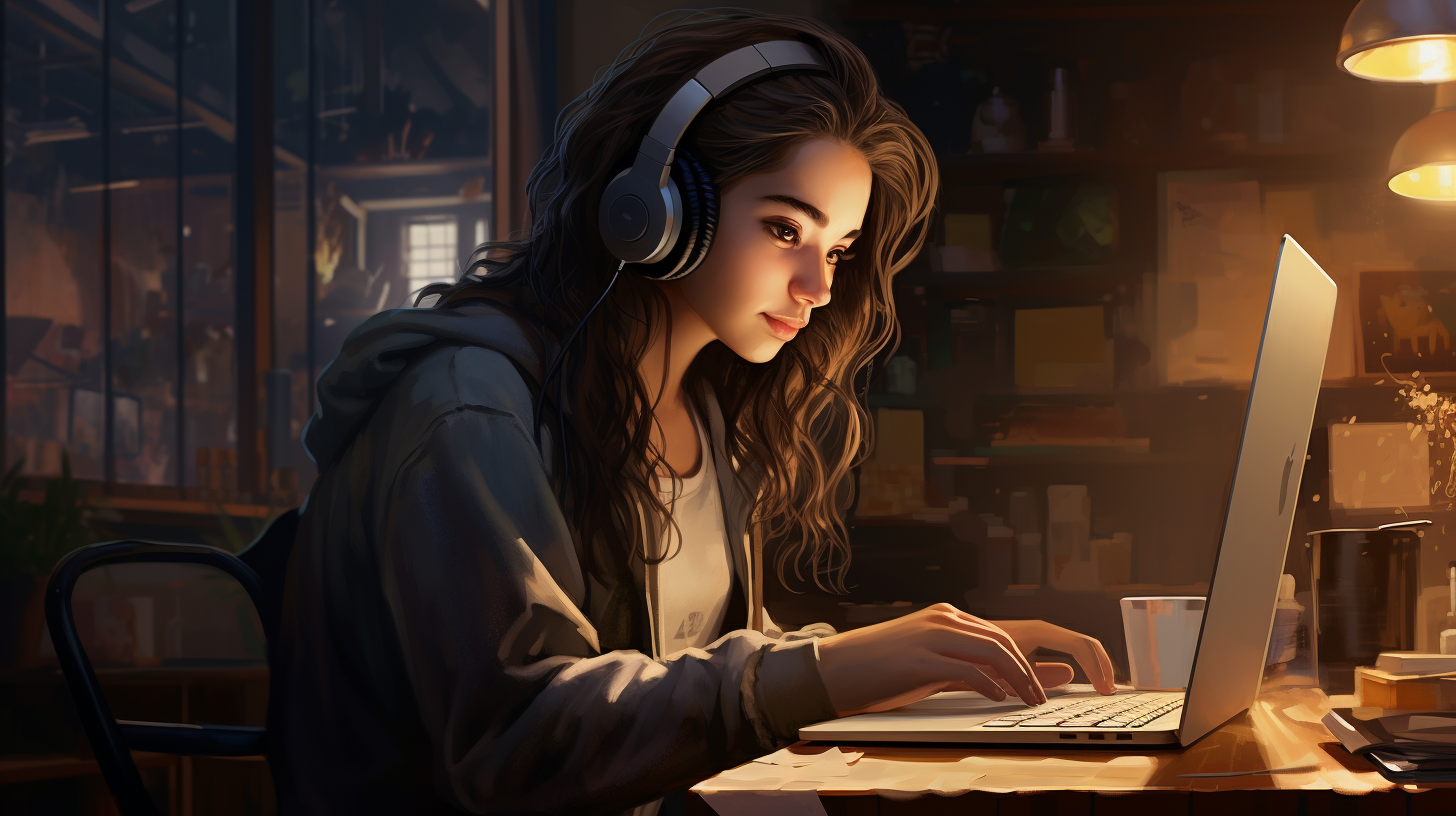
Building Efficient SQL Subqueries
Subqueries, also known as nested queries or inner queries, are a powerful feature of SQL that enable you to perform complex operations by embedding one query within another. Understanding the different types of subqueries and their use cases very important for effective database management and optimization.
