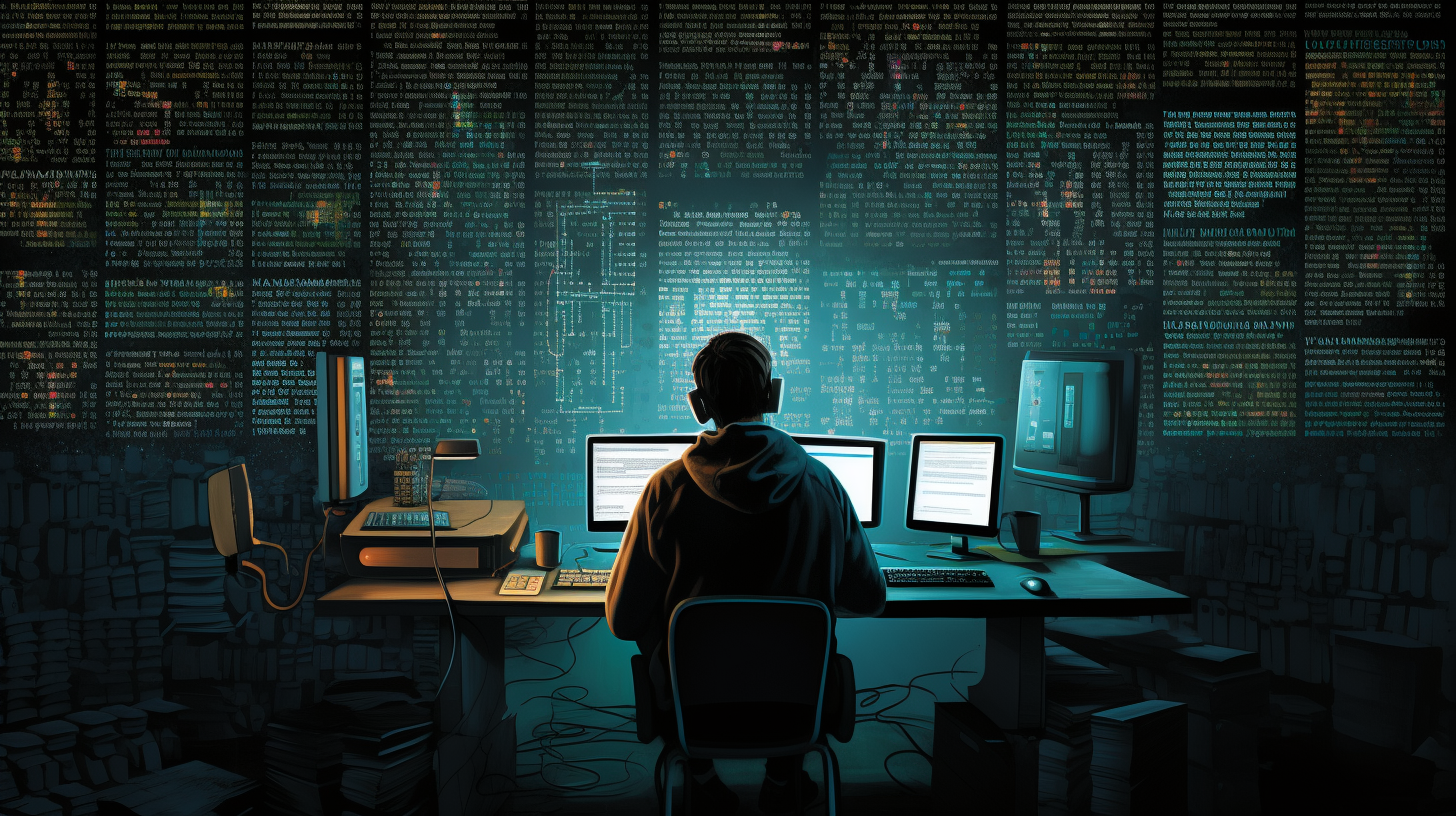
Bash Scripting Basics
Bash scripting offers a flexible and powerful way to automate tasks in a Unix-like environment. At its core, a Bash script is simply a file containing a sequence of commands that the Bash shell can execute. Understanding the syntax and structure of a Bash script is important for writing effective and efficient scripts.
