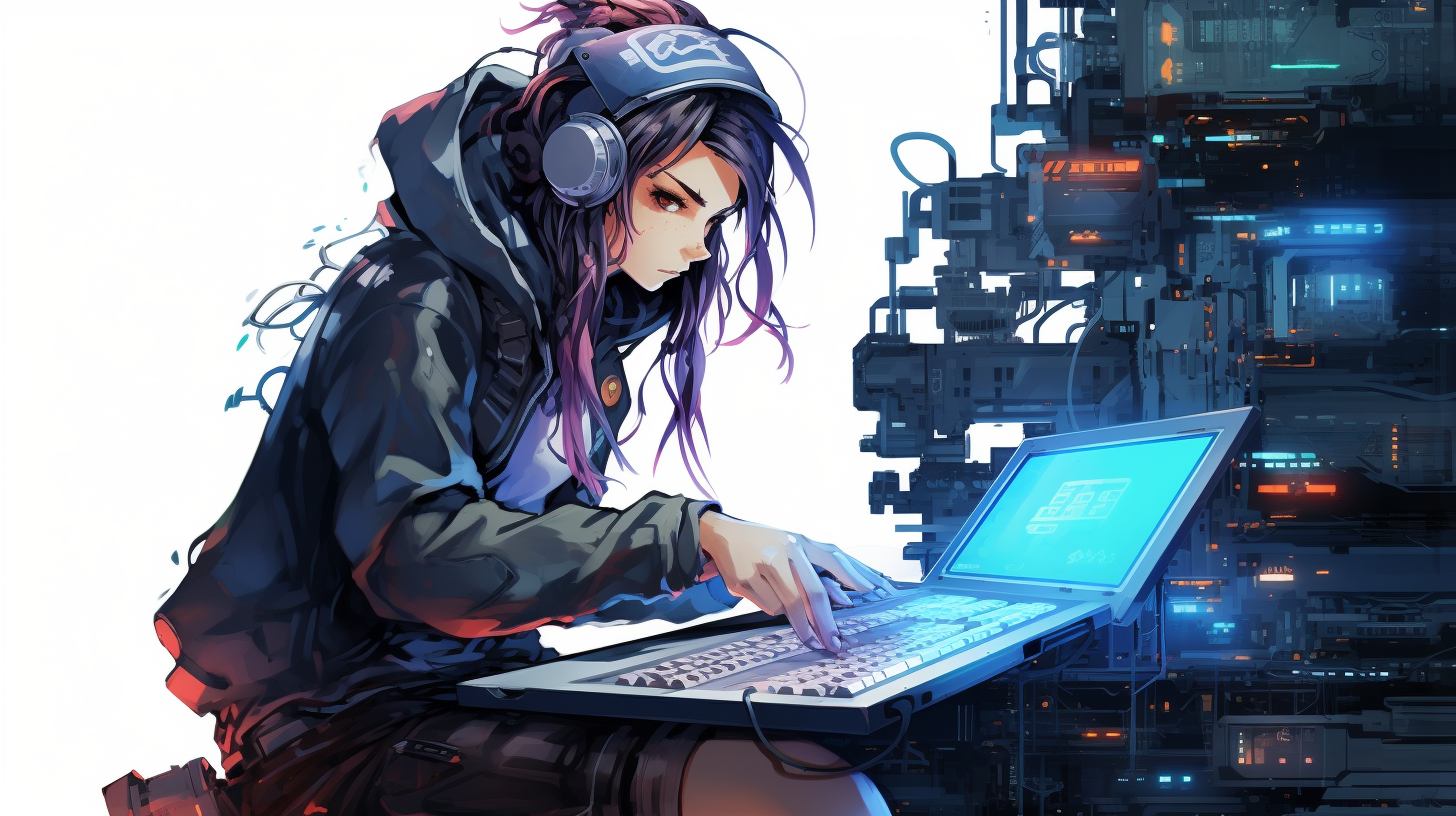
Bash Scripting for Backup Operations
Bash scripting is a powerful tool for automating backup operations in a Unix-like environment. Understanding the fundamentals of Bash is essential for creating effective backup scripts that ensure data security and accessibility. At its core, Bash scripting allows users to write sequences of commands that can be executed together, making it ideal for repetitive tasks such as backups.