There are primarily two types of subqueries:
- These return a single row from the inner query. They are often used when you need to compare a value to a single result. For example:
SELECT employee_id, name FROM employees WHERE salary > (SELECT AVG(salary) FROM employees);
In this example, the outer query retrieves employees whose salaries exceed the average salary calculated by the inner query.
- These return multiple rows and are often used with operators like
IN
,ANY
, orALL
. For instance:
SELECT name FROM products WHERE category_id IN (SELECT category_id FROM categories WHERE category_name LIKE 'Electronics%');
This query retrieves product names that belong to any category related to electronics, using the results from the inner query.
Subqueries can appear in various clauses of a SQL statement, including:
- Used to compute a value for each row returned by the outer query.
- Allows subqueries to serve as derived tables.
- Helps in filtering results based on the conditions defined in the inner query.
- Useful for filtering aggregated results based on the output of a subquery.
Subqueries can also be categorized based on their execution context:
- These depend on the outer query for their values and are executed once for each row processed by the outer query. An example is:
SELECT e1.name FROM employees e1 WHERE e1.salary > (SELECT AVG(e2.salary) FROM employees e2 WHERE e1.department_id = e2.department_id);
Here, the inner query calculates the average salary for the department of each employee individually.
- These are independent of the outer query and can be executed on their own. The previous examples of single-row and multi-row subqueries fall into this category.
Understanding the nuances of subqueries is essential for using their capabilities effectively. They can simplify complex SQL queries and make them more readable, albeit with potential performance costs that need careful consideration in larger datasets.
Best Practices for Writing Efficient Subqueries
When it comes to writing efficient SQL subqueries, there are several best practices you can adopt to enhance performance and maintainability. By following these guidelines, you can minimize the drawbacks often associated with the use of subqueries, such as decreased readability and potential performance issues.
1. Use EXISTS Instead of IN
In many cases, replacing an IN clause with EXISTS can lead to better performance. This is particularly true when the inner query returns a large number of results. The EXISTS operator checks for the existence of rows returned by the inner subquery, which can lead to more optimized execution plans. Ponder the following comparison:
SELECT name FROM products WHERE EXISTS (SELECT 1 FROM categories WHERE categories.category_id = products.category_id AND category_name LIKE 'Electronics%');
In contrast, using IN might not optimize the execution path as effectively, especially with large datasets.
2. Favor JOINs Over Subqueries When Appropriate
While subqueries can be quite powerful, they’re not always the best choice. In situations where you are retrieving data from two or more tables, consider using joins instead. Joins tend to be more efficient as they allow the database engine to optimize the query execution. Here’s an example of a join that can replace a subquery:
SELECT p.name FROM products p JOIN categories c ON p.category_id = c.category_id WHERE c.category_name LIKE 'Electronics%';
This eliminates the need for a nested query, thus improving performance.
3. Limit the Number of Rows Returned
When writing subqueries, it’s wise to limit the number of rows that the inner query returns. Using clauses like LIMIT or adding conditions in the WHERE clause can drastically reduce the size of the dataset that the outer query must process. For example:
SELECT employee_id, name FROM employees WHERE salary > (SELECT AVG(salary) FROM employees WHERE department_id = 1 LIMIT 1);
By ensuring the inner query returns only the necessary data, you improve the efficiency of the overall query.
4. Avoid Correlated Subqueries When Possible
Correlated subqueries can be particularly taxing on performance, as they’re executed repeatedly for each row processed by the outer query. Whenever possible, refactor your queries to eliminate the need for correlated subqueries. For instance, instead of this correlated subquery:
SELECT e1.name FROM employees e1 WHERE e1.salary > (SELECT AVG(salary) FROM employees e2 WHERE e1.department_id = e2.department_id);
You could achieve the same result more efficiently with a join or a derived table.
5. Consider Temporary Tables for Complex Logic
For particularly complex subqueries, ponder using temporary tables to store intermediary results. This approach can help break down complex logic into manageable parts and allow the database to optimize the execution plan better. Here’s a simple example:
CREATE TEMPORARY TABLE avg_salaries AS SELECT department_id, AVG(salary) AS avg_salary FROM employees GROUP BY department_id; SELECT e.name FROM employees e JOIN avg_salaries a ON e.department_id = a.department_id WHERE e.salary > a.avg_salary;
This method reduces the complexity of the queries and can enhance readability and performance.
