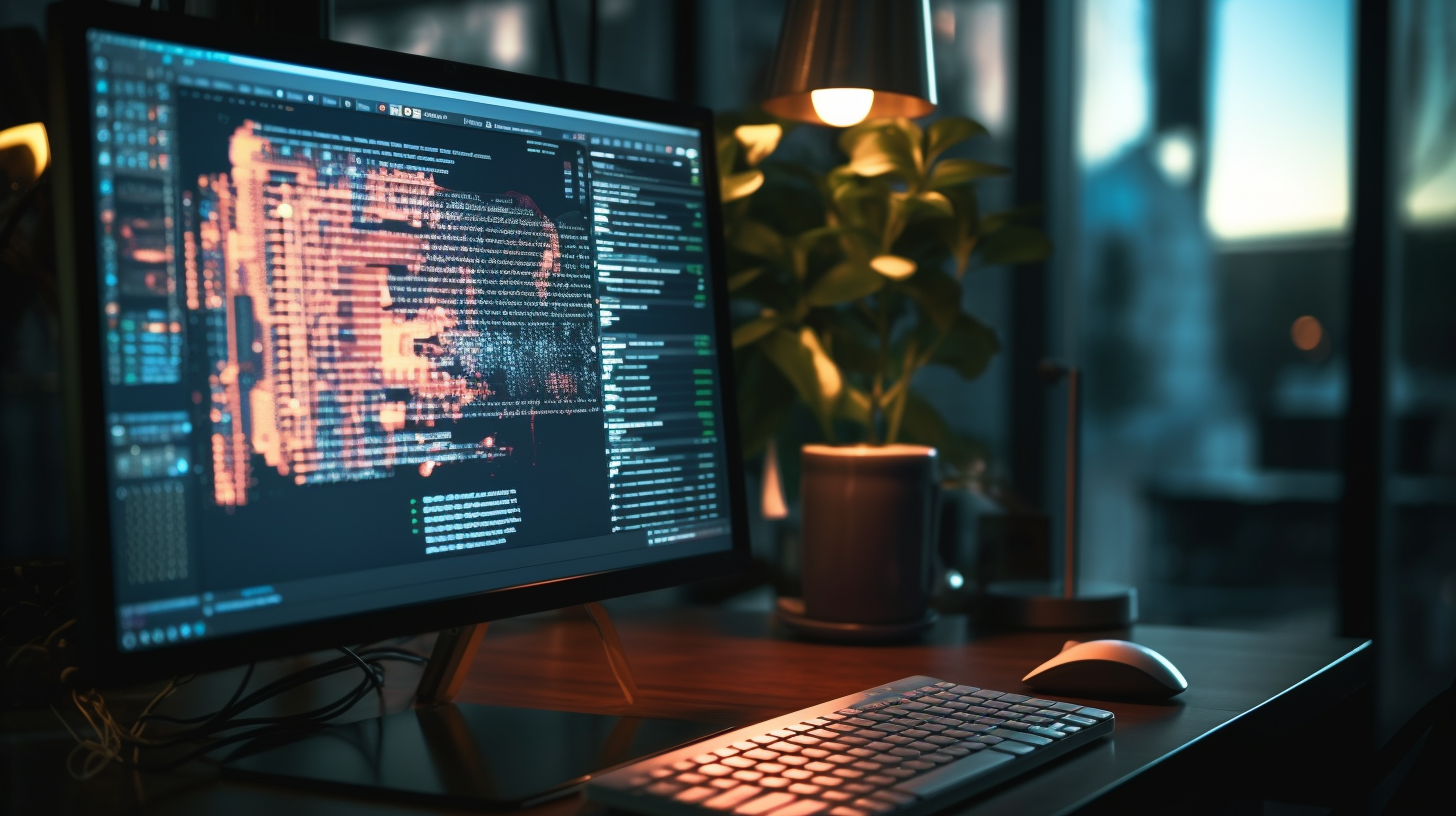
Custom Bash Command-Line Options
Understanding command-line options in Bash is important for creating versatile and easy to use scripts. At its core, a command-line option allows users to modify the behavior of a script or command when it is invoked. These options typically start with a single dash (-) for single-character options or a double dash (–) for long options. For instance, the command ls -l
uses the -l
option to list files in long format.
Bash scripts can be designed to accept these options, providing users with more control over the script’s execution. The key to effectively managing these options is understanding how to parse them accurately and how they’re represented in the context of your script.
