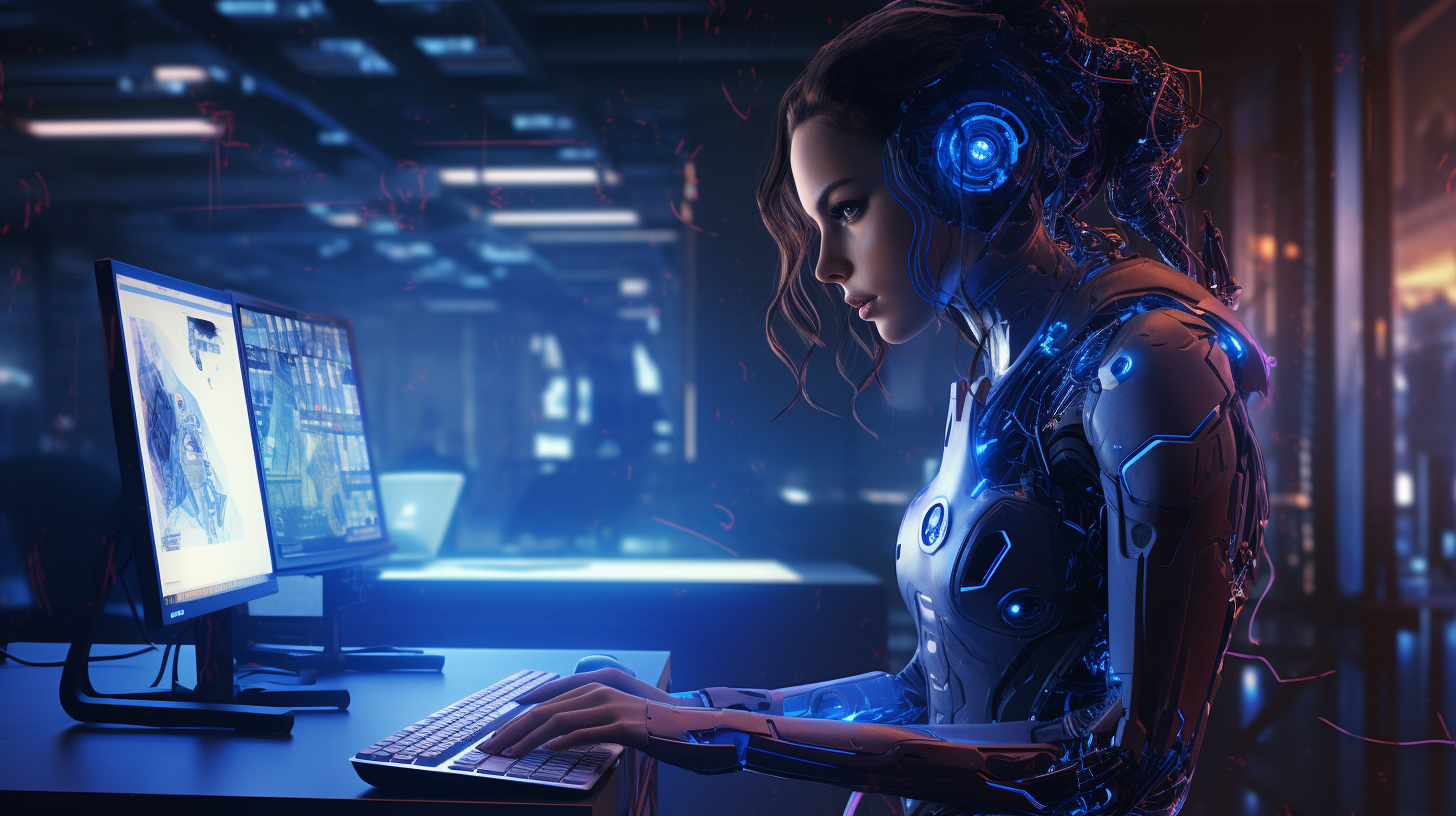
File Management with Bash
File operations in Bash are fundamental to effective file management in any Linux or Unix-like operating system. They enable users to create, move, copy, and delete files with ease. Understanding these operations can significantly enhance your command-line productivity.
