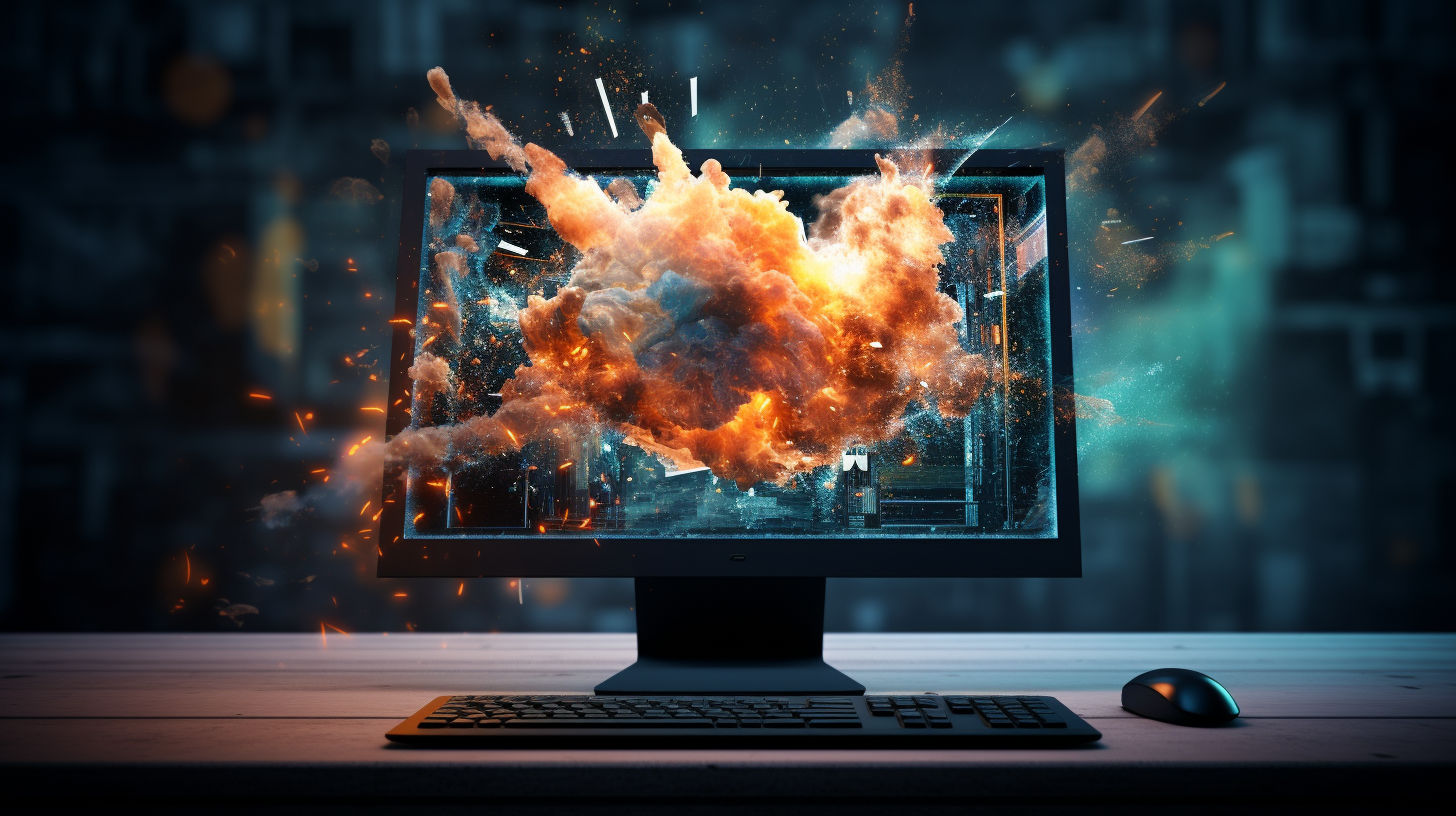
Building RESTful Web Services in Java
RESTful web services represent a paradigm shift in how we design and implement APIs. At its core, REST (Representational State Transfer) is an architectural style that leverages the existing capabilities of the web to facilitate communication between clients and servers. Understanding the principles of REST very important for any Java developer aiming to build efficient and scalable web services.
