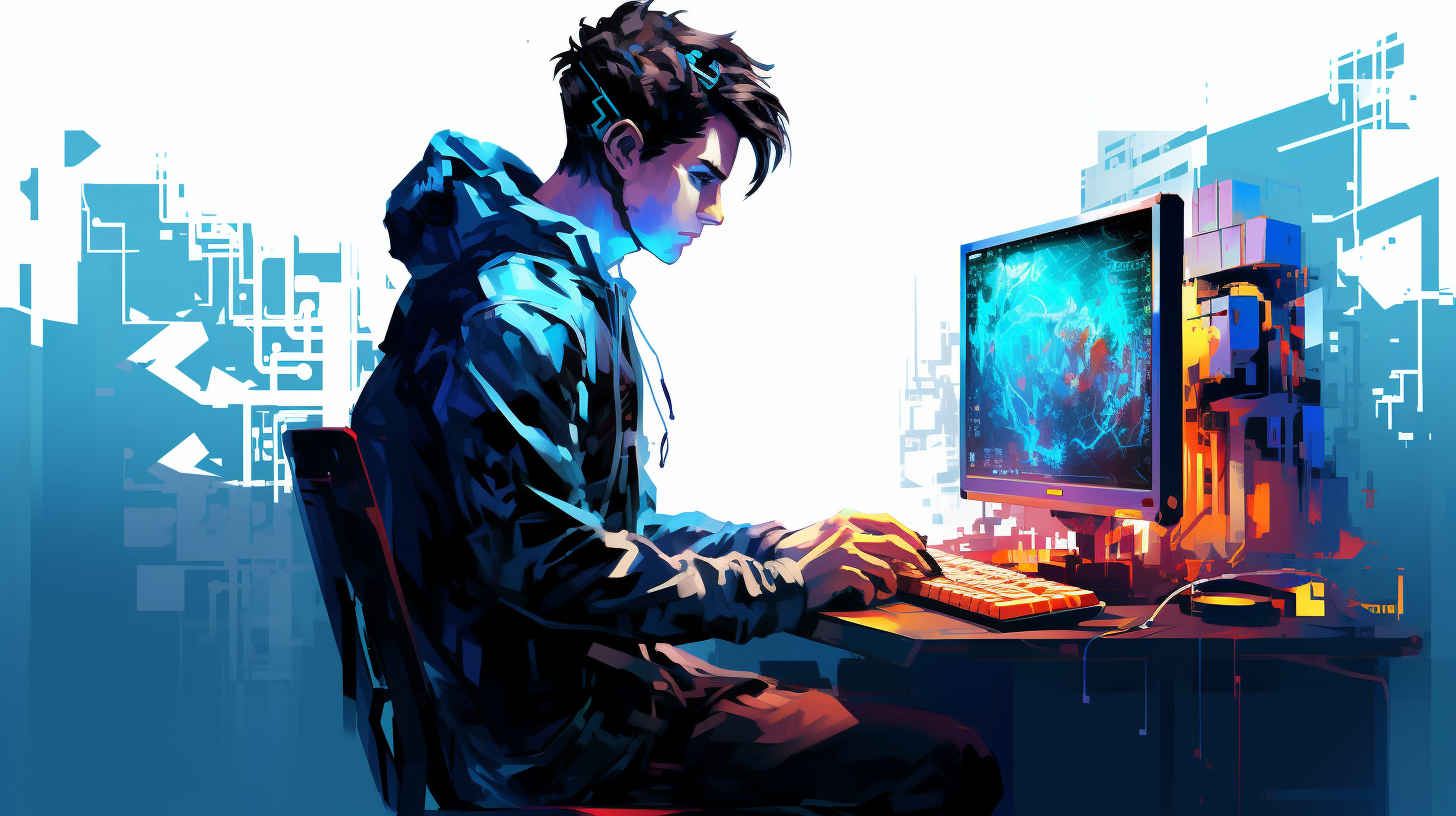
Bash Scripting for Network Troubleshooting
Network diagnostics can often feel like navigating a labyrinth, where each twist and turn reveals potential pitfalls in connectivity and performance. With Bash scripting, you can illuminate the path through the darkness of network issues, which will allow you to automate tests and gather data efficiently. The beauty of using Bash lies in its simplicity and power, enabling you to harness built-in commands and combine them into sophisticated scripts that cater to your specific troubleshooting needs.
