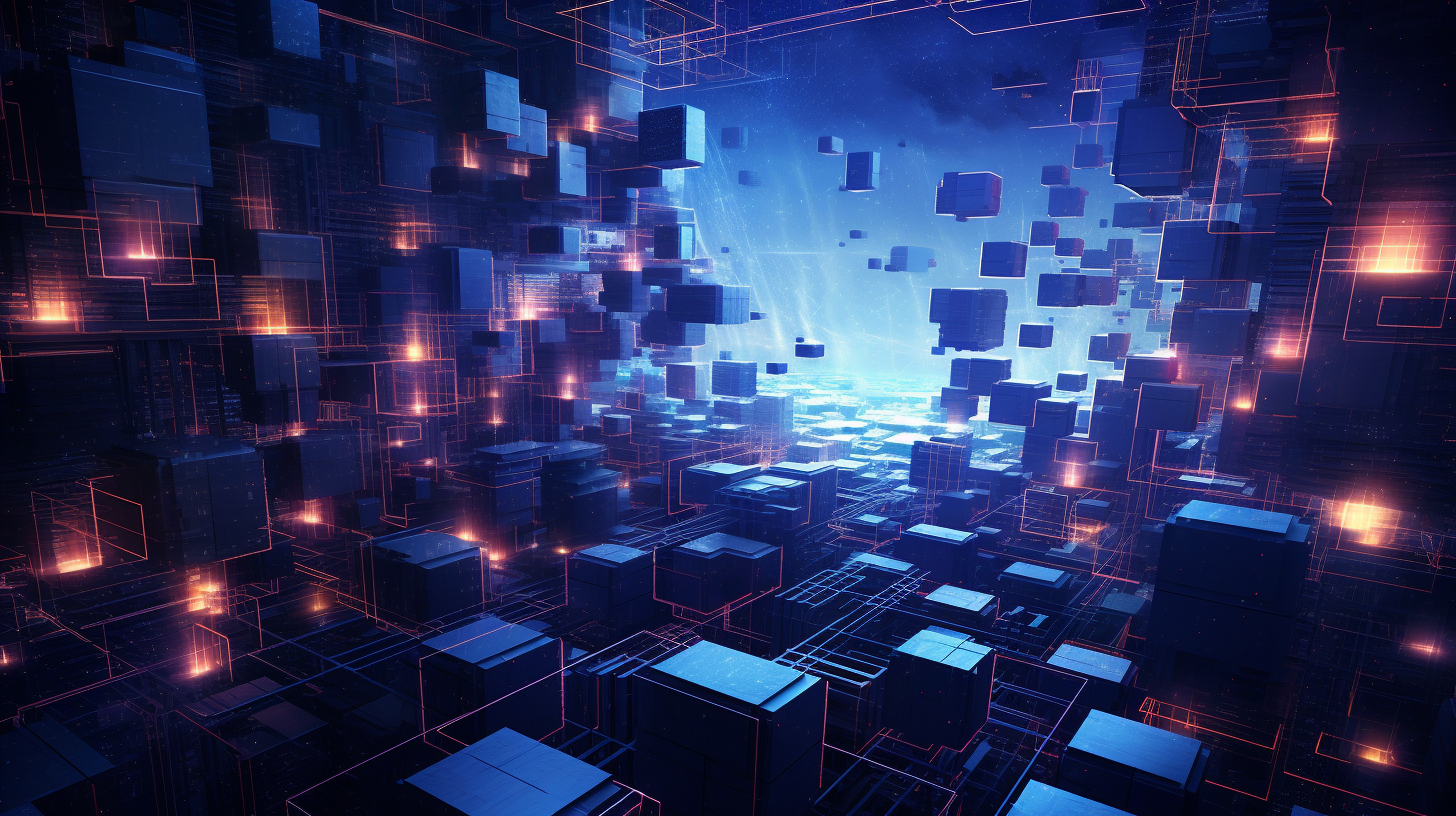
Control Structures in Java: If, Else, Switch
The if statement in Java serves as a fundamental control structure that allows developers to execute specific code blocks conditionally, based on whether a defined expression evaluates to true or false. This mechanism is critical for introducing logic that can dynamically alter the flow of a program.
