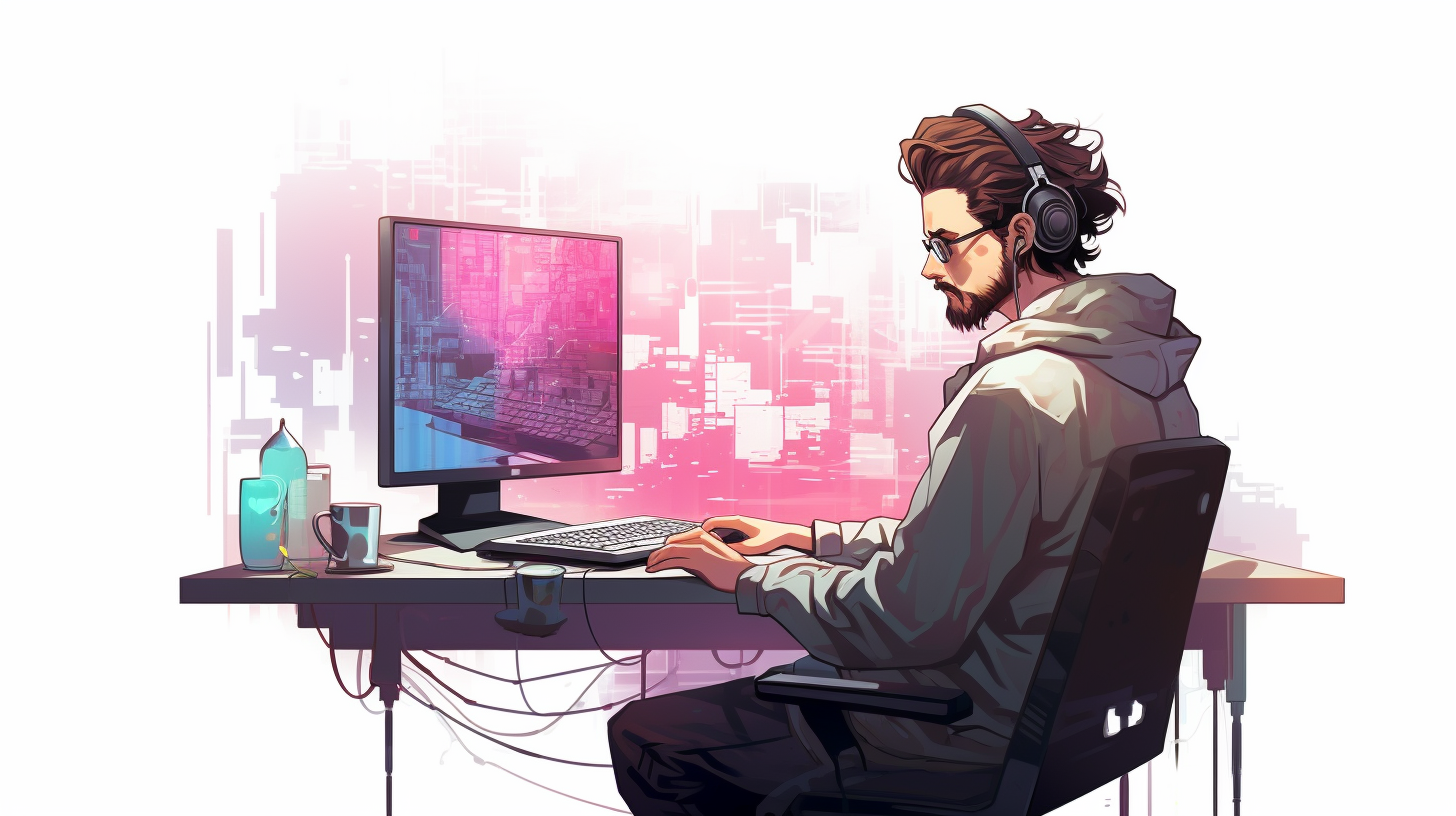
Building Menus in Bash Scripts
Within the scope of Bash scripting, menu structures serve as the backbone for user interaction. A well-constructed menu not only enhances usability but also provides a logical flow for users, guiding them through the various functionalities of a script. At its core, a Bash menu typically consists of a series of options presented to the user, which they can select to execute specific commands or functions.