To begin with, Bash provides a range of commands that can be leveraged for network diagnostics. Commands like ping
, traceroute
, and nslookup
serve as the backbone of your diagnostic toolkit. These commands not only allow you to check connectivity but also help uncover the intricacies of your network’s performance.
For instance, the ping
command is your first line of defense against connectivity issues. It sends ICMP echo requests to a specified host and waits for replies, providing a clear indication of whether the host is reachable. Here’s a simple Bash script to automate a ping test:
#!/bin/bash # A simple script to ping a host and check connectivity HOST="google.com" COUNT=4 echo "Pinging $HOST for $COUNT times..." ping -c $COUNT $HOST
Running the above script will yield results that show the round-trip time for packets sent to the target host, so that you can identify potential latency issues.
Another essential command for network diagnostics is traceroute
, which reveals the path that packets take to reach a destination. This command can help you pinpoint where in the network the issues may be occurring. Here’s how you can script a traceroute test:
#!/bin/bash # A simple script to perform a traceroute HOST="google.com" echo "Tracing route to $HOST..." traceroute $HOST
As you execute this script, you will receive a list of hops, providing insights into the route taken by your packets, and highlighting any delays or failures at intermediate steps.
Using these commands in combination within a script can significantly enhance your troubleshooting capabilities. For example, you can create a script that pings a list of hosts, logs the results, and even sends alerts if any host becomes unreachable. This not only saves time but also ensures consistent monitoring of network health.
#!/bin/bash # Script to monitor multiple hosts and log results HOSTS=("google.com" "yahoo.com" "github.com") LOGFILE="ping_results.log" echo "Monitoring network connectivity..." > $LOGFILE for HOST in "${HOSTS[@]}"; do echo "Pinging $HOST..." >> $LOGFILE ping -c 4 $HOST >> $LOGFILE echo "-------------------" >> $LOGFILE done echo "Results logged to $LOGFILE"
This script iterates over an array of hostnames, pings each one, and appends the output to a log file, which will allow you to analyze the connectivity performance over time. With each executed script, you sharpen your diagnostic skills and deepen your understanding of network behavior.
Basic Network Commands and Their Usage
When diving deeper into network diagnostics, the utility of commands like nslookup and netstat cannot be overstated. These commands expand your ability to analyze network issues by providing crucial insights into DNS resolution and network connections, respectively.
The nslookup command is particularly valuable when investigating domain-related issues. It allows you to query DNS servers to retrieve domain names or IP addresses, assisting in the troubleshooting of DNS-related connectivity problems. Below is an example of how to script a simple nslookup query:
#!/bin/bash # Script to perform nslookup on a given domain DOMAIN="example.com" echo "Performing nslookup for $DOMAIN..." nslookup $DOMAIN
Executing the above script will provide you with the DNS server’s response, including the IP address associated with the domain. If the DNS resolution fails, it may point to issues such as misconfigured DNS servers or unreachable domains.
Moving on, the netstat command offers an in-depth look at network connections and statistics. It displays active connections, routing tables, and interface statistics, which are invaluable for diagnosing network problems. Below is an example script that summarizes active connections:
#!/bin/bash # Script to display active network connections echo "Fetching active network connections..." netstat -tuln
This script will list all active TCP and UDP connections, along with the ports they are using. This information can help you identify services that are listening for incoming connections or diagnose potential port conflicts.