Each Bash script begins with a shebang line, which indicates the script’s interpreter. The most common shebang for Bash scripts is:
#!/bin/bash
This line tells the system to execute the script using the Bash shell. Following the shebang, you can place comments in your script by using the #
symbol. Comments are essential for documenting your code and explaining its functionality:
# That's a comment explaining the following command echo "Hello, World!"
Commands in a Bash script can be as simple as calling built-in commands or executing external programs. Each command is typically placed on its own line, and you can use semicolons (;)
to separate multiple commands on the same line:
echo "Starting script"; echo "This is a simple command"; ls -l
Variables in Bash are crucial for storing data. You can create a variable by assigning a value without spaces around the equals sign:
my_variable="Hello, Bash!"
To access the value of a variable, precede its name with a dollar sign $
:
echo $my_variable
Control structures form the backbone of logic in Bash scripts. For conditionals, the if
statement is widely used. The syntax is as follows:
if [ condition ]; then # commands to execute if condition is true else # commands to execute if condition is false fi
Loops enable executing a block of commands repeatedly. The for
loop is particularly useful:
for i in {1..5}; do echo "Iteration $i" done
Each Bash script can be structured into functions, allowing for modular programming. A function is defined using the following syntax:
my_function() { # function commands }
To call a function, simply use its name followed by parentheses:
my_function
Variables and Data Types
In Bash, variables are key to storing and manipulating data throughout your scripts. Unlike many other programming languages, Bash does not require you to declare a variable type explicitly. You can simply assign a value to a variable using the equals sign, with no spaces on either side of the equals sign. For example:
my_variable="Hello, Bash!"
Once you’ve assigned a value, you can access the variable’s content by prefixing its name with a dollar sign ($). This allows for dynamic interactions and can facilitate complex data handling. For example:
echo $my_variable
Bash supports several data types, including strings, integers, and arrays. Strings are simply sequences of characters, which can be enclosed in either single or double quotation marks. The distinction between these is subtle; double quotes allow for variable expansion, whereas single quotes treat everything literally:
string_var="This is a string" echo "$string_var" string_var='This will not expand: $string_var' echo "$string_var"
For numerical operations, you can perform arithmetic directly using the $((…)) syntax. This allows you to compute expressions and store results in variables:
num1=5 num2=10 result=$((num1 + num2)) echo "The result is: $result"
Arrays in Bash can store multiple values in a single variable. They can be declared using parentheses, and you can access individual elements using their index, which starts at 0. Here’s how you can work with arrays:
my_array=("one" "two" "three") echo "First element: ${my_array[0]}" echo "All elements: ${my_array[@]}"
Bash also offers associative arrays, which use key-value pairs for storing data. They can be declared by using the declare command with the -A option:
declare -A my_associative_array my_associative_array=(["key1"]="value1" ["key2"]="value2") echo "Key1: ${my_associative_array[key1]}"
Control Structures: Conditionals and Loops
Control structures are essential in Bash scripting as they enable you to control the flow of execution based on specific conditions or to repeat tasks. This section covers the primary control structures: conditionals, which allow for decision-making, and loops, which facilitate repeated execution.
Conditionals in Bash are typically implemented using the if statement. The structure of an if statement is simpler, allowing for clear logical expressions. Here’s a basic example:
if [ condition ]; then # commands to execute if condition is true else # commands to execute if condition is false fi
For instance, suppose you want to check if a file exists before attempting to read it. You would structure your code like this:
filename="example.txt" if [ -e "$filename" ]; then echo "$filename exists." else echo "$filename does not exist." fi
In this example, the -e flag is used to check for the existence of the file. Bash provides a variety of test conditions, including checks for equality (-eq), string comparison (=), and more, allowing for versatile conditional logic.