To effectively use Bash for backup operations, one should be familiar with basic concepts such as variables, control structures, and functions. Variables in Bash are declared using the =
operator, and they can store paths, filenames, or any other data necessary for the operation. For example:
BACKUP_DIR="/path/to/backup"
Control structures, such as if
statements and loops, allow for conditional execution and iteration, enabling more robust scripts. Here’s how to check if a directory exists before attempting to back it up:
if [ -d "$BACKUP_DIR" ]; then echo "Backup directory exists." else echo "Creating backup directory." mkdir -p "$BACKUP_DIR" fi
Another crucial aspect is using functions to encapsulate logic that can be reused throughout your script. This not only makes your scripts cleaner but also aids in maintenance. Here’s a simple function that performs the actual backup using the tar
command:
backup() { tar -czf "$BACKUP_DIR/backup_$(date +%F).tar.gz" /path/to/data echo "Backup completed successfully." }
In this function, the tar
command creates a compressed archive of the specified data directory, appending the current date to the filename for easy identification. Using date formatting in filenames is a best practice, as it keeps backups organized and prevents overwriting previous archives.
Additionally, it’s important to understand the execution environment of a Bash script. Scripts need to have executable permissions, which can be set with the chmod
command:
chmod +x my_backup_script.sh
Finally, it’s vital to test your scripts rigorously. You can run a script in a “dry run” mode or simulate the commands with echo statements to ensure they perform as expected before executing them on live data. This minimizes the risk of data loss during backup operations.
By mastering these basic elements of Bash scripting, you set the foundation for creating more sophisticated backup solutions tailored to your specific needs.
Choosing the Right Backup Strategy
Choosing the right backup strategy is an important step in ensuring the safety and integrity of your data. There are various approaches to backup, and each has its distinct advantages and disadvantages. Understanding these strategies will help you select the most suitable one for your specific environment and requirements.
One common strategy is the full backup, where all selected data is copied concurrently. This method provides a complete snapshot of your data at a specific point in time, making it simple to restore in case of data loss. However, full backups can be time-consuming and consume significant storage space. For example, a simple script to perform a full backup might look like this:
#!/bin/bash BACKUP_DIR="/path/to/backup" SOURCE_DIR="/path/to/data" tar -czf "$BACKUP_DIR/full_backup_$(date +%F).tar.gz" "$SOURCE_DIR" echo "Full backup completed successfully."
Incremental backups, on the other hand, only save changes made since the last backup. This approach is more efficient in terms of time and storage, but restoring data can be more complicated, as it may require the last full backup plus all subsequent incremental backups. Here’s an example script to perform an incremental backup:
#!/bin/bash BACKUP_DIR="/path/to/backup" SOURCE_DIR="/path/to/data" INCREMENTAL_FILE="$BACKUP_DIR/incremental_scn" tar -czf "$BACKUP_DIR/incremental_backup_$(date +%F).tar.gz" --newer="$INCREMENTAL_FILE" "$SOURCE_DIR" touch "$INCREMENTAL_FILE" echo "Incremental backup completed successfully."
Differential backups combine aspects of both full and incremental backups. They store changes since the last full backup, making restoration quicker than with incremental backups but still consuming more space than incremental ones. An example script for a differential backup could be:
#!/bin/bash BACKUP_DIR="/path/to/backup" SOURCE_DIR="/path/to/data" LAST_FULL_BACKUP="$BACKUP_DIR/full_backup_YYYY-MM-DD.tar.gz" tar -czf "$BACKUP_DIR/differential_backup_$(date +%F).tar.gz" --newer="$LAST_FULL_BACKUP" "$SOURCE_DIR" echo "Differential backup completed successfully."