Moreover, combining these commands into a single script can yield more comprehensive diagnostics. Here’s an example of a script that performs a series of checks, including pinging a host, looking up its DNS information, and displaying active connections:
#!/bin/bash # Comprehensive network diagnostics script HOST="example.com" # Ping the host echo "Pinging $HOST..." ping -c 4 $HOST # Lookup the DNS echo "Performing nslookup for $HOST..." nslookup $HOST # Display active connections echo "Fetching active network connections..." netstat -tuln
With this single script, you can quickly assess connectivity, DNS resolution, and active connections in one pass. This holistic approach not only streamlines the troubleshooting process but also enhances your ability to diagnose and resolve network issues effectively.
Ultimately, mastering these basic network commands and their usage in Bash scripting forms the foundation of effective network diagnostics. As you become more proficient, your ability to automate and extend these scripts will empower you to tackle complex networking problems with confidence and efficiency.
Automating Ping Tests for Connectivity Checks
Automating ping tests can be a game changer in the sphere of network diagnostics, transforming a tedious task into a streamlined process that provides consistent and timely information. By crafting a Bash script to automate these tests, you can continuously monitor connectivity to crucial hosts and rapidly identify potential issues before they escalate.
The essence of automating ping tests lies in the ability to run these checks at regular intervals, capturing the performance and availability of your network resources. Here’s a refined example of a Bash script that not only pings a given host but also logs the results with timestamps, allowing for an easy review of connectivity history:
#!/bin/bash # Automated ping test script with logging HOST="google.com" LOGFILE="ping_log.txt" INTERVAL=60 # Time in seconds between pings echo "Starting automated ping test for $HOST. Logging results to $LOGFILE..." # Infinite loop to ping the host at regular intervals while true; do TIMESTAMP=$(date +"%Y-%m-%d %H:%M:%S") echo "[$TIMESTAMP] Pinging $HOST..." >> $LOGFILE ping -c 1 $HOST &>> $LOGFILE echo "Sleeping for $INTERVAL seconds..." sleep $INTERVAL done
This script initiates an infinite loop that pings the specified host every 60 seconds, logging both the timestamp and the results to a designated file. The use of `&>>` in the `ping` command ensures that both standard output and standard error are captured, providing a comprehensive view of the ping results, including any packet loss or errors encountered during the test.
For environments where continuous monitoring is essential, consider implementing a mechanism to send alerts when connectivity fails. Below is an enhanced version of the script that sends an email alert if the host becomes unreachable:
#!/bin/bash # Automated ping test with email alert HOST="google.com" LOGFILE="ping_log.txt" INTERVAL=60 EMAIL="[email protected]" # Replace with your email echo "Starting automated ping test for $HOST. Logging results to $LOGFILE..." while true; do TIMESTAMP=$(date +"%Y-%m-%d %H:%M:%S") echo "[$TIMESTAMP] Pinging $HOST..." >> $LOGFILE if ping -c 1 $HOST &>> $LOGFILE; then echo "Ping successful." >> $LOGFILE else echo "Ping failed!" >> $LOGFILE echo "Alert: $HOST is unreachable!" | mail -s "Ping Alert: $HOST" $EMAIL fi echo "Sleeping for $INTERVAL seconds..." sleep $INTERVAL done
In this version, if the ping command fails, an email alert is sent to the specified address. The integration of the `mail` command provides a proactive approach to network monitoring, allowing administrators to address issues promptly.
With these scripts, you are empowered to keep an eye on your network’s health efficiently. The ability to automate ping tests not only saves time but also ensures you have a reliable record of network connectivity, which can prove invaluable for troubleshooting and maintaining optimal network performance.
Using Traceroute for Path Analysis
When it comes to analyzing network paths, the traceroute command is an essential tool that provides insights into how data packets traverse the network to reach their destinations. By understanding the route that packets take, you can identify where delays or failures occur, which can be crucial for troubleshooting complex networking issues. With Bash scripting, you can automate traceroute tests, logging results for further analysis.
