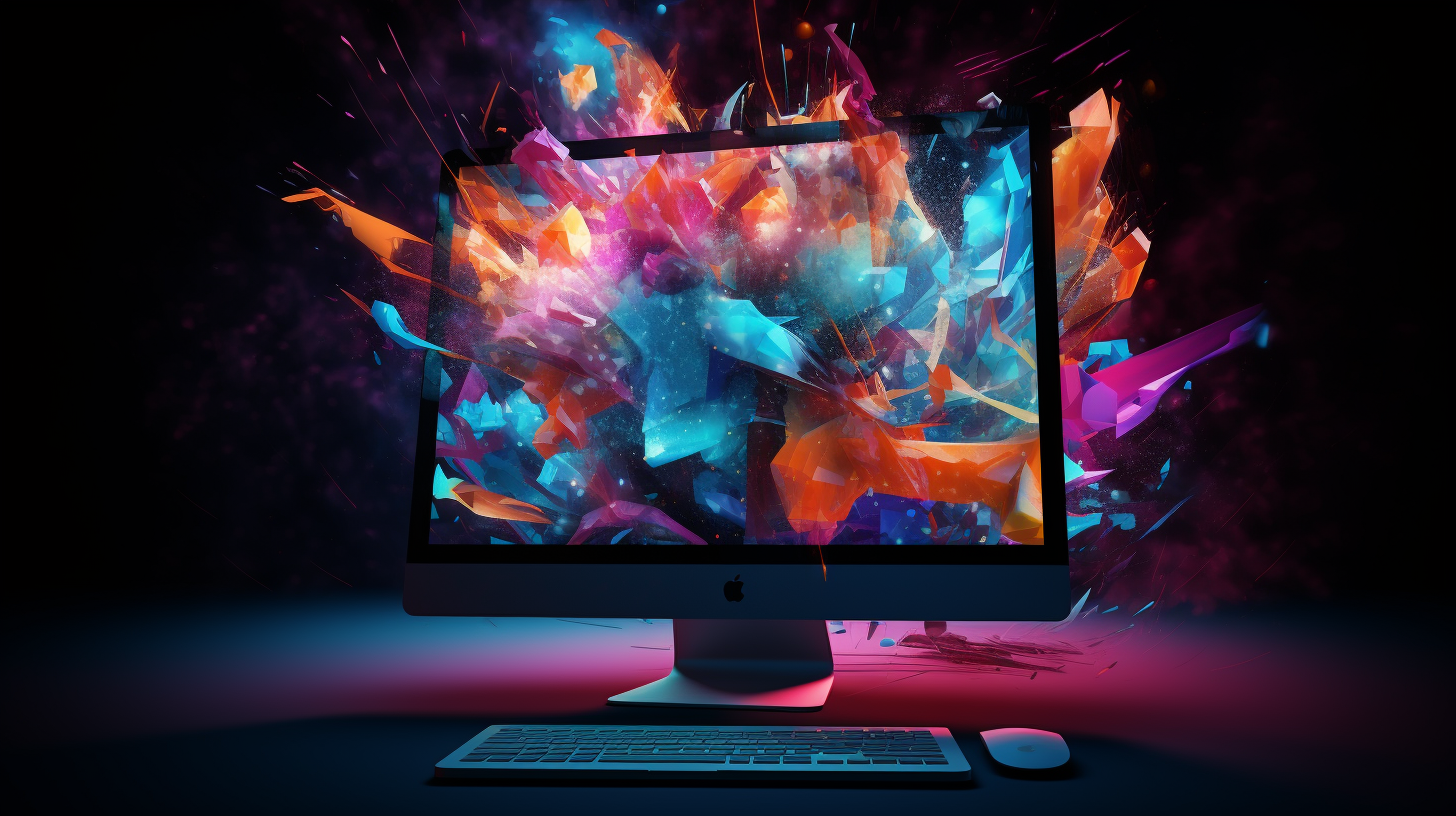
Creating and Managing Cron Jobs in Bash
Cron jobs are a powerful feature in Unix-like operating systems that allow users to schedule tasks to run automatically at specified intervals. This functionality is important for automating repetitive tasks, thereby saving time and reducing the risk of human error. Understanding how cron jobs work and their use cases can significantly enhance your productivity and system management.
At its core, cron is a time-based job scheduler. It executes commands or scripts at predetermined times or intervals based on the configuration specified in a crontab (cron table) file. Each line in this file represents a scheduled task and includes detailed timing information alongside the command to be executed.
Cron jobs can be incredibly versatile, allowing for a wide range of applications, including:
- Regularly running system updates, cleaning temporary files, or backing up data can be scheduled with cron jobs.
- Automate the execution of scripts that process data, such as importing or exporting databases, generating reports, or aggregating logs.
- Regularly check the health of services or applications, notifying administrators if a problem is detected.
- Send automated emails for reminders, alerts, or status updates, ensuring timely communication without manual intervention.
