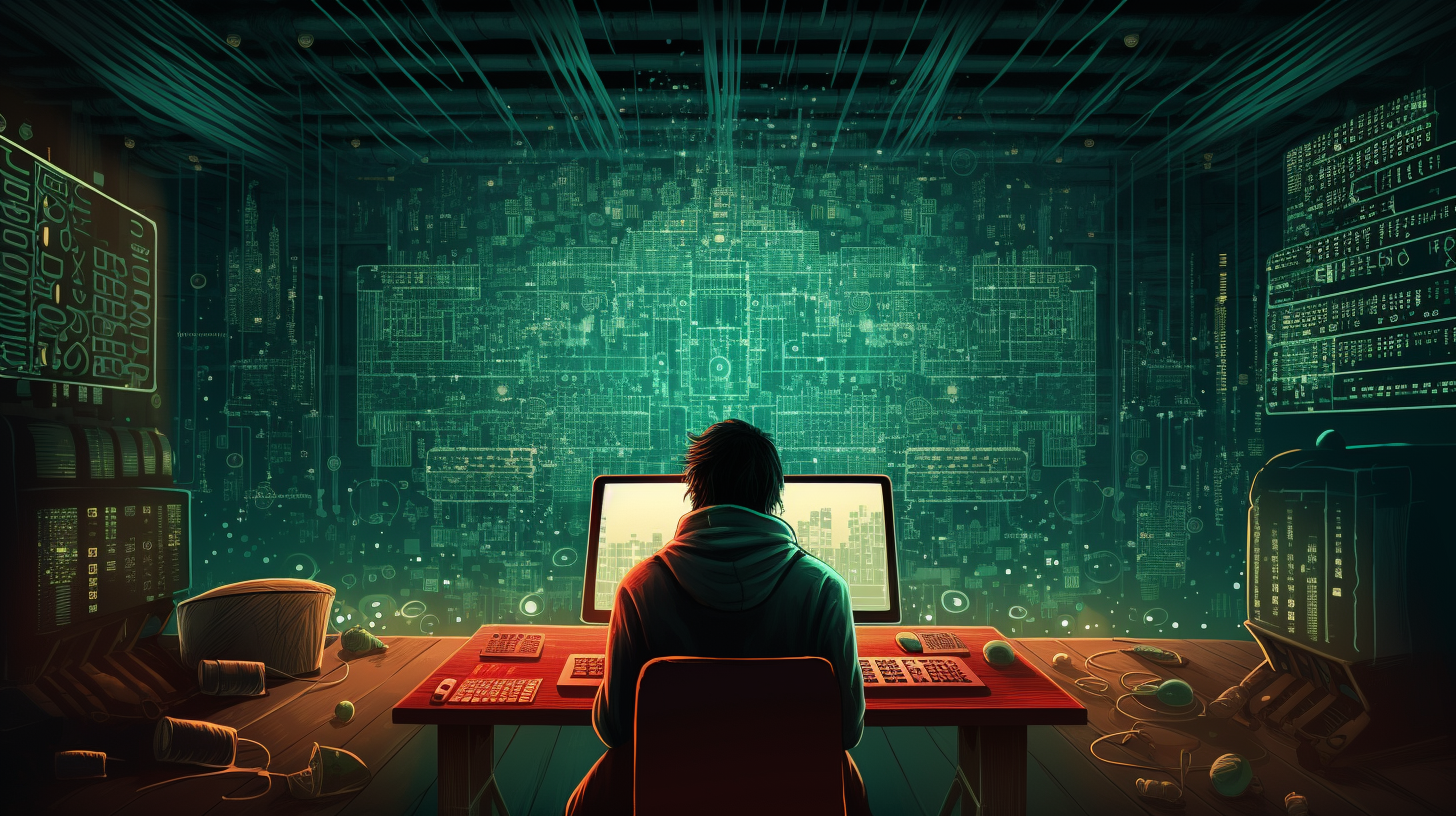
Exploring Data Types in JavaScript
JavaScript is a dynamic language that offers a rich set of data types, enabling developers to handle a wide array of programming challenges. At the core of JavaScript are seven fundamental data types, which can be categorized into two groups: primitive types and reference types.
The primitive types comprise:
- Represents both integer and floating-point numbers.
- A sequence of characters used to represent text.
- Represents a logical entity with two values:
true
andfalse
. - Indicates the absence of a value, assigned by default to uninitialized variables.
- An intentional absence of value, signifying ‘no value’ or ’empty’.
- A unique and immutable primitive value often used as an object property key.
