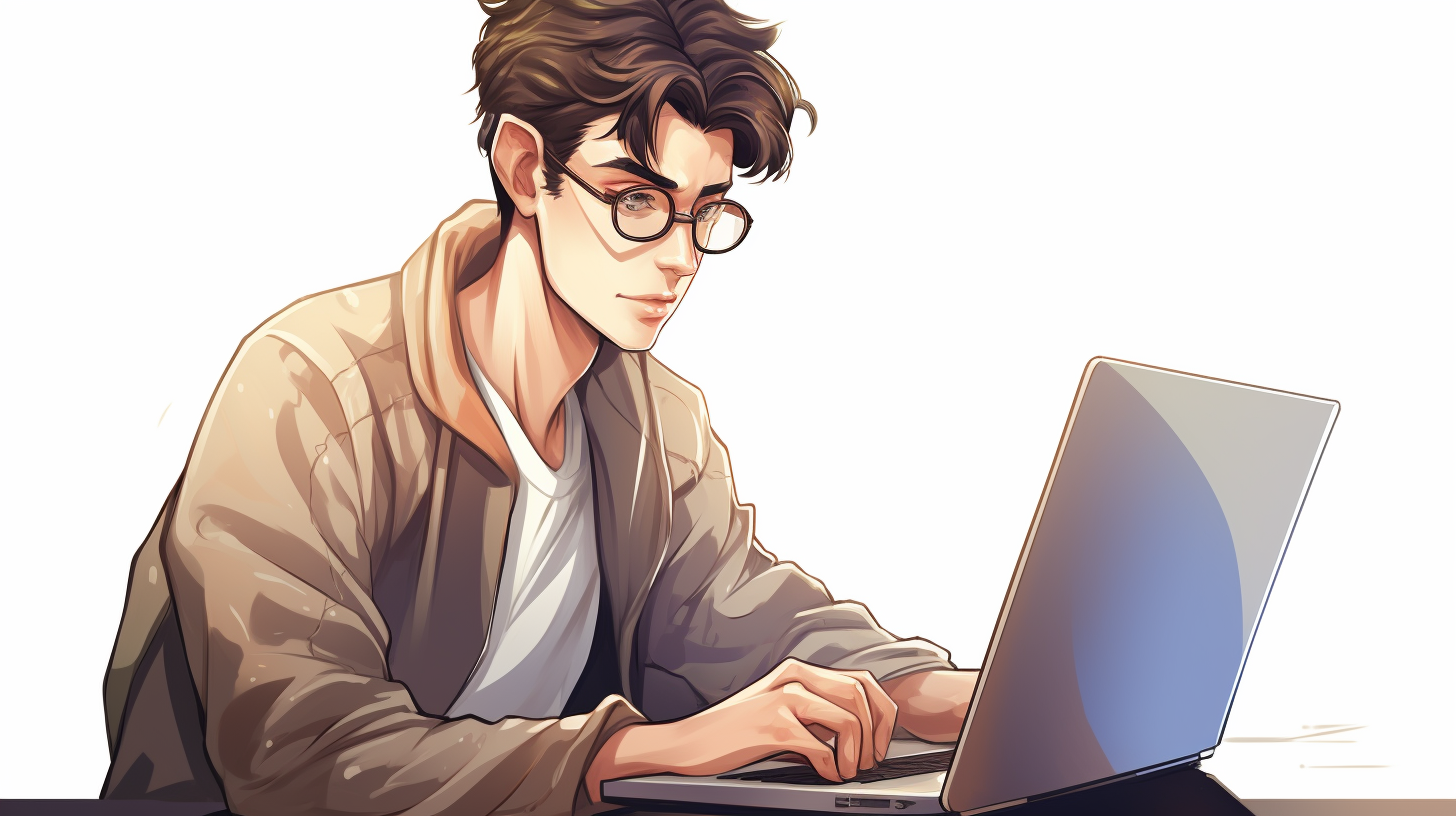
Efficient Paging with SQL OFFSET and FETCH
When working with large datasets in SQL, retrieving a subset of rows efficiently becomes an important task. The SQL clauses OFFSET and FETCH offer a powerful way to achieve pagination, which will allow you to control which rows are returned by your queries.
The OFFSET clause specifies the number of rows to skip before starting to return rows from the query. That is particularly useful when you want to skip a certain number of records, such as when you are displaying pages of results. The FETCH clause then specifies how many rows to return after the OFFSET.