Each cron job is defined by a specific syntax that dictates when and how often a task is executed. The timing format consists of five fields representing minutes, hours, days of the month, months, and days of the week. Here’s a brief overview of the syntax:
# ┌───────────── minute (0 - 59) # │ ┌───────────── hour (0 - 23) # │ │ ┌───────────── day of the month (1 - 31) # │ │ │ ┌───────────── month (1 - 12) # │ │ │ │ ┌───────────── day of the week (0 - 6) (Sunday=0) # │ │ │ │ │ # * * * * * command to be executed
For instance, if you wanted to schedule a script located at /home/user/backup.sh
to run daily at 2 AM, you would add the following line to your crontab:
0 2 * * * /home/user/backup.sh
Setting Up Your First Cron Job
Setting up your first cron job is a simpler task, but it’s essential to follow the correct steps to ensure that your scheduled jobs run smoothly and effectively. The first step is to access your crontab file, which is where you will define your cron jobs. You can do this by using the `crontab` command with the `-e` option, which opens the crontab file in your default text editor.
crontab -e
Upon executing this command, you will be taken to an editor window where you can add your cron jobs. If you’re using this command for the first time, you may be prompted to select an editor. Common choices include `nano`, `vim`, or `emacs`—select the one you’re most comfortable with.
Let’s say you want to create a cron job for a script that backs up your home directory every day at 3 AM. To do this, you would add the following line to your crontab:
0 3 * * * /home/user/backup_script.sh
This line breaks down as follows:
- 0 – The exact minute when the job runs (0 minutes past the hour).
- 3 – The hour when the job runs (3 AM).
- * – Every day of the month.
- * – Every month.
- * – Every day of the week.
After adding your cron job, save the changes and close the editor. Your cron job is now scheduled! To confirm that your job has been added successfully, you can list all current cron jobs with the command:
crontab -l
This command will display the contents of your crontab file, so that you can verify that your new job is present. If you need to make changes later, simply run the `crontab -e` command again, modify the line as required, and save the changes.
One crucial aspect to remember is that the environment in which your cron jobs run is not the same as your user shell. Therefore, if your script depends on certain environment variables or paths, you may want to specify those explicitly within your script or at the beginning of your crontab. For example:
0 3 * * * PATH=/usr/local/bin:/usr/bin:/bin /home/user/backup_script.sh
Editing and Managing Existing Cron Jobs
Editing and managing existing cron jobs is a vital skill for anyone looking to maintain efficient task scheduling. Once you’ve set up your cron jobs, you will inevitably find the need to modify them—whether it’s adjusting the timing, changing the script being executed, or deleting an outdated job.
To edit your existing cron jobs, you again utilize the `crontab -e` command. This opens your crontab file in your chosen text editor, displaying all currently scheduled jobs. You can then navigate through the list, make the necessary changes, and save the file. Here’s how you might modify a job:
# Original cron job 0 3 * * * /home/user/backup_script.sh # Updated to run at 4 AM instead 0 4 * * * /home/user/backup_script.sh
When editing, it’s important to remember that the structure of the cron job especially important. Ensure that you maintain the correct format and syntax to prevent any unexpected behavior. After saving your changes, you can verify them by listing the current cron jobs with:
crontab -l
Sometimes, you may want to disable a cron job temporarily rather than delete it entirely. This can be accomplished simply by commenting out the line by adding a `#` at the beginning:
# 0 4 * * * /home/user/backup_script.sh
To reactivate the job, just remove the `#` and save the changes once again. This practice allows you to quickly enable or disable jobs without losing their configuration.
In addition to editing, managing your cron jobs effectively includes periodic reviews. Regularly check your scheduled tasks to ensure they are still relevant and functioning as expected. Old jobs may accumulate over time, leading to clutter and potential confusion. If you find a job that no longer serves a purpose, it can be removed easily:
# Removing an outdated job # 0 4 * * * /home/user/backup_script.sh
After making your edits, always remember to check the job’s functionality. A common pitfall is syntax errors or misconfigured paths that prevent a cron job from executing properly. A good practice is to redirect output and errors to a log file for easier troubleshooting:
0 4 * * * /home/user/backup_script.sh >> /var/log/backup.log 2>&1
This command appends both standard output and error output to `backup.log`, which can be invaluable for diagnosing issues.
Troubleshooting Common Cron Job Issues
Troubleshooting cron job issues can often feel like unraveling a complex puzzle, where the solution lies hidden amid layers of configuration, environment variables, and system permissions. The first step in diagnosing a problem is understanding potential failure points. There are several common issues that can prevent cron jobs from executing as intended, and knowing how to identify and rectify these can save you valuable time and frustration.
