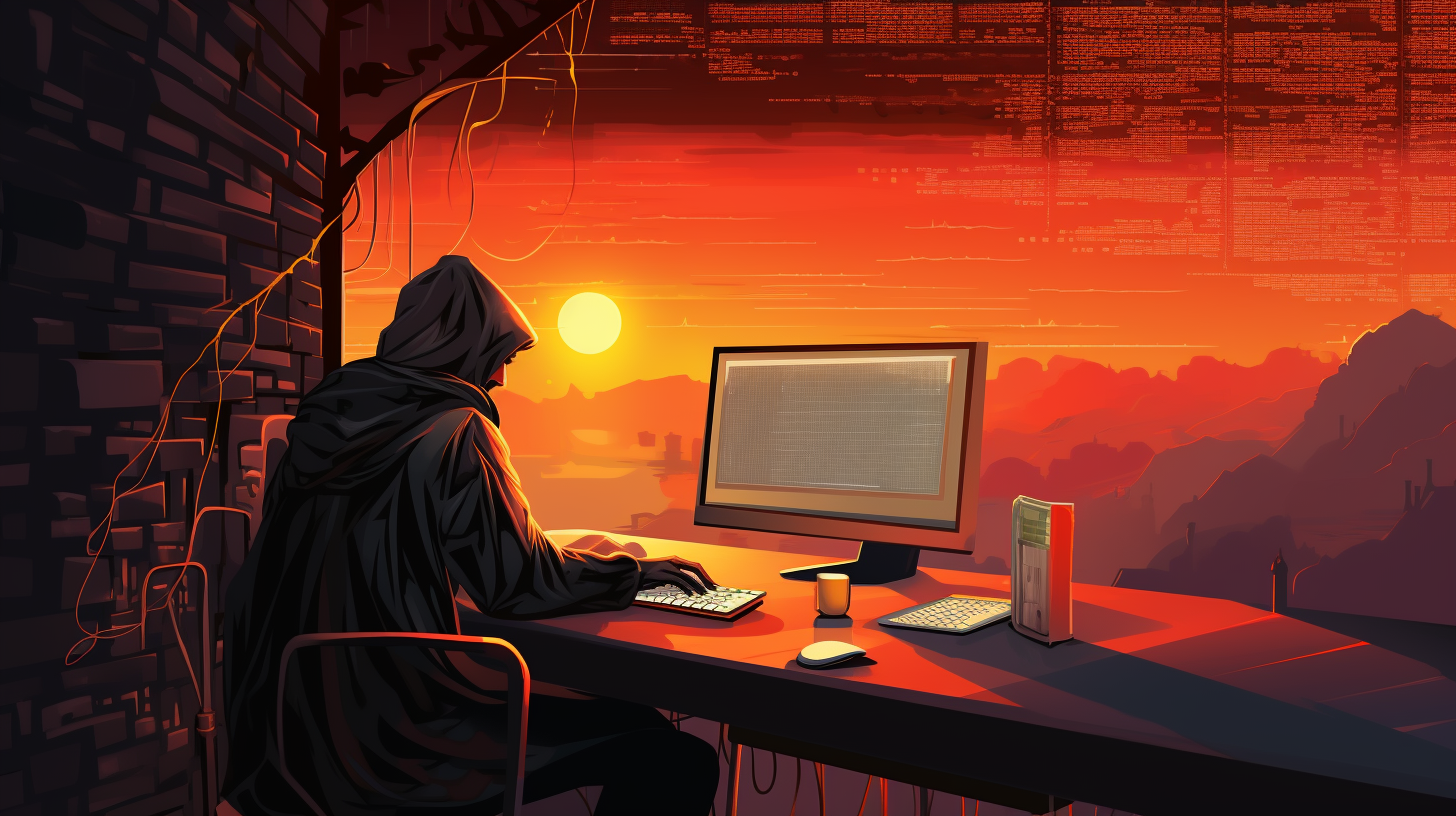
Command Substitution in Bash
Command substitution in Bash allows the output of a command to be used as an argument in another command. This powerful feature lets you dynamically generate input based on the results of prior commands, facilitating more efficient and versatile scripting. Understanding how command substitution works is fundamental for anyone looking to master Bash scripting.