The general syntax for using OFFSET and FETCH looks like this:
SELECT column1, column2 FROM TableName ORDER BY column1 OFFSET @Offset ROWS FETCH NEXT @Fetch ROWS ONLY;
In this example:
column1, column2
are the columns you want to retrieve.TableName
is the name of the table from which you are pulling data.ORDER BY
is essential as OFFSET and FETCH require an ordered result set.@Offset
is a variable that represents the number of rows to skip.@Fetch
is a variable that indicates how many rows to retrieve.
For example, if you want to display results starting from the 11th row, retrieving 10 rows at a time, your SQL query would look like this:
DECLARE @Offset INT = 10; DECLARE @Fetch INT = 10; SELECT column1, column2 FROM TableName ORDER BY column1 OFFSET @Offset ROWS FETCH NEXT @Fetch ROWS ONLY;
In this case, the first ten rows are skipped, and the next ten rows are returned based on the specified ordering. This allows you to implement paging efficiently.
One of the key advantages of using OFFSET and FETCH is their compatibility with other SQL functionality. You can combine them with joins, complex conditions, and other SQL clauses without losing the ability to paginate results effectively. However, it’s essential to note that OFFSET and FETCH are supported in SQL Server 2012 and later versions, as well as other modern SQL databases.
Understanding how to use OFFSET and FETCH not only simplifies the process of paging but also enhances the performance of your applications by allowing for more efficient queries on large datasets. As you explore these clauses further, you’ll discover how they can be seamlessly integrated into your SQL querying practices.
Benefits of Using OFFSET and FETCH for Paging
One of the primary benefits of using OFFSET and FETCH for paging is their ability to reduce memory consumption and improve response times when dealing with large datasets. By which will allow you to fetch only a subset of rows at a time, these clauses enable your application to be more efficient and responsive, particularly in web applications where user experience is paramount.
When implementing paging, traditional methods often involve retrieving all relevant data and then filtering it in the application layer. This can lead to unnecessary overhead, especially as the size of the dataset grows. With OFFSET and FETCH, the database engine handles the pagination internally, minimizing the amount of data transferred over the network and stored in memory. This approach is not only faster but also less resource-intensive.
Another significant advantage is the clarity and simplicity that OFFSET and FETCH bring to your SQL queries. By using these clauses, you can express complex paging logic in a simpler manner without resorting to intricate subqueries or temporary tables. This results in cleaner, more maintainable SQL code, which is easier to understand for both current and future developers working on the same codebase.
Moreover, OFFSET and FETCH provide a consistent way to implement pagination across different SQL queries. This uniformity means that once you familiarize yourself with the syntax and behavior of these clauses, you can apply them seamlessly across various queries and applications. By standardizing pagination, you also reduce the risk of bugs and inconsistencies that can arise from using different methods in different parts of your application.
To illustrate this benefit further, consider a scenario where you have multiple endpoints in a web API, each requiring paginated data. Using OFFSET and FETCH, you can construct each SQL query in a similar manner, leading to less cognitive load and easier debugging:
SELECT column1, column2 FROM TableName ORDER BY column1 OFFSET @Offset ROWS FETCH NEXT @Fetch ROWS ONLY;
This simplicity is not just about aesthetics; it directly impacts development speed and reduces the potential for human error. Developers can quickly grasp how pagination is handled throughout the application, fostering better collaboration and code reviews.
Additionally, OFFSET and FETCH can leverage existing indexing strategies to enhance performance further. When combined with a well-designed indexing scheme, the database can efficiently navigate to the desired starting point of the results, thus optimizing the execution time of your queries. This means that as your application scales, the efficiency provided by OFFSET and FETCH will continue to serve you well.
The benefits of using OFFSET and FETCH for paging are manifold: they provide efficient data retrieval, simplify query structure, promote consistency across your SQL code, and optimize performance through effective use of resources. By embracing these features, you can ensure that your applications are both performant and maintainable, making for a better experience for users and developers alike.
Implementing Paging in SQL Queries
Implementing paging in SQL queries is a simpler process once you’re familiar with the OFFSET and FETCH clauses. The key to effective paging lies in ensuring that you write your queries in a way that optimally retrieves only the rows you need, while also maintaining clarity and performance.
To implement paging, you must first decide on the ordering of your data. The ORDER BY clause is essential because OFFSET and FETCH require a defined order to determine which rows to skip and which to return. Without a proper order, the results can be unpredictable, leading to a poor user experience.