One of the most frequent culprits is the environment in which cron jobs run. Unlike your user shell, cron jobs operate in a more restricted environment. This means that certain environment variables that are available in your interactive shell may not be present when the cron job executes. For instance, if your script relies on a specific version of a program located in your PATH, it may fail because the PATH variable is not set as you expect. To ensure that your cron job has the necessary environment, you can explicitly define the required variables at the top of your crontab:
# Set PATH for cron jobs PATH=/usr/local/bin:/usr/bin:/bin # Example cron job 0 3 * * * /home/user/backup_script.sh
Another common issue is related to file permissions. If your script or the files it interacts with do not have the appropriate permissions set, the cron job will fail to execute. Always check the permissions of the script and any other resources it accesses. You can check and set permissions using the `chmod` command:
# Check permissions ls -l /home/user/backup_script.sh # Change permissions to make it executable chmod +x /home/user/backup_script.sh
Additionally, it is paramount to redirect output and errors to a log file. This practice can illuminate the reasons behind a job’s failure. By appending both standard output and error output to a log file, you can gain insights into what went wrong:
# Redirecting output and errors 0 3 * * * /home/user/backup_script.sh >> /var/log/backup.log 2>&1
After your cron job has run, you can inspect `backup.log` for any error messages or hints regarding the failure. This logging mechanism is an important tool in your troubleshooting arsenal.
Sometimes, the issue might arise from the job’s timing configuration itself. Ensure that your cron timing syntax is correct. A simple oversight, such as using `*` instead of `0` for the minute or hour fields, can result in unexpected behavior. To validate your timing, you can utilize online cron expression validators or simply double-check the syntax against the standard format:
# Correct syntax example # ┌───────────── minute (0 - 59) # │ ┌───────────── hour (0 - 23) # │ │ ┌───────────── day of the month (1 - 31) # │ │ │ ┌───────────── month (1 - 12) # │ │ │ │ ┌───────────── day of the week (0 - 6) (Sunday=0) # │ │ │ │ │ # 0 3 * * * /home/user/backup_script.sh
Another often-overlooked aspect is whether the cron daemon is running. On some systems, the cron service may not be active, preventing any jobs from executing. You can check the status of the cron service and start it if necessary. The commands may vary by distribution, so refer to your system’s documentation:
# Check status of cron service systemctl status cron # Start the cron service if it is not running sudo systemctl start cron
Finally, keep in mind that cron jobs may not run if the system is in a state of low resource availability, such as when the system is in sleep mode or under heavy load. Monitoring system performance and ensuring that the necessary resources are available for scheduled tasks is important for maintaining reliable automation.
Best Practices for Cron Job Management
When it comes to managing cron jobs effectively, adopting best practices can significantly enhance your automation strategy and ensure that your scheduled tasks run smoothly. Below are some vital considerations and techniques to keep in mind.
1. Keep Your Crontab Organized
As your scheduling needs grow, it becomes crucial to maintain an organized crontab file. Grouping similar tasks together and adding comments to clarify the purpose of each job can make your crontab much more navigable. For instance, you might separate system maintenance tasks from data processing tasks:
# System Maintenance Jobs 0 2 * * * /home/user/system_update.sh 0 3 * * * /home/user/cleanup_temp_files.sh # Data Processing Jobs 0 4 * * * /home/user/generate_reports.sh 0 5 * * * /home/user/export_data.sh
By organizing your jobs in this way, you create an easily understandable structure that allows for quick modifications and checks.
2. Use Full Paths for Scripts and Commands
Always specify the full path to the scripts or commands you’re invoking in your cron jobs. This practice helps avoid any potential issues with the execution context of cron jobs, which may not have the same PATH environment variable settings as your user shell:
# Example of using full paths 0 3 * * * /usr/bin/python3 /home/user/scripts/data_processing.py
Using absolute paths ensures that cron can locate the necessary files regardless of the current working directory.
3. Log Output and Errors
As previously mentioned, redirecting output and errors to log files is indispensable for troubleshooting. Ponder implementing a logging strategy for all your cron jobs to capture both successful executions and failures:
0 3 * * * /home/user/backup_script.sh >> /var/log/backup.log 2>&1
Regularly review these log files to identify patterns and ongoing issues, facilitating timely interventions.
4. Test Your Scripts Manually
Before scheduling a cron job, always run the script manually to ensure that it executes as expected. This preemptive step can help you catch errors and fix issues before they affect your automated processes:
bash /home/user/backup_script.sh
By rigorously testing scripts beforehand, you can identify and resolve potential problems early on.
5. Avoid Running Too Many Jobs At the same time
While it may be tempting to schedule multiple jobs at the same time, doing so can lead to resource contention and degraded performance. To prevent overwhelming your system, stagger your cron jobs so that they do not overlap significantly:
# Staggered job schedules 0 2 * * * /home/user/backup_script.sh 15 2 * * * /home/user/system_update.sh
By carefully considering scheduling, you ensure that each job has the resources it needs to execute effectively.
6. Monitor Cron Job Performance
Utilize monitoring tools to keep an eye on the performance of your cron jobs. Many systems provide logs or third-party monitoring solutions that can alert you to failures or performance degradation. Integrating these tools into your workflow can enhance visibility and allow you to respond proactively to issues:
For example, setting up a simple email notification for job failures can keep you informed:
[email protected] 0 3 * * * /home/user/backup_script.sh
This configuration sends an email to the specified address whenever the cron job fails, enabling you to take prompt action.