Resources and Representations
In REST, a resource is any piece of information that can be identified by a URI (Uniform Resource Identifier). This could be a user, a product, or even a collection of items. Each resource can be represented in different formats, such as JSON or XML. The choice of representation should be made based on the client’s needs. For instance, a common choice in contemporary web services is JSON due to its lightweight nature and ease of use.
HTTP Methods
RESTful services utilize standard HTTP methods to perform operations on resources, aligning perfectly with the principles of the web. The most common methods include:
- Retrieve a representation of a resource.
- Create a new resource.
- Update an existing resource.
- Remove a resource.
Each HTTP method has a specific semantic meaning that helps clients understand the intent of their request. For example, using POST
to create a new user communicates a clear intention to the server, while GET
on the same endpoint would retrieve that user’s information.
Statelessness
One of the fundamental principles of REST is statelessness. This means that each request from the client to the server must contain all the information needed to understand and process the request. The server does not store any client context between requests. This simplifies the server design and enhances scalability, as each request can be handled independently.
Client-Server Architecture
REST encourages a separation of concerns by implementing a client-server architecture. The client is responsible for the user interface and the server for data storage and processing. This separation allows for independent evolution of both client and server applications, enabling updates and modifications without affecting the other party.
Layered System
A RESTful architecture can be composed of multiple layers, each serving a specific purpose. For instance, you might have a load balancer, a web server, and the application server itself. Each layer can process requests and responses, enhancing scalability and manageability without the client needing to know about the underlying architecture.
Cacheability
To improve performance, RESTful web services should be designed with cacheability in mind. Responses must indicate whether they’re cacheable or not, allowing clients to store responses and reuse them for subsequent requests without hitting the server again. This can drastically reduce latency and server load.
By embracing these principles, Java developers can create RESTful web services that are efficient, scalable, and easy to maintain. As we move forward, it becomes essential to apply these concepts in practical implementations, using frameworks like Spring Boot to bring RESTful services to life.
Setting Up Your Java Development Environment
Setting up your Java development environment is an important first step in building RESTful web services. This setup will facilitate the creation, testing, and deployment of your applications. Below, we will outline the necessary tools and configurations needed to create a robust environment for Java development.
Java Development Kit (JDK)
The first requirement is to install the Java Development Kit (JDK). The JDK provides the essential tools for developing Java applications, including the Java compiler and runtime environment. You can download the latest version from the official Oracle website or use an open-source alternative like OpenJDK.
# To check if Java is already installed, run: java -version # If not installed, you can download and install JDK from: # https://www.oracle.com/java/technologies/javase-downloads.html
Integrated Development Environment (IDE)
An IDE can significantly enhance your productivity by providing features like code completion, debugging, and project management. Popular IDEs for Java development include IntelliJ IDEA, Eclipse, and NetBeans. Each of these IDEs has built-in support for Maven and Gradle, which are essential for managing your dependencies and building your projects.
# To install IntelliJ IDEA on Ubuntu, you can use: sudo snap install intellij-idea-community --classic # Or download the installer from: # https://www.jetbrains.com/idea/download/
Build Management Tools
Java projects often depend on various libraries and frameworks. To manage these dependencies, you’ll want to use a build management tool like Maven or Gradle. These tools help automate the process of compiling, packaging, and managing dependencies.
Here’s an example of a Maven `pom.xml` file for a simple Spring Boot application:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.example</groupId> <artifactId>restful-web-service</artifactId> <version>1.0-SNAPSHOT</version> <properties> <java.version>17</java.version> <spring.boot.version>2.5.4</spring.boot.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>com.h2database</groupId> <artifactId>h2</artifactId> <scope>runtime</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
Database Setup
Many RESTful applications require a persistent data layer. For this, you can use an in-memory database like H2 for development and testing purposes. H2 is lightweight and easy to set up, making it perfect for quick development cycles. You can also integrate it seamlessly with Spring Boot.
