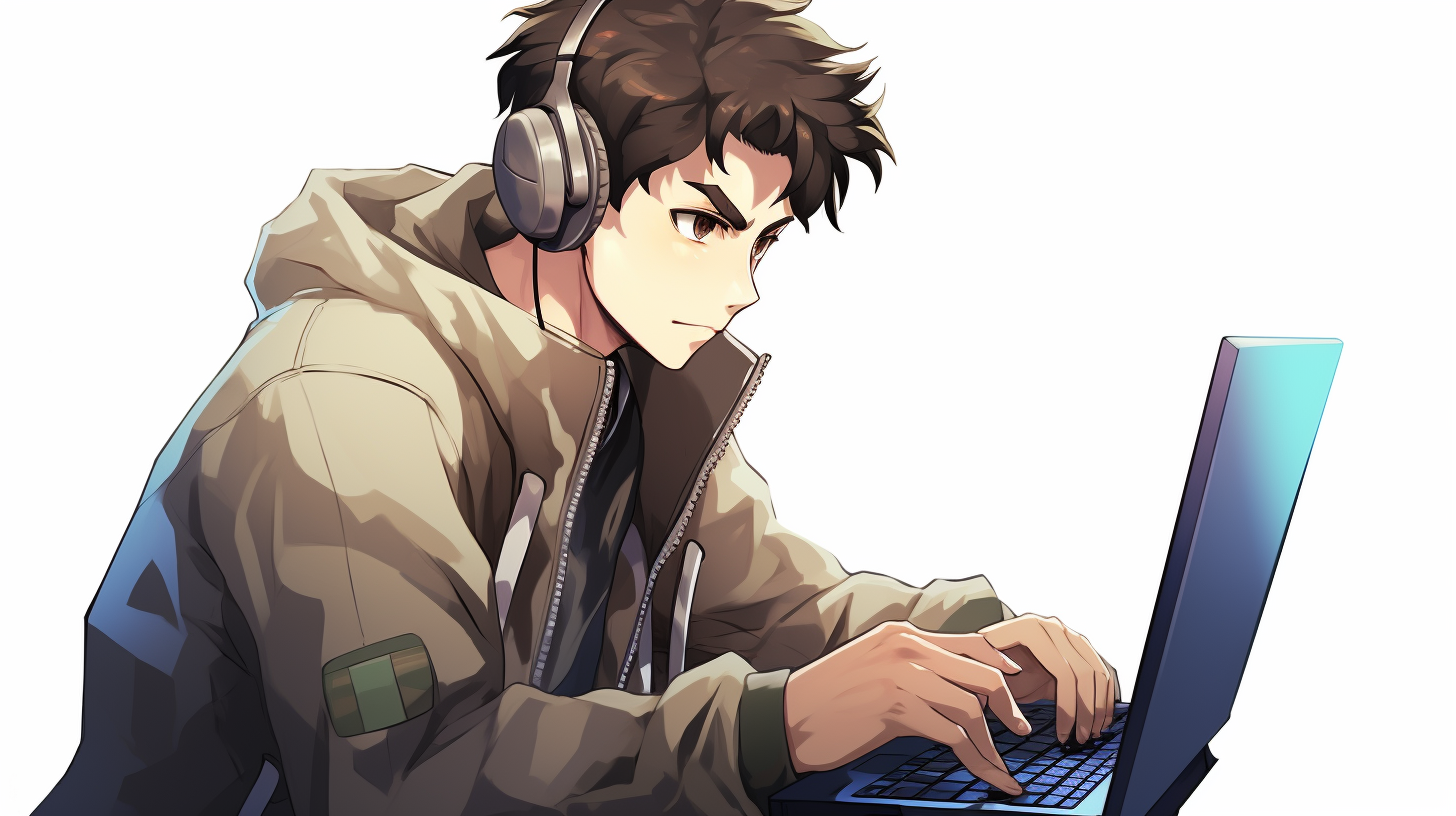
Bash Scripting for SEO Tasks
Understanding the fundamentals of Bash especially important for automating SEO tasks efficiently. Bash, or the Bourne Again SHell, is a powerful scripting language that allows you to interact with the operating system and automate repetitive tasks. This capability is invaluable for SEO specialists who seek to streamline their workflows, execute bulk actions, and handle data analysis with minimal effort.
