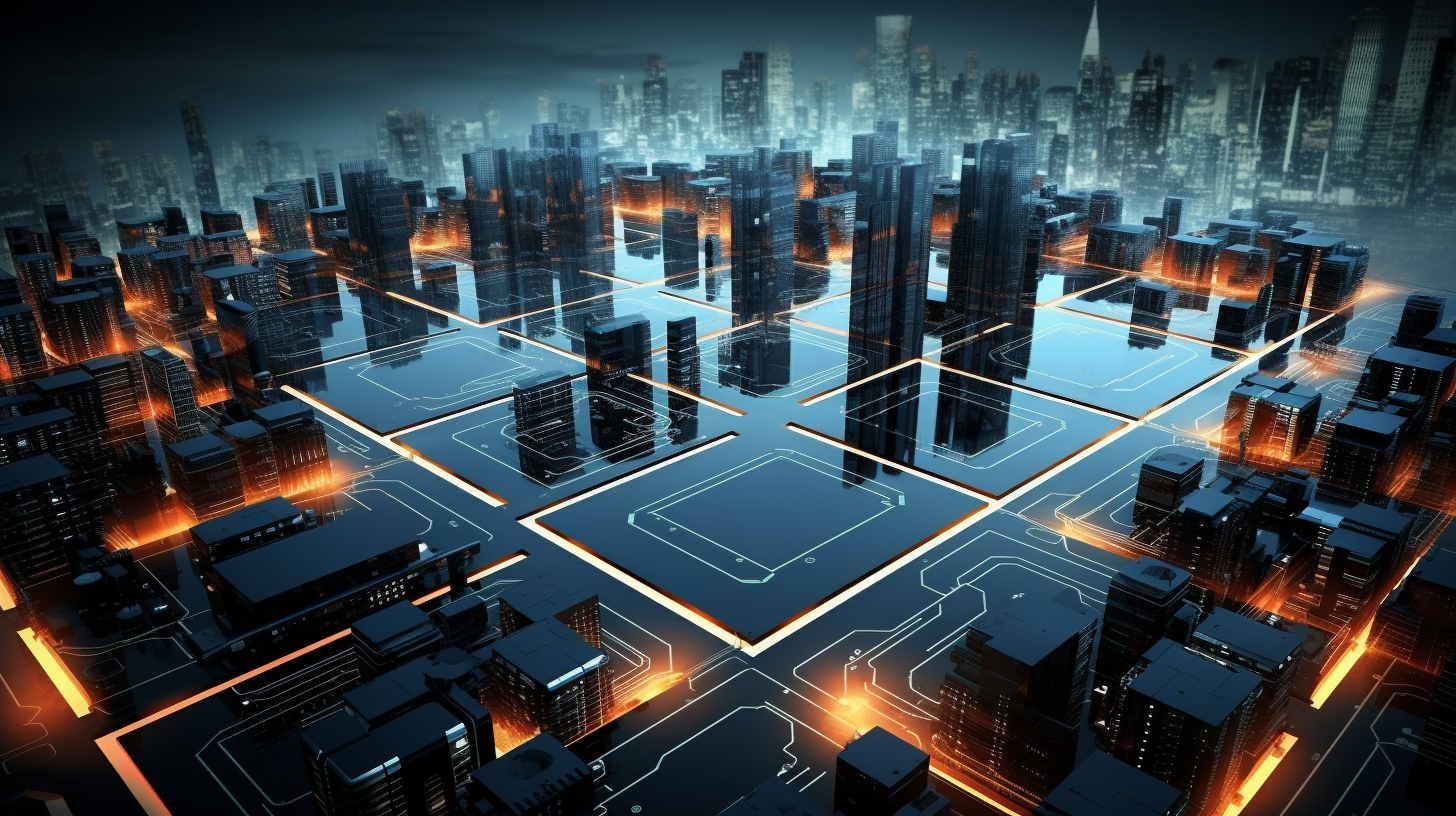
Control Flow in Swift
In Swift, control flow statements are essential for directing the execution of code based on certain conditions or repetitive tasks. These constructs enable developers to write programs that can make decisions, iterate over collections, and control the flow of execution effectively. Swift provides a rich set of control flow statements that are not only powerful but also concise, enhancing readability and maintainability of code.
