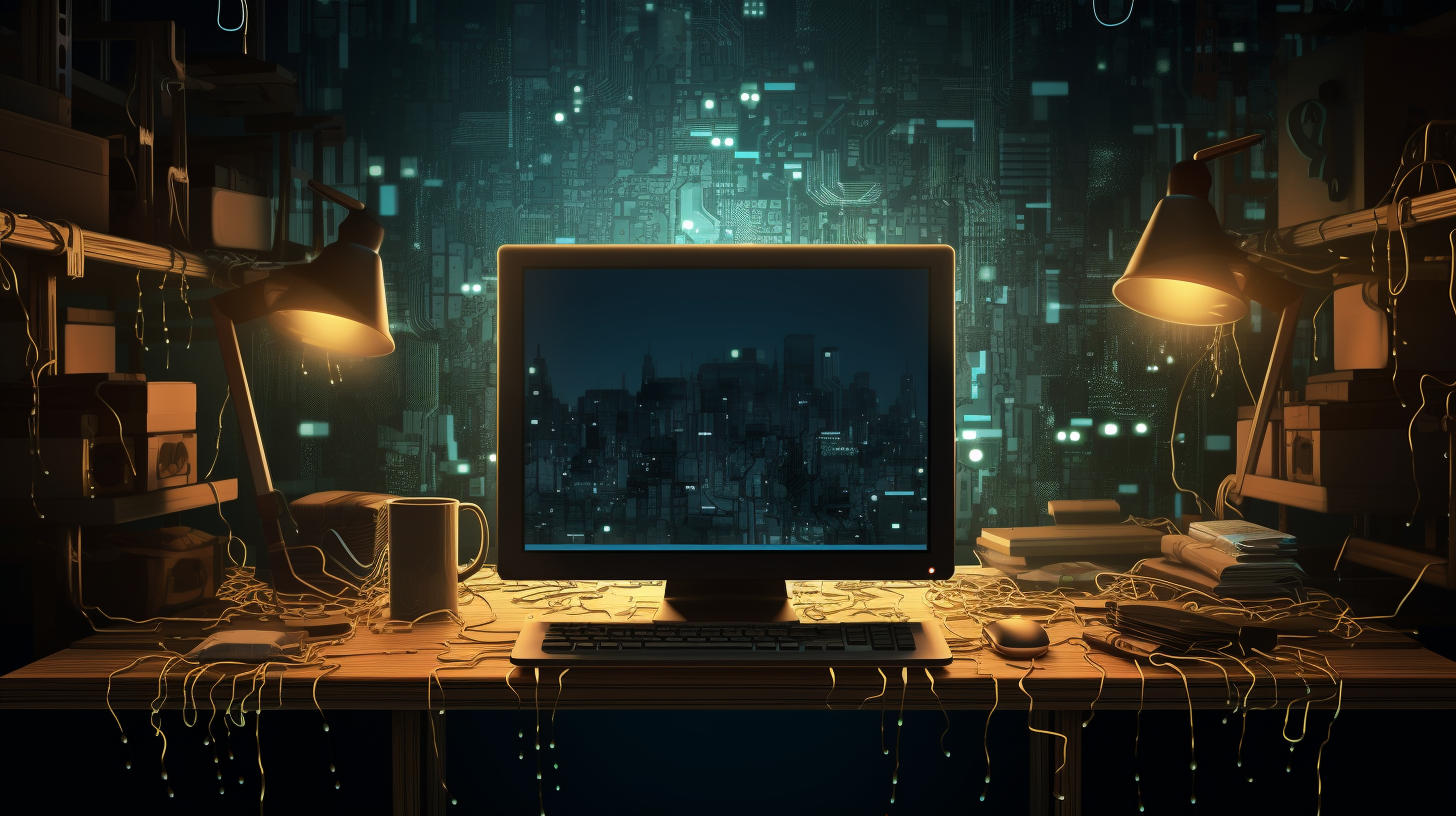
Bash Scripting for Video Processing
When venturing into video processing using Bash, having the right tools and libraries at your disposal especially important. The essence of successful scripting lies in using powerful utilities that can handle various codecs, formats, and tasks efficiently.
One of the most widely used tools in this domain is FFmpeg. FFmpeg is a comprehensive suite of libraries and programs for handling multimedia data. It supports a multitude of formats and codecs, making it an indispensable asset for any video processing task. The beauty of FFmpeg lies in its command-line interface, which allows for extensive manipulation of audio and video streams. Installing FFmpeg is straightforward; on Debian-based systems, you can use:
sudo apt-get install ffmpeg
Once installed, you can check its functionality by running:
ffmpeg -version
Another essential tool is ImageMagick. While primarily known for image manipulation, it can handle video frames, which will allow you to convert videos into a series of images or vice versa. ImageMagick can be installed with:
sudo apt-get install imagemagick
For those who want to delve deeper into video effects or editing, Libav is another library worth exploring. It offers a collection of tools to handle video and audio streams, similar to FFmpeg. However, the choice between Libav and FFmpeg often comes down to personal preference and specific feature requirements.