Using traceroute in a Bash script is simpler. The basic syntax involves specifying the target host, and the command will display each hop along the path. Each line of output represents a router or gateway that the packets pass through, along with the time taken to reach each hop. Here’s how to automate this process:
#!/bin/bash # Automated traceroute script HOST="google.com" LOGFILE="traceroute_results.log" echo "Tracing route to $HOST. Logging results to $LOGFILE..." # Run traceroute and log output traceroute $HOST &> $LOGFILE echo "Traceroute completed. Results are saved in $LOGFILE."
In this script, the traceroute command is executed, and the results are redirected to a log file. This approach allows you to preserve the output for later evaluation, helping to track down intermittent connectivity problems or high latency issues.
To enhance this script, consider adding a timestamp to each traceroute log entry, which can be useful for monitoring changes over time:
#!/bin/bash # Enhanced automated traceroute script with timestamps HOST="google.com" LOGFILE="traceroute_results.log" echo "Starting automated traceroute for $HOST. Logging results to $LOGFILE..." # Adding a timestamp to the log echo "Traceroute initiated at $(date)" >> $LOGFILE traceroute $HOST >> $LOGFILE echo "Traceroute completed at $(date). Results are saved in $LOGFILE."
This modification appends a timestamp before and after the execution of the traceroute, providing context for when the data was captured. Such context is invaluable when analyzing historical logs, especially in environments where network conditions may change frequently.
Another way to expand the functionality of your traceroute scripts is to automate periodic checks. This allows you to monitor the path to critical resources over time:
#!/bin/bash # Periodic traceroute monitoring script HOST="google.com" LOGFILE="traceroute_log.txt" INTERVAL=300 # Time in seconds between traceroutes echo "Starting periodic traceroute monitoring to $HOST. Logging results to $LOGFILE..." while true; do echo "Traceroute initiated at $(date)" >> $LOGFILE traceroute $HOST >> $LOGFILE echo "----------------------------------------" >> $LOGFILE sleep $INTERVAL done
This script runs traceroute at specified intervals (e.g., every 5 minutes) and appends the output to a log file. By monitoring the path over time, you can identify patterns or recurring issues that may not be apparent during a one-off test. This feature is particularly useful in a production environment, where network reliability is paramount.
Using traceroute within Bash scripts empowers you to dissect network paths and analyze connectivity issues with precision. By automating these processes, you not only save time but also equip yourself with a comprehensive toolkit for network diagnostics. The more you delve into these scripts, the more adept you become at navigating the complexities of network behavior.
Monitoring Network Performance with Scripts
When monitoring network performance, it’s crucial to have tools that can provide real-time insights into various metrics such as bandwidth usage, latency, and packet loss. One of the advantages of using Bash scripting for monitoring tasks is its ability to interface with multiple commands and utilities that can gather and analyze network performance data. Here, we will explore how to create scripts that gather network performance metrics and log them for analysis.
A fundamental command for network performance monitoring is iftop
, which displays bandwidth usage on an interface. However, since iftop
requires a terminal interface, we’ll often rely on vnstat
, which allows for logging and reporting network traffic on a specific interface over time.
Here’s how to install vnstat
on a Debian-based system:
sudo apt-get install vnstat
Once installed, we can create a Bash script that logs bandwidth usage at regular intervals. Below is a sample script that captures data from vnstat
and appends it to a log file:
#!/bin/bash # Script to monitor network bandwidth using vnstat INTERFACE="eth0" # Change this to your network interface LOGFILE="vnstat_log.txt" INTERVAL=60 # Time in seconds between logs echo "Starting bandwidth monitoring on $INTERFACE. Logging results to $LOGFILE..." # Infinite loop to log bandwidth usage while true; do TIMESTAMP=$(date +"%Y-%m-%d %H:%M:%S") echo "[$TIMESTAMP] Bandwidth usage for $INTERFACE:" >> $LOGFILE vnstat -i $INTERFACE >> $LOGFILE echo "----------------------------------------" >> $LOGFILE sleep $INTERVAL done
This script runs indefinitely, capturing the bandwidth usage every minute and logging it to a file. The output from vnstat
provides insights into the total data used, both incoming and outgoing, so that you can observe trends over time.