To effectively implement a menu in Bash, one must ponder several key components: the presentation of options, the mechanism for capturing user input, and the subsequent actions associated with each choice. The foundational structure of a Bash menu is often a simple loop that provides options until the user chooses to exit.
Here’s a basic example of a menu structure in a Bash script:
#!/bin/bash while true; do echo "Please select an option:" echo "1) Option One" echo "2) Option Two" echo "3) Exit" read -p "Enter your choice: " choice case $choice in 1) echo "You selected Option One." ;; 2) echo "You selected Option Two." ;; 3) echo "Exiting..." break ;; *) echo "Invalid option, please try again." ;; esac done
In this script, a loop is established using while true
, which ensures that the menu is displayed repeatedly until the user opts to exit. The read
command captures user input, and the case
statement directs the flow based on that input. The use of *)
provides a simple error handling mechanism for invalid selections, enhancing the overall robustness of the menu.
Understanding the structure of menus in Bash is not just about displaying options; it is about designing a user experience that is intuitive and efficient. By carefully considering the organization and flow of your menu, you can create scripts that are not only functional but also easy to use.
Creating Simple Text-based Menus
Creating simple text-based menus in Bash is an exercise in clarity and efficiency. A well-designed menu should present options in a manner this is easy to read and navigate, allowing users to make selections quickly. The fundamental components of a text-based menu include clear prompts, intuitive options, and a simpler selection process.
To illustrate this, let’s expand on our previous example by adding a bit more complexity while maintaining simplicity. Ponder a scenario where we want to provide the user with options to manage files in a directory. Our script may look like this:
#!/bin/bash while true; do echo "File Management Menu" echo "1) List Files" echo "2) Create a New File" echo "3) Delete a File" echo "4) Exit" read -p "Enter your choice: " choice case $choice in 1) echo "Listing files in the current directory:" ls ;; 2) read -p "Enter the name of the new file: " filename touch "$filename" echo "File '$filename' created." ;; 3) read -p "Enter the name of the file to delete: " filename rm -i "$filename" ;; 4) echo "Exiting..." break ;; *) echo "Invalid option, please try again." ;; esac done
In this script, we introduced additional functionality: the ability to list files, create a new file, and delete an existing file. Each option is followed by a specific command that executes upon selection. The use of the ls
command allows users to see their current files, while the touch
command facilitates file creation, and the rm -i
command prompts for confirmation before deletion, adding a layer of safety.
Notice how we utilized the read -p
command to prompt the user for input directly, which eliminates the need for additional echo statements for input instructions. This not only streamlines the code but also improves its readability.
Moreover, the use of rm -i
when deleting files ensures that users are prompted before any irreversible action is taken, underscoring the importance of user validation in script development. The case structure remains central to directing user input, providing a clear pathway for the logic based on user choices.
With this approach, the menu not only becomes functional but also enhances the overall user experience. The simplicity of the text-based menu in Bash allows for rapid development and easy modification, ideally suited for scripts that may evolve over time in response to user needs and feedback.
Handling User Input and Navigation
Handling user input and navigation within a Bash menu is a critical aspect that directly affects usability and functionality. The goal is to create an interactive experience where the user feels in control, capable of making selections that lead to desired outcomes without confusion or frustration. To achieve this, it is essential to consider how input is captured and processed while maintaining a smooth navigational flow.
At its core, capturing user input in a Bash script is typically done using the read
command. This command allows the script to pause and wait for the user to type their choice, storing that input in a variable for later use. However, the simplicity of read
should not overshadow the need for effective feedback and validation mechanisms.