Furthermore, GStreamer stands out as a powerful multimedia framework that can be scripted with Bash to create complex processing pipelines. GStreamer allows you to handle streaming media and can be integrated into various applications. Installation is often available through package managers:
sudo apt-get install gstreamer1.0-tools
Another noteworthy mention is MPV, a versatile media player that can also be leveraged for scripting purposes. It provides a rich set of features for playing multimedia content and can be controlled via the command line, making it suitable for automated tasks.
In addition to these tools, having a solid understanding of bash itself, along with proficiency in text processing utilities like sed and awk, can elevate your video processing scripts. These tools allow for the manipulation of command output, making it easier to handle batch operations and logs effectively.
With these essential tools and libraries at your disposal, the possibilities for video processing in Bash are vast and varied. Each tool brings unique capabilities to the table, empowering you to tackle a high number of tasks with a scripting approach that’s both efficient and elegant.
Writing Bash Scripts for Video Conversion
Writing Bash scripts for video conversion is an exhilarating endeavor, as it allows you to harness the power of command-line tools to manipulate multimedia content seamlessly. The flexibility and efficiency of Bash make it well-suited for automating video conversion tasks, enabling you to convert files between formats, adjust quality settings, and even batch process multiple files with minimal effort.
At the core of many video conversion scripts lies FFmpeg, which provides an extensive range of options for converting video files. The basic syntax for converting a video from one format to another with FFmpeg is straightforward:
ffmpeg -i input.mp4 output.avi
In this example, the command takes an input video file named input.mp4
and converts it to the AVI format, saving it as output.avi
. The -i
flag specifies the input file, while the output file format is determined by the extension you provide.
However, video conversion often requires more than just a simple format change. You might want to adjust the bitrate, resolution, or codec. FFmpeg allows for this level of customization. For instance, if you wish to convert a video while reducing its bitrate to save space, you could use:
ffmpeg -i input.mp4 -b:v 1M output.mp4
Here, the -b:v
option sets the video bitrate to 1 Mbps. Adjusting the bitrate can significantly impact the quality and size of the output file, so it is essential to find a balance that suits your needs.
Additionally, you may want to change the resolution of the video during conversion. This can be achieved with the -s
option, which allows you to specify the desired width and height:
ffmpeg -i input.mp4 -s 1280x720 output.mp4
In this command, the output video will be resized to a resolution of 1280×720 pixels. Such transformations can prove particularly useful when preparing videos for specific platforms or devices.
For more advanced usage, you might want to include audio settings in your script. To convert a video while adjusting the audio codec and bitrate, you can use the following command:
ffmpeg -i input.mp4 -b:a 192k -acodec mp3 output.mp4
In this example, the audio bitrate is set to 192 kbps, and the audio codec is changed to MP3, providing a favorable output for many audio-centric applications.
When writing scripts for video conversion, it is also vital to think error handling and logging. You can capture errors and log output to a file, allowing you to diagnose problems later. Here’s a simple way to log the output of your FFmpeg command:
ffmpeg -i input.mp4 output.mp4 > conversion.log 2>&1
This command redirects both standard output and standard error to conversion.log
, enabling you to review the conversion process, including any errors that may have occurred.
To make your script more effortless to handle, consider adding input parameters. This allows users to specify their own input and output files without modifying the script. Here’s a basic example:
#!/bin/bash input_file="$1" output_file="$2" ffmpeg -i "$input_file" -b:v 1M "$output_file" > conversion.log 2>&1
In this script, $1
and $2
represent the first and second command-line arguments, respectively. This approach enhances the script’s flexibility and usability.
By combining the power of Bash with FFmpeg and implementing these techniques, you can create versatile scripts capable of handling a variety of video conversion tasks. The possibilities are limited only by your creativity and the specific requirements of your project.
Automating Video Editing Tasks with Bash
Automating video editing tasks with Bash can significantly streamline your workflow, enabling you to perform repetitive actions quickly and efficiently. The true power of automation comes from the ability to chain commands together and utilize the strengths of different tools. For instance, if you frequently need to trim videos, apply filters, or concatenate multiple video files, scripting these tasks in Bash can save you a considerable amount of time.