In essence, command substitution captures the results of a command and substitutes that output in place of the command itself. This can be particularly useful in situations where you need to take the output of one command and feed it into another for further processing.
Historically, command substitution can be performed using two different syntaxes. The older form utilizes backticks (`
), while the more modern and preferred method uses the dollar sign and parentheses ($(...)
). The latter is generally favored for its readability and ability to nest commands without ambiguity.
Here’s a basic demonstration using both forms:
# Using backticks current_date=`date` echo "Today's date is: $current_date" # Using $() current_date=$(date) echo "Today's date is: $current_date"
In both examples, the output of the date
command is captured and stored in the current_date
variable, which is then echoed back to the user.
One of the key advantages of using the $(...)
syntax is its ability to nest commands more clearly. For example, if you wanted to find out the number of files in a directory and include that in a message, you could do it like this:
file_count=$(ls | wc -l) echo "There are $file_count files in the directory."
In this command, the output of ls
is piped into wc -l
to count the files, and the result is stored in file_count
for later use. The flexibility of command substitution is invaluable for creating dynamic and responsive scripts.
As you delve deeper into Bash scripting, mastering command substitution will enhance your ability to write efficient, effective scripts that can adapt to varying input and conditions, ultimately streamlining your workflows.
Syntax and Usage
To effectively utilize command substitution in your scripts, it’s important to understand the syntax rules associated with both forms. When using backticks, you must be cautious about nesting commands, as doing so can lead to confusion. For instance, think the following example:
outer_result=`command1 `command2``
Here, the inner command (command2) is enclosed in backticks, but the outer command (command1) also uses backticks. This can quickly become difficult to read and manage, especially with complex commands.
On the other hand, the modern syntax using $(…) offers clarity and ease. You can nest commands without escaping issues:
outer_result=$(command1 $(command2))
When it comes to usage, command substitution can be employed in various scenarios, such as variable assignment, command arguments, and even in conditional statements. For instance, you can use command substitution to assign the output of a command directly to a variable, rendering it effortless to manipulate later:
output=$(some_command) echo "The output is: $output"
In addition to variable assignments, command substitution can also be useful within command-line arguments. For example, if you want to create a backup directory with a timestamp, you could do something like this:
mkdir backup_$(date +%Y%m%d_%H%M%S)
This command will create a directory named with the current date and time, allowing for easy organization of backups without manual renaming.
For conditional checks, you might use command substitution to evaluate the output of a command before proceeding. This can streamline decision-making in your scripts:
if [[ $(grep -c 'error' logfile.txt) -gt 0 ]]; then echo "Errors found in logfile!" fi
In this case, the script counts occurrences of ‘error’ in logfile.txt and prints a message if any are found. The integration of command substitution here enhances the script’s ability to respond to real-time data.
Understanding the syntax and various usages of command substitution allows you to leverage its capabilities fully in your Bash scripts. By doing so, you’ll create scripts that are not only functional but also dynamic and adaptable to changes in their environment.
Types of Command Substitution
In Bash, there are primarily two types of command substitution based on the syntax you choose to use: the traditional backtick method and the more modern dollar-parentheses method. Each type offers a distinct approach to capturing command outputs, but they share the common goal of allowing the output of one command to be used as an input for another.
The backtick method, which harks back to older shells, utilizes backticks (`) to define the command whose output you want to capture. While functional, this method can lead to challenges, particularly when it comes to nesting commands. The readability of the code can quickly diminish, making it harder to debug or understand at a glance. For instance, ponder the following example:
result=`command1 `command2``
In this scenario, you can see how the inner command is enclosed within another pair of backticks, which might confuse anyone reading the script later.
On the other hand, the modern dollar-parentheses method, denoted by $(…), provides a clearer and more manageable way to capture command outputs. This syntax allows for easier nesting, as commands can simply be enclosed within another set of parentheses without additional escaping:
result=$(command1 $(command2))
This method not only improves readability but also helps avoid syntactical pitfalls associated with nesting commands using backticks. The dollar-parentheses syntax is therefore the preferred choice in contemporary Bash scripting.