To improve the user experience, it’s beneficial to provide clear prompts and instructions. For instance, reiterating the options available after a selection can help remind users of their choices. Additionally, implementing a loop that re-displays the menu after each selection allows users to navigate back to the menu easily. Here’s a refined version of our previous file management menu that incorporates these principles:
#!/bin/bash while true; do clear echo "File Management Menu" echo "1) List Files" echo "2) Create a New File" echo "3) Delete a File" echo "4) Exit" read -p "Enter your choice: " choice case $choice in 1) echo "Listing files in the current directory:" ls read -p "Press Enter to return to the menu..." ;; 2) read -p "Enter the name of the new file: " filename touch "$filename" echo "File '$filename' created." read -p "Press Enter to return to the menu..." ;; 3) read -p "Enter the name of the file to delete: " filename rm -i "$filename" read -p "Press Enter to return to the menu..." ;; 4) echo "Exiting..." break ;; *) echo "Invalid option, please try again." read -p "Press Enter to return to the menu..." ;; esac done
In this script, the clear
command is used at the beginning of each loop iteration to refresh the terminal screen, which helps prevent clutter and keeps the focus on the menu options. After executing any command, the script prompts the user to press Enter before returning to the menu. This ensures that users have ample time to view the results of their actions before the menu reappears, thereby preventing confusion.
Furthermore, it’s important to think the validation of user input. The provided example employs a simple case
structure to handle valid and invalid options. However, for more complex menus, you might want to implement additional validation checks to ensure that the input adheres to expected formats. For instance, if the user input is expected to be a number, using a regex check can further enhance reliability:
read -p "Enter your choice: " choice if [[ ! $choice =~ ^[1-4]$ ]]; then echo "Invalid option, please try again." fi
This snippet checks whether the input matches the range of valid options (1 to 4), providing a more robust method for input validation. By incorporating these techniques, you make the menu more resilient against erroneous inputs, which in turn improves the overall user experience.
Ultimately, handling user input and navigation in Bash menus is about creating a seamless interface that guides users smoothly through their options. By focusing on clarity, responsiveness, and validation, you can develop menus that not only function effectively but also foster an intuitive interaction with your scripts.
Implementing Submenus for Enhanced Functionality
Implementing submenus in Bash scripts is an effective method to enhance functionality and organization within your menus. Submenus allow for a more detailed categorization of options, enabling users to navigate through complex tasks without overwhelming them with choices at a single level. This hierarchical structure not only improves readability but also creates a more structured user experience.
To illustrate how to implement submenus, we can build upon our previous file management script by adding options that pertain to specific file operations. For example, we could introduce a submenu for file manipulation that allows users to view file properties or move files between directories. Below is how such a script might be structured:
#!/bin/bash while true; do clear echo "Main Menu" echo "1) File Management" echo "2) Exit" read -p "Enter your choice: " main_choice case $main_choice in 1) while true; do clear echo "File Management Menu" echo "1) List Files" echo "2) Create a New File" echo "3) Delete a File" echo "4) File Properties" echo "5) Move a File" echo "6) Back to Main Menu" read -p "Enter your choice: " file_choice case $file_choice in 1) echo "Listing files in the current directory:" ls read -p "Press Enter to return to the menu..." ;; 2) read -p "Enter the name of the new file: " filename touch "$filename" echo "File '$filename' created." read -p "Press Enter to return to the menu..." ;; 3) read -p "Enter the name of the file to delete: " filename rm -i "$filename" read -p "Press Enter to return to the menu..." ;; 4) read -p "Enter the name of the file to view properties: " filename ls -l "$filename" read -p "Press Enter to return to the menu..." ;; 5) read -p "Enter the name of the file to move: " filename read -p "Enter the destination directory: " dest_dir mv "$filename" "$dest_dir" echo "File '$filename' moved to '$dest_dir'." read -p "Press Enter to return to the menu..." ;; 6) break ;; *) echo "Invalid option, please try again." read -p "Press Enter to return to the menu..." ;; esac done ;; 2) echo "Exiting..." break ;; *) echo "Invalid option, please try again." ;; esac done
In this script, the user is first presented with a main menu that includes a single entry for file management. Upon selecting this option, a submenu is displayed, providing further options for managing files. Each choice in the submenu corresponds to a specific action related to file operations, such as listing files, creating new files, deleting files, viewing properties, and moving files.
Notice the use of a nested while loop within the file management menu. This structure allows the user to navigate back and forth between the main menu and submenu seamlessly. When the user selects an option in the submenu, the corresponding case handles the action, ensuring that feedback is provided after each operation, thus maintaining an interactive experience.