At its core, Bash scripting is about running a series of commands in a sequence. The simplicity of its syntax enables users to craft powerful scripts that can manage files, manipulate text, and automate web-related tasks swiftly. Here are a few fundamental concepts you’ll need to grasp:
Variables: In Bash, you can store data in variables, which can then be used throughout your script. For instance, if you want to store a URL for future use, you can do so like this:
url="https://www.example.com"
Control Structures: Bash provides various control structures such as loops and conditional statements. These allow you to execute certain commands based on specific conditions or to iterate over a series of items. Here’s an example of a simple loop that prints out numbers 1 through 5:
for i in {1..5}; do echo "Number: $i" done
Functions: You can encapsulate commands in functions for better organization and reusability. That is particularly useful when you have repetitive tasks. Here’s how to define a simple function:
function greet { echo "Hello, $1!" } greet "SEO Specialist"
Command-Line Arguments: When executing scripts, you can pass parameters to customize their behavior. In your script, you can access these arguments using special variables like $1, $2, etc. Here’s an example:
echo "First argument: $1" echo "Second argument: $2"
File Handling: A significant part of SEO tasks involves dealing with files, whether it is reading data from a CSV or processing log files. You can easily read and write files using redirection. For example, to save the output of a command to a file, you can do:
echo "This is a log entry" >> logfile.txt
These fundamental concepts form the backbone of Bash scripting, equipping you with the tools needed to automate various SEO tasks. By mastering these basics, you’ll be well on your way to creating scripts that can enhance your efficiency and effectiveness in SEO operations.
Crafting Scripts for Keyword Research
Keyword research is an important component of SEO, as it helps identify the terms and phrases that potential customers are using to find products or services online. By automating this process using Bash scripts, you can save time and ensure that you consistently gather data from various sources. Below are examples of how to craft scripts that will aid in keyword research.
One common approach is to use the curl
command to fetch data from various keyword research tools directly from the command line. For instance, if you are using a tool that provides a simple API for keyword suggestions, you can retrieve results with a script like this:
#!/bin/bash # Set the API endpoint and your API key api_url="https://api.keywordtool.io/v2/search" api_key="YOUR_API_KEY" # Set the keyword to search for keyword="seo" # Fetch keyword suggestions response=$(curl -s "${api_url}?apikey=${api_key}&keyword=${keyword}&country=us") # Parse and display the results echo "Keyword Suggestions for '$keyword':" echo "$response" | jq '.results[] | .keyword'
In this script, we use curl
to send a request to the keyword tool API. The response is then processed using jq
, a powerful command-line JSON processor, to extract and display useful keyword suggestions.
Another effective technique involves using Google Search itself to gather keyword ideas. The following script can help automate the process of fetching related searches from Google:
#!/bin/bash # Set your search query search_query="bash scripting" # Fetch related searches from Google related_searches=$(curl -s "https://www.google.com/search?q=${search_query}" | grep -oP '(?<=<a class="k8XOCe" href="/search?q=).*?(?=")') # Display the related keywords echo "Related Searches for '$search_query':" echo "$related_searches" | sed 's/%20/ /g' | sort -u
This script sends a search query to Google and uses grep
to extract relevant related searches from the HTML response. It then formats the output to make it more readable by replacing URL encoded spaces.