By adhering to these best practices, you can write SQL subqueries that are not only functional but also efficient and maintainable, enabling you to harness the full power of SQL without running into performance bottlenecks.
Performance Analysis: Subqueries vs. Joins
When analyzing performance between subqueries and joins, it becomes vital to understand how each approach interacts with the database engine, particularly regarding execution plans and resource usage. As a general rule, joins are often more efficient than subqueries in scenarios where you need to retrieve data from multiple tables. However, there are conditions under which subqueries can be just as effective, if not more so, than their join counterparts.
To illustrate the performance differences, let’s consider a scenario where you want to find employees whose salaries are above the average salary in their respective departments.
SELECT name FROM employees WHERE salary > (SELECT AVG(salary) FROM employees WHERE department_id = employees.department_id);
This query utilizes a correlated subquery to determine the average salary for each employee’s department. While this approach is simpler, it can lead to significant performance issues, especially in large datasets, because the inner query runs for every row in the outer query.
Now, let’s explore how we can rewrite the same logic using joins:
SELECT e1.name FROM employees e1 JOIN (SELECT department_id, AVG(salary) AS avg_salary FROM employees GROUP BY department_id) e2 ON e1.department_id = e2.department_id WHERE e1.salary > e2.avg_salary;
In this case, we first create a derived table that calculates the average salary per department, which is subsequently joined with the employees table. This method leverages a single scan through the employees table for the average salary calculation, resulting in fewer total operations and a more efficient execution plan.
When evaluating performance, consider the following aspects:
- Database engines create execution plans that dictate how queries are executed. Joins typically result in simpler execution plans. Use tools like
EXPLAIN
orEXPLAIN ANALYZE
to analyze and compare execution plans for both subqueries and joins. - Joins can often take advantage of indexes on foreign key relationships, leading to faster lookups. Subqueries, particularly correlated ones, may not benefit from indexing in the same way, leading to full table scans.
- While performance especially important, the clarity of your SQL code shouldn’t be overlooked. Sometimes, a subquery may be more intuitive, though you should always weigh this against potential performance drawbacks.
Ultimately, the decision between using subqueries and joins should hinge on the specific context of the query, the size of the dataset, and the required performance. In many cases, opting for joins will yield better performance outcomes, but subqueries can still play a valuable role when used judiciously.
Optimizing Subqueries with Indexing Strategies
When it comes to optimizing subqueries, indexing strategies play an important role in enhancing performance. Indexes are database objects that improve the speed of data retrieval operations on a database table at the cost of additional space and maintenance overhead. By strategically applying indexing, you can significantly reduce the execution time of subqueries, especially when dealing with large datasets.
One approach to optimizing subqueries is to ensure that the columns involved in the subquery conditions are indexed. For example, if you frequently use a subquery that filters based on a specific column, indexing that column can improve the performance of both the subquery and the outer query:
CREATE INDEX idx_department_id ON employees(department_id);
With this index in place, any subquery that references the department_id
column can execute more quickly. Consider the following correlated subquery that retrieves employees with salaries above the average for their respective departments:
SELECT e1.name FROM employees e1 WHERE e1.salary > (SELECT AVG(e2.salary) FROM employees e2 WHERE e1.department_id = e2.department_id);
By having an index on department_id
, the database engine can quickly locate entries in the employees
table that match the criteria, improving the overall efficiency of the execution.
Furthermore, consider the use of composite indexes when your subqueries involve multiple columns. A composite index allows you to index a combination of columns, which can be particularly useful when the subquery conditions span multiple fields:
CREATE INDEX idx_salary_department ON employees(department_id, salary);
This index would be beneficial in scenarios where you’re filtering based on both department_id
and salary
, thus speeding up queries that involve these conditions in subqueries.