Another common conditional structure is the case statement, ideal for matching a variable against a set of patterns:
case "$variable" in pattern1) # commands for pattern1 ;; pattern2) # commands for pattern2 ;; *) # commands if no patterns match ;; esac
This method is particularly useful for handling multiple potential values or commands based on the value of a single variable.
Next, we turn our attention to loops, which are crucial for executing a block of code multiple times. The for loop is widely utilized in Bash scripts. There are several ways to implement it, such as iterating over a list or a range of numbers:
for i in {1..5}; do echo "Iteration $i" done
In this example, the loop iterates five times, displaying the current iteration number. You can also loop through items in an array:
my_array=("apple" "banana" "cherry") for fruit in "${my_array[@]}"; do echo "Fruit: $fruit" done
Another commonly used loop is the while loop, which continues executing as long as a specified condition remains true:
count=1 while [ $count -le 5 ]; do echo "Count is $count" ((count++)) # Increment count by 1 done
Here, the loop will execute until the count variable exceeds 5, demonstrating how to manage repetitive tasks efficiently.
Additionally, there’s the until loop, which operates similarly to the while loop but continues until a condition becomes true:
count=1 until [ $count -gt 5 ]; do echo "Count is $count" ((count++)) done
Functions and Modular Scripting
Functions in Bash provide a way to encapsulate code, enabling modular scripting. This modularity allows you to write scripts that are easier to understand and maintain, as well as to reuse code across different scripts. Defining a function is simpler, requiring only the function name and a block of commands enclosed in curly braces:
my_function() { echo "This is my first function." }
To execute the function, you simply call it by name:
my_function
When functions are defined, they can accept parameters, allowing for greater flexibility. Parameters can be accessed within the function using the special variables $1, $2, and so on, which represent the first, second, etc., arguments passed to the function. Here’s an example:
greet() { echo "Hello, $1!" } greet "World"
This function `greet` takes one argument and prints a personalized greeting. When called with the value “World”, it outputs:
Hello, World!
Functions can also return values using the `return` statement. However, it’s important to note that the return statement only allows for returning an exit status (0 for success, or a non-zero value for failure). For returning values, you may want to use echo and capture the output when calling the function:
add() { local sum=$(( $1 + $2 )) echo $sum } result=$(add 5 10) echo "The sum is: $result"
In this example, the `add` function calculates the sum of two numbers and returns the result via echo. The caller captures this output into the `result` variable.
Another powerful feature of functions is local variables. By default, variables declared inside a function are global, but you can limit their scope by declaring them as local using the `local` keyword:
counter() { local count=0 ((count++)) echo "Count inside function: $count" } counter echo "Count outside function: $count"
In this case, the `count` variable is local to the `counter` function, meaning it does not affect or interfere with any variable named `count` defined outside of it.
Error Handling and Debugging Techniques
Error handling and debugging are critical aspects of writing effective Bash scripts. Without proper error management, scripts can fail silently or produce unexpected results, leading to confusion and wasted time. Learning how to handle errors gracefully and debug your scripts can dramatically improve their reliability.