Additionally, you may want to analyze keywords based on search volume and competition. By combining scripts, you can create a comprehensive keyword research tool. For example, you could fetch keyword data from multiple sources and compile it into a single CSV file:
#!/bin/bash output_file="keyword_data.csv" echo "Keyword,Search Volume,Competition" > $output_file keywords=("seo" "bash scripting" "keyword research") for keyword in "${keywords[@]}"; do # Simulate API call to fetch data (replace with actual API calls) search_volume=$((RANDOM % 1000)) # Random volume for demonstration competition=$((RANDOM % 100)) # Random competition for demonstration # Append the data to the CSV file echo "$keyword,$search_volume,$competition" >> $output_file done echo "Keyword data saved to $output_file"
This script initializes a CSV file with headers and iterates over an array of keywords, simulating API calls to fetch search volumes and competition levels. Finally, it saves the results to a CSV file, which can be opened and analyzed in spreadsheet software.
By using these Bash scripts for keyword research, you can not only automate the data collection process but also enhance your ability to make informed decisions based on the gathered insights. As you become more comfortable with these techniques, you can further customize your scripts to suit the specific needs of your SEO strategy.
Automating Site Audits with Bash
# Fetch related searches from Google related_searches=$(curl -s "https://www.google.com/search?q=${search_query}" | grep -oP '(?<=).*?(?=)') # Display the related searches echo "Related Searches for '$search_query':" echo "$related_searches"
By running the above script, you’ll leverage the power of curl combined with grep’s regex capabilities to extract related search terms directly from Google’s search results. This method not only saves you time but also ensures you are capturing evolving keyword trends in real-time.
Transitioning to site audits, automating this process with Bash can drastically reduce the time required for manual checks. A site audit often involves checking HTTP status codes, analyzing page titles, and validating meta descriptions. These checks can be automated with a combination of curl and text processing tools.
For instance, let’s consider a script that checks the HTTP status codes of a list of URLs stored in a file. That is how you could automate that process:
#!/bin/bash # Check if the input file is provided if [ $# -ne 1 ]; then echo "Usage: $0 " exit 1 fi # Read the URLs from the input file input_file="$1" # Loop through each URL and check the HTTP status while IFS= read -r url; do status=$(curl -o /dev/null -s -w "%{http_code}n" "$url") echo "URL: $url - Status Code: $status" done < "$input_file"
In this script, we first check if a file containing URLs is provided as an argument. We then read each URL line by line and use curl to determine the HTTP status code. The results can help you identify broken links or server errors that might be detrimental to your SEO efforts.
Additionally, a site audit often requires checking page titles and meta descriptions for compliance with SEO best practices. This can also be automated with a Bash script that pulls these elements directly from the HTML source. The following script illustrates how to extract the title and meta description from a webpage:
#!/bin/bash # Function to fetch title and meta description fetch_seo_data() { local url="$1" # Fetch the HTML content html_content=$(curl -s "$url") # Extract the title title=$(echo "$html_content" | grep -oP '(?<=)(.*?)(?= )') # Extract the meta description meta_desc=$(echo "$html_content" | grep -oP '(?<=<meta name="description" content=")(.*?)(?=")') # Output the results echo "URL: $url" echo "Title: $title" echo "Meta Description: $meta_desc" echo } # Check if the input file is provided if [ $# -ne 1 ]; then echo "Usage: $0 " exit 1 fi # Read the URLs from the input file input_file="$1" # Loop through each URL while IFS= read -r url; do fetch_seo_data "$url" done < "$input_file"
This script defines a function that retrieves and echoes the title and meta description of each URL provided in the input file. It encapsulates the logic for fetching and parsing content, making your audits more manageable and efficient.
Automation with Bash scripts not only speeds up site audits but also ensures consistency and thoroughness in your SEO assessments. By using these powerful capabilities, SEO professionals can focus more on strategy and analysis rather than getting bogged down in manual tasks.
Log File Analysis for Traffic Insights
Log files are an essential component of web traffic analysis, providing insights into user behavior, server performance, and SEO effectiveness. By analyzing these logs, SEO specialists can identify patterns, monitor traffic sources, and detect issues that may hinder site performance. Bash scripting allows for efficient log file analysis, enabling you to extract meaningful data quickly. Below are some techniques for using Bash to parse and analyze web server logs.
