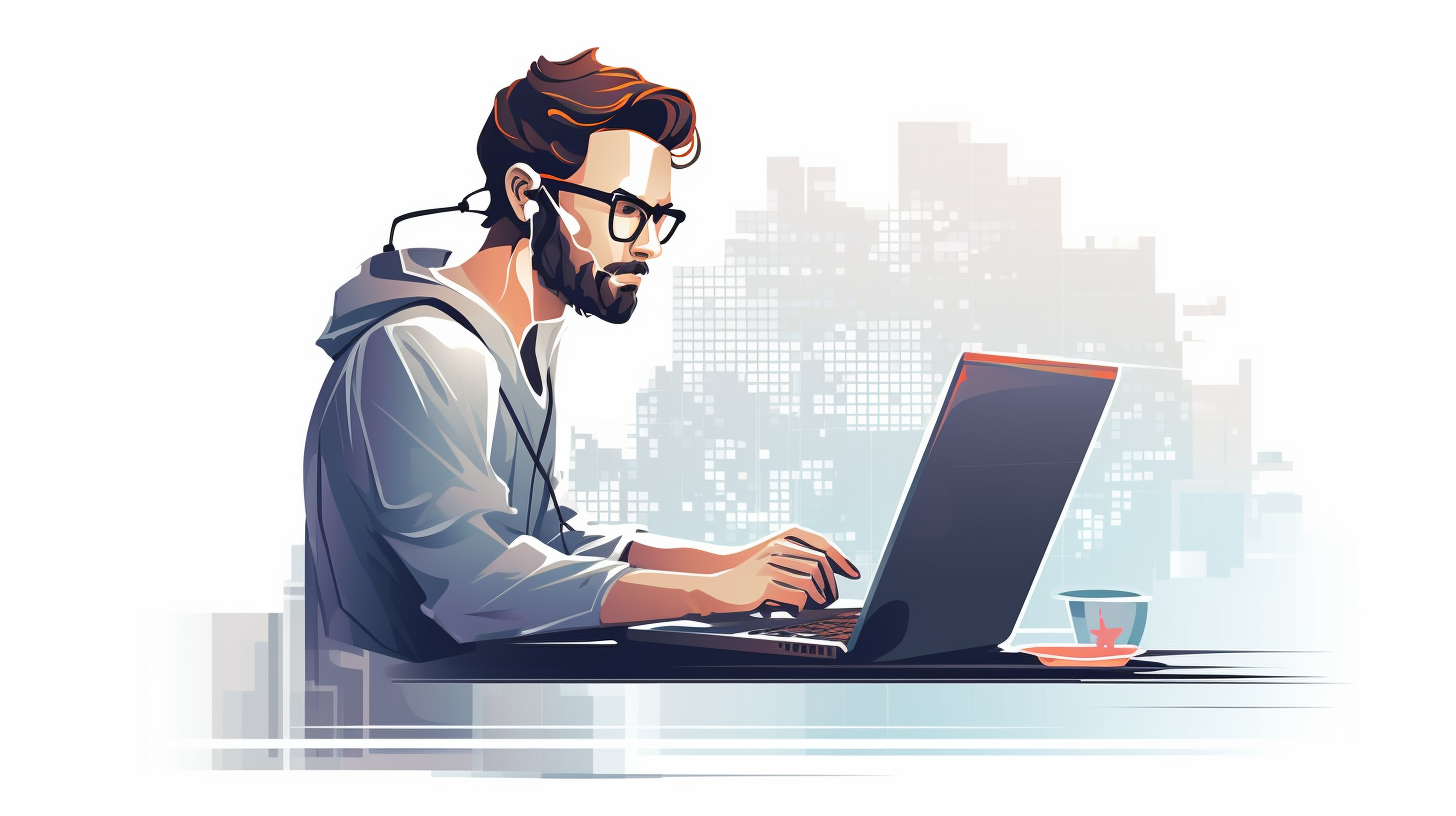
Directory Traversal with Bash
Within the scope of Bash scripting, directory traversal refers to the process of navigating through directories and their contents systematically. Understanding this concept is important for anyone looking to manipulate files or automate tasks within a file system. In Bash, directory traversal allows you to explore directory structures, locate files, and perform operations based on their locations.
At its core, directory traversal is about moving between directories and accessing the files they contain. The common commands used for this purpose are cd
, ls
, and various utilities like find
and grep
. These tools provide powerful capabilities to list, search, and modify files and directories effectively.
One of the foundational aspects of directory traversal in Bash is the ability to understand and utilize relative and absolute paths. An absolute path specifies the location of a directory or file from the root of the filesystem, while a relative path is based on the current working directory. For example:
Basics of Navigation Commands
To delve deeper into the basics of navigation commands in Bash, let’s explore how these commands can be combined with other functionalities to streamline your workflow. The cd command is fundamental, enabling you to change directories with ease. Accompanying it, the ls command lists the contents of a directory, providing a clear view of files and subdirectories. Here’s how you can begin using them together:
cd /path/to/directory ls -l
The -l option with ls gives a detailed listing, including permissions, ownership, size, and modification date, which can be incredibly helpful for managing files effectively.
