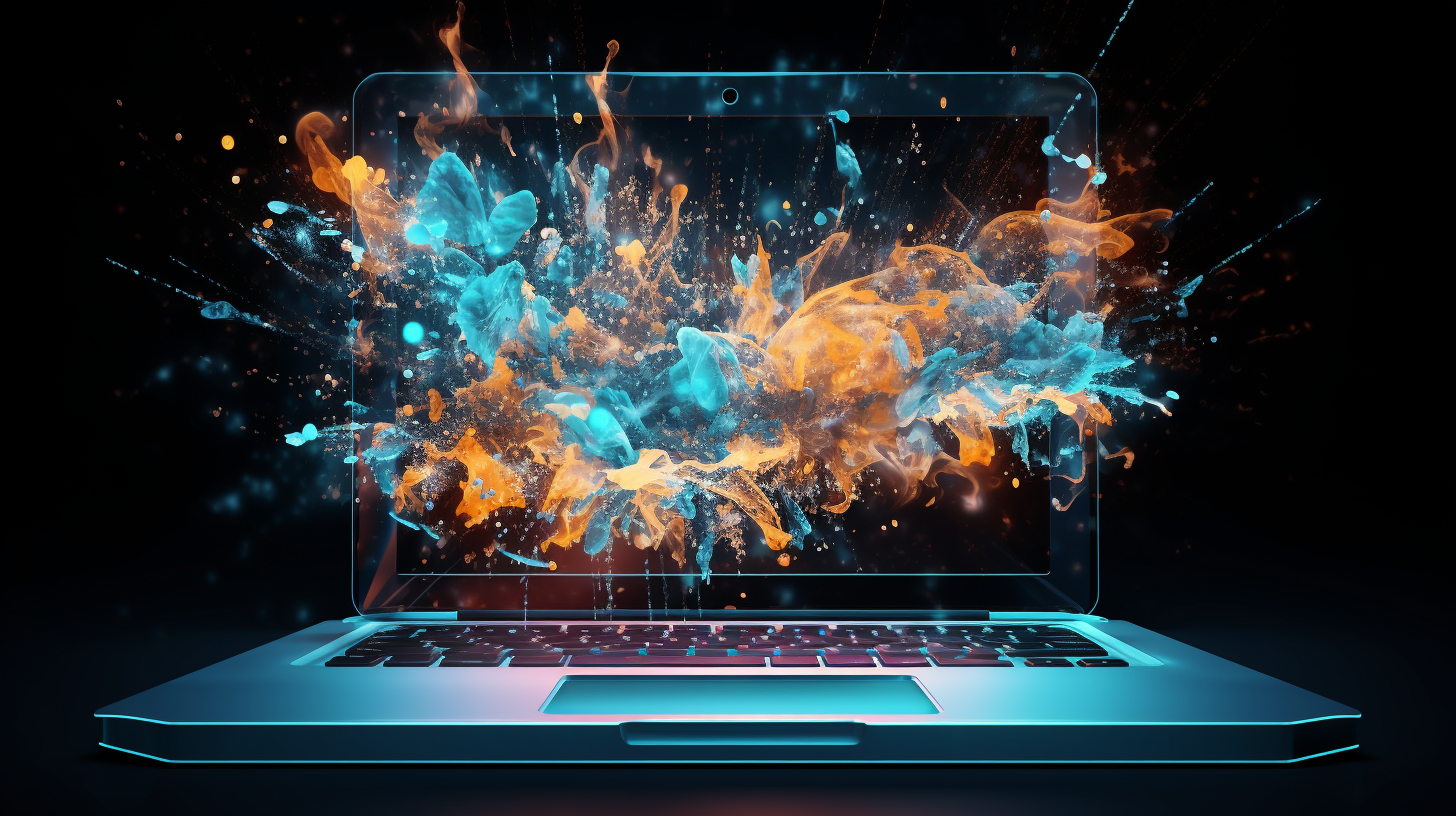
File Compression and Archiving in Bash
File compression is an important technique that reduces the size of files, making them easier to store and transfer. Understanding the underlying principles of compression can significantly enhance your efficiency when managing large datasets or preparing files for distribution. At its core, file compression works by eliminating redundancy within the data. This can be achieved through various algorithms, all of which aim to represent the original data in a more compact form.
Two primary techniques dominate the landscape of file compression: lossless compression and lossy compression. Lossless compression allows the original data to be perfectly reconstructed from the compressed data. That is essential for text files, executable files, and any data where precision especially important. Common lossless algorithms include gzip, bzip2, and zip.