Typically, web server logs are stored in a standard format, such as the Common Log Format (CLF). Each line in these logs represents a request to the server, detailing information like the IP address of the client, the requested resource, the response status code, and the user agent. Here’s an example of a single log entry:
192.168.1.1 - - [12/Oct/2023:14:32:01 +0000] "GET /index.html HTTP/1.1" 200 2326 "http://example.com/" "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/86.0.4240.198 Safari/537.36"
To analyze these logs effectively, you can create scripts that filter and summarize the data. A common task is to count the number of requests per IP address. This can be achieved with the following script:
#!/bin/bash # Set the log file path logfile="/var/log/access.log" # Count requests per IP address echo "Counting requests per IP address:" awk '{print $1}' "$logfile" | sort | uniq -c | sort -nr
In this script, we use awk
to extract the first field (the IP address) from each log entry. The output is then sorted and counted for uniqueness using uniq
, followed by a final sort in reverse numerical order to display the most active IPs first.
Another useful analysis is determining which pages experience the highest traffic. The following script can help you achieve that:
#!/bin/bash # Set the log file path logfile="/var/log/access.log" # Count requests per page echo "Counting requests per page:" awk '{print $7}' "$logfile" | sort | uniq -c | sort -nr | head -n 10
This script focuses on the seventh field of each log entry, which typically contains the requested URL. By counting and sorting the requests, you can easily identify the top ten most visited pages on your website.
Additionally, you may want to analyze response status codes to identify potential issues, such as 404 errors indicating missing pages. Here’s how you can extract those logs:
#!/bin/bash # Set the log file path logfile="/var/log/access.log" # Extract 404 errors echo "Identifying 404 errors:" grep " 404 " "$logfile" | awk '{print $7}' | sort | uniq -c | sort -nr
This script uses grep
to filter log entries for 404 status codes, then extracts and counts the unique URLs that returned this error, helping you pinpoint pages that need attention.
By automating log file analysis with Bash scripts, you can gain deeper insights into traffic patterns and user behavior. These analyses can inform your SEO strategies, helping to improve site performance and enhance user experience.
Generating SEO Reports with Shell Scripting
Generating SEO reports is a vital aspect of tracking and improving website performance. By using the capabilities of Bash, you can automate the creation of comprehensive SEO reports that compile essential metrics into easily digestible formats. This efficiency can save you significant time and help you focus on strategic tasks rather than manual data entry.
One of the most effective ways to generate SEO reports is by gathering data from various sources, such as Google Analytics, server logs, or any web scraping tools that provide vital metrics. You can create scripts that pull in this data, process it, and format it for reporting. Below, I’ll demonstrate how to automate the generation of a simple SEO report using Bash.
Let’s assume you want to create a report containing the number of visits to your website over the last week. You can extract this information from a log file, typically located in your web server’s logs. Here’s an example of how you might write such a script:
#!/bin/bash # Define the log file to analyze log_file="/var/log/apache2/access.log" # Define the output report file report_file="seo_report.txt" # Initialize the report file echo "SEO Report - Visits in the Last Week" > $report_file echo "-----------------------------------" >> $report_file # Get the current date and the date seven days ago current_date=$(date +"%d/%b/%Y") seven_days_ago=$(date -d "7 days ago" +"%d/%b/%Y") # Extract the number of visits in the last week echo "Number of visits from $seven_days_ago to $current_date:" >> $report_file visits=$(grep -E "$seven_days_ago|$current_date" $log_file | wc -l) echo "$visits" >> $report_file # Display a message indicating that the report has been generated echo "Report generated: $report_file"
In this script, we define the path to the server’s log file and the output file for our report. We then initialize the report by writing a header. Using the `grep` command, we filter the log entries for the last week and count the number of visits using `wc -l`. Finally, the script outputs the total visits into the specified report file.
