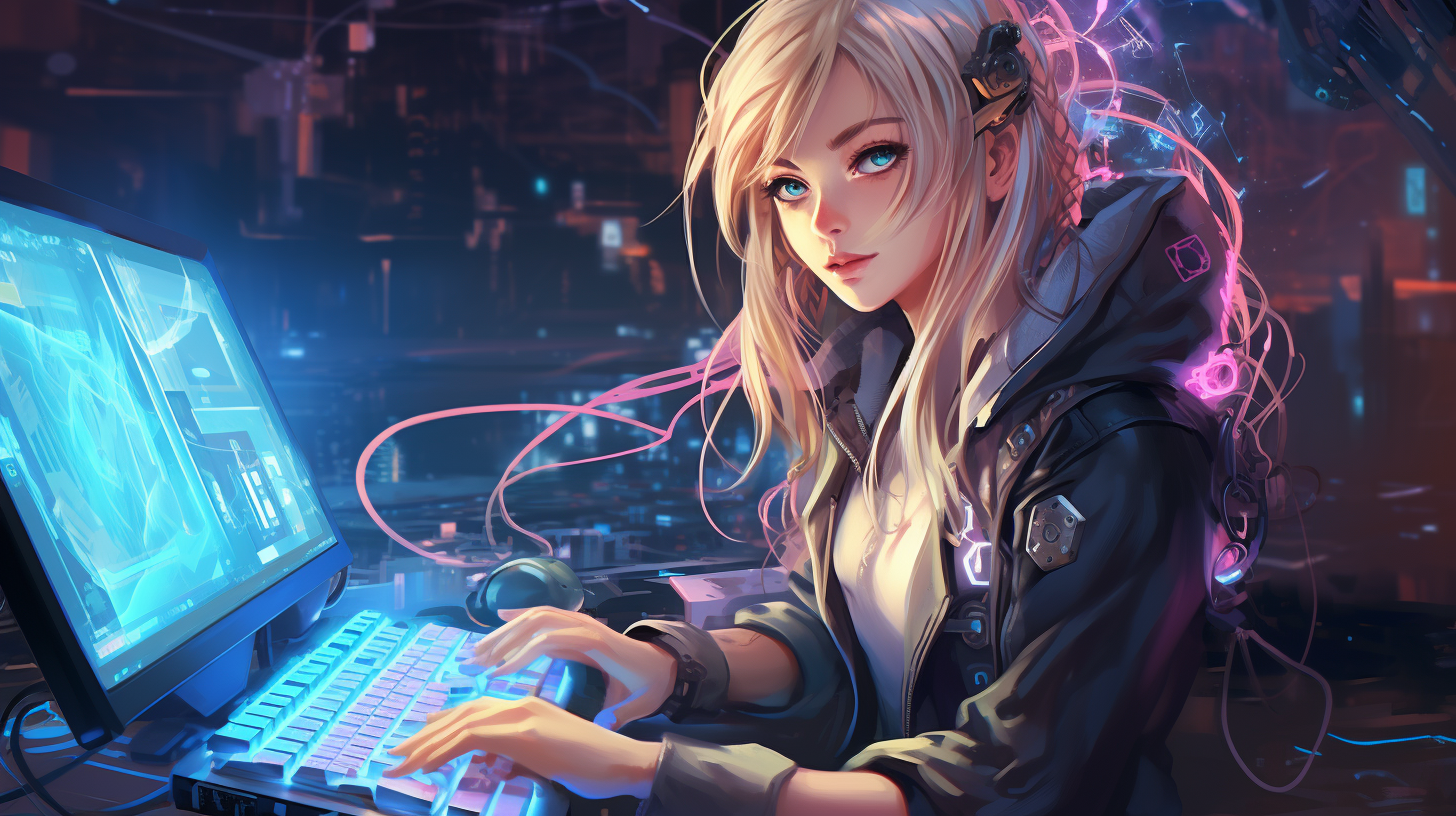
Bash Scripting for Cloud Operations
In the sphere of cloud operations, mastering Bash fundamentals is an essential stepping stone towards automating tasks efficiently. Bash, a powerful shell scripting language, allows you to interface with and control various cloud services seamlessly. Understanding its basic constructs forms the backbone of effective automation.
Bash scripting relies heavily on its syntax and structure. A typical Bash script begins with a shebang line, which tells the system what interpreter to use for executing the script. For Bash, this line looks like:
#!/bin/bash
Variables are a fundamental concept in Bash scripting. You can store and manipulate data using simple assignment operations. Here’s how you can define and use a variable:
my_variable="Hello, Cloud!" echo $my_variable
Control structures, such as loops and conditionals, allow you to create scripts that perform actions based on specific criteria. For instance, implementing a conditional statement can be achieved as follows:
if [ "$my_variable" == "Hello, Cloud!" ]; then echo "Welcome to Cloud Automation!" fi
Loops, such as for-loops and while-loops, enable you to iterate over items or execute a block of code repeatedly. A simple for-loop looks like this:
for i in {1..5}; do echo "Iteration $i" done
Input and output redirection is another powerful feature in Bash, which will allow you to handle data streams effectively. For instance, you can redirect the output of a command to a file using:
echo "Storing this output" > output.txt
Moreover, functions are crucial for reusability and organization of your scripts. Defining a function can enhance the clarity and efficiency of your codebase. Below is how you define and invoke a function in Bash:
my_function() { echo "This is a custom function!" } my_function
In cloud automation, interacting with APIs is a common task. You can leverage tools like curl
to make API requests directly from your Bash scripts. Here’s a simple example of how to fetch data using curl
:
response=$(curl -s https://api.example.com/data) echo "API Response: $response"
By laying a solid foundation in Bash through these fundamental concepts, you will be well-equipped to tackle the complexities of cloud automation, enabling you to streamline operations and enhance productivity.