For a more advanced monitoring setup, you might also want to include alerts for unusual spikes in bandwidth usage. Here’s how you can enhance the script to send an email alert if the incoming or outgoing bandwidth exceeds a certain threshold:
#!/bin/bash # Enhanced bandwidth monitoring script with alert INTERFACE="eth0" # Change this to your network interface LOGFILE="vnstat_log.txt" INTERVAL=60 THRESHOLD=1000000 # Set threshold in bytes (e.g., 1MB) EMAIL="[email protected]" # Replace with your email echo "Starting bandwidth monitoring on $INTERFACE. Logging results to $LOGFILE..." while true; do TIMESTAMP=$(date +"%Y-%m-%d %H:%M:%S") echo "[$TIMESTAMP] Bandwidth usage for $INTERFACE:" >> $LOGFILE vnstat -i $INTERFACE >> $LOGFILE IN=$(vnstat --oneline | awk -F';' '{print $3}') OUT=$(vnstat --oneline | awk -F';' '{print $4}') if [ "$IN" -gt "$THRESHOLD" ] || [ "$OUT" -gt "$THRESHOLD" ]; then echo "Alert: High bandwidth usage detected!" | mail -s "Bandwidth Alert" $EMAIL fi echo "----------------------------------------" >> $LOGFILE sleep $INTERVAL done
This modified script checks the incoming and outgoing bandwidth after each log and sends an email if either exceeds the specified threshold. This real-time alerting mechanism is invaluable for network administrators who need to respond promptly to potential issues.
Another critical tool for network performance monitoring is ping
, which can be employed to assess latency and packet loss. Combining ping
with a logging mechanism can help you maintain a historical record of network performance. Below is a simple script to log ping results:
#!/bin/bash # Ping monitoring script HOST="google.com" LOGFILE="ping_performance.log" INTERVAL=5 # Time in seconds between pings echo "Starting ping monitoring for $HOST. Logging results to $LOGFILE..." while true; do TIMESTAMP=$(date +"%Y-%m-%d %H:%M:%S") echo "[$TIMESTAMP] Pinging $HOST..." >> $LOGFILE ping -c 5 $HOST >> $LOGFILE echo "----------------------------------------" >> $LOGFILE sleep $INTERVAL done
This script sends five pings to the specified host and logs the results, including statistics on packet loss and round-trip time. Such records can be instrumental in understanding the network’s performance history and diagnosing intermittent issues.
By using these Bash scripts, you can create a robust monitoring system tailored to your network’s specific needs. The ability to log, alert, and analyze network performance equips you with the tools necessary for maintaining optimal network health. Continuously refining these scripts will further enhance your capacity to respond to network challenges, ensuring a more reliable and efficient infrastructure.
Logging and Reporting Network Issues
Logging and reporting network issues is a vital aspect of network management, especially for troubleshooting and maintaining optimal performance. By systematically capturing data about network behavior, you can build a compelling narrative of connectivity problems, helping to illuminate the root causes of issues that may otherwise go unnoticed. Bash scripting provides an efficient means of automating this logging process, so that you can gather meaningful insights smoothly.
To effectively log network issues, you should incorporate both connectivity tests (like ping) and performance metrics (like bandwidth and latency) into your reporting scripts. This comprehensive approach not only highlights immediate problems but also reveals trends over time that may indicate underlying issues.
Consider the following script that combines ping tests and logging results to a file, while also implementing a simple error condition to mark failures:
#!/bin/bash # Network troubleshooting logging script HOST="google.com" LOGFILE="network_issue_log.txt" INTERVAL=60 # Check every 60 seconds echo "Starting network issue logging for $HOST. Results will be saved in $LOGFILE..." # Infinite loop for continuous monitoring while true; do TIMESTAMP=$(date +"%Y-%m-%d %H:%M:%S") echo "[$TIMESTAMP] Pinging $HOST..." >> $LOGFILE if ping -c 1 $HOST &>> $LOGFILE; then echo "Ping to $HOST succeeded." >> $LOGFILE else echo "Ping to $HOST failed! Possible network issue." >> $LOGFILE fi echo "----------------------------------------" >> $LOGFILE sleep $INTERVAL done
This script will log every ping attempt to the specified host along with a timestamp. In the event of a failure, it will clearly document the issue, allowing for an easy review later. This kind of structured logging is invaluable for identifying patterns over time.
