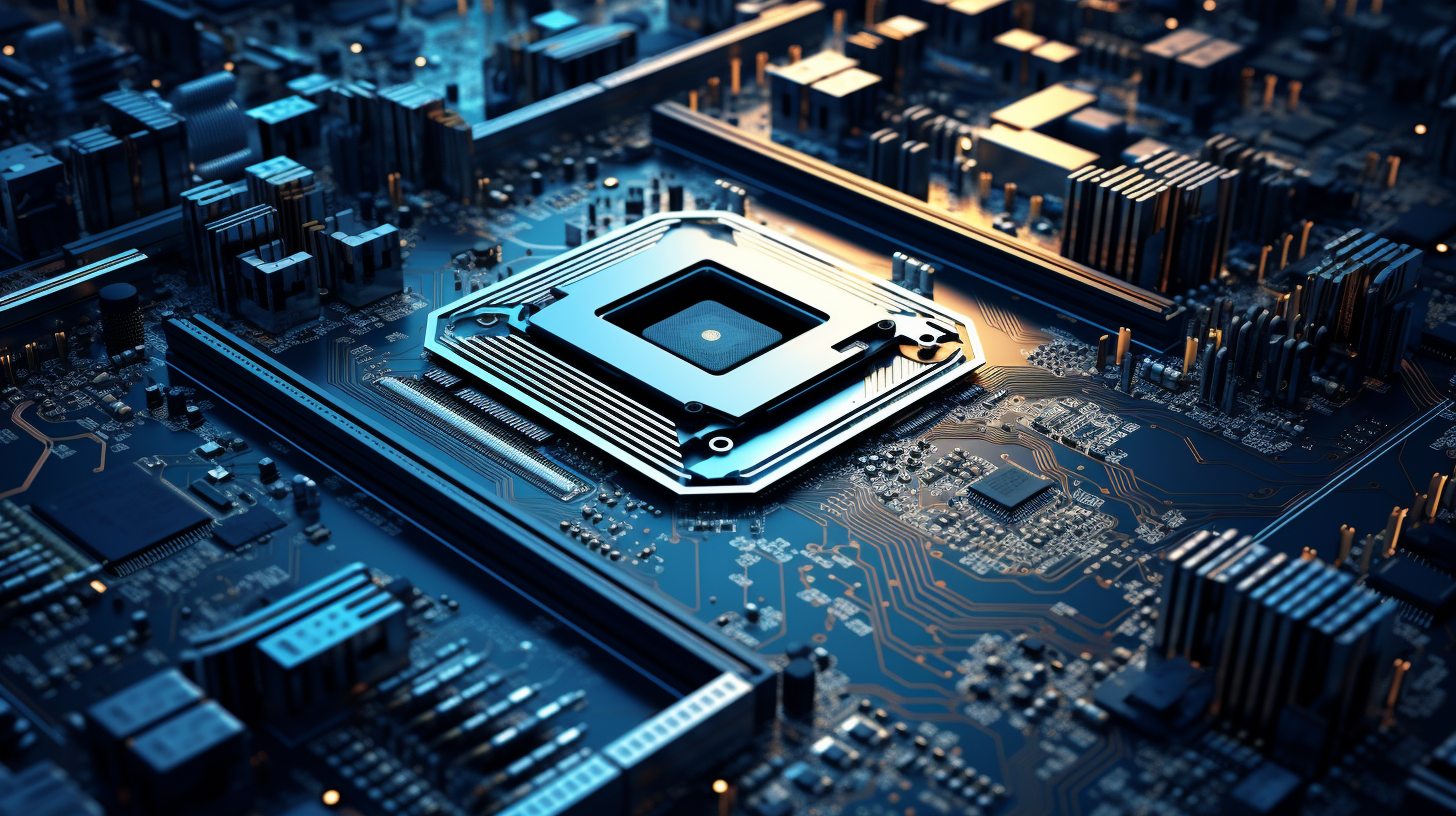
Disk Usage Analysis with Bash
Disk usage analysis is a critical aspect of system administration, allowing users to gain insights into how storage space is utilized across their files and directories. Understanding disk usage basics involves familiarizing oneself with the concepts of blocks, inodes, and file systems.
At its core, disk usage analysis revolves around the concept of blocks. A block is the smallest unit of storage that the filesystem can allocate for files. When you save a file, it may occupy one or more blocks, depending on its size. It is important to note that even if a file is smaller than a block, it will still consume an entire block’s worth of space, leading to potential inefficiencies.
Inodes, on the other hand, are data structures used by the filesystem to store information about files and directories, such as their size, ownership, permissions, and the location of the actual data blocks on the disk. Each file or directory is associated with a unique inode. Understanding inodes very important, especially on filesystems with a limited number of inodes, as exhausting inodes can prevent the creation of new files even if there is free space available.
File systems themselves dictate how data is organized and accessed on disk, with various types such as ext4, NTFS, and HFS+. Each filesystem has its own structure and characteristics, influencing performance and efficiency. Familiarity with the type of filesystem in use can help users optimize their disk usage strategies.
