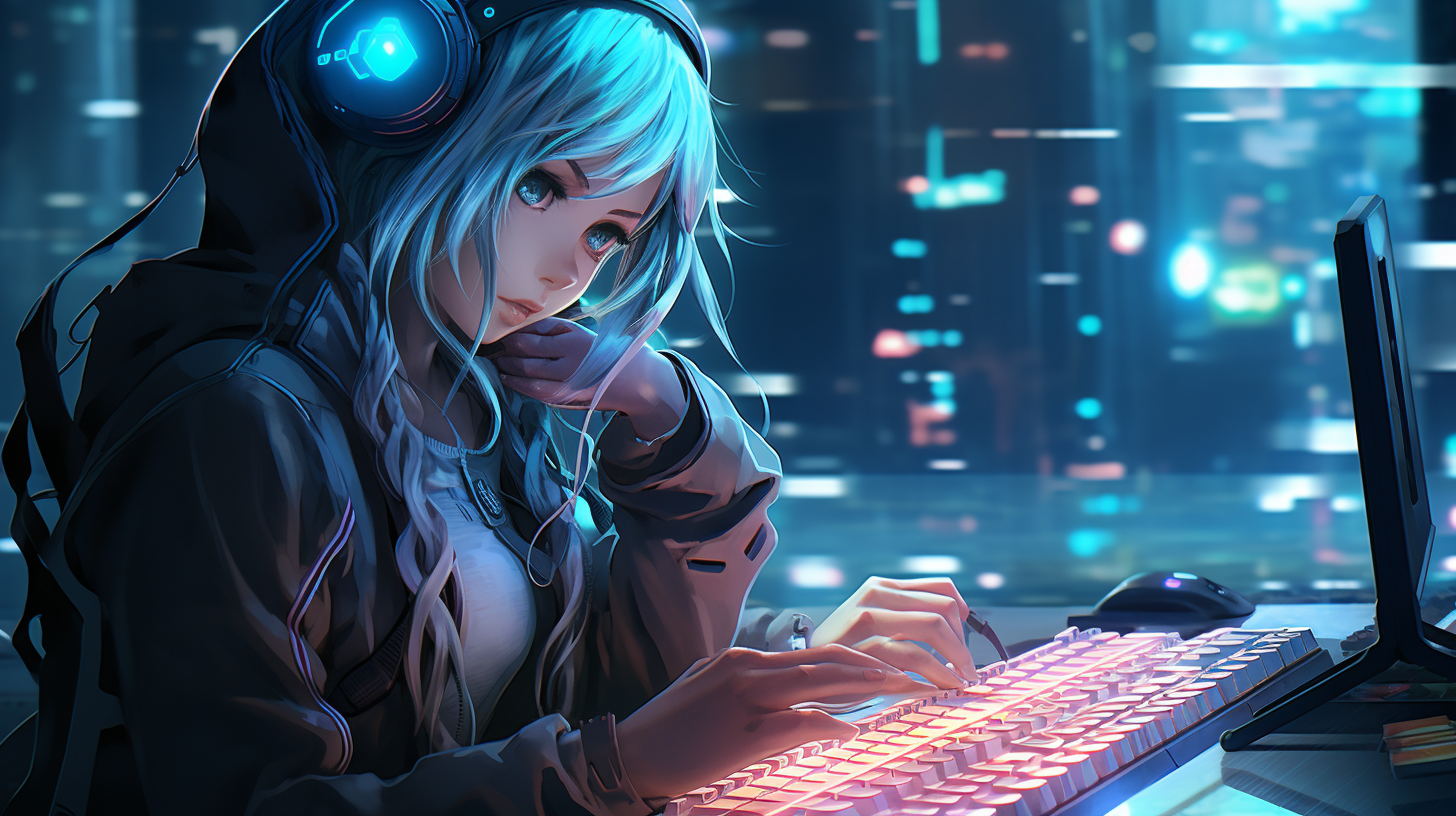
Dependency Injection in Swift
Dependency injection is a design pattern that allows for the separation of concerns within your applications, leading to more modular, testable, and maintainable code. At its core, dependency injection involves providing an object (often referred to as a client) with its dependencies rather than having the object construct them itself. This approach helps to reduce the coupling between classes and enables easier substitution of components, particularly useful in unit testing.
In Swift, dependency injection can be implemented in several ways, each with its own advantages and trade-offs. By using this pattern, you can create more flexible applications that are easier to manage as they grow in complexity.
Think a simple example where a class Car
depends on another class Engine
. Without dependency injection, the Car
class might look like this:
class Engine { func start() { print("Engine started") } } class Car { private var engine: Engine init() { self.engine = Engine() // Directly creating the dependency } func start() { engine.start() } }
This implementation tightly couples the Car
class to the Engine
class, making it difficult to test the Car
class in isolation. If you wanted to test the Car
class without starting an actual engine, you would have a challenging time.