In contrast, lossy compression sacrifices some data fidelity for a more significant reduction in file size. This approach is typically applied to media files, such as images, audio, and video, where a perfect reproduction is less critical. Formats like JPEG for images and MP3 for audio exemplify lossy compression techniques.
Compression algorithms can be categorized into two groups: dictionary-based algorithms and transform-based algorithms. Dictionary-based methods replace repeated occurrences of data with shorter representations from a dynamically generated dictionary. The widely-used DEFLATE algorithm combines this technique with Huffman coding to achieve effective compression ratios.
Transform-based algorithms, on the other hand, transform the data into a different format or domain, allowing for more efficient representation. The Discrete Cosine Transform (DCT) is a classic example, widely employed in image compression.
Ultimately, the choice between these techniques hinges on the nature of the data and the specific requirements for fidelity and file size. In a Bash environment, using the right tools to apply these compression methods effectively can streamline workflow and enhance data management strategies.
Popular Compression Tools in Bash
In the sphere of file compression within the Bash environment, several robust tools stand out, each with its unique features and advantages. Understanding these tools is essential for any Bash programmer or system administrator aiming to optimize their file management processes.
gzip is perhaps the most widely recognized compression tool in the Unix and Linux ecosystems. It focuses on lossless compression and is particularly effective for compressing single files. The syntax is simpler, and its usage can be summarized as follows:
gzip filename.txt
This command replaces the original file with a compressed version named filename.txt.gz
. For those who wish to keep the original file intact, the -k
option can be utilized:
gzip -k filename.txt
Next in line is bzip2, which generally provides better compression rates than gzip, albeit at the cost of speed. It is particularly useful for larger files. To compress a file using bzip2, the command is:
bzip2 filename.txt
This results in a compressed file named filename.txt.bz2
. Similar to gzip, bzip2 can also preserve the original file with the -k
option:
bzip2 -k filename.txt
zip is another popular choice, especially when dealing with multiple files. Unlike gzip and bzip2, which compress only single files, zip supports archiving multiple files into a single compressed file. The basic syntax is as follows:
zip archive.zip file1.txt file2.txt
This command creates archive.zip
, containing file1.txt
and file2.txt
. To also include a directory and its contents, one can use:
zip -r archive.zip directory/
Another noteworthy tool is xz, known for its high compression ratios and speed. XZ uses the LZMA algorithm, which often yields better results than gzip and bzip2 for certain types of data. The command for compressing a file is:
xz filename.txt
This command produces a file named filename.txt.xz
. Like its counterparts, -k
can be used to keep the original:
xz -k filename.txt
For users needing to work with tarballs, the tar command deserves mention. While tar itself does not compress files, it’s often used in conjunction with compression tools like gzip or bzip2 to create compressed archives. The following command creates a compressed tarball:
tar -czf archive.tar.gz directory/
Here, the -c
option creates an archive, -z
applies gzip compression, and -f
specifies the filename of the archive.
Each of these tools has its strengths and ideal use cases, making it essential to choose the right one based on the specific requirements of the task at hand. Mastery of these tools empowers users to manage their files efficiently, enhancing both productivity and data management capabilities in the Bash environment.
Creating Compressed Archives with tar
Creating compressed archives with tar is a fundamental skill for any Bash user, especially when managing a multitude of files or directories. The tar command, short for “tape archive,” is primarily used for archiving files, but it can also be seamlessly combined with compression tools to create compact and efficient file bundles.
The basic syntax of the tar command is straightforward:
tar [options] [archive-file] [file or directory to archive]
When working with tar, the most common options include:
- Create a new archive
- Verbosely list files processed (useful for monitoring progress)
- Specify the archive file name
- Use gzip to compress the archive
- Use bzip2 to compress the archive
- Use xz to compress the archive
- Change to a directory before performing operations
A simple example of creating a compressed archive using gzip is as follows:
tar -czf archive.tar.gz directory/
This command instructs tar to:
- Create a new archive
- Compress the archive with gzip
- Write to a file named archive.tar.gz
In this example, the contents of “directory/” will be archived and compressed into a single file named “archive.tar.gz”. If you want to see what files are being archived, simply add the -v option:
tar -czvf archive.tar.gz directory/
For those looking to use bzip2 instead of gzip, the command is almost identical, just replace the -z with -j:
tar -cjf archive.tar.bz2 directory/
In this case, “archive.tar.bz2” will contain the contents of “directory/” compressed with bzip2. For maximum compression, you can employ the xz compression algorithm with the -J option:
tar -cJf archive.tar.xz directory/
Once you have your compressed archive, extracting it’s equally simpler. The -x option is used for extraction:
tar -xzf archive.tar.gz
This command extracts the contents of “archive.tar.gz” into the current directory. If you are dealing with a bzip2 compressed archive, remember to use the -j option:
tar -xjf archive.tar.bz2
For users who frequently create and extract archives, mastering tar’s various options can significantly streamline file management tasks. Incorporating tar into your workflow not only allows for efficient storage but also facilitates easier file transfers, especially when bundling multiple files and directories into a single compressed archive.
