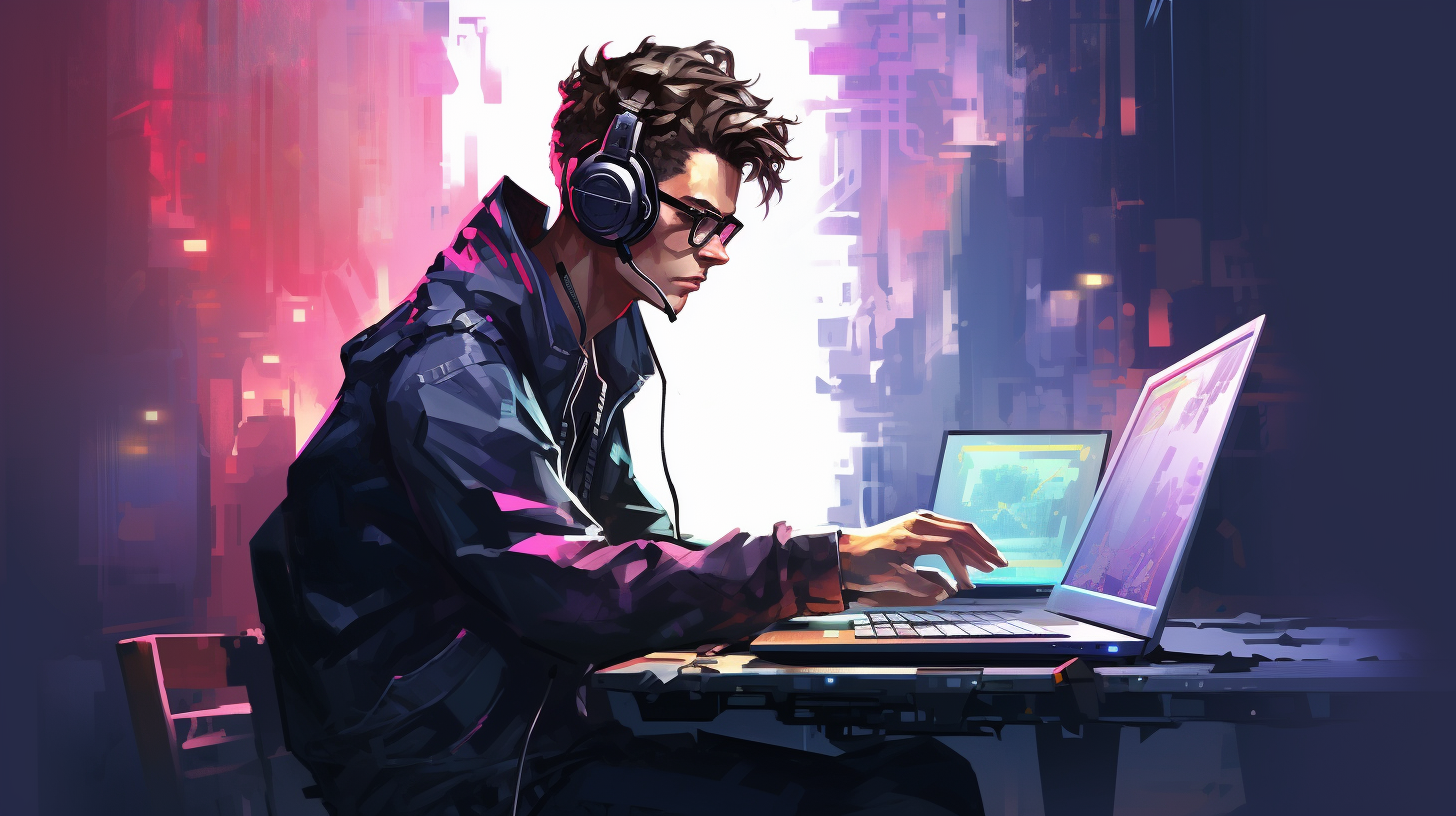
Customizing Bash for Productivity
Optimizing your Bash environment is critical for enhancing your productivity as a developer or system administrator. A well-tuned environment can significantly reduce the time spent navigating and executing commands, so that you can focus on the task at hand. Here are several strategies for achieving this.
1. Use a Custom Shell Configuration File
Start by creating a personalized shell configuration file, typically located at ~/.bashrc
. This file is executed whenever a new shell session starts, and it’s the ideal place to set your environment variables, aliases, and functions.
2. Set Environment Variables
Environment variables can streamline your workflow. For example, you might frequently access specific directories or tools. Setting variables can save you from typing full paths repeatedly. Here’s how to define a couple of useful variables:
export PROJECT_DIR=~/projects export SCRIPTS_DIR=~/scripts
After setting these, you can quickly navigate to your project directory with cd $PROJECT_DIR
or run a script with bash $SCRIPTS_DIR/myscript.sh
.