By customizing this script, you can add more metrics to your report, such as unique visitors, page views per visit, or even specific errors encountered. This can be achieved by modifying the `grep` and `wc` commands or integrating additional processing using tools like `awk` or `sed`.
Additionally, you can automate the execution of this script using cron jobs, scheduling it to run weekly or monthly, so you always have the latest data at your fingertips. This not only enhances efficiency but also ensures that your reports are consistent and timely.
With these Bash scripting techniques, generating detailed SEO reports becomes a streamlined process, which will allow you to focus on analyzing the data and implementing improvements based on your findings.
Integrating Bash with SEO Tools and APIs
Integrating Bash with SEO tools and APIs can significantly enhance your SEO workflows, enabling you to automate tasks that would otherwise consume valuable time. By using the power of command-line tools and REST APIs, you can fetch data, manipulate it, and generate insights without the need for extensive manual effort. Here’s how you can effectively integrate Bash with popular SEO tools and APIs.
One popular tool is the Ahrefs API, which allows users to pull a wealth of data regarding backlinks, keywords, and site audits. By integrating this API into your Bash scripts, you can automate the retrieval of valuable SEO metrics. The following example demonstrates how you might do this:
#!/bin/bash # Set your Ahrefs API endpoint and access token api_url="https://apiv2.ahrefs.com/" access_token="YOUR_ACCESS_TOKEN" # Define the target domain target_domain="example.com" # Fetch backlinks data response=$(curl -s "${api_url}?token=${access_token}&target=${target_domain}&output=json&from=backlinks&mode=domain") # Display the number of backlinks echo "Number of backlinks for ${target_domain}:" echo "$response" | jq '.refpages | length'
In this script, we define the Ahrefs API URL and access token, set the target domain, and make a request to fetch backlinks data. The response is processed using jq to extract the number of backlinks, demonstrating how you can easily pull and analyze data from a powerful SEO tool.
Another common integration involves Google Analytics and Google Search Console. Automating data retrieval from these platforms can provide insights into your site’s performance. Below is an example of how to fetch organic search traffic data from Google Analytics using the Google Analytics Reporting API:
#!/bin/bash # Set the Google Analytics API endpoint and access token api_url="https://analyticsreporting.googleapis.com/v4/reports:batchGet" access_token="YOUR_ACCESS_TOKEN" # Set the view ID and date range view_id="YOUR_VIEW_ID" date_range="last_30_days" # Request organic search traffic data response=$(curl -s -X POST -H "Authorization: Bearer ${access_token}" -H "Content-Type: application/json" -d '{ "reportRequests": [ { "viewId": "'${view_id}'", "dateRanges": [{"startDate": "'${date_range}'", "endDate": "today"}], "metrics": [{"expression": "ga:sessions"}, {"expression": "ga:users"}], "dimensions": [{"name": "ga:date"}] } ] }' "${api_url}") # Display the results echo "Organic search traffic for the last 30 days:" echo "$response" | jq '.reports[0].data.rows[] | {date: .dimensions[0], sessions: .metrics[0][0], users: .metrics[0][1]}'
This script sends a POST request to the Google Analytics Reporting API, pulling key metrics such as sessions and users over the past 30 days. By processing the JSON response, you can easily extract and display relevant data in a readable format.
For integrations that require authentication, you may need to implement OAuth 2.0. This can involve more complex flows, but Bash can still handle it. Using tools like `curl` in conjunction with `jq` allows you to authenticate and make calls to various APIs efficiently.
As you integrate these tools into your Bash scripts, remember to consider error handling and API rate limits. Adding conditional checks and logging can help ensure that your scripts run smoothly and provide you with accurate data without interruptions.
Integrating Bash with various SEO tools and APIs can greatly streamline your SEO tasks, turning a manual process into an automated powerhouse. The ability to fetch, manipulate, and analyze data through simple scripts not only saves time but also enhances the accuracy of your SEO efforts.