- An arbitrary precision integer, allowing for the representation of integers larger than 253 – 1.
The reference types consist of Objects and Arrays. Unlike primitive types, which hold their value directly, reference types store a reference to the actual data.
To demonstrate these fundamental data types, ponder the following examples:
// Number let integer = 42; let float = 3.14; // String let greeting = "Hello, World!"; // Boolean let isJavaScriptFun = true; // Undefined let notAssigned; // Null let emptyValue = null; // Symbol let uniqueKey = Symbol('key'); // BigInt let bigInteger = 1234567890123456789012345678901234567890n; // Object let person = { name: "Alice", age: 30, }; // Array let fruits = ["apple", "banana", "cherry"];
Understanding these fundamental data types is essential for mastering JavaScript, as they form the foundation upon which more complex structures are built. Each data type has specific behaviors and characteristics that affect how operations are performed and how memory is managed.
Type Coercion and Type Conversion
Type coercion and type conversion are integral concepts in JavaScript that influence how data is manipulated and compared. Understanding these concepts enables developers to write more predictable and effective code. While both terms are often used interchangeably, they have distinct meanings in the context of JavaScript.
Type Coercion refers to the automatic conversion of one data type to another when performing operations. JavaScript is known for its dynamic typing, which means that it tries to make sense of the operations you are performing by implicitly converting types. This can sometimes lead to unexpected results, particularly when using the equality operator (==) versus the strict equality operator (===).
console.log(5 == "5"); // true, type coercion occurs console.log(5 === "5"); // false, no type coercion, types are different
In the first example, the number 5 is compared to the string “5”. JavaScript converts the string to a number before performing the comparison, hence it returns true. In the second example, since both the value and type must match for strict equality, it returns false.
Type coercion can also occur in various operations, such as addition and concatenation. When using the + operator, if one of the operands is a string, JavaScript converts the other operand to a string as well.
let result = 5 + "5"; // result is "55", a string let anotherResult = 5 + 5; // result is 10, a number
In the first case, the number 5 is coerced into a string, resulting in a concatenation rather than an arithmetic addition.
Type Conversion is a more deliberate process where developers manually convert a value from one type to another. JavaScript provides several functions to facilitate this process:
- The
String()
function can be used to convert any value to a string. - The
Number()
function converts a value to a number. If the conversion fails, it returns NaN (Not-a-Number). - The
Boolean()
function converts a value to a boolean, where 0, “”, null, undefined, and NaN are converted to false, and all other values are converted to true.
Ponder the following examples for type conversion:
let numStr = "123"; let num = Number(numStr); // Converts to number 123 let boolVal = Boolean(0); // Converts to false let strVal = String(123); // Converts to "123"
While type coercion can lead to implicit and sometimes unexpected behavior, type conversion allows for explicit control over how data types are handled in JavaScript. Being aware of these concepts and their implications is vital for writing robust and maintainable code.
Understanding Objects and Arrays
Objects and Arrays are fundamental structures in JavaScript, both classified as reference types. This means they store references to their values rather than the values themselves, allowing for more complex data and relationships to be represented. Objects are used to group related data and functionality, while arrays are specialized objects for managing ordered collections of data.
Objects in JavaScript are collections of key-value pairs where keys are strings (or Symbols) and values can be of any type, including other objects, functions, or arrays. This makes objects exceptionally versatile for modeling real-world entities. The syntax for creating an object is as follows:
let car = { brand: "Toyota", model: "Camry", year: 2020, start: function() { console.log("Engine started!"); } };
In this example, the car object has properties like brand
, model
, and year
, along with a method start
that defines behavior. You can access or modify these properties using either dot notation or bracket notation:
console.log(car.brand); // Output: Toyota car.year = 2021; // Updating the year console.log(car.year); // Output: 2021 car.start(); // Output: Engine started!
Arrays are a special kind of object in JavaScript designed to store ordered lists of elements. They’re zero-indexed, meaning the first element is at index 0. Arrays come with a plethora of built-in methods that facilitate tasks such as adding, removing, and manipulating elements. Creating an array is straightforward:
let colors = ["red", "green", "blue"];
To access elements in an array, use the index:
console.log(colors[0]); // Output: red colors.push("yellow"); // Adding an element console.log(colors); // Output: ["red", "green", "blue", "yellow"]
Arrays can also hold various data types, including nested arrays and objects, allowing for complex data structures:
let users = [ { name: "Alice", age: 30 }, { name: "Bob", age: 25 } ];
In this example, the users
array contains objects, each representing a user with a name and an age. You can access the properties of these objects in a similar manner:
console.log(users[0].name); // Output: Alice users[1].age += 1; // Increment Bob's age console.log(users[1].age); // Output: 26
Understanding how to work with objects and arrays very important for using the full power of JavaScript. These structures provide the needed flexibility to model complex behaviors and data relationships, creating a robust foundation for developing effective applications.