A useful command for getting a quick overview of disk space usage is df
. This command reports the amount of disk space used and available on filesystems. Executing
df -h
will provide a human-readable output, rendering it effortless to interpret the results.
To delve deeper into individual directory sizes, the du
command is invaluable. This command summarizes disk usage for files and directories, so that you can pinpoint which areas are consuming the most space. Running
du -sh *
within a directory will display the size of each item in a concise format.
In summary, a solid grasp of disk usage basics—blocks, inodes, and filesystems—paired with essential commands like df
and du
, provides a robust foundation for effective disk space management. Through these tools, users can actively monitor their storage health and make informed decisions regarding their filesystem usage.
Essential Bash Commands for Disk Analysis
When it comes to analyzing disk usage effectively, several essential Bash commands come into play. Each command offers unique insights and capabilities that are indispensable for system administrators and power users alike.
The df command, short for “disk filesystem,” is often the first command users turn to for a snapshot of disk usage across all mounted filesystems. By running df -h
, you can quickly view the total space, used space, available space, and the percentage of usage in a human-readable format. This command is particularly useful for identifying filesystems that are nearing capacity.
df -h
In addition to df, the du (disk usage) command is invaluable for drilling down into specific directories. This command provides information on the disk space used by files and subdirectories. A common usage is du -sh *
, which gives a summary of the sizes of all items in the current directory. The -s
flag provides a summary, while -h
makes the output human-readable.
du -sh *
For a more thorough analysis, the du
command can be combined with the sort command to identify which files or directories are the largest. For instance, you can run du -ah . | sort -rh | head -n 10
to list the top ten largest files and directories in the current directory. This combination is powerful for quickly locating space hogs in your filesystem.
du -ah . | sort -rh | head -n 10
Another essential command is find, which can be used in conjunction with du
to locate and analyze large files specifically. For example, find . -type f -size +100M
will search for files larger than 100 MB in the current directory and its subdirectories. This command is useful for identifying files that could be candidates for deletion or archiving.
find . -type f -size +100M
Additionally, the ncdu (NCurses Disk Usage) command provides a more interactive way to visualize disk usage in a directory. Unlike du
, which outputs plain text, ncdu
presents a navigable interface that lets you explore your directory sizes and delete files or directories directly from the interface. To use it, simply run ncdu
followed by the directory path you want to analyze.
ncdu /path/to/directory
Finally, the ls command can also aid in disk analysis by listing files with their sizes. Using ls -lhS
will display files in the current directory sorted by size in a human-readable format. This command is particularly useful for quickly identifying the largest files at a glance.
ls -lhS
With these essential Bash commands at your disposal, you can effectively analyze disk usage, pinpoint large files and directories, and take proactive measures to manage your disk space efficiently. Each command plays a critical role in forming a comprehensive approach to disk usage analysis, enabling users to maintain optimal storage conditions in their systems.
Automating Disk Usage Reports with Scripts
Automating disk usage reports with Bash scripts can significantly enhance your ability to monitor storage use without requiring constant manual checks. By creating simple yet powerful scripts, you can schedule regular reports that help in identifying space hogs, optimizing storage, and preventing unexpected storage shortages.
One of the foundational elements of automating disk usage reports is the use of the df
and du
commands in a Bash script. For instance, you could write a script that generates a daily report of disk usage across all mounted filesystems. Here’s a basic example:
#!/bin/bash # Script to report disk usage echo "Disk Usage Report - $(date)" echo "-----------------------------------" df -h echo echo "Directory Sizes:" du -sh * | sort -rh
This script begins by printing a header that includes the current date, followed by the output of df -h
to display disk usage for all filesystems. Then, it uses du -sh *
to list the sizes of all directories in the current directory, sorted in human-readable format. By saving this script to a file (e.g., disk_usage_report.sh
) and making it executable with chmod +x disk_usage_report.sh
, you can run it whenever needed.
To automate the execution of this script, you can utilize cron
, a time-based job scheduler in Unix-like operating systems. For example, to run this script daily at 2 AM, you can add an entry to your crontab using crontab -e
:
0 2 * * * /path/to/disk_usage_report.sh > /path/to/disk_usage.log
This line schedules the script to run at 2 AM every day and redirects the output to a log file for review. By checking this log, you can monitor disk usage trends over time and identify when and where storage issues might arise.
For a more comprehensive report that includes the largest directories, you could extend the original script. Here’s an updated version that combines the power of find
and du
to locate large files alongside directory sizes:
#!/bin/bash # Script for comprehensive disk usage report echo "Comprehensive Disk Usage Report - $(date)" echo "-------------------------------------------" echo "Overall Disk Usage:" df -h echo echo "Top 10 Largest Directories:" du -ah . | sort -rh | head -n 10 echo echo "Files larger than 100MB:" find . -type f -size +100M -exec ls -lh {} ; | awk '{ printf "%s %sn", $9, $5 }'
This enhanced script not only reports overall disk usage but also lists the ten largest directories and identifies files larger than 100 MB, providing a detailed view of your storage landscape. Each of these script components can be tailored to your specific needs, making it simple to adapt them as your disk usage analysis requirements evolve.