To configure H2 in your Spring Boot application, you can add the following properties to your `application.properties` file:
spring.datasource.url=jdbc:h2:mem:testdb spring.datasource.driverClassName=org.h2.Driver spring.datasource.username=sa spring.datasource.password=password spring.h2.console.enabled=true
Testing Framework
Lastly, for testing your RESTful web services, you will want to integrate a testing framework such as JUnit and Mockito. These frameworks allow you to write unit tests and integration tests, ensuring your application behaves as expected.
Here’s a basic example of a test case using JUnit 5:
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get; import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status; import org.junit.jupiter.api.Test; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTest; import org.springframework.test.web.servlet.MockMvc; @WebMvcTest public class MyControllerTest { @Autowired private MockMvc mockMvc; @Test public void testGetEndpoint() throws Exception { mockMvc.perform(get("/api/resource")) .andExpect(status().isOk()); } }
By establishing a solid development environment with these components, you’re well on your way to building effective RESTful web services in Java. The right tools can save you countless hours of development time, enabling you to focus on crafting quality code and robust services.
Creating a Simple RESTful Service with Spring Boot
Creating a simple RESTful service with Spring Boot is a simpler yet powerful way to engage with the principles of REST while using Java’s capabilities. Spring Boot provides an opinionated framework that simplifies the process of building production-ready applications. It eliminates much of the boilerplate code required by traditional Spring applications, allowing developers to focus on what matters most: implementing the business logic.
To get started, ensure you have a basic Spring Boot application set up using the Maven configuration detailed in the previous section. With that foundation in place, you can create your first RESTful service by following these essential steps.
Step 1: Define Your Model
Your RESTful service will typically interact with a model that represents the entities you’re dealing with. For instance, let’s create a simple model for a `Product`:
package com.example.model; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; @Entity public class Product { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String name; private double price; // Getters and Setters public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public double getPrice() { return price; } public void setPrice(double price) { this.price = price; } }
Step 2: Create a Repository Interface
Spring Data JPA simplifies data access by which will allow you to define repository interfaces. These interfaces provide methods for CRUD operations without the need for implementation:
package com.example.repository; import com.example.model.Product; import org.springframework.data.jpa.repository.JpaRepository; public interface ProductRepository extends JpaRepository { }
Step 3: Implement the REST Controller
Next, you need to expose your `Product` model via HTTP endpoints. That is where the controller comes into play. A REST controller handles incoming requests and returns appropriate responses:
package com.example.controller; import com.example.model.Product; import com.example.repository.ProductRepository; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.http.HttpStatus; import org.springframework.http.ResponseEntity; import org.springframework.web.bind.annotation.*; import java.util.List; @RestController @RequestMapping("/api/products") public class ProductController { @Autowired private ProductRepository productRepository; @GetMapping public List getAllProducts() { return productRepository.findAll(); } @PostMapping public ResponseEntity createProduct(@RequestBody Product product) { Product savedProduct = productRepository.save(product); return new ResponseEntity(savedProduct, HttpStatus.CREATED); } @GetMapping("/{id}") public ResponseEntity getProductById(@PathVariable Long id) { return productRepository.findById(id) .map(product -> new ResponseEntity(product, HttpStatus.OK)) .orElse(new ResponseEntity(HttpStatus.NOT_FOUND)); } @PutMapping("/{id}") public ResponseEntity updateProduct(@PathVariable Long id, @RequestBody Product product) { if (!productRepository.existsById(id)) { return new ResponseEntity(HttpStatus.NOT_FOUND); } product.setId(id); Product updatedProduct = productRepository.save(product); return new ResponseEntity(updatedProduct, HttpStatus.OK); } @DeleteMapping("/{id}") public ResponseEntity deleteProduct(@PathVariable Long id) { if (!productRepository.existsById(id)) { return new ResponseEntity(HttpStatus.NOT_FOUND); } productRepository.deleteById(id); return new ResponseEntity(HttpStatus.NO_CONTENT); } }
Step 4: Run Your Application
With your model, repository, and controller set up, you can run your Spring Boot application. The built-in server will start, and you can access your RESTful endpoints using tools like Postman or cURL. Here’s an example of how to create a new product using cURL:
curl -X POST http://localhost:8080/api/products -H "Content-Type: application/json" -d '{"name":"Sample Product", "price":19.99}'
This command sends a POST request to your service, creating a new product. You can retrieve the list of products by sending a GET request to the same base URL.