By using dependency injection, we can modify the Car
class to accept an Engine
instance through its initializer:
class Car { private var engine: Engine init(engine: Engine) { // Injecting the dependency self.engine = engine } func start() { engine.start() } }
Now, Car
is no longer responsible for creating its own Engine
instance. This change decouples the two classes and allows us to pass in different Engine
implementations. That’s particularly beneficial when it comes to writing unit tests:
class MockEngine: Engine { var didStart = false override func start() { didStart = true // Capturing that the engine has started } } // In your test case let mockEngine = MockEngine() let car = Car(engine: mockEngine) car.start() assert(mockEngine.didStart) // Ensure that the engine's start method was called
Through this approach, you’ve ensured that your Car
class can work with any implementation of Engine
, promoting flexibility and testability. In a broader sense, dependency injection can streamline your architecture by allowing each class to focus on its specific responsibilities while relying on others to fulfill their roles.
Types of Dependency Injection
When discussing the various types of dependency injection in Swift, it’s essential to recognize that while the overarching goal remains the same—decoupling components for better testability and maintainability—the methods of achieving this can vary significantly. The prominent types of dependency injection include constructor injection, property injection, and method injection. Each method has its own use cases and implications on design.
1. Constructor Injection: That is perhaps the most common method of dependency injection. As demonstrated in the previous example, constructor injection allows dependencies to be provided at the moment of an object’s creation. This approach ensures that the instance is fully initialized and ready to use, and it enforces that the client must have its dependencies supplied to it. The main advantage of constructor injection is that it makes dependencies explicit, enhancing the readability of the code.
class Car { private var engine: Engine init(engine: Engine) { self.engine = engine } func start() { engine.start() } }
Constructor injection can be particularly useful when a class has multiple dependencies. However, too many dependencies can lead to a bloated constructor, making it harder to manage.
2. Property Injection: In contrast to constructor injection, property injection allows dependencies to be set after an object has been created. This can be done through publicly accessible properties or specialized setter methods. Property injection can offer more flexibility, making it possible to change dependencies at runtime.
class Car { private var engine: Engine? var engine: Engine? { didSet { // Optional logic when engine is set } } func start() { engine?.start() } }
While property injection can simplify the initialization of complex objects, it also carries the risk of leaving the object in an incomplete state since the dependencies may not be set when methods are called. Therefore, it’s crucial to establish clear guidelines for when and how properties should be set.
3. Method Injection: Method injection involves passing dependencies directly to the methods that require them. This can be particularly useful for dependencies that are only needed in specific contexts or operations, rather than being associated with the entire lifecycle of the object.
class Car { func start(engine: Engine) { engine.start() } }
Method injection promotes a high degree of flexibility as different engines can be passed for different operations. However, if overused, it can lead to unclear method signatures and make the code harder to read.
Each type of dependency injection serves its purpose, and the choice between them should be guided by the specific needs of your application. Constructor injection is excellent for mandatory dependencies, property injection offers flexibility, and method injection is ideal for occasional use cases. By understanding these types, you can make informed decisions that enhance the modularity and clarity of your codebase.
Implementing Dependency Injection with Protocols
Implementing dependency injection with protocols in Swift allows for a high degree of flexibility and adherence to the principles of abstraction and interface segregation. By defining a protocol that outlines the expected behavior of a dependency, you can create multiple implementations that can be injected into the client class. This approach paves the way for a more robust, testable architecture while keeping your code clean and manageable.
Let’s take a look at how this can be achieved by refining our previous Car and Engine example. First, we define a protocol that specifies the methods our Engine should implement. This decouples the Car class from any concrete Engine implementation and allows for easier testing and extension.
protocol Engine { func start() } class GasolineEngine: Engine { func start() { print("Gasoline engine started") } } class ElectricEngine: Engine { func start() { print("Electric engine started") } } class Car { private var engine: Engine init(engine: Engine) { self.engine = engine } func start() { engine.start() } }
In the code above, we define an Engine
protocol that declares the start()
method. We then create two concrete classes, GasolineEngine
and ElectricEngine
, that conform to this protocol. The Car
class now depends on the Engine
protocol rather than a specific implementation.
This abstraction allows us to easily swap different engine types without altering the Car class. For instance, to test the Car class without needing a real engine, you can create a mock implementation of the Engine protocol:
class MockEngine: Engine { var didStart = false func start() { didStart = true // Capturing that the start method was called } } // In your test case let mockEngine = MockEngine() let car = Car(engine: mockEngine) car.start() assert(mockEngine.didStart) // Ensure that the start method was called
Using a protocol for dependency injection not only enhances testability but also promotes adherence to the Dependency Inversion Principle, one of the core tenets of SOLID design principles. This principle encourages high-level modules (like Car) to depend on abstractions (like Engine) rather than concrete implementations.
Another benefit of this approach is that it allows for easy compliance with future requirements. If you need to introduce a new engine type, you simply create a new class that conforms to the Engine protocol without modifying the Car class. This makes your codebase more maintainable and scalable in the long run.
Implementing dependency injection through protocols in Swift fosters a clean separation of concerns, facilitating easier updates, testing, and adherence to design principles that lead to maintainable software architecture.