Consider the following example, which shows a script that accepts a couple of options:
#!/bin/bash while getopts ":h:v" option; do case ${option} in h ) echo "Usage: script.sh [-h] [-v]" exit 0 ;; v ) echo "Verbose mode activated." ;; ? ) echo "Invalid option: -$OPTARG" 1>&2 exit 1 ;; esac done
In this example, the script uses the `getopts` built-in utility to handle command-line arguments. The :h:v
string defines two options: -h
for help and -v
for verbose mode. Notice how each option is treated within the case
statement, which allows the script to respond appropriately to user input.
Defining Custom Options with `getopts`
To define custom command-line options in your Bash scripts, the `getopts` built-in command offers a concise and effective method for parsing options and arguments. The basic syntax involves a string that specifies the options to be recognized, followed by the handling of those options in a loop.
The `getopts` command processes options in a way that is efficient and adheres to the conventions of Unix-style command-line interfaces. Each character in the option string represents a valid option, and if the option requires an argument, it should be followed by a colon. For instance, if you want to create an option `-o` that requires an argument, the option string would include `o:`.
Here’s a simple example that illustrates how to define and use a custom option that accepts an argument:
#!/bin/bash while getopts ":o:h" option; do case ${option} in o ) echo "Option -o specified with value: ${OPTARG}" ;; h ) echo "Usage: script.sh [-h] [-o value]" exit 0 ;; ? ) echo "Invalid option: -$OPTARG" 1>&2 exit 1 ;; esac done
In this example, the script defines two options: `-o` which accepts a value, and `-h` for help. The script responds to the `-o` option by echoing the provided value. The `OPTARG` variable holds the argument associated with the option, making it accessible for further processing.
When implementing options through `getopts`, it’s essential to ponder how your script handles invalid options or missing arguments. The error handling illustrated in the case statement demonstrates how to provide useful feedback to users, ensuring a better user experience.
Parsing Multiple Arguments
When it comes to parsing multiple arguments in Bash, the flexibility of the `getopts` command shines even brighter. This capability allows scripts to handle complex input scenarios where multiple options may be specified concurrently, enabling a richer interface for user interaction. The key to managing multiple arguments effectively lies in understanding how `getopts` processes these inputs sequentially while allowing for combinations of both options and their associated values.
Consider the following example, where we extend our previous script to accept multiple options, including one that requires an argument:
#!/bin/bash while getopts ":o:h:v" option; do case ${option} in o ) echo "Option -o specified with value: ${OPTARG}" ;; h ) echo "Usage: script.sh [-h] [-o value] [-v]" exit 0 ;; v ) echo "Verbose mode activated." ;; ? ) echo "Invalid option: -$OPTARG" 1>&2 exit 1 ;; esac done
In this script, the `-o` option requires a value, while `-h` serves as a help flag and `-v` activates verbose mode. The `getopts` loop processes each option as it encounters it. When the user runs the script with multiple options, such as:
./script.sh -o "example.txt" -v
the script will recognize and handle the `-o` option with its argument “example.txt” and also activate verbose mode. The output will be:
Option -o specified with value: example.txt Verbose mode activated.
It’s important to note that when using `getopts`, the order of options does not matter. The user can specify options in any sequence, and the script will still process them correctly. This feature contributes significantly to the usability of command-line interfaces, as users can prioritize their input according to their needs.
However, care must be taken when dealing with required arguments. If a user specifies `-o` without a corresponding value, `getopts` will return an error. You can manage such scenarios by adding validation logic in your case statement. For instance:
#!/bin/bash while getopts ":o:h:v" option; do case ${option} in o ) if [[ -z "${OPTARG}" ]]; then echo "Option -o requires an argument." 1>&2 exit 1 fi echo "Option -o specified with value: ${OPTARG}" ;; h ) echo "Usage: script.sh [-h] [-o value] [-v]" exit 0 ;; v ) echo "Verbose mode activated." ;; ? ) echo "Invalid option: -$OPTARG" 1>&2 exit 1 ;; esac done
In this enhanced version, we check if the `OPTARG` variable is empty when the `-o` option is specified. If it’s, an error message is displayed, and the script exits gracefully. This ensures that users receive immediate feedback about their input, enhancing the overall robustness of the script.
Handling Flags and Values
When dealing with flags and values in Bash scripts, it is essential to design your options in a manner that provides clarity and functionality. Flags are typically single-character options that toggle specific behaviors in your script, while values often represent associated data that modifies the action performed by the script. Understanding how to manage these components effectively can greatly enhance the usability of your scripts.
