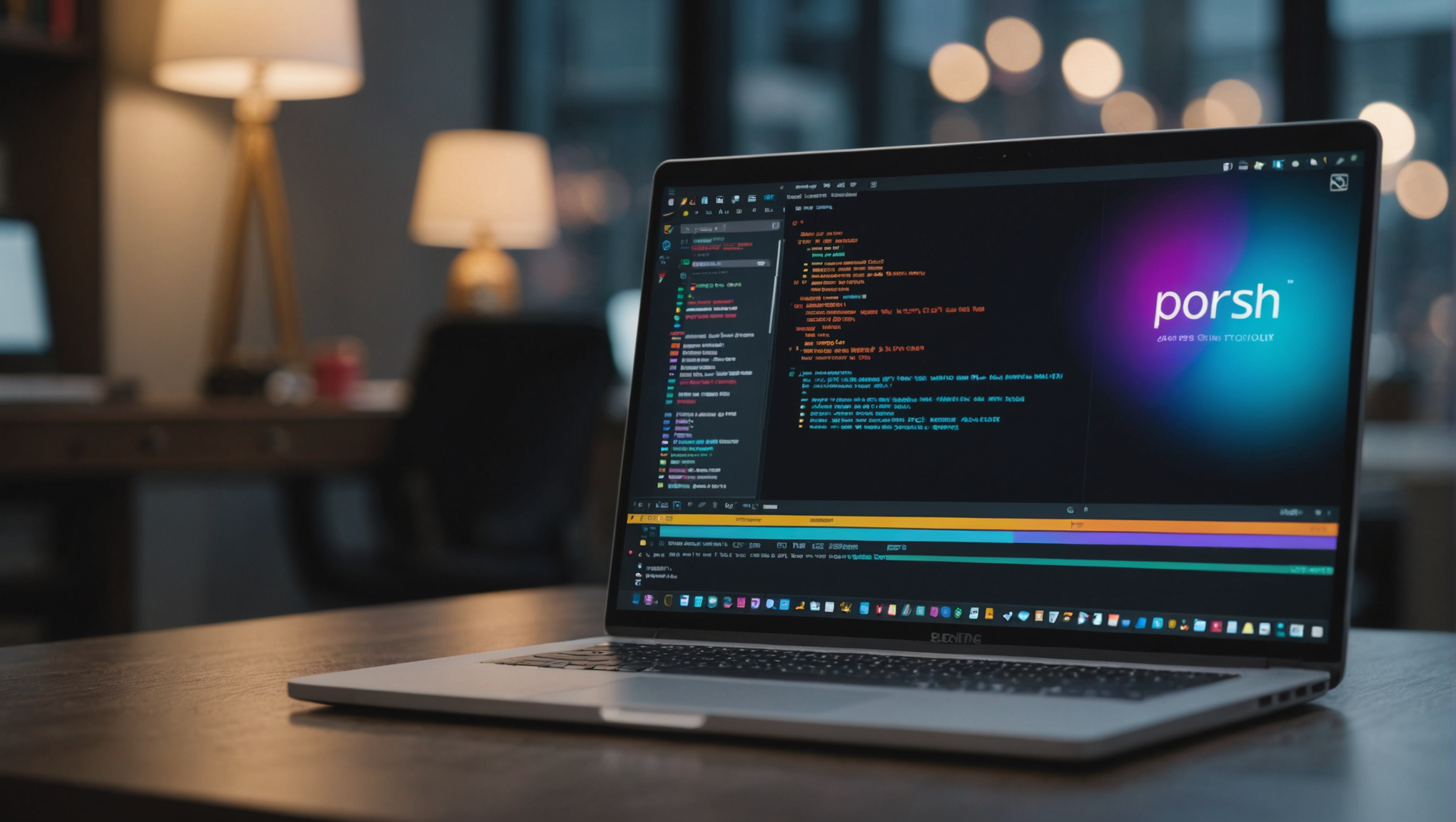
Building Help Systems in Bash Scripts
Designing easy to use help interfaces especially important for ensuring that users can easily understand how to use a Bash script. The aim is to create a simpler and intuitive experience that allows users to quickly access the information they need without excess confusion.
To achieve this, ponder the following principles:
- Use clear and simple language. Avoid jargon unless it’s common in your target audience.