To create a new file, the touch
command is often utilized. It creates an empty file if it doesn’t exist, or updates the timestamp if it does. Here’s a practical example:
touch myfile.txt
To write content to a file, you can use redirection with the echo
command:
echo "Hello, World!" > myfile.txt
This command will create myfile.txt
with the text “Hello, World!”. If the file already exists, it will be overwritten. To append text to an existing file, use >>
instead:
echo "Appending this line." >> myfile.txt
Copying files is performed with the cp
command. To make a copy of myfile.txt
named myfile_copy.txt
, use:
cp myfile.txt myfile_copy.txt
Moving (or renaming) files can be done with the mv
command. To rename myfile.txt
to renamed_file.txt
, execute:
mv myfile.txt renamed_file.txt
If you want to move a file to another directory, specify the path:
mv renamed_file.txt /path/to/destination/
Deleting files is simpler with the rm
command. Use caution, as this action is irreversible:
rm myfile_copy.txt
For removing directories, the rmdir
command is used if the directory is empty:
rmdir my_empty_directory
If the directory contains files and you want to remove it along with all its contents, combine rm
with the -r
(recursive) flag:
rm -r my_directory
Working with Directories
Working with directories in Bash is just as crucial as handling files, as directories serve as the organizational structure for your file system. Understanding how to navigate, create, remove, and manage directories will empower you to efficiently handle your project files and improve your overall workflow.
To navigate through directories, the cd
command is your primary tool. By default, you start in your home directory, but you can move to any directory by providing its path. For example, to change to a directory named Documents, execute:
cd Documents
You can also navigate up to the parent directory using:
cd ..
If you need to return to your home directory from anywhere, the cd
command without any arguments will take you back:
cd
Creating a new directory is done with the mkdir
command. For instance, to create a new directory named Projects, you would run:
mkdir Projects
If you need to create a nested directory structure, you can use the -p
option. This will create parent directories as needed. For example:
mkdir -p Projects/2023/January
To remove a directory, you can use the rmdir
command, but remember it only works on empty directories. Attempting to remove a non-empty directory will result in an error:
rmdir Projects/Old
For directories that contain files or other directories, you must use the rm
command with the -r
flag to remove it recursively. Use caution with this command, as it will permanently delete everything within the specified directory:
rm -r Projects/Old
Listing the contents of a directory is accomplished with the ls
command. It’s a versatile command with several options. To see all files, including hidden ones (those beginning with a dot), use:
ls -a
For a more detailed view, including file permissions, ownership, and timestamps, add the -l
flag:
ls -l
Combining these options gives you comprehensive information about the files and directories in your current location:
ls -la
File Permissions and Ownership
Understanding file permissions and ownership in Bash is essential for maintaining security and control over your files. In a multi-user environment, permissions dictate who can read, write, or execute a file or directory. Bash uses a permission system that can be modified for individual users and groups, allowing for a flexible approach to file management.
Each file and directory has an associated owner and group, which can be viewed by using the ls -l
command. The output displays permission settings in the following format:
-rwxr-xr-- 1 owner group size date time filename
The first character indicates the file type (a dash for a regular file, “d” for directory). The next nine characters represent the permissions, divided into three sets: user (owner), group, and others. Each set can contain:
- Read permission
- Write permission
- Execute permission
For example, rwxr-xr--
means that the owner has read, write, and execute permissions, the group has read and execute permissions, and others have only read permissions.
To change the owner of a file or directory, you can use the chown
command. For instance, to change the owner of myfile.txt
to a user named newuser
, you would execute:
chown newuser myfile.txt
If you want to change both the owner and the group, you can do so by specifying both in the command:
chown newuser:newgroup myfile.txt
To modify the permissions of a file or directory, the chmod
command is used. This command can alter permissions using either symbolic notation or octal numbers. For example, to add execute permission for the group to myfile.txt
, you could use:
chmod g+x myfile.txt
Alternatively, you can set permissions using numeric values, where r is 4, w is 2, and x is 1. To set the permissions to read and write for the owner, and read for the group and others, you would use:
chmod 644 myfile.txt
To remove a permission, you can prefix with a –. For instance, to remove write permission for others, the command is:
chmod o-w myfile.txt
Searching and Filtering Files
Searching and filtering files in Bash is a powerful technique that enhances productivity by enabling users to locate specific files or content within files quickly. With the right commands, you can efficiently sift through directories and pinpoint exactly what you need, regardless of the size of your file system.