In Bash, a flag is indicated by a single dash followed by a character, such as `-v` for verbose mode or `-h` for help. Values associated with these flags can either be mandatory or optional, depending on how you want to structure your script. The `getopts` command is your ally here, as it facilitates the parsing of these options seamlessly.
Here’s how you might implement a script that combines both flags and values:
#!/bin/bash while getopts ":v:o:h" option; do case ${option} in v ) echo "Verbose mode activated." ;; o ) if [[ -z "${OPTARG}" ]]; then echo "Option -o requires a value." 1>&2 exit 1 fi echo "Option -o specified with value: ${OPTARG}" ;; h ) echo "Usage: script.sh [-h] [-v] [-o value]" exit 0 ;; ? ) echo "Invalid option: -$OPTARG" 1>&2 exit 1 ;; esac done
In this example, the script defines three options: `-v` for verbose mode, `-o` for specifying a value, and `-h` for displaying help information. When the script is executed, it checks which options were provided. If the `-o` flag is used without a corresponding value, the script will notify the user that a value is required.
To run the script with both a flag and a value, you might execute the following command:
./script.sh -v -o "example.txt"
The output would indicate that verbose mode is on and display the value for the `-o` option:
Verbose mode activated. Option -o specified with value: example.txt
When implementing flags and values, it’s also a good practice to ensure that your script can handle scenarios where flags might be present without the expected values. Using checks like the one illustrated above helps maintain a high standard of user interaction. By providing appropriate feedback, users can adjust their input accordingly.
Furthermore, managing the order of flags and values can enhance the script’s flexibility. Users expect to be able to specify options in various arrangements, and the `getopts` utility accommodates this expectation. This adaptability not only improves usability but also aligns with the conventions of other command-line tools, creating a familiar experience for the user.
Error Handling and Usage Messages
#!/bin/bash while getopts ":o:h:v" option; do case ${option} in o ) if [[ -z "${OPTARG}" ]]; then echo "Option -o requires a value." 1>&2 exit 1 fi echo "Option -o specified with value: ${OPTARG}" ;; h ) echo "Usage: script.sh [-h] [-o value] [-v]" exit 0 ;; v ) echo "Verbose mode activated." ;; ? ) echo "Invalid option: -$OPTARG" 1>&2 exit 1 ;; esac done
Error handling is a critical aspect of writing robust Bash scripts. The primary goal is to ensure that your scripts provide meaningful feedback to users when they make mistakes or misconfigure options. Using `getopts`, you can define specific error messages and exit codes to guide the user back on track.
In the previous examples, we see how invalid options trigger an error message. Specifically, when an unrecognized option is provided, `getopts` sets the variable `OPTARG`, which can be used to inform the user about the invalid input. This gives users immediate and clear feedback, helping them understand what went wrong:
#!/bin/bash while getopts ":o:h:v" option; do case ${option} in ? ) echo "Invalid option: -$OPTARG" 1>&2 exit 1 ;; esac done
Moreover, handling missing arguments is important for options that require a value. In the case of the `-o` option, if a user forgets to provide a value, your script should handle this gracefully:
#!/bin/bash while getopts ":o:h:v" option; do case ${option} in o ) if [[ -z "${OPTARG}" ]]; then echo "Error: Option -o requires a value." 1>&2 exit 1 fi echo "Option -o specified with value: ${OPTARG}" ;; h ) echo "Usage: script.sh [-h] [-o value] [-v]" exit 0 ;; esac done
By checking if `OPTARG` is empty, you can provide a tailored error message that directs users to correct their input. This not only enhances the user experience but also reduces the frustration that can arise from unclear command failures.
In addition to standard error messages, it’s also beneficial to include usage information when errors occur. This way, users can quickly see how to correctly use the script. The `-h` flag can be utilized effectively for this purpose:
#!/bin/bash while getopts ":o:h:v" option; do case ${option} in h ) echo "Usage: script.sh [-h] [-o value] [-v]" exit 0 ;; ? ) echo "Invalid option: -$OPTARG" 1>&2 echo "Run 'script.sh -h' for usage information." 1>&2 exit 1 ;; esac done
This approach not only informs users about the invalid option but also encourages them to learn how to use the script correctly by providing the usage command directly in the error message. This can make a significant difference in user satisfaction and script usability.