Constructor vs. Property Injection
When it comes to dependency injection, the choice between constructor injection and property injection can significantly impact how your application is structured and how easily it can be modified or tested. Both methods have their advantages and trade-offs, and understanding them is important for making informed design decisions.
Constructor Injection is a simpler method where dependencies are provided at the time of object creation. This approach promotes immutability and makes it clear that the object cannot function without its dependencies, which are explicitly defined in the initializer. Consider the following Swift code:
class Car { private var engine: Engine init(engine: Engine) { self.engine = engine } func start() { engine.start() } }
In this example, the Car
class requires an Engine
instance to function. The dependency is injected via the initializer, ensuring that whenever a Car
is created, it has a valid Engine
to work with. This makes the class easier to test because you can pass in mock or stub engines during testing, ensuring that the Car
class behaves correctly under various conditions.
However, constructor injection can lead to issues when a class has many dependencies. If a class requires several different types of objects to be initialized, the constructor can become unwieldy, potentially making the code harder to read and maintain. It’s essential to keep this in mind and strive to limit the number of dependencies that are passed through the constructor.
Property Injection, on the other hand, allows dependencies to be set after an object’s creation. This method can make the initialization process simpler, especially for classes with a high number of dependencies. Here’s how property injection can be implemented:
class Car { private var engine: Engine? var engineProperty: Engine? { didSet { // Optional logic when engine is set } } func start() { engine?.start() } }
In this example, the Car
class exposes a property for the Engine
, which can be assigned after the object is created. While this approach adds flexibility, it also introduces the risk that methods like start()
might be called before the engine
is set, leading to runtime errors. To mitigate this risk, it very important to establish a clear contract for when properties should be set and to consider implementing safeguards that enforce the presence of necessary dependencies before methods are invoked.
Ultimately, the choice between constructor and property injection should be guided by the specific requirements and design of your application. Constructor injection is best suited for mandatory dependencies that an object needs to function correctly right from the start, while property injection offers flexibility for optional dependencies that may vary throughout the object’s lifecycle. By carefully considering the implications of each method, you can design more robust and maintainable Swift applications.
Best Practices and Common Pitfalls
When implementing dependency injection in Swift, adhering to best practices especially important to avoid common pitfalls that can undermine the benefits of this pattern. A few key principles can guide your implementation, ensuring your architecture remains clean, testable, and maintainable.
1. Favor Constructor Injection Over Property Injection: While property injection can offer flexibility, it often leads to incomplete or inconsistent object states if dependencies are not set before methods are invoked. Constructor injection, on the other hand, necessitates providing all required dependencies at instantiation, making it clear what the object needs to operate correctly. This can prevent runtime errors and promote immutability, as the dependencies remain consistent throughout the object’s lifecycle.
class Car { private var engine: Engine init(engine: Engine) { self.engine = engine } func start() { engine.start() } }
2. Limit the Number of Dependencies: A class that requires an excessive number of dependencies can become difficult to manage and test. Strive to limit dependencies to a manageable number, ideally no more than three or four. If a class has too many dependencies, consider refactoring it into smaller, more focused components. This separation can enhance readability and promote adherence to the Single Responsibility Principle.
class Vehicle { private var engine: Engine private var fuel: Fuel private var transmission: Transmission init(engine: Engine, fuel: Fuel, transmission: Transmission) { self.engine = engine self.fuel = fuel self.transmission = transmission } }
3. Use Protocols for Abstraction: Relying on concrete implementations can lead to brittle code. By using protocols for your dependencies, you can achieve greater flexibility and easier testing. This allows for different implementations to be swapped without impacting the client class, fostering a more modular architecture.
protocol Engine { func start() } class MockEngine: Engine { var didStart = false func start() { didStart = true } }
4. Be Cautious with Method Injection: While method injection can promote flexibility by allowing dependencies to be passed directly to methods, overusing this pattern can lead to unclear code. It can become difficult to determine the expectations for method parameters, especially if the method signatures become overly complicated or numerous. Maintain clarity by using method injection sparingly and ensuring that the method signatures remain intuitive and manageable.
class Car { func start(with engine: Engine) { engine.start() } }
5. Document Assumptions and Expectations: Clear documentation of your classes and their dependencies is vital. This includes specifying which dependencies are required, optional, or expected to be set before method invocations. Good documentation can alleviate confusion for anyone maintaining the code, especially in larger projects where developers may come and go.
6. Employ Dependency Injection Frameworks Judiciously: While frameworks can simplify dependency injection, introducing them into your project can add complexity. Use them only when the benefits outweigh the costs, and ensure that they align with your project’s goals. Frameworks can obscure what’s happening under the hood, making debugging more difficult, so weigh the decision carefully.
By following these best practices and being mindful of common pitfalls, you can leverage dependency injection in Swift to create clean, maintainable, and testable code. Keeping your code modular and adhering to established design principles will enhance your application’s longevity and adaptability as requirements evolve.