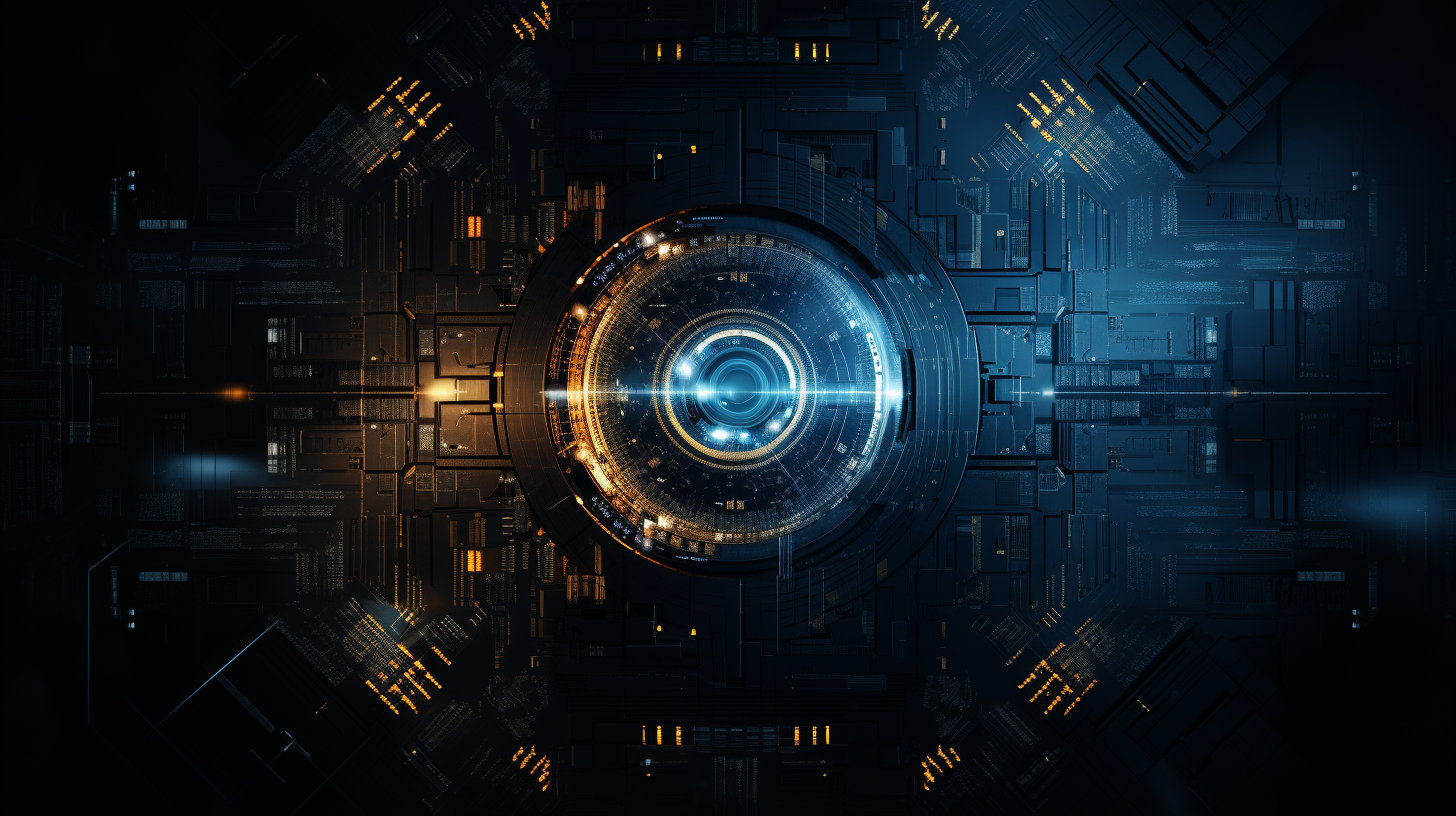
Bash Scripting Best Practices
Within the scope of Bash scripting, adhering to conventions and standards is important for ensuring that scripts are not only functional but also maintainable and comprehensible by others (or even by yourself at a later date). Following a consistent naming scheme for variables, functions, and files can significantly enhance the readability of your scripts.