Step 5: Test Your Service
Finally, it’s crucial to test your RESTful service to ensure it works as expected. You can use the testing framework you set up earlier to write unit tests for your controller and verify that each endpoint behaves correctly.
package com.example.controller; import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.post; import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status; import com.example.model.Product; import com.example.repository.ProductRepository; import org.junit.jupiter.api.Test; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTest; import org.springframework.http.MediaType; import org.springframework.test.web.servlet.MockMvc; @WebMvcTest(ProductController.class) public class ProductControllerTest { @Autowired private MockMvc mockMvc; @Autowired private ProductRepository productRepository; @Test public void testCreateProduct() throws Exception { String jsonContent = "{"name":"Test Product", "price":29.99}"; mockMvc.perform(post("/api/products") .contentType(MediaType.APPLICATION_JSON) .content(jsonContent)) .andExpect(status().isCreated()); } }
In this way, you can create, read, update, and delete products through your RESTful service, following the principles of REST while using Spring Boot’s robust features. This simple yet effective example illustrates the power of combining Java with powerful frameworks, allowing for rapid development of scalable web services.
Handling Data and Persistence with JPA
Handling data and persistence in your RESTful web services is paramount for ensuring that the information your application processes is reliably stored and retrieved. Java Persistence API (JPA) provides a powerful and flexible way to manage relational data in Java applications. When working with Spring Boot, JPA integrates seamlessly, allowing developers to interact with databases through object-oriented paradigms while abstracting the complexities of JDBC.
In this section, we will delve into how to effectively use JPA to handle data persistence in your Spring Boot RESTful services.
Setting Up JPA with Spring Boot
To start working with JPA, ensure that you have included the necessary dependencies in your Maven `pom.xml` file. The Spring Boot starter for JPA simplifies the inclusion of JPA and Hibernate:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>com.h2database</groupId> <artifactId>h2</artifactId> <scope>runtime</scope> </dependency>
The above configuration also includes the H2 database as a runtime dependency, which is useful for development and testing.
Defining Entity Classes
JPA relies heavily on entity classes that define the structure of your data. Each class represents a table in the database, and each field corresponds to a column. Let’s think a `Customer` entity:
package com.example.model; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; @Entity public class Customer { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String name; private String email; // Getters and Setters public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } }
In this example, the `Customer` class is annotated with `@Entity`, marking it as a JPA entity. The `@Id` annotation specifies the primary key, and `@GeneratedValue` indicates that the value of the primary key will be automatically generated.
Creating a Repository Interface
Once you have your entity defined, the next step is to create a repository interface that extends `JpaRepository`. This interface provides essential CRUD operations without needing to implement them:
package com.example.repository; import com.example.model.Customer; import org.springframework.data.jpa.repository.JpaRepository; public interface CustomerRepository extends JpaRepository<Customer, Long> { }
By extending `JpaRepository`, you inherit methods for standard CRUD operations, which simplifies your code significantly.
Implementing the Service Layer
To separate business logic from the controller, it’s a good practice to implement a service layer. This layer can encapsulate the data access logic, allowing for cleaner controllers:
package com.example.service; import com.example.model.Customer; import com.example.repository.CustomerRepository; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import java.util.List; @Service public class CustomerService { @Autowired private CustomerRepository customerRepository; public List<Customer> getAllCustomers() { return customerRepository.findAll(); } public Customer createCustomer(Customer customer) { return customerRepository.save(customer); } public Customer getCustomerById(Long id) { return customerRepository.findById(id).orElse(null); } public void deleteCustomer(Long id) { customerRepository.deleteById(id); } }
In the `CustomerService` class, you define methods to interact with the repository. This keeps your controllers clean and focused on handling HTTP requests.