In addition to the basic types of command substitution, it is crucial to recognize that the choice of syntax can also influence how commands are interpreted. For example, the use of backticks might inadvertently lead to issues if your command output contains special characters or spaces, as these can be misinterpreted or cause unexpected behavior. The dollar-parentheses syntax mitigates these issues by providing more robust parsing of the command output.
To illustrate, consider a case where you want to extract the current user and use it in a command. Using the backtick method might look like this:
current_user=`whoami` echo "User: $current_user"
While effective, if you needed to nest this command within another, it would quickly become unwieldy. Using the dollar-parentheses method streamlines this process:
current_user=$(whoami) echo "User: $current_user"
Both commands accomplish the same task, but the latter is simpler to read and manage, especially as complexity increases. In addition to these two syntaxes, command substitution can be used in various contexts such as variable assignment, arguments to other commands, and even within conditionals.
Ultimately, while both types of command substitution serve their purpose, the modern dollar-parentheses syntax is generally recommended for its enhanced readability, ease of nesting, and robustness in handling complex commands. As you continue to build your Bash scripting skills, embracing this modern syntax will not only improve your script clarity but also contribute to more efficient and maintainable code.
Quoting and Escaping
When working with command substitution in Bash, it is essential to understand how quoting and escaping interact with this feature. Quoting determines how Bash interprets the characters within a string, while escaping allows you to include characters that would otherwise be interpreted differently by the shell. This interplay can significantly affect the output of your commands, especially when dealing with special characters or whitespace.
There are three primary types of quoting in Bash: single quotes (‘), double quotes (“), and backslashes (). Each type serves a specific purpose and has different effects on command substitution.
Single quotes are the most restrictive; they preserve the literal value of each character within the quotes. This means that any variable expansion or command substitution within single quotes is ignored. Here’s an example:
output='$(date)' echo "The output is: $output"
In this case, the variable output will literally hold the string “$(date)” instead of the current date. Thus, the output will be:
The output is: $(date)
On the other hand, double quotes allow for command substitution and variable expansion. This means that anything inside double quotes this is prefixed with a dollar sign ($), as well as command substitutions, will be evaluated. For example:
output="$(date)" echo "The output is: $output"
Here, the output variable will contain the result of the date command, and the echo statement will provide the current date and time:
The output is: Thu Oct 12 14:23:01 UTC 2023
Escaping is useful when you need to include special characters without invoking their special meanings. You can escape characters using a backslash. For example, if you want to include a dollar sign in a string that also uses command substitution, you can write:
output="$(echo "The price is $100")" echo "$output"
In this case, the backslash before the dollar sign prevents it from being interpreted as the start of a variable name, allowing you to include the dollar sign literally in the output:
The price is $100
Combining quoting and escaping effectively allows you to control the output of command substitutions in complex scenarios. For instance, if you have a command that generates output with spaces or special characters, proper quoting can ensure that your script behaves as expected:
file_list="$(ls -1)" echo "Files in the directory: $file_list"
In this situation, if the directory contains files with spaces in their names, the command substitution captures the output correctly due to the use of double quotes. However, if you mistakenly used single quotes:
file_list='$(ls -1)' echo "Files in the directory: $file_list"
Then the output would not reflect the actual files present in the directory, as the command would not be executed.
Understanding how to apply quoting and escaping in conjunction with command substitution is important for writing robust Bash scripts. It ensures that your scripts can handle various types of input and output, ultimately leading to more predictable and maintainable code.
Common Use Cases
Command substitution in Bash opens up an array of possibilities for dynamic scripting, allowing you to integrate the output of commands into your scripts seamlessly. Here are some common use cases that showcase the versatility of command substitution.
One fundamental application of command substitution is in variable assignment. By capturing the output of a command, you can store it in a variable for later use. For instance, if you need to retrieve the current user’s username and use it in a greeting message, you can do the following:
username=$(whoami) echo "Hello, $username!"