- Maintain a consistent format throughout the help interface. This includes using similar phrasing, punctuation, and structure for commands and options.
- Group related commands and options together, and use sections and subsections to make navigation easier.
- Provide practical examples that demonstrate how to use the commands effectively in real-world scenarios.
A well-designed help interface can be presented through a dedicated help command within the script. Here’s a practical example of how to implement this:
#!/bin/bash function show_help { echo "Usage: script.sh [OPTIONS]" echo "" echo "Options:" echo " -h, --help Show this help message" echo " -v, --version Show the script version" echo "" echo "Examples:" echo " ./script.sh -v" echo " ./script.sh --help" } # Main script logic if [[ "$1" == "-h" || "$1" == "--help" ]]; then show_help exit 0 fi if [[ "$1" == "-v" || "$1" == "--version" ]]; then echo "Script Version 1.0" exit 0 fi # Rest of the script goes here...
In this example, the show_help
function is responsible for displaying usage information and examples if the user invokes the help option. This function clearly outlines the commands, options, and usage patterns, making it easier for users to understand how to interact with the script.
In addition to the basics, consider the use of color-coding or formatting options to highlight important sections of the help output, making it even more uncomplicated to manage. Bash’s built-in echo
command can be enhanced with ANSI escape codes to add color:
function show_help { echo -e "33[1;34mUsage:33[0m script.sh [OPTIONS]" echo "" echo -e "33[1;32mOptions:33[0m" echo " -h, --help Show this help message" echo " -v, --version Show the script version" echo "" echo -e "33[1;33mExamples:33[0m" echo " ./script.sh -v" echo " ./script.sh --help" }
This level of attention to detail in your help interface design will not only improve user experience but also enhance the overall usability of your Bash scripts. Crafting a uncomplicated to manage help interface is an art that, when done right, invites users to engage more confidently with your scripts.
Implementing Command-Line Arguments for Help
Having established the importance of a simple to operate help interface, we now turn our attention to implementing command-line arguments specifically for help functionalities. This phase is where we define how users will interact with our script to access the help they need. Command-line arguments are essential not just for triggering help displays but also for enhancing the script’s flexibility and usability.
When a script is executed, it can accept specific flags or options, which can seamlessly lead to displaying help information. A common practice is to include a -h
or --help
flag, as previously highlighted. However, it’s crucial to construct your argument parsing in a way that allows for scalability and clarity.
Here’s how you can implement command-line argument handling in a Bash script:
#!/bin/bash function show_help { echo "Usage: script.sh [OPTIONS]" echo "" echo "Options:" echo " -h, --help Display this help message" echo " -v, --version Show the script version" echo " -o, --option An example option" echo "" echo "Examples:" echo " ./script.sh --help" echo " ./script.sh --version" } # Handle command-line arguments while [[ $# -gt 0 ]]; do case $1 in -h|--help) show_help exit 0 ;; -v|--version) echo "Script Version 1.0" exit 0 ;; -o|--option) # Additional option handling can be implemented here echo "Option selected: $1" shift # Move to the next argument ;; *) # Unknown option echo "Error: Unknown option $1" show_help exit 1 ;; esac shift # Move to the next argument done # Main script functionality goes here...
In this script, we utilize a while
loop combined with a case
statement to process each command-line argument. This structure allows the script to handle multiple flags in a single execution and provides room for future expansions, like adding more options without cluttering the code.
The shift
command is particularly useful here; it enables the script to traverse through all provided arguments effectively. This method not only aids in displaying help information but also in implementing features that respond to user input dynamically.
Additionally, you could further enhance the user experience by providing feedback for invalid options, thus guiding users back to the correct usage. Always ensure that your help function is descriptive enough to provide users with a clear understanding of available options and their purposes. By doing so, you lay the groundwork for a robust, effortless to handle command-line interface that ultimately reflects well on the quality of your script.
Structuring Help Content for Clarity
Structuring help content for clarity is a fundamental aspect of building effective help systems in Bash scripts. When users access help information, they should be greeted with a well-organized layout that makes navigation intuitive and content easy to digest. It’s essential to break down the help content into logical sections, ensuring that users can quickly find the information they need.