Sometimes, it may be necessary to check your current directory to ensure you are in the right location before performing operations. The pwd command serves this purpose:
pwd
This command outputs the full pathname of the current working directory, confirming your location in the directory structure.
Furthermore, using combination commands can elevate your navigation efficiency. For example, you might want to change to a directory and immediately list its content. This can be achieved with a simple command sequence:
cd /path/to/directory && ls
This command changes the directory and, upon successful navigation, lists the files within it. The && operator ensures that ls only runs if cd is successful, preventing potential errors from executing in a non-existent directory.
Additionally, you can use the find command to traverse directories while allowing for more complex search criteria. For instance, if you are interested in locating all the files with a specific extension, the command looks like this:
find /path/to/search -type f -name "*.txt"
This command searches for files (denoted by -type f) with a .txt extension, starting from the specified path. The find command is a powerful ally in directory traversal, significantly enhancing your ability to locate files across extensive directory structures.
Lastly, using environment variables can make navigation easier. For example, you can set a variable for a frequently accessed directory:
export MY_DIR="/home/user/documents"
Once this variable is set, you can easily change to this directory at any point by referencing the variable:
cd $MY_DIR
Using Loops for Recursive Directory Traversal
When it comes to recursive directory traversal in Bash, loops become an invaluable tool. They allow you to systematically explore each directory and its subdirectories without having to specify each path manually. This is particularly useful when dealing with large directory structures where you need to perform operations on all files or specific subsets of files. The two primary types of loops in Bash are the for loop and the while loop, both of which can be effectively employed to navigate through directories.
To initiate a recursive traversal, the find command is often leveraged in tandem with a loop. The find command iterates through a directory and its subdirectories, which will allow you to specify criteria such as file type or name patterns. For example, if you want to execute a command on every text file found within a directory and its subdirectories, you can use:
find /path/to/start -type f -name "*.txt"
This command finds all files with a .txt extension starting from the specified directory. However, to perform operations on each of these files, you can pipe the output of find into a while loop. Here’s how it looks:
find /path/to/start -type f -name "*.txt" | while read file; do echo "Processing $file" # Insert any operations you want to perform on the file here done
In this snippet, for each file found, the script outputs a message indicating that it is processing that file, and you can add additional commands within the loop to manipulate the files as needed. This approach is particularly clean and efficiently handles the traversal without needing to maintain a separate list of files.
Another way to achieve recursive directory traversal is through the use of a for loop with an array. This technique can be particularly useful when you want to collect files into an array for later processing. Here’s an example of how to do this:
files=( $(find /path/to/start -type f -name "*.txt") ) for file in "${files[@]}"; do echo "Found file: $file" # Additional operations can be performed here done
In this case, the find command populates the array files
with all the matching file names. The for loop then iterates over each element of the array, which will allow you to handle each file individually. This offers flexibility as you can easily manipulate the contents of the array before processing the files.
When constructing loops for directory traversal, it’s crucial to manage potential pitfalls like spaces in filenames. To handle filenames containing spaces or special characters robustly, you can use a while loop with double quotes around the variable:
find /path/to/start -type f -name "*.txt" | while IFS= read -r file; do echo "Processing file: "$file"" done
Using IFS=
ensures that leading/trailing whitespace is preserved, and -r
prevents backslashes from being interpreted as escape characters, both of which are vital for processing filenames correctly.
Implementing Find and Grep in Directory Traversal
find /path/to/start -type f -name "*.txt" | while IFS= read -r file; do echo "Processing file: "$file"" done
The power of combining the `find` and `grep` commands in directory traversal cannot be overstated. When you need to search through files for specific content while traversing directories, `grep` complements the file locating capabilities of `find` perfectly. This synergy allows you to pinpoint not just the files you need, but the exact lines within those files that meet your search criteria.
To illustrate, if you’ve already identified a target directory and want to search through all `.txt` files for a specific keyword, you can streamline this operation using a command like:
find /path/to/start -type f -name "*.txt" -exec grep -H "search_term" {} ;
Here, the `-exec` flag tells `find` to execute `grep` on each file it finds that matches the criteria. The `{}` placeholder is replaced by the current file name, and the `;` signifies the end of the command. The `-H` option with `grep` ensures that the filename is prefixed to the matching lines in the output, which is invaluable for clarity when scanning through results.
For more nuanced searches, you might want to include options with `grep`. For instance, if you’re interested in case-insensitive matches or want to search for whole words only, you can add the `-i` or `-w` flags, respectively:
find /path/to/start -type f -name "*.txt" -exec grep -i -w "search_term" {} ;
Now, this command will ignore case and ensure that only complete words are matched, reducing false positives.