Submenus can significantly reduce the visual clutter of your script, making it easier for users to find what they’re looking for. As your scripts grow in complexity, ponder using submenus to keep options organized and accessible. This not only enhances usability but also contributes to a more professional and polished appearance of your Bash scripts.
Error Handling and Input Validation
Error handling and input validation are crucial components in the development of Bash scripts, particularly when it comes to menu systems. A well-designed menu not only presents options but also effectively manages user inputs and potential errors that could arise during interactions. The objective is to create a robust user experience that handles unexpected situations gracefully, guiding users back to a stable state without causing frustration.
At the core of any effective error handling strategy is the recognition that users may not always follow the intended path. Invalid inputs can lead to unexpected behavior, which can be detrimental to the overall usability of a script. Therefore, implementing input validation before executing any commands is paramount. This involves checking user input against predefined criteria to ensure that it meets the expected format or value range.
Ponder the following example that enhances our previous file management script by integrating thorough input validation:
#!/bin/bash while true; do clear echo "File Management Menu" echo "1) List Files" echo "2) Create a New File" echo "3) Delete a File" echo "4) Exit" read -p "Enter your choice (1-4): " choice # Input validation for main menu selection if [[ ! $choice =~ ^[1-4]$ ]]; then echo "Invalid option. Please enter a number between 1 and 4." read -p "Press Enter to try again..." continue fi case $choice in 1) echo "Listing files in the current directory:" ls read -p "Press Enter to return to the menu..." ;; 2) read -p "Enter the name of the new file: " filename if [[ -z "$filename" ]]; then echo "File name cannot be empty." read -p "Press Enter to try again..." continue fi touch "$filename" echo "File '$filename' created." read -p "Press Enter to return to the menu..." ;; 3) read -p "Enter the name of the file to delete: " filename if [[ -z "$filename" ]]; then echo "File name cannot be empty." read -p "Press Enter to try again..." continue fi if [[ -e "$filename" ]]; then rm -i "$filename" else echo "File '$filename' does not exist." fi read -p "Press Enter to return to the menu..." ;; 4) echo "Exiting..." break ;; esac done
In this enhanced version, we first validate the user’s choice for the main menu. If the input does not match the expected numeric range (1-4), an error message is presented, and the loop continues, prompting the user to try again. This prevents the script from executing any commands that rely on valid input.
Further validation is added when creating and deleting files. If the user attempts to create or delete a file without providing a name, an appropriate error message is displayed, and they’re prompted to enter a valid name. Additionally, when attempting to delete a file, the script checks if the file exists before attempting to delete it, thereby avoiding unnecessary errors.
Error messages are designed not merely to inform the user of what went wrong but also to guide them toward the correct action. This approach fosters a better user experience by reducing confusion and providing clear instructions on how to proceed.
Implementing such robust error handling and input validation techniques transforms a simple menu into a uncomplicated to manage interface that anticipates common pitfalls. By proactively addressing potential issues, you create a more resilient script that can handle a variety of user inputs without breaking down or producing unintended results.
Ultimately, the goal is to build a Bash menu that not only performs the desired functions but does so in a manner that is intuitive and forgiving. This requires a commitment to crafting thoughtful validation checks and informative feedback, resulting in a script that users will find reliable and easy to navigate.
Advanced Menu Features and Customization Techniques
Advanced menu features and customization techniques can elevate a simple Bash script from functionality to a more engaging user experience. By incorporating enhancements like color coding, dynamic option generation, and improved user feedback mechanisms, you can create a menu that feels polished and professional.