Another important aspect of indexing strategies is understanding when to use indexed views or materialized views. If your subquery involves complex calculations or aggregations that are frequently accessed, ponder creating an indexed view. An indexed view stores the result set of a query physically and allows for faster access:
CREATE VIEW vw_avg_salaries WITH SCHEMABINDING AS SELECT department_id, AVG(salary) AS avg_salary FROM employees GROUP BY department_id; CREATE UNIQUE CLUSTERED INDEX idx_vw_avg_salaries ON vw_avg_salaries(department_id);
With the indexed view in place, you can simplify your main query while benefiting from the pre-computed averages, thus drastically improving performance:
SELECT e.name FROM employees e JOIN vw_avg_salaries a ON e.department_id = a.department_id WHERE e.salary > a.avg_salary;
Indexing strategies should always be accompanied by monitoring and analysis. Use database performance monitoring tools to evaluate query execution times, index usage statistics, and overall database performance. Regularly assess whether existing indexes are still beneficial or if adjustments are necessary based on changing query patterns.
The optimization of subqueries through effective indexing can lead to substantial performance enhancements. By understanding how indexes work and applying them strategically, you can ensure that your SQL queries not only perform optimally but also scale gracefully as your data grows.
Common Pitfalls and How to Avoid Them
Working with SQL subqueries can be rewarding, but they come with their own set of common pitfalls that can hinder performance and lead to inefficient database interactions. Recognizing these pitfalls and actively avoiding them is essential for any database developer or administrator striving for optimal query execution. Here are some common issues to watch out for:
1. Overuse of Correlated Subqueries
Correlated subqueries, while powerful, can significantly impact performance due to their repeated execution for each row processed by the outer query. This results in unnecessary overhead, particularly in large datasets. For instance, when trying to find employees earning above the average salary in their department, a correlated subquery could look like this:
SELECT e1.name FROM employees e1 WHERE e1.salary > (SELECT AVG(e2.salary) FROM employees e2 WHERE e1.department_id = e2.department_id);
This query runs the inner AVG calculation for each employee, which can lead to performance degradation. Instead, you can rewrite it using a join or a derived table to calculate the averages first and then filter:
SELECT e1.name FROM employees e1 JOIN (SELECT department_id, AVG(salary) AS avg_salary FROM employees GROUP BY department_id) e2 ON e1.department_id = e2.department_id WHERE e1.salary > e2.avg_salary;
2. Neglecting to Use Indexes
Indexes are vital for speeding up data retrieval. Failing to utilize indexes, especially on columns referenced in subqueries, can lead to full table scans, which are slow and resource-intensive. For example, if your subquery filters based on a specific column, ensure that column is indexed:
CREATE INDEX idx_department_id ON employees(department_id);
This index can greatly enhance the performance of queries that depend on the department_id column, allowing the database to pinpoint relevant rows faster.
3. Writing Complex and Deeply Nested Subqueries
While SQL allows for the nesting of subqueries, overly complex or deeply nested queries can lead to confusion and inefficiency. Each level of nesting can introduce additional processing time and make the query harder to read and maintain. Instead, try to break down complex logic into smaller, simpler queries or use temporary tables to manage intermediary results:
CREATE TEMPORARY TABLE avg_salaries AS SELECT department_id, AVG(salary) AS avg_salary FROM employees GROUP BY department_id;
Then, you can easily join this temporary table with the employees table to filter results:
SELECT e.name FROM employees e JOIN avg_salaries a ON e.department_id = a.department_id WHERE e.salary > a.avg_salary;
4. Ignoring Execution Plans
Execution plans are critical to understanding how SQL queries are executed. Ignoring them can lead to missed opportunities for optimization. Use tools like EXPLAIN to analyze your queries’ execution plans. This will provide insights into how the database engine processes your subqueries and can highlight where indexes might be beneficial or where you might need to refactor your queries.
5. Failing to Monitor Query Performance
Finally, neglecting to monitor the performance of your SQL queries can lead to long-term issues. Regularly review and analyze the execution time of your queries, especially after any significant changes in the database or data volume. Implementing logging or using performance monitoring tools can help keep track of how your queries are performing over time and alert you to any potential issues that arise.
By staying vigilant about these common pitfalls, you can craft subqueries that are not only functional but also efficient, paving the way for optimal database performance and maintainability.