To illustrate this, let’s consider a common requirement: trimming a video to a specific duration. Using FFmpeg, you can automate this process with a simple script. Here’s how you can create a script that trims a video to a desired length:
#!/bin/bash input_file="$1" start_time="$2" duration="$3" output_file="$4" ffmpeg -ss "$start_time" -i "$input_file" -t "$duration" -c:v copy -c:a copy "$output_file" > trimming.log 2>&1
In this script, you provide the input file, the start time for trimming, the duration, and the output file as command-line arguments. The FFmpeg command uses the -ss option to specify the start time and the -t option for the duration, while using -c:v copy and -c:a copy to copy the video and audio streams without re-encoding, which speeds up the process.
Another common task is applying a filter to a video, such as adding a watermark. This can also be automated with a Bash script using FFmpeg. Here’s an example:
#!/bin/bash input_file="$1" watermark_file="$2" output_file="$3" ffmpeg -i "$input_file" -i "$watermark_file" -filter_complex "overlay=10:10" "$output_file" > watermarking.log 2>&1
This script overlays a watermark image onto the input video at coordinates (10,10). The -filter_complex option allows you to specify complex filtering operations, rendering it effortless to add visual elements to your videos programmatically.
Concatenating multiple video files is another frequent requirement in video editing. With FFmpeg, this can be efficiently automated as well. First, you need to create a text file listing all the video files you want to concatenate:
# Create a text file named files.txt with the following format: file 'video1.mp4' file 'video2.mp4' file 'video3.mp4'
Then, you can use the following Bash script to concatenate the videos listed in the text file:
#!/bin/bash input_file_list="$1" output_file="$2" ffmpeg -f concat -safe 0 -i "$input_file_list" -c copy "$output_file" > concatenation.log 2>&1
This script takes the name of the text file containing the list of input videos and the desired output file name as arguments. The -f concat option tells FFmpeg to use the concat demuxer, which allows for seamless joining of videos.
Moreover, for automating tasks that require condition checks or looping through a list of files, Bash’s control structures come in handy. For example, if you want to apply a certain effect to every video file in a directory, you can use a loop:
#!/bin/bash for video in *.mp4; do output_file="processed_$video" ffmpeg -i "$video" -vf "scale=640:360" "$output_file" > processing.log 2>&1 done
This script iterates over every MP4 file in the current directory, processes it to scale it down to a resolution of 640×360 pixels, and saves the output with a prefix of “processed_”. By using loops and conditionals, you can create sophisticated automation scripts that handle a wide range of video editing tasks.
In essence, by combining Bash scripting with the capabilities of tools like FFmpeg, you can automate most of your video editing workflow. This not only enhances efficiency but also ensures consistency across your video processing tasks, which will allow you to focus more on the creative aspects of your projects while leaving the repetitive tasks to your scripts.
Batch Processing Videos Using Loops
#!/bin/bash for video in *.mp4; do output_file="processed_$video" ffmpeg -i "$video" -vf "scale=640:360" "$output_file" > processing.log 2>&1 done
Batch processing videos using loops in Bash is a powerful technique that can significantly enhance your productivity when dealing with large collections of video files. The concept revolves around automating repetitive tasks, allowing you to apply a series of commands to multiple files without manual intervention.
To begin with, think a scenario where you have a directory filled with video files that you want to convert to a different format or modify in some way. Instead of processing each file individually—an approach that can be tedious and error-prone—you can harness the power of a simple loop in your Bash script.
Here’s a classic example: let’s say you want to convert all MP4 files in a directory to AVI format. The following Bash script demonstrates how to accomplish this:
#!/bin/bash for video in *.mp4; do output_file="${video%.mp4}.avi" ffmpeg -i "$video" "$output_file" > conversion.log 2>&1 done
In this script, the `for` loop iterates through each MP4 file in the current directory. The variable `video` holds the name of each file as the loop progresses. The `${video%.mp4}.avi` syntax is a parameter expansion that modifies the file name, removing the `.mp4` extension and appending `.avi` instead. This way, every converted file retains its original name while adopting the new format. The output and any potential errors are logged to `conversion.log`, ensuring you have a record of the process.
Now, suppose you need to apply a specific filter to each video, such as adjusting the brightness. In this case, your script can be easily modified to incorporate the necessary command:
#!/bin/bash for video in *.mp4; do output_file="bright_$video" ffmpeg -i "$video" -vf "eq=brightness=0.05" "$output_file" > filter.log 2>&1 done
Here, the `-vf “eq=brightness=0.05″` option applies an equalizer filter that increases the brightness of the video. The output file is prefixed with `bright_` to distinguish it from the original.