In addition to choosing a backup type, think the frequency of your backups based on how often data changes. Daily backups might be necessary for environments with frequent updates, while weekly backups may suffice for static data. Furthermore, always ensure that backups are stored in a secure location, preferably offsite or in the cloud, to protect against physical disasters.
Lastly, it’s essential to have a tested recovery plan in place. Regularly practice restoring from your backups to ensure that, in the event of data loss, you can quickly and confidently recover your files. This proactive approach not only safeguards your data but also reduces downtime and potential losses in critical situations.
Creating a Simple Backup Script
Creating a simple backup script is an essential step in automating your backup operations with Bash. The simplicity of Bash allows you to quickly set up a script that can save critical data with minimal effort. The following sections break down the components of a basic backup script, which can be tailored to fit your specific needs.
The first step is to define the necessary variables that will hold your source directory and backup destination. This modular approach makes it easy to modify paths without altering the core logic of your script. Here’s a basic template:
#!/bin/bash # Define backup directory and source directory BACKUP_DIR="/path/to/backup" SOURCE_DIR="/path/to/data"
Next, it’s prudent to check if the backup directory exists. If not, the script can create it automatically. This automation ensures that your script runs smoothly without manual intervention:
# Check if backup directory exists, create if it doesn't if [ ! -d "$BACKUP_DIR" ]; then echo "Backup directory does not exist. Creating it now." mkdir -p "$BACKUP_DIR" else echo "Backup directory already exists." fi
Once the necessary directories are in place, the next step is to perform the actual backup operation. The tar command is a powerful utility for creating archive files. You can use it to compress your source directory into a single file, which not only saves space but also simplifies the backup process:
# Create the backup using tar BACKUP_FILE="$BACKUP_DIR/backup_$(date +%F).tar.gz" echo "Starting backup..." tar -czf "$BACKUP_FILE" "$SOURCE_DIR" echo "Backup completed successfully. Backup file is located at: $BACKUP_FILE"
Finally, it’s essential to include some basic logging to keep track of the backup operations. This can be done by appending the output of your backup process to a log file. This practice helps in audits and troubleshooting:
# Log the backup operation LOG_FILE="$BACKUP_DIR/backup.log" echo "$(date +'%Y-%m-%d %H:%M:%S') - Backup created: $BACKUP_FILE" >> "$LOG_FILE"
Putting it all together, here’s the complete script:
#!/bin/bash # Define backup directory and source directory BACKUP_DIR="/path/to/backup" SOURCE_DIR="/path/to/data" # Check if backup directory exists, create if it doesn't if [ ! -d "$BACKUP_DIR" ]; then echo "Backup directory does not exist. Creating it now." mkdir -p "$BACKUP_DIR" else echo "Backup directory already exists." fi # Create the backup using tar BACKUP_FILE="$BACKUP_DIR/backup_$(date +%F).tar.gz" echo "Starting backup..." tar -czf "$BACKUP_FILE" "$SOURCE_DIR" echo "Backup completed successfully. Backup file is located at: $BACKUP_FILE" # Log the backup operation LOG_FILE="$BACKUP_DIR/backup.log" echo "$(date +'%Y-%m-%d %H:%M:%S') - Backup created: $BACKUP_FILE" >> "$LOG_FILE"
With this script, you’ve automated the creation of a backup with error handling and logging. Make sure to test your script in a safe environment to confirm that it runs correctly and meets your backup needs before deploying it in a live setting. This foundational script can be expanded further with additional features such as email notifications or automated scheduling, paving the way for more sophisticated backup solutions.
Automating Backups with Cron Jobs
Automating backups with Cron jobs is an important step in ensuring that your backup operations run smoothly and consistently without requiring manual intervention. Cron is a time-based job scheduler in Unix-like operating systems, allowing you to schedule scripts to run at specified intervals, making it an ideal tool for handling backups.