Variable Names: Use descriptive names that convey the purpose of the variable. A good convention is to use lowercase letters, with underscores to separate words. For example:
my_variable_name="value"
Function Names: Similarly, function names should be descriptive and use the same casing convention. Prefixing function names with a verb can help clarify their actions. For instance:
function calculate_sum() { # function body }
File Names: When naming your script files, it is advantageous to use lowercase letters and separate words with hyphens or underscores. This way, you can quickly identify the purpose of the script just by looking at its name:
my-bash-script.sh
Another important convention is the use of comments. Comments should be used liberally to explain the logic behind complex operations or to denote sections of the script. Use the #
symbol for single-line comments:
# That is a single-line comment
For longer explanations, multi-line comments can be created by placing : ' ... '
around the block of text:
: ' That's a multi-line comment that explains the following section of the script. '
Additionally, adhering to indentation and spacing conventions fosters better readability. Nesting should be clearly indented to visually represent the structure of the script:
if [[ condition ]]; then # Do something for i in {1..5}; do echo "Number: $i" done fi
Exit Status: Every Bash command returns an exit status. It’s essential to check these statuses, particularly when executing commands that can fail. Utilize the trap
command to catch errors and handle them gracefully:
trap 'echo "An error occurred. Exiting..."; exit 1;' ERR
Error Handling and Debugging Techniques
When it comes to error handling and debugging in Bash scripting, the strategies you employ can make a world of difference in the robustness and reliability of your scripts. Bash provides a variety of tools and techniques to gracefully manage errors, so that you can anticipate and respond to issues that may arise during script execution.
One of the foundational concepts in error handling is understanding exit statuses. Every command you run in a Bash script returns an exit code, where a code of 0 typically indicates success, while any non-zero code signifies an error. To check the exit status of the last command executed, you can use the special variable $?
. For instance:
some_command if [[ $? -ne 0 ]]; then echo "Command failed!" fi
Using this technique throughout your script can help flag errors immediately, which will allow you to take corrective action as needed. However, for more comprehensive error handling, you can incorporate the trap
command, which allows you to define a command to be executed when the script exits, whether due to an error or a normal exit. Here’s how you might set this up:
trap 'echo "An error occurred. Exiting..."; exit 1;' ERR
This command sets a trap for any command failure (ERR), ensuring that your script provides a useful error message before exiting. You can also specify cleanup tasks or logging operations within the trap to ensure that resources are released or states are logged adequately.
Another useful technique in debugging is using the set
command to enable debugging options. The -x
option can be instrumental in tracing what your script is doing, as it prints each command along with its arguments to the terminal before executing it. This can be done at the beginning of your script:
set -x
To disable this tracing, you can use set +x
. That is particularly helpful in isolating where a problem might be occurring in your script.
Another approach that can enhance your debugging process is to use the echo
command strategically to output variable values or checkpoints within your script. By sprinkling echo
statements throughout, you can gain insight into how data is flowing through your script:
echo "Current value of my_variable: $my_variable"
However, excessive use of echo
can clutter your output, so consider using a debug flag to control whether these messages are printed, enabling you to toggle verbosity on and off as needed.
Finally, think implementing logging mechanisms to capture output and errors into a log file. This can help you review script behavior after execution without wading through terminal output:
exec > >(tee -a script.log) 2>&1
This command redirects both standard output and standard error to a log file, which can be invaluable for diagnosing issues that occur during execution.
Optimizing Performance and Efficiency
When it comes to optimizing performance and efficiency in Bash scripts, one must think various aspects that can significantly enhance execution speed and resource usage. Bash, while powerful, can sometimes be less efficient than other programming environments; however, with a few smart practices, you can maximize performance.
First and foremost, it is essential to minimize the use of external commands. Each call to an external command incurs overhead, hence, wherever possible, leverage built-in Bash functionality. For example, instead of using the external grep
command to filter a list, you can use built-in pattern matching:
for item in "${array[@]}"; do [[ $item == *pattern* ]] && echo "$item" done
This approach significantly reduces the time taken to execute the script by avoiding the overhead associated with launching external processes.
Another effective optimization technique is to minimize subshell usage. Subshells can be created when you use parentheses or certain commands like $(...)