Here’s a basic example of a paginated query that retrieves data about products from a Product table, returning a specific page of results:
DECLARE @PageNumber INT = 2; -- Current page number DECLARE @PageSize INT = 10; -- Number of records per page DECLARE @Offset INT = (@PageNumber - 1) * @PageSize; -- Calculate offset SELECT ProductID, ProductName, Price FROM Products ORDER BY ProductName OFFSET @Offset ROWS FETCH NEXT @PageSize ROWS ONLY;
In this example, the query calculates the offset based on the current page number and page size. For the second page, with a page size of 10, the offset would be 10, meaning the first 10 records are skipped, and the next 10 records are returned. This pattern allows users to navigate through pages of results seamlessly.
It’s worth noting that the choice of columns in the ORDER BY clause can significantly affect the performance of your pagination. Ideally, you should order by indexed columns to take advantage of existing indexes, which can drastically reduce the query execution time.
When implementing paging, consider scenarios where the underlying data might change between requests. If new rows are added or existing rows are deleted, the order of results may shift, leading to inconsistencies in paging. A practical approach is to ensure that your ORDER BY clause includes a unique identifier, such as a primary key, to maintain a stable sort order.
SELECT ProductID, ProductName, Price FROM Products ORDER BY ProductID, ProductName OFFSET @Offset ROWS FETCH NEXT @PageSize ROWS ONLY;
In this revised example, ordering by ProductID first guarantees that each page of results will return the same set of rows, assuming no changes to the data occur between requests. This approach helps mitigate issues with duplicate records or missing entries as users paginate through the dataset.
In addition to the basic implementation, you might want to improve the user experience further by adding options for sorting and filtering. By allowing users to specify their preferences, you can create more dynamic and responsive applications. Here’s a simple example that includes filtering:
DECLARE @CategoryID INT = 1; -- Filter by category DECLARE @PageNumber INT = 2; DECLARE @PageSize INT = 10; DECLARE @Offset INT = (@PageNumber - 1) * @PageSize; SELECT ProductID, ProductName, Price FROM Products WHERE CategoryID = @CategoryID ORDER BY ProductName OFFSET @Offset ROWS FETCH NEXT @PageSize ROWS ONLY;
This query filters the results based on the specified CategoryID while implementing paging, enabling users to view products from a specific category without loading unnecessary data. By carefully structuring your SQL queries with OFFSET and FETCH, you can build efficient and simple to operate applications that scale gracefully as your data grows.
Best Practices for Efficient Paging
When implementing paging in SQL queries, it’s essential to adopt best practices that not only enhance performance but also ensure the clarity and maintainability of your code. Here are some of the key practices that can help achieve efficient paging using OFFSET and FETCH.
1. Use Indexed Columns in ORDER BY: The performance of your paginated queries heavily relies on the columns used in the ORDER BY clause. Always prefer indexed columns to optimize retrieval speeds. If you sort by columns that are not indexed, the database has to scan the entire dataset, which can drastically slow down the paging process. For example:
SELECT column1, column2 FROM TableName ORDER BY indexedColumn OFFSET @Offset ROWS FETCH NEXT @Fetch ROWS ONLY;
2. Include a Unique Identifier in ORDER BY: As previously mentioned, including a unique identifier (like a primary key) in your ORDER BY clause is important. This practice ensures that even if the data changes between requests, the result set remains consistent. That’s particularly important in environments where data is frequently modified:
SELECT column1, column2 FROM TableName ORDER BY uniqueID, column1 OFFSET @Offset ROWS FETCH NEXT @Fetch ROWS ONLY;
3. Calculate Offset Dynamically: In applications that support user interaction, dynamically calculating the offset based on user input (such as current page number) ensures flexibility and adaptability in your queries. This method allows easy navigation through pages, accommodating different user preferences:
DECLARE @PageNumber INT = 3; -- User-selected page DECLARE @PageSize INT = 10; -- Number of records per page DECLARE @Offset INT = (@PageNumber - 1) * @PageSize; SELECT column1, column2 FROM TableName ORDER BY column1 OFFSET @Offset ROWS FETCH NEXT @PageSize ROWS ONLY;
4. Avoiding Large Offsets: While OFFSET and FETCH are incredibly useful, using a large OFFSET value can lead to performance degradation, as the database still processes rows that are skipped. Instead of relying solely on OFFSET for deeper pages, consider alternative approaches like keyset pagination, where you remember the last record from the previous page and use that as a starting point for the next query:
DECLARE @LastID INT = 100; -- Last ID from previous page DECLARE @PageSize INT = 10; SELECT column1, column2 FROM TableName WHERE uniqueID > @LastID ORDER BY uniqueID FETCH NEXT @PageSize ROWS ONLY;
5. Set a Reasonable Page Size: Another best practice is to set a reasonable page size that balances user experience and system performance. Loading too many records concurrently can overwhelm both the server and the client, leading to slow response times. Conversely, too few records may require excessive navigation. Analyze your application’s data access patterns to determine an optimal page size.