Batch processing also becomes advantageous when resizing videos for various platforms. Consider a situation where you need to resize all videos in a directory to 1280×720 resolution. The following script accomplishes this:
#!/bin/bash for video in *.mp4; do output_file="${video%.mp4}_720p.mp4" ffmpeg -i "$video" -s 1280x720 "$output_file" > resizing.log 2>&1 done
Notice how we’ve reused the parameter expansion to create output file names that reflect the new resolution. By running this script, all MP4 files will be resized and saved with the new naming convention, making it clear that they have been processed.
Error handling is critical in batch processing, especially when dealing with numerous files. You can enhance your script to log errors more effectively by checking the exit status of each FFmpeg command:
#!/bin/bash for video in *.mp4; do output_file="${video%.mp4}.avi" if ffmpeg -i "$video" "$output_file" >&2; conversion.log; then echo "Successfully processed $video" >&2; success.log else echo "Failed to process $video" >&2; error.log fi done
In this version, the script checks if the FFmpeg command was successful. If it was, it logs a success message; if not, it logs an error. This added layer of monitoring can help you quickly identify problematic files without sifting through a massive log file.
In summary, using loops in Bash for batch processing video files opens up a world of possibilities for efficient multimedia management. You can automate tasks, minimize manual errors, and maintain a structured workflow, all while enjoying the flexibility that scripting offers. With each iteration of your script, you can refine and expand your processing capabilities, ultimately shaping a streamlined approach to video editing and conversion that scales with your needs.
Error Handling and Logging in Video Scripts
Error handling and logging are pivotal aspects when writing Bash scripts for video processing, especially given the potential complexities involved. Video files may be corrupted, incompatible formats may arise, or the processing itself might encounter unforeseen issues, leading to errors that can disrupt your workflow. Therefore, implementing robust error handling and logging mechanisms can help you troubleshoot and maintain a smooth operation.
At its core, error handling in a Bash script involves checking the exit status of commands. In Bash, every command returns an exit status, where a status of 0 signifies success and any non-zero value indicates an error. Using this feature especially important in video processing scripts, particularly when relying on external tools like FFmpeg. For instance, you can use an if statement to check whether the FFmpeg command executed successfully:
#!/bin/bash input_file="$1" output_file="$2" ffmpeg -i "$input_file" "$output_file" if [ $? -ne 0 ]; then echo "Error: Conversion failed for $input_file" >&2; error.log else echo "Successfully converted $input_file to $output_file" > success.log fi
In this example, after attempting to convert a video file, the script checks the exit status using `$?`. If the command failed (i.e., exit status not equal to 0), it logs an error message to `error.log`, allowing for easy identification of issues. Conversely, a success message is recorded in `success.log` for successfully processed files.
Logging both outputs and errors is essential for diagnosing problems later. Redirecting command output to log files can be achieved by using the following syntax:
ffmpeg -i "$input_file" "$output_file" > conversion.log 2>&1
This command redirects both standard output and standard error to a single file, `conversion.log`. However, separating the logs can sometimes be beneficial, providing clearer insight into what occurred during processing:
ffmpeg -i "$input_file" "$output_file" > output.log 2> error.log
By logging output and errors separately, you can easily track what was processed and what issues occurred without sifting through a combined file. Additionally, you can enhance your logging by including timestamps, which is particularly useful when processing large batches of files:
echo "$(date): Started processing $input_file" > process.log ffmpeg -i "$input_file" "$output_file" >&2; error.log if [ $? -ne 0 ]; then echo "$(date): Error processing $input_file" >&2; error.log else echo "$(date): Successfully processed $input_file" > process.log fi
In this scenario, each log entry is prefixed with the current date and time, helping you track when each file was processed or when an error occurred. This level of detail can be invaluable when troubleshooting long-running scripts.
Moreover, incorporating functions into your Bash scripts can further streamline error handling and logging. For instance, you could create a dedicated logging function to handle all log entries, ensuring consistency:
log_message() { local message="$1" local log_type="$2" echo "$(date): $message" >&2; "${log_type}.log" } ffmpeg -i "$input_file" "$output_file" if [ $? -ne 0 ]; then log_message "Error processing $input_file" "error" else log_message "Successfully processed $input_file" "process" fi
This function takes a message and a log type as parameters, which will allow you to easily log messages to the appropriate file with a timestamp, enhancing maintainability and reducing code duplication.
Effective error handling and logging not only aid in identifying and resolving issues but also contribute to the overall reliability of your video processing scripts. By implementing these practices, you’ll ensure that your Bash scripts are not only powerful but also resilient, providing a smooth experience in your video processing endeavors.