Special Data Types: null and undefined
In JavaScript, two special data types that often lead to confusion are null and undefined. Both represent the absence of a value, but they serve different purposes and have distinct behaviors that are critical to understand.
Undefined is a primitive value automatically assigned to variables that have been declared but not yet initialized. It signifies that a variable exists in the environment but has not been assigned any meaningful value. For example:
let uninitializedVariable; // Declared but not initialized console.log(uninitializedVariable); // Output: undefined
In this case, the variable uninitializedVariable
is defined in the scope but lacks a value, which is why it prints undefined
when logged to the console. Additionally, functions that do not explicitly return a value will also return undefined
:
function noReturnValue() {} console.log(noReturnValue()); // Output: undefined
Null, on the other hand, is an intentional assignment that represents the absence of any object value. It’s typically used to indicate that a variable should hold no value or that a property is temporarily non-existent. In essence, null
is a signal that a variable is deliberately empty:
let emptyValue = null; // Explicitly set to null console.log(emptyValue); // Output: null
When comparing null
and undefined
, they’re loosely equal (using ==) but not strictly equal (using ===). This can lead to subtle bugs if not carefully handled:
console.log(null == undefined); // Output: true console.log(null === undefined); // Output: false
These comparisons highlight the importance of understanding the distinction between the two. While both indicate a lack of value, undefined
signifies an uninitialized state, whereas null
is a deliberate assignment. This distinction plays an important role in debugging and writing clear, maintainable code.
Another key aspect of null
and undefined
is their behavior in type coercion. When performing operations, JavaScript applies its coercion rules, interpreting null
as a falsy value while also treating undefined
as falsy. However, their treatment can vary depending on the context:
let values = [null, undefined, 0, "", false]; values.forEach(value => { console.log(value ? "Truthy" : "Falsy"); // All print "Falsy" });
This example demonstrates that both null
and undefined
evaluate to false
in a boolean context. Yet, knowing their differences allows developers to make more informed decisions about how to handle variables and their states throughout their code.
In summary, while both null
and undefined
signify the absence of a value, they serve different roles within the language. Understanding these roles allows for better code clarity and helps avoid pitfalls associated with their implicit behaviors.
Using the typeof Operator and instanceof
The typeof operator in JavaScript is a powerful tool for determining the type of a given variable. It returns a string that indicates the type of the unevaluated operand. This can be particularly useful for debugging and conditional logic, allowing developers to make informed decisions based on the type of data they are working with. The syntax is straightforward:
typeof variableName
Here’s how typeof behaves with different data types:
console.log(typeof 42); // "number" console.log(typeof "Hello"); // "string" console.log(typeof true); // "boolean" console.log(typeof undefined); // "undefined" console.log(typeof null); // "object" (that is a known quirk in JavaScript) console.log(typeof Symbol("id")); // "symbol" console.log(typeof BigInt(123)); // "bigint" console.log(typeof {}); // "object" console.log(typeof []); // "object" (arrays are also objects) console.log(typeof function(){}); // "function"
As seen above, the typeof operator is not without its peculiarities. Notably, it returns “object” for both objects and arrays, which can be misleading. To differentiate between the two, developers often resort to additional checks or methods.
The instanceof operator serves as another important tool for type checking in JavaScript. It determines whether an object is an instance of a particular constructor or class. The syntax for using instanceof is as follows:
object instanceof Constructor
Ponder the following examples that demonstrate its use:
let arr = [1, 2, 3]; console.log(arr instanceof Array); // true console.log(arr instanceof Object); // true console.log(arr instanceof Date); // false let date = new Date(); console.log(date instanceof Date); // true console.log(date instanceof Object); // true console.log(date instanceof Array); // false
As demonstrated, instanceof is particularly effective for distinguishing between instances of different types, such as differentiating an Array from a generic Object. This capability becomes essential when dealing with complex data structures and ensuring that the right operations are applied to the right types.
Both typeof and instanceof play vital roles in a developer’s toolkit, enabling clearer code that is resilient against type-related bugs. By using these operators, developers can create more robust and maintainable applications, enhancing their ability to manage dynamic and diverse data types effectively.