Managing Cloud Resources with Bash Scripts
When it comes to managing cloud resources with Bash scripts, the ability to automate and orchestrate tasks becomes a tremendous advantage. Cloud providers typically offer APIs to interact programmatically with their services, which will allow you to create, modify, and delete resources directly from your scripts. This capability allows you to streamline operations and drastically reduce the time needed to perform repetitive tasks.
To interact effectively with cloud services, you’ll often find yourself using command-line tools specific to the cloud provider, such as aws
for Amazon Web Services, az
for Microsoft Azure, and gcloud
for Google Cloud Platform. These tools can be invoked directly from your Bash scripts to manage resources efficiently.
For example, if you want to create an EC2 instance in AWS, you can write a Bash script that uses the aws
CLI as follows:
#!/bin/bash # Create an EC2 instance using AWS CLI INSTANCE_TYPE="t2.micro" KEY_NAME="my-key-pair" IMAGE_ID="ami-0abcdef1234567890" aws ec2 run-instances --image-id $IMAGE_ID --count 1 --instance-type $INSTANCE_TYPE --key-name $KEY_NAME
In this script, we define the instance type, key name, and the Amazon Machine Image (AMI) ID, then call the aws ec2 run-instances
command to create an instance. The parameters for the command can be adjusted based on your requirements, such as adding tags or configuring security groups.
Managing existing resources is equally important. You may need to retrieve information about your instances or perform operations like stopping or terminating them. Below is an example script that lists all running EC2 instances:
#!/bin/bash # List all running EC2 instances echo "Running EC2 Instances:" aws ec2 describe-instances --query "Reservations[*].Instances[*].[InstanceId,State.Name]" --filters "Name=instance-state-name,Values=running" --output table
This command utilizes the aws ec2 describe-instances
function to retrieve information and formats the output as a readable table, displaying the instance IDs and their respective states.
In addition to managing individual resources, you can also automate workflows that involve multiple resources and services. For example, suppose you want to create a virtual machine and set up a security group in AWS. You can sequence multiple commands within a single script:
#!/bin/bash # Create a security group and an EC2 instance SECURITY_GROUP_NAME="my-security-group" # Create security group aws ec2 create-security-group --group-name $SECURITY_GROUP_NAME --description "My Security Group" aws ec2 authorize-security-group-ingress --group-name $SECURITY_GROUP_NAME --protocol tcp --port 22 --cidr 0.0.0.0/0 # Launch EC2 instance INSTANCE_TYPE="t2.micro" KEY_NAME="my-key-pair" IMAGE_ID="ami-0abcdef1234567890" aws ec2 run-instances --image-id $IMAGE_ID --count 1 --instance-type $INSTANCE_TYPE --key-name $KEY_NAME --security-groups $SECURITY_GROUP_NAME
This script first creates a security group and allows SSH access from any IP address. Then, it launches an EC2 instance associated with that security group. Such automation not only saves time but also ensures consistency across deployments.
As you develop your Bash scripts for cloud resource management, ponder incorporating logging and monitoring functionalities. This practice will help you track script executions and troubleshoot any issues that arise. A simple way to implement logging is to redirect output to a log file:
#!/bin/bash # Example script with logging LOGFILE="script.log" echo "Starting script execution..." >> $LOGFILE # Your cloud commands go here echo "Script execution completed." >> $LOGFILE
By appending messages to the log file, you can maintain a record of your script’s activity, which is invaluable for debugging and understanding resource changes over time.
Error Handling and Debugging Techniques
Error handling and debugging are critical components of effective Bash scripting, especially in the context of cloud automation where the stakes can be high. When automating cloud operations, scripts can fail for various reasons, such as network issues, misconfigured commands, or unexpected API responses. Therefore, building robust error handling mechanisms into your scripts is essential to ensure reliability and maintainability.
An effective way to manage errors in Bash is by using the exit status of commands. In Bash, each command returns an exit status, with a value of 0 indicating success, and any non-zero value indicating an error. You can check these statuses using the special variable $?
immediately after a command executes. Here’s an example:
#!/bin/bash # Check if a command was successful mkdir /some/directory if [ $? -ne 0 ]; then echo "Failed to create directory!" >&2 exit 1 fi echo "Directory created successfully."
In this snippet, we attempt to create a directory. If the command fails (i.e., it returns a non-zero exit status), we print an error message to standard error and exit the script with a status of 1.