. This can lead to increased memory usage and performance bottlenecks. Instead, try to use constructs that avoid subshells, such as using arrays and loops directly:
array=(one two three) for item in "${array[@]}"; do echo "$item" done
Moreover, when dealing with large files or data sets, ponder using built-in string manipulation features instead of external tools. For example, to extract parts of a string, you can use parameter expansion:
filename="example.txt" base="${filename%.*}" echo "Base name: $base"
When working with loops, be mindful of the loop structure. Using a while
loop with read can be more efficient than a for
loop when processing lines from a file, particularly for large files:
while IFS= read -r line; do echo "Processing: $line" done < input_file.txt
Optimization also extends to arithmetic operations. Bash performs arithmetic operations in a suboptimal manner using external commands when it can use built-in arithmetic expansion. For instance:
result=$((a + b)) echo "Result: $result"
Furthermore, ponder the use of associative arrays for scenarios requiring fast lookups. Associative arrays offer a time-efficient way to handle key-value pairs compared to traditional arrays:
declare -A colors colors=( ["red"]="#FF0000" ["green"]="#00FF00" ["blue"]="#0000FF" ) echo "The color red is ${colors[red]}"
Another significant factor in improving performance is reducing the number of times you read the environment or external resources. Caching values that are accessed frequently can save time in scripts that require repeated access to the same data:
cached_value=$(command_to_get_value) echo "Cached value is: $cached_value" # Use $cached_value in further processing
Finally, always profile your scripts. The time
command is helpful for measuring the duration of script execution, and you can use it to identify bottlenecks:
time ./your_script.sh
Maintaining Readability and Documentation
Maintaining readability and documentation in your Bash scripts is essential for fostering an environment of collaboration and efficient code management. A well-documented script is not only easier to understand but also significantly reduces the time spent diagnosing issues or enhancing functionality later on. Here are several crucial practices to keep in mind.
Use Clear Comments: Comments are critical in explaining the purpose and functionality of your code. They should clarify not just the “what,” but also the “why” behind your code decisions. Use inline comments to explain complex logic:
# Check if the user is root before proceeding if [[ $EUID -ne 0 ]]; then echo "This script must be run as root" exit 1 fi
When documenting sections of your script, particularly lengthy or complex blocks, consider adding a comment block at the beginning of that section:
: ' This section handles the backup of user data by compressing the /home directory and storing it in the /backup directory. ' tar -czf /backup/home_backup.tar.gz /home
Consistent Formatting: Consistency in formatting is vital for readability. This includes consistent indentation, spacing, and line breaks. A common convention is to use 4 spaces for indentation. Here’s an example of how clear formatting can enhance readability:
if [[ -f "config.txt" ]]; then # Load configuration settings source config.txt else echo "Configuration file not found!" exit 1 fi
Additionally, you should structure your scripts in a way that reflects their logical flow. Group related functions together and separate them from the main execution logic to enhance clarity.
Function Documentation: Each function in your script should begin with a comment block explaining its purpose, parameters, and return values. That is especially useful in larger scripts where functions may be reused or modified:
# Function to calculate the factorial of a number # Arguments: # $1 - The number to calculate the factorial for # Returns: # The factorial of the given number function factorial() { local num=$1 local result=1 for ((i=1; i<=num; i++)); do result=$((result * i)) done echo $result }
Use Descriptive Variable Names: As previously mentioned, the names of your variables should reflect their purpose. This not only aids in understanding the code but also helps avoid confusion. For example, instead of using cryptic names like `x` or `temp`, use names like `backup_directory` or `user_count`:
backup_directory="/backup" user_count=$(wc -l < /home/users.txt)
Structure and Organization: A well-structured script can significantly improve readability. Ponder breaking large scripts into smaller, modular functions that each perform a distinct task. This not only enhances readability but also makes testing and debugging individual components easier:
function setup_environment() { echo "Setting up environment variables..." # Set necessary variables } function execute_tasks() { echo "Executing main tasks..." # Main execution logic } setup_environment execute_tasks
Documentation Outside the Script: In addition to inline comments and documentation within the script, consider maintaining external documentation, such as a README file. This can provide an overview of the script’s functionality, usage examples, and dependencies. This way, someone new to the script can quickly grasp its purpose and how to use it effectively.