At its core, the syntax for an if statement is straightforward:
if (condition) { // code to be executed if the condition is true }
In this construct, the condition
is a boolean expression, which can involve comparison operators, logical operators, or even method calls that return a boolean value. When the condition
evaluates to true, the code block within the curly braces is executed. If false, that block is skipped entirely.
Ponder the following example that demonstrates a simple use of the if statement:
int number = 10; if (number > 5) { System.out.println("The number is greater than 5."); }
In this code snippet, the condition number > 5
evaluates to true, so the message is printed to the console. If we were to change the value of number
to 3, the message would not be printed, illustrating the conditional nature of the if statement.
In practice, the if statement can be extended with additional clauses like else or else if to create more complex branching logic. This allows developers to handle various scenarios by evaluating multiple conditions in sequence.
Here’s an example using the else clause:
int number = 3; if (number > 5) { System.out.println("The number is greater than 5."); } else { System.out.println("The number is 5 or less."); }
In this case, since the condition is false, the else block executes instead, providing a clear path for handling alternate scenarios. The beauty of the if statement lies in its simplicity and versatility, making it an indispensable tool in every Java developer’s toolkit.
The Else Clause: Expanding Conditional Logic
The else clause serves as a powerful extension to the if statement, allowing developers to define a clear and logical path for handling scenarios where the primary condition evaluates to false. It acts as a catch-all, ensuring that a specific block of code executes when the initial condition is not met. This capability especially important in branching logic, as it provides a defined outcome for each pathway of execution.
Like the if statement, the else clause simplifies control flow, but it’s specific to the scenario where the preceding if condition fails. The syntax is elegantly simple:
if (condition) { // code to be executed if the condition is true } else { // code to be executed if the condition is false }
To illustrate how the else clause works in practice, consider the following example that evaluates a student’s grade:
int grade = 75; if (grade >= 60) { System.out.println("The student has passed."); } else { System.out.println("The student has failed."); }
In this example, when the variable grade is set to 75, the condition grade >= 60 evaluates to true, so the output will be “The student has passed.” If we set grade to 50 instead, the else block executes, leading to the output “The student has failed.” This demonstrates how the else clause effectively accommodates alternate conditions, enriching the decision-making process within the program.
The flexibility of the else clause becomes even more apparent when combined with the if statement to form intricate decision trees. By nesting additional if statements within an else block, developers can construct multi-layered logical evaluations. Here’s an example that categorizes a score:
int score = 85; if (score >= 90) { System.out.println("Grade: A"); } else if (score >= 80) { System.out.println("Grade: B"); } else if (score >= 70) { System.out.println("Grade: C"); } else { System.out.println("Grade: D or F"); }
Here, the program checks the score against multiple conditions, with each else if providing a new pathway based on the score’s value. If none of the conditions are met, the final else clause catches any remaining values, delivering a fallback response. This structure not only organizes the logic neatly but also enhances readability and maintainability of the code.
The else clause expands the functionality of the if statement, introducing a way to handle alternative outcomes when conditions are not satisfied. It promotes clarity in control structures, allowing developers to define explicit paths of execution for various scenarios. The effective use of else, along with if and else if, empowers Java programmers to craft robust and logical decision-making processes in their applications.
Using the Else If for Multiple Conditions
When dealing with multiple conditions in Java, the else if clause becomes an essential tool that allows developers to evaluate additional expressions without deeply nesting if statements. This construct enhances both the readability and manageability of code by allowing a clear linear flow of conditional checks.
The syntax for using else if is simpler and follows this pattern:
if (condition1) { // code to execute if condition1 is true } else if (condition2) { // code to execute if condition2 is true } else { // code to execute if neither condition1 nor condition2 is true }
The else if clause acts as a bridge between the initial if statement and the final else, enabling the evaluation of additional conditions in a sequential manner. This eliminates the need for deeply nested if statements, which can be cumbersome and hard to read.