To begin, you need to familiarize yourself with the Cron syntax. A typical Cron job entry consists of six fields:
* * * * * /path/to/command - - - - - | | | | | | | | | +---- Day of the week (0 - 7) (Sunday is both 0 and 7) | | | +------ Month (1 - 12) | | +-------- Day of the month (1 - 31) | +---------- Hour (0 - 23) +------------ Minute (0 - 59)
For example, if you want to schedule your backup script to run every day at 2 AM, you would add the following line to the Cron configuration:
0 2 * * * /path/to/my_backup_script.sh
This entry instructs Cron to execute the my_backup_script.sh
script daily at 2 AM. To modify your Cron jobs, you can use the crontab -e
command, which opens the Cron table for editing. Remember that each user has their own Cron table, and using sudo crontab -e
allows you to edit the root user’s Cron jobs.
It is important to ensure that your backup script has the appropriate permissions to execute, as previously mentioned. You can set the executable permission with:
chmod +x /path/to/my_backup_script.sh
After setting up your Cron job, it’s prudent to verify that it is running as expected. You can check the system mail or log files for output from Cron jobs. By default, Cron sends an email to the user account that owns the job for any output produced by the script, including errors. If your system is not configured to send emails, you can redirect the output of your script to a log file instead:
0 2 * * * /path/to/my_backup_script.sh >> /path/to/backup/backup.log 2>&1
This command appends both the standard output and standard error of your backup script to backup.log
, allowing you to review the log for any issues that may arise during execution.
When automating backups, think implementing a strategy for managing old backups. For instance, you may want to delete backups older than a certain number of days to conserve storage space. This can be accomplished using the find
command within your backup script:
find /path/to/backup -type f -name "*.tar.gz" -mtime +30 -exec rm {} ;
This command finds and removes any compressed backup files older than 30 days, ensuring that your backup directory does not grow indefinitely.
By using Cron jobs in your backup strategy, you create a seamless and automated process that significantly reduces the risk of data loss. By scheduling regular backups and implementing logging and retention policies, you can maintain a reliable backup regimen that operates with minimal oversight, which will allow you to focus on other critical tasks.
Error Handling and Logging in Backup Scripts
Error handling and logging in backup scripts are essential for ensuring that your backup operations not only succeed but also provide you with the necessary insights to diagnose issues when they arise. A well-crafted script will not only perform the backup but will also manage errors gracefully and maintain a log for future reference.
When writing a backup script, it’s crucial to anticipate potential failure points. For example, the script should check if the source directory exists before attempting to back it up. If the source directory does not exist, the script should exit early, preventing further failure. Here’s how you can implement such a check:
#!/bin/bash BACKUP_DIR="/path/to/backup" SOURCE_DIR="/path/to/data" # Check if source directory exists if [ ! -d "$SOURCE_DIR" ]; then echo "Error: Source directory $SOURCE_DIR does not exist." >&2 exit 1 fi
In the above snippet, the script checks for the existence of the source directory. If it doesn’t exist, an error message is printed to standard error (using >&2), and the script exits with a non-zero status, signaling an error.
Another common error to handle is the failure of the backup command itself. The tar command can fail for various reasons, such as insufficient disk space or permissions issues. To handle this, you can check the exit status of the tar command right after it is executed:
# Create the backup using tar BACKUP_FILE="$BACKUP_DIR/backup_$(date +%F).tar.gz" echo "Starting backup..." if tar -czf "$BACKUP_FILE" "$SOURCE_DIR"; then echo "Backup completed successfully. Backup file is located at: $BACKUP_FILE" else echo "Error: Backup failed!" >&2 exit 2 fi
In this example, the script uses an if statement to determine whether the tar command was successful. If the command fails, an error message is printed, and the script exits with a different non-zero status to indicate a backup failure.