One of the simplest forms of error handling in Bash is to check the exit status of commands. Every command executed in a Bash script returns an exit status, which can be accessed using the special variable `$?`. A status of `0` indicates success, while any non-zero value signifies an error. You can use this feature to ensure that a command executed successfully before proceeding:
command1 if [ $? -ne 0 ]; then echo "command1 failed!" exit 1 fi command2
This method allows you to respond to errors immediately by stopping script execution or handling the error in a specific way. You can also use the `set` command to control error handling globally. By adding `set -e` at the beginning of your script, you instruct Bash to exit immediately if any command fails:
#!/bin/bash set -e # Following commands will stop execution if any fails command1 command2
Another powerful feature for error management is the `trap` command, which allows you to specify commands to execute upon receiving signals or when an error occurs. This can be incredibly useful for cleanup operations before exiting the script:
#!/bin/bash cleanup() { echo "Cleaning up..."; } trap cleanup EXIT # Script commands here command1 command2
Debugging Bash scripts can initially seem daunting due to the lack of a built-in debugger. However, Bash provides several options to help diagnose issues. The most common method is to run the script with the `-x` option, which enables a trace mode that prints each command and its arguments as they’re executed:
#!/bin/bash set -x # Enable debugging # Commands to debug command1 command2
Additionally, you can insert `set -x` and `set +x` around sections of your script to selectively enable tracing for specific blocks of code:
#!/bin/bash echo "Before debugging" set -x command1 command2 set +x echo "After debugging"
Another useful debugging technique is using `echo` statements to output variable values and script milestones. This method can help clarify the script’s flow and identify where things might be going wrong:
my_variable="Hello" echo "Value of my_variable: $my_variable" # Other commands
For more complex debugging, think using the `PS4` variable to customize the prompt displayed during tracing. By default, the prompt is a `+`, but you can change it to include more context, such as line numbers:
#!/bin/bash export PS4='+$BASH_SOURCE:$LINENO: ' set -x command1 command2
Best Practices for Writing Clean Scripts
Writing clean scripts in Bash is fundamental not only for the sake of readability but also for maintainability and efficiency. Clean scripts are easier to understand, debug, and enhance, reducing the overall time spent on development and troubleshooting. Here are some best practices to keep in mind when crafting your Bash scripts.
1. Use Meaningful Variable Names: Choose variable names that clearly convey their purpose. Avoid single-letter variable names unless used in loops or as temporary placeholders. For example:
file_count=0 user_input=""
These names provide context, helping anyone reading the script (including your future self) understand what the variables represent.
2. Comment Liberally: Use comments to explain the purpose of complex commands, loops, and functions. Good comments help demystify your logic. For example:
# Check if the directory exists if [ -d "$directory" ]; then echo "Directory exists." else echo "Creating directory." mkdir "$directory" fi
Comments should clarify the intent of the code, especially for non-obvious logic. However, avoid stating the obvious in comments, as this clutters the script.
3. Organize Code into Functions: As mentioned previously, functions allow for modular design. Group related commands into functions, which can make scripts shorter and cleaner. For instance:
process_files() { for file in "$@"; do echo "Processing $file" # Add processing logic here done } process_files *.txt
This abstraction not only simplifies the main script flow but also enhances reusability.
4. Follow Consistent Formatting: Consistency in formatting improves readability. Use consistent indentation, spacing, and line breaks. For instance, always place brackets and parentheses with a space before and after:
if [ "$value" -eq 1 ]; then echo "Value is one." fi
Making these formatting choices consistently throughout your script will allow others to read your code intuitively.
5. Handle Errors Gracefully: Implement error handling to capture and respond to potential issues gracefully. For example, using `set -e` at the beginning of your script can prevent it from continuing after a command fails:
#!/bin/bash set -e # Command that could fail rm "$file" echo "File removed."
You can also use conditional checks after commands to manage error states specifically, providing clear feedback to users.
6. Utilize Command-Line Arguments: Allow your scripts to take command-line arguments for flexibility. Use `getopts` for parsing options, which enhances script usability:
while getopts ":f:n:" opt; do case $opt in f) file="$OPTARG" ;; n) number="$OPTARG" ;; ?) echo "Invalid option -$OPTARG" >&2 ;; esac done
This approach allows users to customize script behavior at runtime, making your scripts more versatile.
7. Test Your Scripts: Always test your scripts in a safe environment before deploying them. Incorporate debugging options and logs to help trace issues:
set -x # Enable debugging # Your script commands set +x # Disable debugging