By using the power of Bash scripting, you can proactively manage disk usage, automate reports, and ensure that your systems remain healthy and performant. Such automation not only saves time but also helps to maintain an optimal filesystem environment, enabling you to focus on other critical tasks.
Visualizing Disk Usage with Graphical Tools
Visualizing disk usage effectively is essential for understanding how storage is utilized on your system. While command-line tools provide powerful insights into disk usage, graphical tools can offer a more intuitive way of interpreting this data. In this section, we will explore some graphical tools that can enhance your ability to visualize disk usage, making the task not only easier but also more engaging.
One of the most popular graphical tools for disk usage visualization is baobab, also known as Disk Usage Analyzer. This tool comes with many Linux distributions, including GNOME desktop environments. It provides a simpler interface that displays disk usage as a series of concentric rings or a treemap, so that you can quickly spot which directories are consuming the most space. To install baobab on a Debian-based system, you can use:
sudo apt install baobab
After installation, simply launch it from your applications menu. You can analyze specific folders or entire filesystems, and the visual representation helps you make quick decisions about where to free up space.
Another excellent graphical option is ncdu, which combines the simplicity of command-line tools with an interactive interface. While ncdu primarily runs in the terminal, it offers a much more uncomplicated to manage way to navigate through your directories. You can install ncdu using:
sudo apt install ncdu
To analyze a directory, run:
ncdu /path/to/directory
As you navigate through the directory structure, you will see the sizes of files and subdirectories in real time, so that you can identify space-hogging directories quickly. Additionally, you can delete files directly from the ncdu interface, making it a powerful tool for managing disk space.
For those using a graphical desktop environment on Linux, Filelight is another noteworthy tool that provides a beautiful representation of disk usage in the form of a pie chart. Each slice of the pie represents a directory, and hovering over a slice reveals more information about the files contained within. To install Filelight, use:
sudo apt install filelight
Once installed, launch Filelight and select the directory you wish to analyze. The graphical representation makes it easy to visualize which folders are taking up the most space, allowing for informed decision-making regarding file management.
For users who prefer web-based interfaces, DiskUsage is a handy tool that can provide a web interface for visualizing disk usage. DiskUsage can be deployed easily on a server and accessed through a browser. The setup typically involves cloning the repository and running it on a web server. You can find the installation instructions on its GitHub page.
Regardless of the tool you choose, visualizing disk usage through graphical interfaces drastically improves the user experience compared to command-line tools alone. The ability to see disk usage represented visually helps users grasp complex data quickly and identify areas that require attention. By integrating these graphical tools into your disk usage management strategy, you can maintain a healthy filesystem and ensure effective use of storage resources.
Best Practices for Disk Space Management
Effective disk space management especially important for maintaining system performance and ensuring that your applications run smoothly. Here are some best practices that can help you manage disk space more effectively, using both command-line tools and strategic organization.
Regular Monitoring: One of the simplest yet most effective practices is to regularly monitor your disk usage. Schedule periodic checks using commands like df -h
and du -sh *
. Regular monitoring helps you stay aware of your storage usage and can prevent unexpected shortages.
df -h du -sh *
Identify and Remove Unnecessary Files: Analyzing your disk for large and unneeded files is essential. Use the find
command to locate files that haven’t been accessed in a long time or to identify large files that are candidates for deletion. For example, you can find files not accessed in the last year with:
find /path/to/directory -type f -atime +365
This command will list files that haven’t been accessed for over a year, which will allow you to decide what to keep and what to delete. Additionally, using du
combined with sort
can help spot large files quickly:
du -ah /path/to/directory | sort -rh | head -n 10
Implement a File Organization Strategy: A well-structured directory hierarchy can also aid in efficient disk space management. Organizing files into categories and using meaningful naming conventions makes it easier to locate and manage files, thereby reducing the chances of accumulating unnecessary data.
Utilize Disk Quotas: If you are managing a multi-user environment, consider implementing disk quotas. Disk quotas allow you to limit the amount of disk space a user or group can consume, thus preventing any single user from monopolizing disk resources. The quota
command can be used to check user quotas, while you can set them up in the filesystem configuration.
quota -u username
Regularly Clean Temporary Files: System and application temporary files can accumulate over time and consume a significant amount of disk space. Using commands like rm
to delete temporary files from directories such as /tmp
and /var/tmp
can help in reclaiming space. Automating this process with cron jobs will ensure that temporary files are regularly cleaned up:
0 3 * * * rm -rf /tmp/*
Backup and Archive: Regularly backing up and archiving older files can free up disk space. Consider using tools like tar
to compress and archive old data that you don’t need immediate access to but must keep for future reference.
tar -czvf archive.tar.gz /path/to/old/data
Leverage Cloud Storage: For files that are not frequently accessed, think using cloud storage solutions. This can significantly reduce local disk usage while still providing access to your data when needed.
By implementing these best practices for disk space management, you can maintain a clean, efficient system that performs optimally and minimizes the risk of running out of storage space. Remember, proactive management is key to long-term systems health.