3. Create Shortcuts with Aliases
Aliases are a great way to create shortcuts for frequently used commands. This can speed up your workflow considerably. For example, you might define an alias to update your system easily:
alias update='sudo apt update && sudo apt upgrade'
Now you can simply type update
to perform both actions instead of typing out the full commands each time.
4. Configure the Command History
Efficient use of command history allows you to retrace your steps without retyping commands. You can enhance your history management by adding these lines to your ~/.bashrc
:
export HISTSIZE=10000 export HISTFILESIZE=20000 shopt -s histappend PROMPT_COMMAND="history -a; history -n"
With these settings, Bash will keep a larger history, append new entries to the history file instead of overwriting it, and load new entries automatically in the current session.
5. Customize the Prompt
Your command prompt can contain a wealth of information, such as the current directory, git branch, or even the execution time of the last command. Modifying the PS1
variable allows for a customized prompt. For instance:
export PS1="u@h:w [e[32m]$(git branch 2>/dev/null | grep '^*' | colrm 1 2)[e[0m] $ "
This configuration displays your username, hostname, current working directory, and the current git branch if you are in a repository, all in a visually appealing format.
6. Leverage Bash Completion
Bash completion allows you to automatically complete commands and file paths, significantly speeding up your command-line interactions. It’s often enabled by default, but you can ensure it’s active by adding the following to your ~/.bashrc
:
if [ -f /etc/bash_completion ]; then . /etc/bash_completion fi
This script checks if the bash completion file exists and sources it, giving you the power of context-aware command completion.
By implementing these optimization techniques, you will foster a more efficient and responsive Bash environment that caters to your specific workflow needs. Each adjustment you make brings you closer to a streamlined and productive command-line experience.
Essential Bash Aliases and Functions
When it comes to enhancing your productivity in Bash, the use of aliases and functions is paramount. These tools allow you to create shortcuts for complex commands and automate repetitive tasks, freeing you from the drudgery of typing the same lines over and over again. Let’s delve into how to effectively implement essential Bash aliases and functions.
Creating Bash Aliases
Aliases can significantly cut down on your typing and minimize the chances of errors. You can define an alias in your ~/.bashrc file using the following syntax:
alias name='command'
For example, if you often find yourself navigating to a project’s directory, you can set an alias like this:
alias proj='cd ~/projects/my_project'
Now, instead of typing the full command, you can simply type proj
to jump directly to your project directory.
You can also create more complex aliases by combining commands. For instance, if you frequently check the status of your Git repository, you might want to create an alias that performs multiple commands:
alias gs='git status && git diff'
This alias, when executed, will show you the status of your Git repository and any changes that are not yet staged, right in one go.
Defining Functions for More Complex Tasks
While aliases work great for simpler commands, you might find that you need more functionality for repetitive tasks. This is where functions come into play. Defining a function in your ~/.bashrc follows this structure:
function_name() { command1 command2 ... }
For example, you might want to create a function that updates your system and cleans up old packages:
update_system() { sudo apt update && sudo apt upgrade -y && sudo apt autoremove -y }
Once defined, you can simply type update_system
to execute all those commands at the same time, making your workflow much more efficient.
Combining Functions and Arguments
Functions in Bash can also take arguments, which allows for even more flexibility. For instance, if you often create directories and want to immediately navigate into them, you could define a function like this:
mkcd() { mkdir -p "$1" && cd "$1" }
With this function, you can create a directory and change into it in a single command by typing mkcd new_directory
.
Using `eval` for Dynamic Aliases
Sometimes, you may want to create aliases or functions that are generated dynamically based on the current environment or user inputs. The eval
command can be useful here. For instance, if you want to create an alias for the current directory:
eval "alias here='cd $(pwd)'"
This command will create an alias called here
that always refers to the directory from which it was called.
By using aliases and functions, you can reduce the cognitive load of remembering complex commands and improve your overall efficiency in Bash. The simplicity of these mechanisms belies their power—once you start incorporating them into your daily routine, you’ll wonder how you ever managed without them.
Advanced Prompt Customization
Advanced prompt customization in Bash can transform your command-line experience, providing not only aesthetic improvements but also valuable contextual information. A well-designed prompt can help you stay oriented within your file system, keep track of version control states, and even display the execution time for commands—all of which contribute to a more productive workflow.
The cornerstone of prompt customization lies in the PS1 variable, which defines how your prompt appears. Understanding the escape sequences that PS1 supports will enable you to create a prompt tailored to your needs. Here’s a breakdown of some useful escape sequences:
- u – Displays the username of the current user.
- h – Shows the hostname up to the first period.
- w – Indicates the current working directory, displaying the full path.
- $ – Represents the prompt character; it’s a $ for normal users and a # for the root user.
- MM:SS format.
- d – Shows the date in “Weekday Month Date” format.