Ponder the following example where we want to assign a letter grade based on a student’s score:
int score = 88; if (score >= 90) { System.out.println("Grade: A"); } else if (score >= 80) { System.out.println("Grade: B"); } else if (score >= 70) { System.out.println("Grade: C"); } else if (score >= 60) { System.out.println("Grade: D"); } else { System.out.println("Grade: F"); }
In this example, the program evaluates the score sequentially. If the score is 88, the condition score >= 90 is false, so it moves to the next condition, score >= 80, which is true. Hence, it prints “Grade: B.” This structured approach allows for easy addition of new conditions; for instance, if we wanted to introduce a grade of “C+” for scores between 75 and 79, the code could be extended without significantly disrupting the existing logic.
Using else if clauses not only streamlines decision-making but also enhances performance by reducing unnecessary evaluations. When a condition evaluates to true, subsequent conditions are not checked, making the control structure more efficient. This is particularly important in scenarios where the checks involve resource-intensive calculations or operations.
Another practical application of the else if structure can be seen in an application that determines the type of weather based on temperature:
int temperature = 32; if (temperature > 30) { System.out.println("It's hot outside."); } else if (temperature > 20) { System.out.println("It's warm outside."); } else if (temperature > 10) { System.out.println("It's cool outside."); } else { System.out.println("It's cold outside."); }
In this case, the program evaluates the temperature and responds accordingly. If the temperature is set to 32, it prints “It’s hot outside.” This simpler approach allows for ease of understanding and quick adjustments, should the thresholds need modification in the future.
The else if construct is an elegant solution for managing multiple conditions in a sequential manner. It maintains clarity in the control structure and promotes efficient execution, making it an invaluable asset for Java developers striving for clean and maintainable code.
The Switch Statement: Simplifying Complex Conditions
The switch statement in Java is a powerful control structure that provides a means to simplify complex conditional logic, particularly when dealing with a variable that can take on multiple distinct values. Unlike the if-else constructs, which can become cumbersome when evaluating numerous conditions, the switch statement offers a more readable and structured approach for handling multiple possible execution paths.
The syntax of a switch statement is both clear and concise:
switch (expression) { case value1: // code to execute if expression equals value1 break; case value2: // code to execute if expression equals value2 break; // more cases as needed default: // code to execute if no case matches }
In this construct, the expression is evaluated once, and its result is compared against the values specified in each case. When a match is found, the corresponding code block is executed until a break statement is encountered, which exits the switch statement. If none of the cases match, the default block, if defined, will execute.
To illustrate the use of the switch statement, consider the following example, which evaluates a variable to determine the day of the week:
int day = 3; switch (day) { case 1: System.out.println("Monday"); break; case 2: System.out.println("Tuesday"); break; case 3: System.out.println("Wednesday"); break; case 4: System.out.println("Thursday"); break; case 5: System.out.println("Friday"); break; case 6: System.out.println("Saturday"); break; case 7: System.out.println("Sunday"); break; default: System.out.println("Invalid day"); }
In this example, the variable day is set to 3, which matches the case for Wednesday. The program outputs “Wednesday” to the console. If we were to set day to 8, the default case would execute, indicating an invalid day.
The switch statement shines when there are numerous possible values to evaluate, especially when they’re discrete, such as enums, characters, or integers. It enhances readability by avoiding long chains of if-else statements, which can quickly become difficult to follow. Additionally, the grouping of cases allows for the execution of the same block of code for multiple values without duplicating code, as illustrated below:
char grade = 'B'; switch (grade) { case 'A': case 'B': case 'C': System.out.println("You passed!"); break; case 'D': case 'F': System.out.println("You failed!"); break; default: System.out.println("Invalid grade"); }
Here, the switch statement checks for grades ‘A’, ‘B’, or ‘C’ and executes the same block of code if any of those conditions are true. This approach not only reduces redundancy but also improves the maintainability of the code.
It is also worth noting that the switch statement in Java can handle strings (since Java 7), which allows for even broader applications. Ponder the following example where we categorize a fruit based on its name:
String fruit = "Apple"; switch (fruit) { case "Apple": System.out.println("You have an apple."); break; case "Banana": System.out.println("You have a banana."); break; case "Cherry": System.out.println("You have a cherry."); break; default: System.out.println("Unknown fruit."); }
This capability of the switch statement to work with strings further enhances its utility, allowing developers to write cleaner, more expressive code for scenarios where multiple potential matches must be handled.