This approach not only simplifies the script but also enhances its readability. Another common use of command substitution is generating file names or directory names dynamically. Suppose you want to create a backup directory that includes a timestamp in its name. You could use command substitution like this:
mkdir "backup_$(date +%Y%m%d_%H%M%S)"
This command generates a directory named with the current date and time, making it simple to manage backups without any manual renaming.
Command substitution is also invaluable in scripts that require conditional execution based on the output of other commands. For instance, if you wanted to check whether a specific service is running, you could write:
if [[ $(systemctl is-active my_service) == "active" ]]; then echo "Service is running." else echo "Service is not running." fi
In this example, the output of `systemctl is-active my_service` is captured, allowing the conditional statement to respond accurately based on the service’s status.
Another practical use case involves parsing command outputs and extracting specific information. For example, if you want to find the disk usage of your home directory, you could do:
disk_usage=$(du -sh ~ | cut -f1) echo "Your home directory uses $disk_usage of disk space."
This command not only captures the output of `du -sh` but also filters it to retrieve only the size, which is then displayed to the user.
Moreover, command substitution can be effectively coupled with other commands to streamline workflows. For example, if you want to find the most recently modified file in a directory, you could use:
latest_file=$(ls -t | head -n 1) echo "The most recently modified file is: $latest_file"
This snippet lists the files sorted by modification time and retrieves the first one, demonstrating how command substitution can facilitate efficient data handling in scripts.
Command substitution empowers Bash scripts by allowing for the integration of dynamic command output into various workflows. Whether it’s for variable assignment, generating dynamic names, executing conditionals, or parsing information, command substitution remains an essential tool for any Bash scripter looking to enhance their scripting capabilities.
Best Practices and Pitfalls
When employing command substitution in Bash, it very important to adhere to best practices and be aware of potential pitfalls that may arise. By doing so, you can write more efficient, maintainable, and error-free scripts.
One of the foremost best practices is to favor the use of the modern dollar-parentheses syntax ($(…)) over the older backtick method. While both methods perform command substitution, the dollar-parentheses syntax provides greater clarity and better handling of nested commands. That is particularly important when your scripts grow in complexity, as it helps maintain readability and reduces the chance of errors.
# Preferred method result=$(command1 $(command2)) # Less preferred (and harder to read) result=`command1 `command2`
Another essential practice involves proper quoting when using command substitution. Always use double quotes around your command substitution to prevent word splitting and globbing. This ensures that your commands handle filenames or output containing spaces or special characters correctly. For instance:
files=$(ls -1) echo "Files: $files"
If the filenames contain spaces, encapsulating the command substitution in double quotes keeps the integrity of each filename intact. Conversely, failing to quote the command substitution can lead to unexpected behavior, such as the command treating spaces as delimiters.
# Without quotes - potential pitfalls files=$(ls -1) echo "Files: $files" # This may output files incorrectly
Another common pitfall to be aware of is the performance impact of executing commands that generate extensive output. When using command substitution, the command’s entire output is captured in memory. If you’re working with commands that produce large volumes of data, ponder alternative approaches to avoid memory issues. For instance, you might want to process data in a loop rather than capturing it all at once:
while read -r file; do echo "Processing: $file" done < <(ls -1)
This technique, known as process substitution, allows you to handle output line by line without storing it all in a variable. It can enhance performance and reduce memory usage.
Additionally, be mindful of the exit status of commands used in command substitution. When a command’s output is captured, the exit status of that command is not automatically preserved. If you need to check whether the command succeeded, capture its exit status separately:
output=$(some_command) status=$? if [[ $status -ne 0 ]]; then echo "Command failed with status: $status" else echo "Command succeeded: $output" fi
By explicitly checking the command’s exit status, you can handle errors more gracefully and ensure your script behaves as expected.
Finally, always think readability and maintainability in your scripts. Use meaningful variable names to convey the purpose of the data stored in them, and document your code where necessary to explain complex command substitutions. This practice not only helps others understand your code but also assists you in future revisions.
By following these best practices and being aware of potential pitfalls, you can leverage command substitution effectively in your Bash scripts, leading to cleaner, more reliable, and more efficient code.