At the heart of control flow in Swift are conditional statements, which allow the program to execute different blocks of code based on specified conditions. The primary conditional statements in Swift are if, else, and switch. Alongside these, looping constructs such as for, while, and repeat-while facilitate repeated execution of code blocks. Additionally, control transfer statements like break, continue, and return enable developers to manage the flow of execution more precisely.
Let’s explore these control flow statements in more detail with some examples:
To illustrate the if statement, think the following example that checks the value of a variable and prints a corresponding message:
let temperature = 30 if temperature > 25 { print("It's a hot day.") } else { print("It's a pleasant day.") }
In this snippet, if the temperature exceeds 25, it prints that it’s a hot day; otherwise, it indicates that the day is pleasant.
For situations where multiple conditions need to be evaluated, the switch statement comes into play. It offers a more elegant solution to handling multiple potential cases:
let score = 85 switch score { case 90...100: print("Grade: A") case 80..<90: print("Grade: B") case 70..<80: print("Grade: C") default: print("Grade: D") }
In this example, depending on the value of score, a different grade is printed. The switch statement here is not only cleaner but also more efficient than a series of if statements.
When it comes to looping, Swift provides several options. The for loop is commonly used to iterate over a range of numbers or elements in a collection:
for i in 1...5 { print("Iteration (i)") }
This loop iterates from 1 to 5 and prints the current iteration. Alternatively, while loops allow for iterative execution as long as a specified condition remains true:
var count = 0 while count < 5 { print("Count is (count)") count += 1 }
In this case, the loop continues until count reaches 5.
Finally, control transfer statements help in managing the flow. The break statement exits from loops or switch cases, whereas continue skips to the next iteration:
for number in 1...5 { if number == 3 { continue } print("Number is (number)") }
This loop will print numbers 1, 2, 4, and 5, effectively skipping the number 3.
Conditional Statements: if, else, and switch
In Swift, the if statement is a powerful construct that lets us execute a block of code based on a boolean condition. It can also be paired with else to provide an alternative path of execution. The syntax for an if-else statement is simpler, making it simple to read and understand. Here’s a more complex example that demonstrates how multiple conditions can be handled using if and else if:
let age = 20 if age < 13 { print("You are a child.") } else if age < 20 { print("You are a teenager.") } else if age < 65 { print("You are an adult.") } else { print("You are a senior.") }
In this example, we evaluate the age variable against various thresholds, resulting in different messages being printed depending on the value of age.
On the other hand, when we have a finite set of possible values to evaluate, the switch statement provides a cleaner, more efficient alternative. The switch statement matches the value of a variable against several possible cases. In contrast to if, where conditions can be complex boolean expressions, switch cases typically evaluate specific values or ranges:
let direction = "north" switch direction { case "north": print("You are heading north.") case "south": print("You are heading south.") case "east": print("You are heading east.") case "west": print("You are heading west.") default: print("Unknown direction.") }
Here, the variable direction is compared against several string cases, executing the corresponding print statement for the matched case. The default case acts as a catch-all for any values that do not match the specified cases.
Moreover, Swift’s switch statement can handle more complex patterns, including interval matching and tuples. Here’s an example that utilizes a range in a switch case:
let temperature = 15 switch temperature { case Int.min..<0: print("Freezing") case 0..<10: print("Very Cold") case 10..<20: print("Cool") case 20..<30: print("Warm") case 30...: print("Hot") default: print("Unknown temperature") }
Looping Constructs: for, while, and repeat-while
for i in 1...5 { print("Iteration (i)") }
In this example, the for loop iterates through a range of numbers from 1 to 5, printing the current iteration number on each pass. Swift’s for loop is particularly versatile, allowing iteration over ranges, arrays, or any sequence conforming to the Sequence
protocol. This means developers can easily traverse collections without worrying about indices, making the code cleaner and less error-prone.
let names = ["Alice", "Bob", "Charlie"] for name in names { print("Hello, (name)!") }
Here, we have a for loop that iterates over an array of names. For each element in the array, it prints a greeting. This illustrates not only the simplicity of using for loops in Swift but also their ability to work with collections directly.
Next, the while loop offers a mechanism for executing a block of code repeatedly as long as a specified condition remains true. This is useful when the number of iterations is not known upfront.
var countdown = 5 while countdown > 0 { print("Countdown: (countdown)") countdown -= 1 }
In this countdown example, the while loop continues to execute until the countdown
variable reaches zero, demonstrating how a condition governs the loop’s execution. However, developers should be cautious to ensure that the condition will eventually become false, or else they risk creating an infinite loop.