Additionally, you can enhance your logging by including performance metrics such as response times and packet loss. Here’s an enhanced version of the previous script that logs these additional metrics:
#!/bin/bash # Enhanced network logging script with performance metrics HOST="google.com" LOGFILE="detailed_network_log.txt" INTERVAL=60 echo "Starting detailed network logging for $HOST. Results will be saved in $LOGFILE..." while true; do TIMESTAMP=$(date +"%Y-%m-%d %H:%M:%S") echo "[$TIMESTAMP] Pinging $HOST..." >> $LOGFILE RESULT=$(ping -c 1 $HOST) if [ $? -eq 0 ]; then echo "Ping succeeded:" >> $LOGFILE echo "$RESULT" >> $LOGFILE else echo "Ping failed! Possible network issue." >> $LOGFILE fi echo "----------------------------------------" >> $LOGFILE sleep $INTERVAL done
The above script captures the entire output of the ping command, providing detailed metrics such as response time and packet loss statistics. This data can be critical for diagnosing intermittent connectivity problems or identifying trends in network performance.
Incorporating logging for other network diagnostics, such as traceroute or bandwidth monitoring, can further enhance your reporting capabilities. For example, consider combining ping results with traceroute outputs to create a comprehensive log of connectivity events:
#!/bin/bash # Comprehensive network issue logging script HOST="google.com" LOGFILE="comprehensive_network_log.txt" INTERVAL=60 echo "Starting comprehensive network issue logging for $HOST. Results will be saved in $LOGFILE..." while true; do TIMESTAMP=$(date +"%Y-%m-%d %H:%M:%S") echo "[$TIMESTAMP] Pinging $HOST..." >> $LOGFILE if ping -c 1 $HOST &>> $LOGFILE; then echo "Ping succeeded." >> $LOGFILE else echo "Ping failed! Possible network issue." >> $LOGFILE fi echo "[$TIMESTAMP] Running traceroute..." >> $LOGFILE traceroute $HOST >> $LOGFILE echo "----------------------------------------" >> $LOGFILE sleep $INTERVAL done
With this script, each ping and traceroute result is logged in sequence, creating a detailed account of the network’s performance at regular intervals. This comprehensive dataset enables quicker diagnostics and more informed decision-making when addressing network issues.
Moreover, you could think integrating system notifications or alerts based on the logged conditions. For example, if the script detects a series of ping failures, it could send an email or a message to a monitoring system, allowing for rapid response to critical issues:
#!/bin/bash # Network logging script with alerting feature HOST="google.com" LOGFILE="alert_network_log.txt" INTERVAL=60 EMAIL="[email protected]" echo "Starting network logging with alerts for $HOST. Results will be saved in $LOGFILE..." while true; do TIMESTAMP=$(date +"%Y-%m-%d %H:%M:%S") echo "[$TIMESTAMP] Pinging $HOST..." >> $LOGFILE if ping -c 1 $HOST &>> $LOGFILE; then echo "Ping succeeded." >> $LOGFILE else echo "Ping failed! Possible network issue." >> $LOGFILE echo "Alert: $HOST is unreachable!" | mail -s "Network Alert: $HOST Down" $EMAIL fi echo "----------------------------------------" >> $LOGFILE sleep $INTERVAL done
In this example, an alert is sent via email if the ping fails, ensuring that network administrators can address issues promptly. This proactive approach to network monitoring can dramatically reduce downtime and enhance overall network reliability.
Ultimately, the combination of logging, reporting, and alerting through Bash scripts provides a powerful framework for diagnosing and addressing network issues. By continuously refining these scripts and tailoring them to your specific network environment, you can greatly enhance your capacity to maintain network health and performance.