One of the most simpler enhancements is to add color to your menu options. Color can help draw attention to different parts of the menu and make it more visually appealing. Here’s an example of how to implement color in your Bash menu:
#!/bin/bash # Define color codes RED="e[31m" GREEN="e[32m" YELLOW="e[33m" RESET="e[0m" while true; do clear echo -e "${YELLOW}File Management Menu${RESET}" echo -e "${GREEN}1) List Files${RESET}" echo -e "${GREEN}2) Create a New File${RESET}" echo -e "${GREEN}3) Delete a File${RESET}" echo -e "${GREEN}4) Exit${RESET}" read -p "Enter your choice (1-4): " choice if [[ ! $choice =~ ^[1-4]$ ]]; then echo -e "${RED}Invalid option. Please enter a number between 1 and 4.${RESET}" read -p "Press Enter to try again..." continue fi case $choice in 1) echo "Listing files in the current directory:" ls read -p "Press Enter to return to the menu..." ;; 2) read -p "Enter the name of the new file: " filename touch "$filename" echo "File '$filename' created." read -p "Press Enter to return to the menu..." ;; 3) read -p "Enter the name of the file to delete: " filename rm -i "$filename" read -p "Press Enter to return to the menu..." ;; 4) echo "Exiting..." break ;; esac done
In this example, color codes are defined using ANSI escape sequences. The echo command is enhanced with the -e option to interpret these sequences, applying color to the text output. This simple adjustment makes the menu stand out and enhances user engagement.
Another powerful feature to ponder is dynamic option generation. Instead of hardcoding menu options, you can retrieve options from a directory or a configuration file. This makes your script more flexible and adaptable to changes. For instance, if you want to list all text files in a directory and let users choose one to view, your script can look like this:
#!/bin/bash while true; do clear echo "Select a text file to view:" files=(*.txt) # Dynamically generate a list of .txt files if [ ${#files[@]} -eq 0 ]; then echo "No text files found." break fi for i in "${!files[@]}"; do echo "$((i + 1))) ${files[$i]}" done echo "$(( ${#files[@]} + 1 ))) Exit" read -p "Enter your choice: " choice if [[ ! $choice =~ ^[0-9]+$ || $choice -lt 1 || $choice -gt $((${#files[@]} + 1)) ]]; then echo "Invalid option. Please try again." read -p "Press Enter to continue..." continue fi if [ "$choice" -eq $((${#files[@]} + 1)) ]; then echo "Exiting..." break fi selected_file="${files[$((choice - 1))]}" echo "Displaying contents of '$selected_file':" cat "$selected_file" read -p "Press Enter to return to the menu..." done
In this script, the array `files` is populated dynamically with all `.txt` files in the current directory. The user is then presented with a numbered list of these files, making it easy to select one for viewing. This flexibility means that as files are added or removed, the menu adapts accordingly without requiring code changes.
Improving user feedback is another essential aspect of customizing Bash menus. It is important to acknowledge user actions and provide clear guidance. For instance, you might add confirmation prompts before executing destructive actions like file deletion. Building on our previous example, here’s how you might implement such feedback:
#!/bin/bash while true; do clear echo "File Management Menu" echo "1) List Files" echo "2) Create a New File" echo "3) Delete a File" echo "4) Exit" read -p "Enter your choice (1-4): " choice if [[ ! $choice =~ ^[1-4]$ ]]; then echo "Invalid option. Please enter a number between 1 and 4." read -p "Press Enter to try again..." continue fi case $choice in 1) echo "Listing files in the current directory:" ls read -p "Press Enter to return to the menu..." ;; 2) read -p "Enter the name of the new file: " filename touch "$filename" echo "File '$filename' created." read -p "Press Enter to return to the menu..." ;; 3) read -p "Enter the name of the file to delete: " filename if [[ -e "$filename" ]]; then read -p "Are you sure you want to delete '$filename'? (y/n): " confirm if [[ $confirm =~ ^[Yy]$ ]]; then rm "$filename" echo "File '$filename' deleted." else echo "Deletion of '$filename' canceled." fi else echo "File '$filename' does not exist." fi read -p "Press Enter to return to the menu..." ;; 4) echo "Exiting..." break ;; esac done
Here, before deleting a file, the user is prompted to confirm the action, which provides an additional safeguard against accidental deletions. This kind of feedback not only prevents user errors but also makes the script feel more interactive and responsive to user needs.
By integrating advanced features such as color coding, dynamic option generation, and improved feedback mechanisms, you can create a Bash menu this is not only functional but also enhances user experience significantly. These techniques empower users to navigate your scripts with confidence and ease, ultimately leading to a more satisfying interaction with your Bash applications.