One of the primary tools for searching files is the find command. This command allows you to search for files based on various criteria such as name, type, size, or modification date. For example, to find all files named myfile.txt in the current directory and its subdirectories, the command would be:
find . -name "myfile.txt"
Using wildcards, you can expand your search. If you want to find all text files (*.txt) in a specific directory, you can run:
find /path/to/directory -name "*.txt"
This command will traverse through the specified directory and all its subdirectories, listing every file that matches the pattern.
Another useful command is grep, which allows you to search for specific patterns within files. This is particularly useful for locating strings or keywords in large text files. For instance, if you wanted to find the term “error” in a log file named application.log, you would execute:
grep "error" application.log
You can refine your search to ignore case sensitivity by adding the -i flag:
grep -i "error" application.log
To search through multiple files, you can use wildcards. For example, to search for the term “success” in all text files within a directory, you can run:
grep "success" *.txt
If you want to display the line numbers where the matches occur, you can include the -n option:
grep -n "success" *.txt
The combination of find and grep can be even more powerful. For instance, to find all text files in a directory containing the word “important,” you can combine the two commands using a pipe:
find . -name "*.txt" | xargs grep "important"
In this example, xargs takes the output from find and passes it as arguments to grep, which will allow you to search through all found text files for the specified term.
Additionally, the locate command can be used for quick searches across your entire file system, relying on a pre-built database of files. To search for a file named report.pdf, you can simply use:
locate report.pdf
Keep in mind that the locate database may not always be up-to-date, so you can refresh it with:
sudo updatedb
Automating File Management Tasks
Automating file management tasks in Bash can dramatically streamline your workflow, saving you time and reducing the possibility of human error. By using the power of shell scripting, you can create scripts that perform repetitive tasks with just a single command. This approach not only enhances productivity but also allows you to enforce consistency across your file management operations.
One common automation task is batch renaming files. Suppose you have multiple files named file1.txt, file2.txt, and so on, and you want to rename them to document1.txt, document2.txt, etc. You can accomplish this with a simple loop in a Bash script:
for i in {1..5}; do mv "file${i}.txt" "document${i}.txt" done
This script utilizes a for loop to iterate over the numbers 1 through 5, renaming each file accordingly. Such scripts can easily be adapted to different naming conventions or file extensions.
Another frequent automation requirement is to back up important files or directories. You can create a script that performs this task, using the cp command to copy files to a backup directory. Here’s how you might structure that:
#!/bin/bash SOURCE_DIR="/path/to/source" BACKUP_DIR="/path/to/backup" DATE=$(date +%Y-%m-%d) # Create a backup with the current date cp -r "$SOURCE_DIR" "$BACKUP_DIR/backup_$DATE"
In this script, the SOURCE_DIR variable holds the path of the files you want to back up, while BACKUP_DIR specifies where the backup will be stored. The cp -r command copies the entire directory recursively, and a timestamp is added to the backup folder name for easy identification.
To further enhance your automation capabilities, you might want to incorporate cron jobs for scheduled tasks. Cron is a time-based job scheduler in Unix-like operating systems. For instance, if you want to back up your files every day at midnight, you would first create your backup script as shown above and then add it to your crontab:
crontab -e
Inside the crontab file, you would add the following line:
0 0 * * * /path/to/your/backup_script.sh
This line tells cron to execute your backup script every day at midnight. Automation via scripts and cron jobs can significantly reduce the manual effort required for regular maintenance tasks.
In addition to these tasks, you can also automate file downloads, organization, and cleanup operations. For instance, consider a scenario where you want to clean up a directory by removing files older than a certain date. You can achieve this with the find command combined with the -exec option:
find /path/to/directory -type f -mtime +30 -exec rm {} ;
This command finds all files in the specified directory that haven’t been modified in the last 30 days and deletes them. Such cleanup tasks are essential for maintaining an organized file system, and automating them ensures they are performed consistently.
Finally, when developing your automation scripts, remember to include error handling to manage unexpected outcomes gracefully. For instance, you can check if a directory exists before trying to copy files into it:
if [ ! -d "$BACKUP_DIR" ]; then mkdir -p "$BACKUP_DIR" fi