Another powerful feature for error handling is the use of trap
command, which allows you to specify commands that will run when the script receives specific signals or exits unexpectedly. This can be invaluable for cleanup operations, such as removing temporary files or gracefully shutting down processes:
#!/bin/bash # Trap signals and clean up cleanup() { echo "Cleaning up temporary files..." rm -f /tmp/mytempfile } trap cleanup EXIT # Script logic here echo "Running script..." # Simulate a command that may fail sleep 1 exit 0
In this example, the cleanup
function is called when the script exits, regardless of whether it exits normally or due to an error.
For debugging purposes, you can introduce debugging options to your scripts. The -x
option can be particularly useful, as it prints each command and its arguments as they are executed. You can enable this by adding the following line at the top of your script:
#!/bin/bash set -x # Your script logic here
This will generate verbose output that can help trace the flow of execution and pinpoint where errors occur.
Additionally, ponder using logging mechanisms to track runtime information. By directing output to a log file, you can maintain a history of operations that can be reviewed later, aiding in troubleshooting:
#!/bin/bash # Logging example LOGFILE="script.log" exec > >(tee -a $LOGFILE) 2>&1 echo "Starting script execution..." # Simulate processing if ! command_that_might_fail; then echo "An error occurred!" >&2 exit 1 fi echo "Script execution completed."
Here, the output and error messages are both logged to script.log
, providing a comprehensive log of actions taken during script execution.
Employing effective error handling and debugging techniques in Bash scripting will not only enhance the reliability of your scripts but will also streamline the development process by making it easier to diagnose issues. As you delve deeper into cloud automation with Bash, integrating these practices will be invaluable in ensuring your scripts perform consistently and correctly.
Best Practices for Writing Maintainable Bash Scripts
When writing Bash scripts, especially in the context of cloud automation, adhering to best practices is important to ensure that your scripts remain maintainable, understandable, and scalable over time. By following certain conventions and strategies, you can significantly reduce the complexity associated with managing scripts, making it easier for yourself and others to work with your code.
1. Use Meaningful Names
Choose descriptive names for your scripts, variables, and functions. This practice makes it easier to understand the purpose of each component without needing extensive comments. For instance, rather than naming a variable simply var1
, name it instance_id
when it stores an instance ID.
instance_id="i-1234567890abcdef0"
2. Comment Strategically
While clear naming reduces the need for comments, strategic commentary is still important. Use comments to explain the “why” behind complex logic or the purpose of a script section. Avoid stating the obvious; instead, focus on parts of the code that might confuse others.
# Retrieve the current instance state to determine if we need to stop it current_state=$(aws ec2 describe-instances --instance-ids $instance_id --query "Reservations[].Instances[].State.Name" --output text)
3. Structure Your Scripts
Organize your scripts with a clear structure. Group related functions together, and consider using sections or modules for complex scripts. Start with a brief description at the top, followed by variable declarations, function definitions, and finally the main execution block.
#!/bin/bash # Script to stop an EC2 instance and clean up resources # Define variables instance_id="i-1234567890abcdef0" # Function to stop instance stop_instance() { echo "Stopping instance $instance_id..." aws ec2 stop-instances --instance-ids $instance_id } # Main execution stop_instance
4. Avoid Hardcoding Values
Wherever possible, avoid hardcoding values in your scripts. Instead, use variables, configuration files, or command-line arguments. This approach enhances flexibility and adaptability, allowing users to modify script behavior without altering the code itself.
#!/bin/bash # Script to launch an EC2 instance with configurable parameters # Accept instance type and key pair as arguments instance_type=${1:-"t2.micro"} key_name=${2:-"my-key-pair"} aws ec2 run-instances --instance-type $instance_type --key-name $key_name
5. Handle Errors Gracefully
Integrate robust error handling mechanisms to manage unexpected failures. Use checks after critical commands and provide informative error messages. This not only prevents your script from failing silently but also aids in debugging.
aws ec2 stop-instances --instance-ids $instance_id if [ $? -ne 0 ]; then echo "Error: Failed to stop instance $instance_id" >&2 exit 1 fi
6. Test Rigorously
Before deploying any script in a production environment, thoroughly test it in a safe environment. Consider edge cases, and ensure the script behaves as expected under various conditions. Automated tests can also be beneficial for ensuring ongoing reliability.
7. Use Version Control
Implement version control for your scripts using systems like Git. This allows you to track changes over time, collaborate with others, and roll back to previous versions if necessary. Additionally, maintaining a changelog can help document the evolution of your scripts.
8. Document Your Code
Maintain clear documentation for your scripts, including their purpose, usage instructions, and examples. This practice is invaluable for onboarding new team members and for yourself when revisiting scripts after a period of time.
# Example script for launching an EC2 instance # Usage: ./launch_ec2.sh [instance_type] [key_name] # Defaults: instance_type=t2.micro, key_name=my-key-pair
By adhering to these best practices, you will foster a development environment that prioritizes clarity and efficiency. This will not only enhance the maintainability of your Bash scripts but also improve collaboration and productivity within your cloud operations team.