Extracting and Managing Compressed Files
Extracting and managing compressed files in a Bash environment is a critical skill that enhances your ability to handle data efficiently. Once files are compressed, the need for quick and effective extraction becomes paramount. Fortunately, Bash provides a simpler way to manage these tasks, making it simple to manipulate compressed archives.
The most common tools for extracting compressed files include gzip, bzip2, zip, and tar. Each tool has its specific commands for extraction, and understanding these commands will empower you to work with compressed files seamlessly.
For files compressed with gzip, the extraction command is simple:
gunzip filename.txt.gz
This command will decompress the file, resulting in the restoration of the original filename.txt. If you wish to retain the compressed file after extraction, you can use the -k option:
gunzip -k filename.txt.gz
When dealing with bzip2, the extraction process is similarly simpler. To decompress a file, you would execute:
bunzip2 filename.txt.bz2
Again, the original file will be restored, and to keep the compressed version, apply the -k option:
bunzip2 -k filename.txt.bz2
If you’re working with zip files, extraction can be done using the unzip command:
unzip archive.zip
This command extracts all the files contained within archive.zip to the current directory. If you need to extract to a specific directory, the -d option allows you to specify the destination:
unzip archive.zip -d /path/to/destination
For tarballs, which are archives created with the tar command, extraction is done using the -x option. Depending on the compression method used during the creation of the tarball, the commands vary:
For gzip-compressed tar files:
tar -xzf archive.tar.gz
For bzip2-compressed tar files:
tar -xjf archive.tar.bz2
And for xz-compressed tar files:
tar -xJf archive.tar.xz
When extracting files, it is also helpful to monitor the progress. By adding the -v option to the tar command, you can view a verbose output of the extraction process:
tar -xzvf archive.tar.gz
Another important aspect of managing compressed files is knowing how to navigate within the directories you are extracting to. The -C option in tar allows you to change to a directory before performing the extraction:
gunzip -k filename.txt.gz
0
Beyond simple extraction, managing compressed files involves techniques such as verifying the integrity of extracted files and cleaning up any unnecessary compressed versions post-extraction. Using commands like md5sum or sha256sum allows you to check the integrity of files after extraction, ensuring they match expected checksums.
By mastering these extraction commands and techniques, you can effectively manage compressed files in Bash, enabling you to organize, archive, and retrieve your data with confidence.
Automating Compression Tasks with Scripts
Automating compression tasks with scripts is an essential skill for any Bash user seeking to optimize their workflow and improve efficiency. The ability to automate repetitive tasks not only saves time but also minimizes the potential for human error. By incorporating file compression into scripts, users can streamline their processes and ensure consistency across operations.
To begin, let’s ponder a simple script that compresses all the text files in a specified directory using gzip. This script will iterate through each .txt file, compress it, and optionally keep the original file intact. The following example demonstrates this:
#!/bin/bash # Directory containing files DIRECTORY="/path/to/directory" # Loop through all .txt files in the directory for file in "$DIRECTORY"/*.txt; do # Check if the file exists if [[ -f "$file" ]]; then gzip -k "$file" # Compress and keep original echo "Compressed: $file" fi done
In this script, we specify the target directory and use a for loop to iterate through each .txt file. The if statement ensures that only existing files are processed, avoiding errors. Each file is then compressed with gzip, and the original is preserved using the -k option.
Now, suppose we want to create a more comprehensive script that compresses files based on their extensions, allowing users to specify which type of files to compress. This can be achieved by passing an extension as an argument to the script. Here’s how that might look:
#!/bin/bash # Check for an argument if [[ $# -ne 2 ]]; then echo "Usage: $0 " exit 1 fi DIRECTORY="$1" EXTENSION="$2" # Loop through specified files in the directory for file in "$DIRECTORY"/*."$EXTENSION"; do # Check if the file exists if [[ -f "$file" ]]; then case "$EXTENSION" in txt) gzip -k "$file" echo "Compressed: $file with gzip" ;; bz2) bzip2 -k "$file" echo "Compressed: $file with bzip2" ;; zip) zip "$file.zip" "$file" echo "Compressed: $file with zip" ;; *) echo "Unsupported file extension: $EXTENSION" ;; esac fi done
This script takes two arguments: the directory and the file extension. It performs a check to ensure that both arguments are provided. The case statement allows for different compression tools based on the file extension. This approach makes the script versatile and suitable for various file types.