For example, you can customize your prompt to display your username, the current directory, and the time like so:
export PS1="u@h:w t $ "
This configuration provides a clear context every time you see your prompt, making it easier to track your position in the directory structure and the time elapsed since starting your session.
Perhaps one of the most powerful features of prompt customization is integrating version control status directly into your prompt. If you frequently use Git, you can include the current branch name in your prompt. Here’s how to do it:
export PS1="u@h:w [e[32m]$(git branch 2>/dev/null | grep '^*' | colrm 1 2)[e[0m] $ "
In this example, the prompt will display the current Git branch in green when you’re within a Git repository, so that you can see where you are without having to run a separate command.
Furthermore, you might want to track the execution time of your last command. That’s particularly useful for assessing performance during development or debugging sessions. To display the execution time, you can modify your PS1 as follows:
export PS1="[t][u@h w] [33[0;32m]$(echo $? == 0 ? '✓' : '✗')[33[0m] $ "
This prompt includes the time the command was executed and indicates whether the last command was successful or not, with a check mark or a cross.
To make your prompt visually appealing, you can also add colors and formatting. Bash supports ANSI escape codes, allowing you to modify the text color, style, and background. Here’s an example of a colorful prompt:
export PS1="[e[1;34m]u@h[e[0m]:[e[1;32m]w[e[0m] [e[1;31m]$(git branch 2>/dev/null | grep '^*' | colrm 1 2)[e[0m] $ "
In this version, the username and hostname are shown in blue, while the current working directory is green, and the Git branch name is displayed in red. This kind of customization not only provides functional benefits but also enhances your command-line experience by making it visually richer and more informative.
Finally, don’t forget that any changes you make to your prompt will only take effect for new terminal sessions unless you source your ~/.bashrc file after editing it:
source ~/.bashrc
By investing time in customizing your prompt, you will create a command-line environment this is not only efficient but also reflective of your personal style and workflow, ultimately enhancing your productivity and enjoyment while working in Bash.
Enhancing Productivity with Shell Scripts
Shell scripts are a powerful way to automate repetitive tasks, streamline your workflow, and enhance your productivity in Bash. By using the scripting capabilities of Bash, you can create scripts that handle everything from simple file management to complex system administration tasks. Below are some strategies and examples that show how to effectively use shell scripts to boost your output.
Writing Your First Shell Script
To get started with shell scripting, create a new file with a .sh extension, for example, script.sh. Begin the script with the shebang line, which tells the system that this file is a Bash script:
#!/bin/bash
After the shebang, you can add your commands just as you would type them in the terminal. For example:
#!/bin/bash echo "Hello, World!"
Make the script executable with the chmod command:
chmod +x script.sh
Now you can run your script with:
./script.sh
Automating Daily Tasks
Shell scripts excel at automating routine tasks. For instance, if you find yourself backing up certain directories every day, you can automate this with a simple script:
#!/bin/bash tar -czf ~/backup_$(date +%F).tar.gz ~/important_directory
This script creates a compressed backup of the important_directory and timestamps the backup file with the current date. Running this script daily ensures that you always have the latest backup without manual intervention.
Using Command-Line Arguments
Making your scripts flexible can significantly enhance their utility. You can pass arguments to your scripts, allowing them to perform different tasks based on input. Here’s an example of a script that takes a directory path as an argument:
#!/bin/bash if [ -d "$1" ]; then echo "Listing files in $1:" ls -l "$1" else echo "Directory $1 does not exist." fi
Call this script with a directory path:
./list_files.sh /path/to/directory
Implementing Control Structures
Control structures in Bash, such as loops and conditionals, can empower your scripts to handle more complex scenarios. For instance, if you want to process files in a directory, you can use a loop:
#!/bin/bash for file in ~/documents/*; do echo "Processing $file" # Add your processing commands here done
This script iterates over each file in the ~/documents directory and processes it according to your specified commands.
Logging and Error Handling
Effective logging and error handling can make your scripts more robust and easier to debug. You can redirect output and error messages to a log file like this:
#!/bin/bash exec > script.log 2>&1 echo "Script started at $(date)" # Your commands here echo "Script finished at $(date)"
This setup captures both standard output and errors in script.log, allowing you to review the script’s execution later.
Scheduling Scripts with Cron
To further enhance productivity, you can schedule your scripts to run at specific intervals using cron jobs. To edit your cron jobs, use:
crontab -e
Then add a line specifying when to run your script. For example, to run your backup script daily at midnight, you could add:
#!/bin/bash echo "Hello, World!"
0
This ensures that your backup runs automatically without any manual effort on your part.
By incorporating shell scripts into your workflow, you can significantly reduce the time spent on repetitive tasks, minimize errors, and maintain a clear focus on your primary objectives. The flexibility and power of Bash scripting offer endless possibilities for enhancing productivity in your daily operations.