Overall, the switch statement is a robust alternative to if-else chains for specific use cases, particularly when the number of potential values is fixed and known in advance. Its clear structure, combined with the ability to handle multiple cases and types, makes it an essential tool in the Java programmer’s toolkit for managing complex decision-making scenarios.
Best Practices for Control Structures in Java
When it comes to control structures in Java, adhering to best practices can significantly enhance the readability, maintainability, and performance of your code. Control structures like if, else, else if, and switch statements are powerful tools that, when used correctly, can lead to a clear logical flow in your programs. However, misusing them can result in convoluted code that’s hard to understand and debug. Here are some best practices to keep in mind when working with control structures in Java.
1. Keep Conditions Simple
Avoid complex conditions that can confuse readers of your code. Instead, break them down into smaller, more manageable pieces. When conditions are clear and simpler, it enhances comprehension and reduces the likelihood of mistakes. For instance:
int age = 25; if (age >= 18 && age < 65) { System.out.println("You are an adult."); } else { System.out.println("You are either a minor or a senior."); }
In this snippet, the condition is concise and easy to interpret. If the logic were more complex, consider using helper methods to encapsulate that logic, thus keeping the if statement tidy.
2. Use Braces Consistently
Even when control structures contain a single line of code, always use braces ({}) to define the block of code. This practice prevents errors during maintenance, particularly when additional lines of code are added later. For example:
if (score >= 90) { System.out.println("Grade: A"); } else { System.out.println("Grade: B"); }
Using braces consistently clarifies the intended structure and reduces the risk of introducing bugs.
3. Limit Nesting of Control Structures
Nesting too many control structures can lead to code this is difficult to follow. If you find yourself nesting if statements beyond two levels, it might be a sign that your code could benefit from refactoring. Ponder using early exit strategies (like return statements) or breaking down the logic into separate methods. For example:
if (user.isLoggedIn()) { if (user.hasPermission()) { System.out.println("Access granted."); } else { System.out.println("Access denied."); } } else { System.out.println("Please log in."); }
This can be refactored for clarity:
if (!user.isLoggedIn()) { System.out.println("Please log in."); return; } if (!user.hasPermission()) { System.out.println("Access denied."); return; } System.out.println("Access granted.");
This approach clarifies each condition’s intent and reduces indentation depth, making the code easier to read and maintain.
4. Prefer Switch for Multiple Conditions
When evaluating a variable against multiple distinct values, prefer using a switch statement over multiple if-else statements. This not only improves readability but also aids in reducing the risk of fall-through errors when cases are not properly terminated with break statements.
String day = "Monday"; switch (day) { case "Monday": System.out.println("Start of the work week."); break; case "Friday": System.out.println("End of the work week."); break; case "Saturday": case "Sunday": System.out.println("Weekend!"); break; default: System.out.println("Midweek day."); }
By consolidating multiple cases, the switch statement becomes clearer, and the intent of the code is more evident.
5. Optimize for Performance
While clarity is critical, performance should also be considered, especially in scenarios involving large data sets or frequent evaluations. For instance, when using multiple if-else conditions that could benefit from early returns or breaks, evaluate the most likely conditions first to minimize unnecessary checks.
if (condition1) { // handle condition1 } else if (condition2) { // handle condition2 } else if (condition3) { // handle condition3 } // and so on
Place the most probable condition at the top of the list, as it will exit the structure quicker, thereby optimizing the execution time.
By following these best practices, Java developers can create control structures that are not only effective but also scalable and maintainable. Clear, concise, and well-structured code is a hallmark of professional programming, elevating the quality of the software and easing future modifications.
It’s worth noting that while the article covers the foundational aspects of control structures in Java, it misses the significance of using contemporary features such as lambda expressions and Streams, especially for simplifying complex conditional processing. These constructs allow for a functional programming style that can drastically reduce boilerplate code, enhance readability, and improve performance by using parallel operations. Including examples using these features could provide a more comprehensive understanding of how to effectively manage conditional logic in Java.