Logging is also a critical component of error handling. It allows you to review what happened during the backup process, which is invaluable when troubleshooting. You can append messages to a log file throughout your script. For instance:
LOG_FILE="$BACKUP_DIR/backup.log" echo "$(date +'%Y-%m-%d %H:%M:%S') - Starting backup..." >> "$LOG_FILE" if tar -czf "$BACKUP_FILE" "$SOURCE_DIR"; then echo "$(date +'%Y-%m-%d %H:%M:%S') - Backup completed successfully: $BACKUP_FILE" >> "$LOG_FILE" else echo "$(date +'%Y-%m-%d %H:%M:%S') - Error: Backup failed!" >> "$LOG_FILE" exit 2 fi
In this case, every significant action in the script is logged with a timestamp, which helps in understanding the sequence of events leading up to any potential issues.
Moreover, you may also want to log the exit status of the script itself. This can be useful for any automated monitoring systems that track the success or failure of scheduled backups:
# At the end of your script if [ $? -eq 0 ]; then echo "$(date +'%Y-%m-%d %H:%M:%S') - Script completed successfully." >> "$LOG_FILE" else echo "$(date +'%Y-%m-%d %H:%M:%S') - Script exited with errors." >> "$LOG_FILE" fi
By implementing these error handling and logging strategies, you create a robust backup script that not only performs its function but also provides clear and actionable feedback when things go wrong. This level of detail is what separates a simple script from a professional tool, making it invaluable in a production environment.
Restoring Data from Backup Archives
Restoring data from backup archives is a critical operation that ensures data integrity and recoverability in case of data loss. When the time comes to retrieve lost or corrupted files, having a clear and efficient restoration process in place is essential. In Bash, the restoration process typically involves using the tar command to extract files from the backup archives created during the backup operations.
To begin, you’ll first want to identify the backup archive from which you wish to restore data. It’s a good practice to keep a clear naming convention for your backup files that includes date information. This way, you can easily locate the backup you need. For example, you might have a backup file named backup_2023-10-01.tar.gz.
Once you have identified the backup file, you can use the tar command to extract its contents. The basic syntax for restoring files from a tar archive is as follows:
tar -xzvf /path/to/backup/backup_YYYY-MM-DD.tar.gz -C /path/to/restore
In this command:
- Tells tar to extract files.
- Instructs tar to decompress the archive using gzip.
- Provides verbose output, showing the files being extracted.
- Specifies the filename of the archive to be extracted.
- Changes to the specified directory before extracting files, enabling you to specify where the files should be restored.
Here’s a practical example of a restoration script that could be used to automate the extraction of files from a specified backup archive:
#!/bin/bash # Specify the backup file and the target restore location BACKUP_FILE="/path/to/backup/backup_$(date +%F).tar.gz" RESTORE_DIR="/path/to/restore" # Check if the backup file exists if [ ! -f "$BACKUP_FILE" ]; then echo "Error: Backup file $BACKUP_FILE does not exist." >&2 exit 1 fi # Create the restore directory if it doesn't exist if [ ! -d "$RESTORE_DIR" ]; then mkdir -p "$RESTORE_DIR" fi # Extract the files from the backup archive echo "Restoring files from $BACKUP_FILE to $RESTORE_DIR..." tar -xzvf "$BACKUP_FILE" -C "$RESTORE_DIR" if [ $? -eq 0 ]; then echo "Restoration completed successfully." else echo "Error: Restoration failed!" >&2 exit 2 fi
In this script, we first check if the specified backup file exists. If not, an error message is printed, and the script exits. If the backup file is present, we ensure that the target restore directory exists, creating it if necessary. The actual restoration command is executed, and upon completion, a message is displayed indicating whether the operation was successful or if it encountered issues.
Additionally, it’s important to consider the context in which you’re restoring data. Be aware of potential overwrites: if files in the restore directory already exist, you may unintentionally overwrite them. To prevent this, you could either restore to a new directory or implement checks within your script to handle existing files appropriately.
With this knowledge, you can confidently create restoration scripts to retrieve your data efficiently. Ensuring that you have a reliable and tested restoration process very important in any backup strategy, preventing downtime and minimizing the impact of data loss.