The first step is to categorize the help content into distinct sections. Common sections include usage instructions, option descriptions, examples, and additional resources. Each section should be clearly labeled and formatted to enhance readability. Here’s how you can implement structured help content in your Bash script:
#!/bin/bash function show_help { echo "Usage: script.sh [OPTIONS]" echo "" echo "Options:" echo " -h, --help Display this help message" echo " -v, --version Show the script version" echo " -o, --option An example option" echo "" echo "Examples:" echo " ./script.sh --help" echo " ./script.sh --version" echo "" echo "For more information, visit our documentation at: https://example.com/docs" } # Main script logic if [[ "$1" == "-h" || "$1" == "--help" ]]; then show_help exit 0 fi
In this example, the show_help
function is structured to provide a clear hierarchy of information. Each part starts with a brief statement followed by specific details, grouped logically. The use of whitespace helps separate different sections visually, making it easier for users to follow.
To enhance the clarity of your help content further, ponder the following best practices:
- When listing options or features, consider using bullet points or numbered lists to improve readability.
- Use consistent terminology throughout the help content. This prevents confusion and helps users familiarize themselves with the script’s language.
- Provide clear, practical examples that demonstrate how to use the script effectively. Real-world scenarios help users visualize how each option can be applied.
Here’s an enhanced version of the help function that incorporates bullet points and consistent terminology:
function show_help { echo "Usage: script.sh [OPTIONS]" echo "" echo "Options:" echo " -h, --help Display this help message" echo " -v, --version Show the script version" echo " -o, --option An example option that performs a specific task" echo "" echo "Examples:" echo " ./script.sh --version # Displays the version of the script" echo " ./script.sh --option value # Executes the script with an option" echo "" echo "For further information, please check the documentation at: https://example.com/docs" }
By implementing these strategies, you not only foster a more effortless to handle experience but also build a help system that stands as a testament to the script’s overall quality. Users will appreciate the effort put into providing clear and effective help content, making it far more likely that they’ll utilize your script efficiently and effectively.
Using Built-in Help Features in Bash
Using built-in help features in Bash can significantly enhance the usability of your scripts, providing users with quick access to information without needing to delve into external documents or complex interfaces. Bash itself offers several established mechanisms for implementing help systems, which can be leveraged to improve user satisfaction and efficiency.
One of the most simpler methods is to use the built-in help command. This command is commonly used in Bash to provide users with information about built-in shell commands. While it primarily serves to inform users about the shell itself, you can take inspiration from its structure when crafting your own scripts. Here’s a practical example of how to create a help function that mimics the style of Bash’s built-in help:
#!/bin/bash function show_help { echo "Built-in Help for My Script" echo "" echo "Usage: script.sh [OPTIONS]" echo "" echo "Options:" echo " -h, --help Display this help message" echo " -v, --version Show the script version" echo " -o, --option VALUE An example option with a value" echo "" echo "For more information, visit the official documentation or run the script with --help." } # Main execution logic if [[ "$1" == "-h" || "$1" == "--help" ]]; then show_help exit 0 fi
This function provides a succinct overview of the script, including usage information and a description of options. The layout is designed to be clean and clear, drawing upon the conventions of built-in help commands to create familiarity for users.
Another useful feature is the use of the “type” command, which can help users understand what a command does, especially when it is a function within the script. You can incorporate this into your help system to provide additional context:
function show_help { echo "Available commands:" echo " $(type -t command_name) command_name # Description of what command_name does" # Add more commands as needed... }
Moreover, consider implementing a version command within your script that provides the user with the current version and a brief change log if applicable. This not only helps with troubleshooting but also keeps users informed about the latest improvements:
function show_version { echo "Script Version: 1.0.0" echo "Last Updated: $(date '+%Y-%m-%d')" echo "Change Log: Initial release." } if [[ "$1" == "-v" || "$1" == "--version" ]]; then show_version exit 0 fi
Incorporating these built-in help features not only enhances the functionality of your script but also aligns it with user expectations based on their experience with Bash itself. This familiarity can greatly reduce the learning curve for new users and improve overall satisfaction with your script.
Ultimately, using built-in help features in Bash is about striking a balance between functionality and user experience. By providing accessible, clear, and helpful information, you empower users to make the most of your script, reinforcing its value and effectiveness in their workflow.