Creating the Controller
After setting up the service layer, you can now implement the controller that will handle incoming requests:
package com.example.controller; import com.example.model.Customer; import com.example.service.CustomerService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.http.HttpStatus; import org.springframework.http.ResponseEntity; import org.springframework.web.bind.annotation.*; import java.util.List; @RestController @RequestMapping("/api/customers") public class CustomerController { @Autowired private CustomerService customerService; @GetMapping public List<Customer> getAllCustomers() { return customerService.getAllCustomers(); } @PostMapping public ResponseEntity<Customer> createCustomer(@RequestBody Customer customer) { Customer createdCustomer = customerService.createCustomer(customer); return new ResponseEntity<>(createdCustomer, HttpStatus.CREATED); } @GetMapping("/{id}") public ResponseEntity<Customer> getCustomerById(@PathVariable Long id) { Customer customer = customerService.getCustomerById(id); return customer != null ? new ResponseEntity<>(customer, HttpStatus.OK) : new ResponseEntity<>(HttpStatus.NOT_FOUND); } @DeleteMapping("/{id}") public ResponseEntity<Void> deleteCustomer(@PathVariable Long id) { customerService.deleteCustomer(id); return new ResponseEntity<>(HttpStatus.NO_CONTENT); } }
This controller allows clients to interact with the customer data through various HTTP methods, adhering to RESTful practices.
Testing Your JPA Integration
To ensure that your persistence layer functions correctly, it is essential to implement tests. Using Spring Boot’s testing support, you can create integration tests that validate the behavior of your JPA layer:
package com.example.service; import com.example.model.Customer; import com.example.repository.CustomerRepository; import org.junit.jupiter.api.Test; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.autoconfigure.orm.jpa.DataJpaTest; import static org.assertj.core.api.Assertions.assertThat; @DataJpaTest public class CustomerRepositoryTest { @Autowired private CustomerRepository customerRepository; @Test public void testCreateAndRetrieveCustomer() { Customer customer = new Customer(); customer.setName("Neil Hamilton"); customer.setEmail("[email protected]"); customerRepository.save(customer); Customer foundCustomer = customerRepository.findById(customer.getId()).orElse(null); assertThat(foundCustomer).isNotNull(); assertThat(foundCustomer.getName()).isEqualTo("Vatslav Kowalsky"); } }
Through the above tests, you can verify that your data persistence layer is functioning as expected, ensuring that your application can reliably store and retrieve data.
By effectively using JPA within your Spring Boot application, you can create robust RESTful services capable of handling various data persistence scenarios with ease. JPA abstracts away the complexities of database interactions, which will allow you to focus on building the core functionality of your services.
Securing Your RESTful Web Services
Securing your RESTful web services is a critical aspect of API development that ensures the integrity and confidentiality of the data being exchanged between clients and servers. With the rise of cyber threats, understanding how to implement effective security measures is essential for any Java developer. In this section, we will explore various strategies for securing RESTful web services, particularly using Spring Security, which integrates seamlessly with Spring Boot applications.
Authentication and Authorization
At the heart of securing an API are authentication and authorization. Authentication verifies the identity of the user, while authorization determines what an authenticated user is allowed to do. In RESTful services, these concepts can be implemented through token-based authentication, which is stateless and aligns well with REST principles.
Implementing Basic Authentication
Basic authentication is one of the simplest ways to secure a RESTful service. It involves sending user credentials (username and password) along with each HTTP request. Although simpler, basic authentication transmits credentials in an easily decodable format, so it should only be used over HTTPS to encrypt the data in transit.