6. Paginate at the Database Level: Whenever possible, ensure that pagination is handled at the database level rather than in the application layer. This means using OFFSET and FETCH in your SQL queries rather than retrieving all records and filtering them in your application. This practice not only decreases network load but also leverages the database’s optimized querying capabilities.
By adhering to these best practices, you can ensure that your implementation of OFFSET and FETCH remains efficient, clear, and maintainable. These strategies will help you create robust SQL queries that provide a seamless user experience while maintaining optimal performance in your applications.
Handling Edge Cases in Paging with SQL
When working with paging in SQL, it is important to ponder potential edge cases that may arise and how to handle them effectively. Edge cases can lead to unexpected behavior or performance issues, especially when the underlying data changes between paginated queries. Here are some common scenarios to watch out for and strategies to mitigate their impact.
One of the most significant challenges arises when records are added, deleted, or updated during the pagination process. For instance, if a user is viewing the first page of results and a new record is inserted, the items on the subsequent pages may shift, leading to a confusing experience as users navigate through the results. To address this, it’s advisable to include a stable ordering mechanism in your queries. Using a column with a unique constraint, like a primary key, can help maintain consistency across paginated results.
SELECT column1, column2 FROM TableName ORDER BY uniqueID, column1 OFFSET @Offset ROWS FETCH NEXT @Fetch ROWS ONLY;
In this example, ordering by a unique identifier (uniqueID) ensures that even if new rows are inserted, the relative order of existing rows remains unchanged. This practice helps prevent the “pagination shift,” where users may encounter the same record or miss records entirely as they navigate through pages.
Another edge case to ponder is the scenario where a user might request a page number that exceeds the total available pages. In such cases, returning an empty set or providing a uncomplicated to manage message can improve the experience. To implement this, you can first determine the total count of records and only allow page requests that fall within valid ranges.
DECLARE @TotalCount INT; SELECT @TotalCount = COUNT(*) FROM TableName; IF @TotalCount > 0 BEGIN DECLARE @TotalPages INT = CEILING(@TotalCount / (1.0 * @PageSize)); IF @PageNumber > @TotalPages BEGIN -- Handle case where requested page exceeds total pages PRINT 'Requested page exceeds available pages.'; END ELSE BEGIN -- Proceed with the paginated query SELECT column1, column2 FROM TableName ORDER BY uniqueID OFFSET (@PageNumber - 1) * @PageSize ROWS FETCH NEXT @PageSize ROWS ONLY; END END
This approach not only prevents unnecessary database hits for non-existent pages but also makes the application more robust against user errors.
Additionally, be aware that if your application allows users to sort data dynamically, the combination of sorting and pagination can create complexity. When users change sorting criteria, the results may differ significantly from their previous request, further complicating the navigation experience. To address this, always ensure that the pagination logic is tightly coupled with the sorting logic, preserving the integrity of both features.
DECLARE @SortColumn NVARCHAR(50) = 'column1'; -- Dynamic sorting DECLARE @SortDirection NVARCHAR(4) = 'ASC'; -- Sorting direction EXEC('SELECT column1, column2 FROM TableName ORDER BY ' + @SortColumn + ' ' + @SortDirection + ' OFFSET @Offset ROWS FETCH NEXT @PageSize ROWS ONLY;');
In this example, the dynamic SQL execution helps to maintain a consistent paging experience even when users change sort criteria. However, be cautious with dynamic SQL, as it opens up vulnerabilities for SQL injection if not handled properly.
Lastly, think implementing caching strategies for frequent queries. If your application retrieves similar pages repeatedly, caching can reduce load times and database strain. That is especially useful in read-heavy environments where data does not change frequently. By integrating caching with your pagination logic, you can enhance performance while ensuring users receive speedy responses.
To summarize, handling edge cases in paging with SQL involves understanding the implications of data changes, managing page requests intelligently, coupling sorting and pagination effectively, and using caching techniques. By anticipating these scenarios and implementing robust solutions, you can create a seamless and efficient paging experience for users, ensuring they can navigate through your data without encountering frustrating inconsistencies.