In addition to compressing files, it’s often beneficial to automate the cleanup of old compressed files. A script can be created to identify and remove compressed files older than a specified number of days. This can help manage disk space effectively:
#!/bin/bash # Directory containing compressed files DIRECTORY="/path/to/directory" # Number of days DAYS=30 # Find and remove compressed files older than specified days find "$DIRECTORY" -type f -name '*.gz' -mtime +$DAYS -exec rm {} ; echo "Removed compressed files older than $DAYS days from $DIRECTORY."
In this script, the find command is employed to locate gzip files older than the specified number of days and remove them. The -exec option allows for executing the rm command on each file found, effectively cleaning up the directory.
By automating these compression tasks with Bash scripts, you can greatly enhance your file management efficiency. The ability to quickly compress, extract, and clean up files automatically ensures that your system remains organized and that your workflow is as streamlined as possible. These scripted solutions provide a foundation that can be further customized to suit specific needs, making them invaluable tools in any Bash user’s arsenal.
Best Practices for File Compression and Archiving
When it comes to file compression and archiving, adhering to best practices can significantly enhance your efficiency and minimize potential issues. These practices not only streamline the compression process but also ensure that the integrity and accessibility of data are maintained throughout. Here are some key best practices to think when working with file compression in Bash.
1. Choose the Right Compression Tool: Selecting the appropriate tool for your specific task is paramount. Different tools excel in various scenarios; for instance, use gzip for fast compression of single files, bzip2 for higher compression rates on larger files, and zip for archiving multiple files into one. Understanding the strengths and limitations of each tool will guide you in making informed decisions.
Example of using gzip:
gzip filename.txt
2. Maintain Original Files When Necessary: In many cases, preserving the original file is essential, particularly during the initial testing of compression. Tools like gzip and bzip2 provide the option to keep the original file intact using the -k flag. This practice allows you to verify the integrity and correctness of the compressed files before deleting the originals.
Example:
gzip -k filename.txt
3. Use Verbose Output for Monitoring: Enabling verbose output (using the -v option) during compression and extraction provides a clear indication of progress and success. This can be especially useful when dealing with large files or directories, as it allows you to monitor the process and troubleshoot any potential issues that arise.
Example:
tar -czvf archive.tar.gz directory/
4. Organize Compressed Files: Adopting a systematic approach to file naming and organization can save time and reduce confusion later. Use meaningful names for compressed files, include timestamps or version numbers when applicable, and maintain a consistent directory structure. This will facilitate easier access and retrieval when you need to extract files.
Example of a structured approach:
tar -czvf backups_$(date +%Y%m%d).tar.gz /path/to/data/
5. Test Compressed Files: After compression, it’s prudent to test the integrity of the compressed files. For archives created with tar, the -t option can be used to list the contents without extracting them. This ensures that the files are intact and accessible.
Example:
tar -tzf archive.tar.gz
6. Consider Automation for Regular Tasks: If you frequently perform compression tasks, think automating them with Bash scripts. This not only saves time but also ensures consistency across operations. Scripts can be designed to perform regular clean-ups, compress files based on extensions, and even log operations for future reference.
Example of a script to automate compression:
#!/bin/bash for file in *.txt; do gzip -k "$file" done
7. Monitor Disk Space and Manage Old Archives: Regularly monitor disk usage and manage old or unnecessary compressed files. Use commands like find to identify and remove archived files that are no longer needed, especially those that exceed a certain age. This practice helps maintain a clean and efficient file system.
Example of removing old compressed files:
find /path/to/compressed -type f -name '*.gz' -mtime +30 -exec rm {} ;
8. Document Your Processes: Maintain clear documentation of your file compression processes, including the tools used, commands executed, and any scripts developed. This not only aids in troubleshooting but also serves as a reference for future compression tasks or for colleagues who may encounter similar scenarios.
By implementing these best practices when compressing and archiving files in Bash, you can enhance your productivity, ensure data integrity, and maintain an organized file system. Mastery of these techniques will empower you to tackle any file management challenge with confidence.