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder; import org.springframework.security.crypto.password.PasswordEncoder; @Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .authorizeRequests() .antMatchers("/api/public/**").permitAll() // Allow public access .anyRequest().authenticated() // Secure all other endpoints .and() .httpBasic(); // Enable Basic Auth } @Bean public PasswordEncoder passwordEncoder() { return new BCryptPasswordEncoder(); // Use BCrypt to hash passwords } }
This configuration allows public access to endpoints under `/api/public/**` while securing the rest of the API using Basic Authentication. The `PasswordEncoder` bean is essential for securely hashing passwords before storing them in a database.
Token-Based Authentication with JWT
For a more robust and stateless approach, JSON Web Tokens (JWT) are widely used. JWTs consist of a header, payload, and signature, allowing claims to be securely transmitted between two parties. Here’s how to implement JWT authentication in a Spring Boot application.
import io.jsonwebtoken.Claims; import io.jsonwebtoken.Jwts; import io.jsonwebtoken.SignatureAlgorithm; import org.springframework.stereotype.Component; import java.util.Date; import java.util.HashMap; import java.util.Map; @Component public class JwtTokenUtil { private String secret = "your_secret_key"; // Use a strong secret public String generateToken(String username) { Map claims = new HashMap(); return createToken(claims, username); } private String createToken(Map claims, String subject) { return Jwts.builder() .setClaims(claims) .setSubject(subject) .setIssuedAt(new Date(System.currentTimeMillis())) .setExpiration(new Date(System.currentTimeMillis() + 1000 * 60 * 60 * 10)) // 10 hours expiration .signWith(SignatureAlgorithm.HS256, secret) .compact(); } public Boolean validateToken(String token, String username) { final String extractedUsername = extractUsername(token); return (extractedUsername.equals(username) && !isTokenExpired(token)); } private String extractUsername(String token) { return extractAllClaims(token).getSubject(); } private Claims extractAllClaims(String token) { return Jwts.parser().setSigningKey(secret).parseClaimsJws(token).getBody(); } private Boolean isTokenExpired(String token) { return extractAllClaims(token).getExpiration().before(new Date()); } }
This `JwtTokenUtil` class provides methods to generate and validate JWTs. When a user logs in, you authenticate their credentials and issue a JWT, which they can use in subsequent requests by including it in the Authorization header as a Bearer token.
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Value; import org.springframework.security.authentication.AuthenticationManager; import org.springframework.security.authentication.UsernamePasswordAuthenticationToken; import org.springframework.security.core.Authentication; import org.springframework.security.core.userdetails.UserDetailsService; import org.springframework.web.bind.annotation.*; @RestController @RequestMapping("/api/auth") public class AuthController { @Autowired private AuthenticationManager authenticationManager; @Autowired private JwtTokenUtil jwtTokenUtil; @Autowired private UserDetailsService userDetailsService; @PostMapping("/login") public String login(@RequestParam String username, @RequestParam String password) { Authentication authentication = authenticationManager.authenticate( new UsernamePasswordAuthenticationToken(username, password)); return jwtTokenUtil.generateToken(username); } }
In this controller, users can authenticate using their username and password. Upon successful authentication, they receive a JWT, which they can use for further requests to protected endpoints.
Securing Endpoints
With JWT in place, you can secure your RESTful endpoints effectively. You need to configure Spring Security to filter requests and validate JWTs for protected resources.
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Autowired private JwtRequestFilter jwtRequestFilter; @Override protected void configure(HttpSecurity http) throws Exception { http.csrf().disable() .authorizeRequests() .antMatchers("/api/auth/login").permitAll() // Allow login endpoint .anyRequest().authenticated() // Secure all other endpoints .and() .addFilterBefore(jwtRequestFilter, UsernamePasswordAuthenticationFilter.class); // Add JWT filter } }
Here, the `JwtRequestFilter` is a custom filter that intercepts requests and validates JWTs before they reach the controller. This separation of concerns enhances security and maintainability.
By implementing these security measures, you can protect your RESTful web services against unauthorized access and ensure that sensitive data remains secure. Whether through Basic Authentication or JWT, Spring Security provides robust mechanisms to secure your APIs without compromising the principles of REST. As a Java developer, mastering these techniques will elevate your ability to deliver secure and reliable applications.