For scenarios where the block of code must be executed at least once before the condition is tested, the repeat-while loop is invaluable. This loop checks the condition after executing its code block.
var number = 0 repeat { print("Number: (number)") number += 1 } while number < 5
In this example, the repeat-while loop prints the value of number
and then increments it. The loop will continue until the number
reaches 5, but importantly, it guarantees that the body of the loop is executed at least once, regardless of the initial value of number
.
Handling Multiple Conditions: Switch Cases and Pattern Matching
Swift’s switch statement is a powerful construct that allows you to evaluate multiple conditions and execute corresponding blocks of code based on the matched case. Unlike many other programming languages, Swift’s switch statement is quite advanced, supporting a wide variety of types, including strings and tuples, as well as advanced pattern matching capabilities. This versatility makes switch an ideal choice when you have multiple discrete values to check against.
Let’s take a closer look at how the switch statement works with a simple example. Consider a scenario where we want to determine the status of a traffic light:
let trafficLight = "Green" switch trafficLight { case "Red": print("Stop") case "Yellow": print("Caution") case "Green": print("Go") default: print("Invalid traffic light color") }
In this example, we evaluate the value of trafficLight against several possible cases. If the light is red, we print “Stop”; if it’s yellow, we print “Caution”; and if it’s green, we print “Go”. If the value doesn’t match any of the specified cases, the default case is executed, handling unexpected values.
Moreover, Swift’s switch statement supports range matching, so that you can group multiple values seamlessly:
let score = 72 switch score { case 90...100: print("Grade: A") case 80..<90: print("Grade: B") case 70..<80: print("Grade: C") case 60..<70: print("Grade: D") default: print("Grade: F") }
Here, we categorize a score into a grade range. The ranges utilize the closed range operator (90…100) and the half-open range operator (80..<90), making the switch statement both expressive and concise.
Pattern matching is another powerful feature of Swift’s switch statement. This allows you to check against specific criteria or deconstruct complex data types. For instance, ponder the following example that uses tuples to evaluate coordinates:
let point = (2, 3) switch point { case (0, 0): print("Origin") case (_, 0): print("On the X-axis") case (0, _): print("On the Y-axis") case let (x, y) where x == y: print("On the line y = x") case let (x, y): print("Point at ((x), (y))") }
This example demonstrates how we can utilize pattern matching to identify the point’s location relative to the axes and the line y = x. The case (0, 0) checks for the origin, while the underscore (_) acts as a wildcard, allowing us to ignore specific values. The let pattern in the last two cases captures the coordinates for further use.
Control Transfer Statements: break, continue, and return
In Swift, control transfer statements provide developers with mechanisms to influence the execution flow within loops and functions. The three primary control transfer statements are break, continue, and return. Each serves a unique purpose that enhances the management of code execution in various scenarios.
The break statement is used to exit a loop or switch statement immediately. When a break is encountered, control transfers to the statement immediately following the loop or switch. This is particularly useful when a certain condition is met, and you want to terminate the current flow without executing any further iterations or cases.
for number in 1...10 { if number == 5 { break } print(number) }
In this example, the loop iterates through numbers from 1 to 10. However, when the number reaches 5, the break statement is executed, terminating the loop. As a result, the output will only display the numbers 1 through 4.
On the other hand, the continue statement allows for skipping the current iteration of a loop and proceeding to the next one. That is helpful when you want to ignore certain cases without entirely exiting the loop.
for number in 1...10 { if number % 2 == 0 { continue } print(number) }
In this code snippet, the loop checks each number from 1 to 10. When it encounters an even number, the continue statement is executed, resulting in the loop skipping the print statement for even numbers. Therefore, the output will only display the odd numbers: 1, 3, 5, 7, and 9.
Lastly, the return statement is critical within functions, as it exits the function and optionally returns a value to the caller. When the return statement is executed, control transfers back to the point where the function was called, making it a fundamental aspect of function design.
func calculateSquare(of number: Int) -> Int { return number * number } let result = calculateSquare(of: 4) print(result)
In this example, the function calculateSquare takes an integer as a parameter, computes its square, and returns the result. The return statement specifies the value being sent back to the caller, which is captured in the variable result and printed to the console. The output will be 16.