Furthermore, if your search needs to be limited to a certain depth in the directory structure, you can utilize the `-maxdepth` option with `find`. That is particularly useful when you want to search only within the top levels of a directory without delving deeper into nested subdirectories:
find /path/to/start -maxdepth 2 -type f -name "*.txt" -exec grep -H "search_term" {} ;
This command restricts the search to two levels down from the specified starting directory, allowing for more controlled and efficient searches.
Handling Symbolic Links and Special Directories
When navigating through directories in Bash, it’s essential to recognize how symbolic links and special directories can influence your operations. Symbolic links, or symlinks, are essentially pointers that reference another file or directory, allowing for flexible file system navigation. However, they can also introduce complexities when traversing directories, particularly if your script doesn’t account for them.
To handle symbolic links effectively, the find
command provides options that allow you to either follow these links or ignore them. By default, find
will not follow symlinks, but if you want to include them in your search, the -L
option is your ally. For example:
find -L /path/to/start -type f -name "*.txt"
This command will traverse through the specified path, following any symbolic links it encounters along the way. Consequently, files that are pointed to by symlinks will also be included in your search results. However, be mindful that this can lead to infinite loops if your symlinks create circular references. To mitigate this risk, you can use the -maxdepth
option in conjunction with -L
to limit how deep find
will search:
find -L /path/to/start -maxdepth 2 -type f -name "*.txt"
In contrast, if you want to avoid following symlinks altogether, you can use the -P
option (which is the default behavior) to ensure that only regular files and directories are processed:
find -P /path/to/start -type f -name "*.txt"
Special directories, such as ..
(parent directory) and .
(current directory), also play an important role in directory traversal. When you wish to perform actions in these directories, be cautious with your commands to prevent unintended consequences. For example, using rm
indiscriminately in the current directory can lead to the deletion of all files therein:
rm -rf ./*
This command clears all files in the current directory, which might include critical configuration files or data. To ensure safety, always verify your working directory with pwd
before executing commands that modify or delete files.
Additionally, when dealing with hidden files—those whose names start with a dot (.)—ensure your commands account for them. For instance, when listing files, you might want to include hidden files using:
ls -la
Best Practices for Secure Directory Traversal
When engaging in directory traversal, particularly in scripts that may operate on various file systems or user data, adhering to best practices for secure directory traversal is paramount. Security vulnerabilities can arise from improper handling of paths, leading to issues such as directory traversal attacks, where malicious users may manipulate input to gain unauthorized access to files outside the designated directories. Therefore, a robust approach is necessary to safeguard against such risks.
One of the first steps in securing directory traversal operations is to validate and sanitize user input rigorously. When accepting directory paths or filenames from user input, it is essential to check for any patterns that may indicate manipulation attempts, such as the use of ‘../’ sequences designed to traverse up the directory tree. By implementing validation checks, you can ensure that the input adheres to expected formats. For example:
if [[ "$input_path" =~ ^/home/user/documents/.* ]]; then echo "Valid path: $input_path" else echo "Invalid path!" exit 1 fi
This snippet checks if the user-provided path starts with a specified base directory. If the input deviates from this expected structure, it’s deemed invalid, and the script terminates, preventing potential unauthorized access.
Additionally, when performing file operations, it’s prudent to use absolute paths derived from a trusted base directory rather than relying on relative paths. This approach limits the script’s exposure to unintended directories and files. For instance, instead of allowing users to specify arbitrary paths directly, you might enforce a base directory:
BASE_DIR="/home/user/documents" FULL_PATH="$BASE_DIR/$input_file" if [[ -f "$FULL_PATH" ]]; then echo "Processing file: $FULL_PATH" else echo "File does not exist or is not accessible." fi
This pattern ensures that the script only interacts with files within the defined base directory, thereby limiting the potential for a malicious actor to access sensitive files elsewhere on the system.
Moreover, employing the principle of least privilege is important when executing directory traversal scripts. Running scripts under a user account with restricted permissions minimizes the impact of any unintended actions. For example, avoid running your scripts as the root user unless absolutely necessary. Instead, create a dedicated user with limited access to specific directories and files required by the script.
Furthermore, when using commands like `find`, be cautious with the `-exec` flag, as executing external commands can inadvertently expose your system to vulnerabilities if not controlled properly. Always validate any variables used in these commands. For example:
find "$BASE_DIR" -type f -name "*.txt" -exec grep -H "search_term" {} ;
In this case, ensuring that `”$BASE_DIR”` is sanitized and controlled reduces the risk of executing commands on unintended files.
Finally, always maintain a robust logging mechanism. Log all operations performed during directory traversal, including successful and failed attempts, as well as any user inputs. This practice not only aids in debugging but also provides a trail for auditing operations, which can be invaluable in identifying potential security breaches. A simple logging example could look like this:
{ echo "$(date): Accessed file $FULL_PATH" >> access.log } || { echo "$(date): Failed to access $FULL_PATH" >> access.log }